Zig for backend development
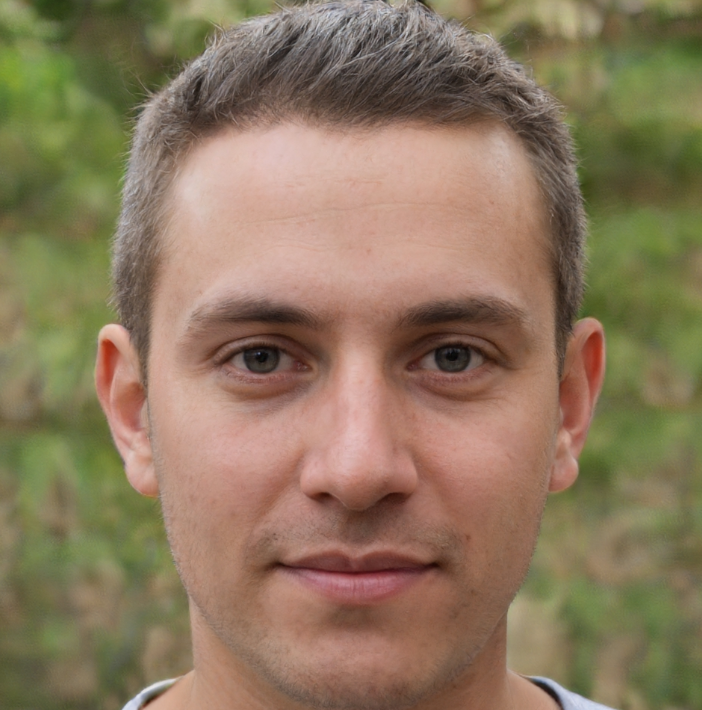
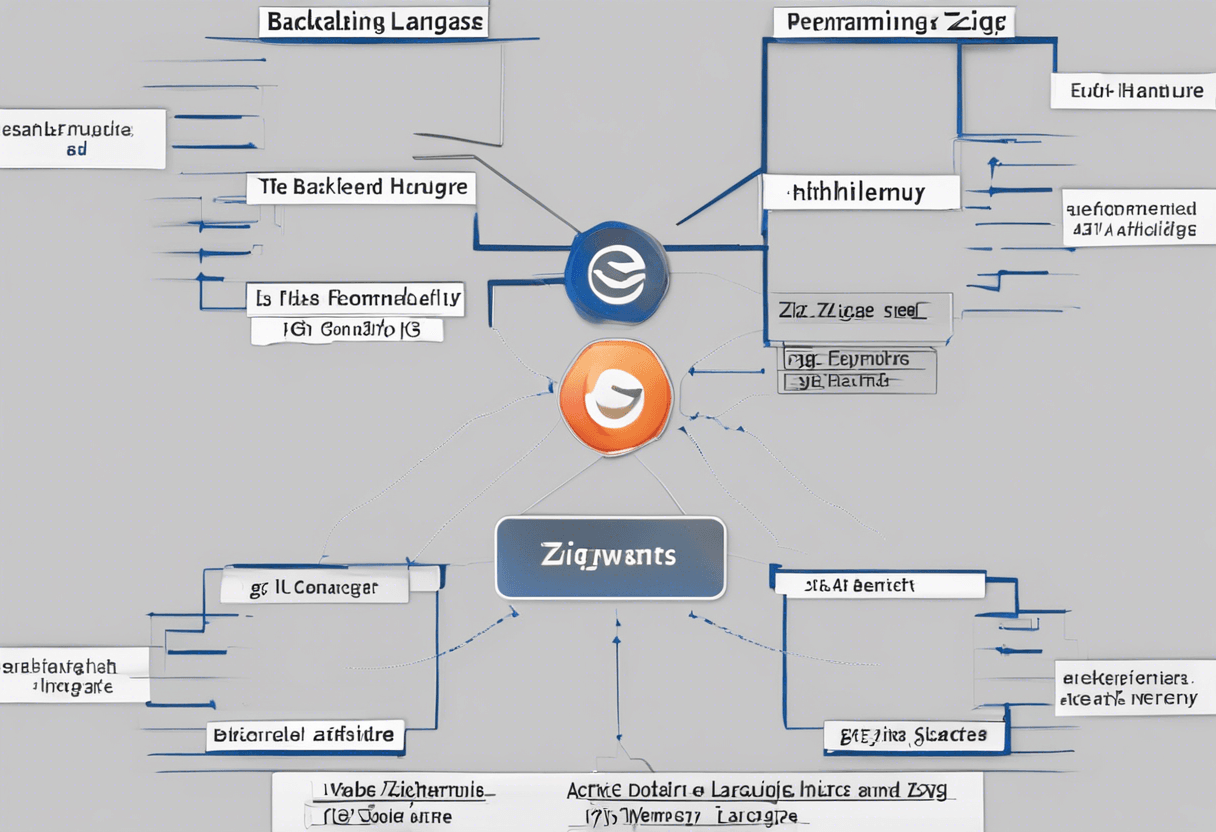
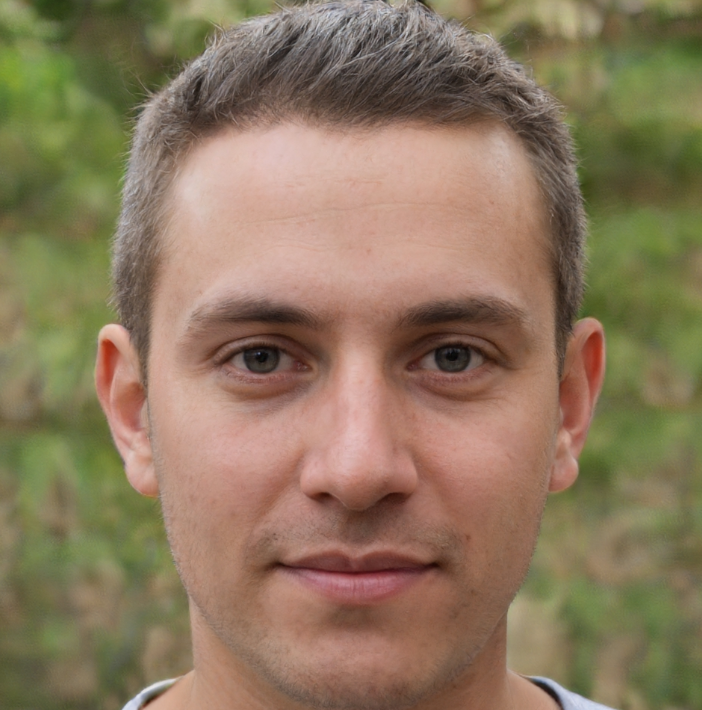
Zig: A Promising Language for Backend Development
=====================================================
The world of backend development is constantly evolving, with new languages and frameworks emerging to challenge the status quo. One such language that has been gaining attention in recent years is Zig. Developed by Andrew Kelley, Zig is a general-purpose programming language that aims to provide a robust, efficient, and safe alternative to traditional backend development languages like C and C++. In this article, we'll explore the features and benefits of using Zig for backend development, and examine its potential as a viable option for building scalable and maintainable server-side applications.
A Brief History of Zig
Zig was first announced in 2015, with the goal of creating a language that could provide a balance between performance, safety, and ease of use. Since then, the language has undergone significant development, with a growing community of contributors and users. In 2019, Zig reached version 0.4.0, which marked a major milestone in its development. Today, Zig is used in a variety of applications, from systems programming to game development.
Key Features of Zig
So what makes Zig an attractive choice for backend development? Here are some of the key features that set it apart from other languages:
Performance
Zig is designed to be a high-performance language, with a focus on generating efficient machine code. Its compiler is capable of producing code that is comparable to, or even surpasses, the performance of C and C++. This makes Zig an excellent choice for applications that require low latency and high throughput.
Memory Safety
One of the biggest advantages of Zig is its focus on memory safety. The language provides a number of features that help prevent common errors like null pointer dereferences and buffer overflows. This includes a robust type system, bounds checking, and a borrow checker that ensures memory is accessed safely.
Error Handling
Zig has a unique approach to error handling, which is based on the concept of "error sets." Error sets allow developers to define a set of possible errors that can occur in a function, and provide a way to handle those errors in a explicit and safe manner. This approach helps to prevent errors from being ignored or propagated, making it easier to write robust and reliable code.
Concurrency
Zig provides a number of features that make it easy to write concurrent code. This includes support for coroutines, which allow developers to write asynchronous code that is efficient and easy to reason about. Zig also provides a number of synchronization primitives, such as mutexes and semaphores, that make it easy to coordinate access to shared resources.
Building a Backend Application with Zig
So how does Zig fare in practice? To find out, let's build a simple backend application using Zig. We'll create a RESTful API that allows users to create, read, update, and delete (CRUD) blog posts.
Setting up the Project
To start, we'll create a new Zig project using the zig init
command. This will create a basic directory structure and a build.zig
file that we can use to configure our project.
$ zig init
Next, we'll add the necessary dependencies to our build.zig
file. For this example, we'll use the zig-http
library to handle HTTP requests and responses.
const std = @import("std");
pub fn build(b: *std.build.Builder) void {
const target = b.standardTargetOptions(.{});
const mode = b.standardReleaseOptions();
const exe = b.addExecutable(.{
.name = "blog_api",
.root_source_file = .{ .path = "src/main.zig" },
.target = target,
.optimize = mode,
});
exe.linkLibC();
exe.linkSystemLibraryName("zig-http");
const run_cmd = exe.run();
run_cmd.step.dependOn(b.getInstallStep());
const run_step = b.step("run", "Run the app");
run_step.dependOn(&run_cmd.step);
}
Defining the API
Next, we'll define the API endpoints for our blog application. We'll create a BlogPost
struct to represent individual blog posts, and a BlogApi
struct to handle API requests.
const std = @import("std");
pub const BlogPost = struct {
id: u32,
title: []u8,
content: []u8,
};
pub const BlogApi = struct {
posts: std.ArrayList(BlogPost),
pub fn init(allocator: std.mem.Allocator) !BlogApi {
return BlogApi{
.posts = std.ArrayList(BlogPost).init(allocator),
};
}
pub fn createPost(self: *BlogApi, title: []u8, content: []u8) !u32 {
var post = BlogPost{
.id = @truncate(u32, self.posts.items.len),
.title = try std.mem.dupe(self.allocator, u8, title),
.content = try std.mem.dupe(self.allocator, u8, content),
};
try self.posts.append(post);
return post.id;
}
pub fn getPost(self: *BlogApi, id: u32) !?BlogPost {
for (self.posts.items) |post| {
if (post.id == id) {
return post;
}
}
return null;
}
pub fn updatePost(self: *BlogApi, id: u32, title: []u8, content: []u8) !void {
for (self.posts.items) |*post| {
if (post.id == id) {
post.title = try std.mem.dupe(self.allocator, u8, title);
post.content = try std.mem.dupe(self.allocator, u8, content);
return;
}
}
}
pub fn deletePost(self: *BlogApi, id: u32) !void {
for (self.posts.items) |*post| {
if (post.id == id) {
_ = self.posts.orderedRemove(self.posts.items.len - 1);
return;
}
}
}
};
Handling API Requests
Finally, we'll use the zig-http
library to handle API requests. We'll create a main
function that sets up an HTTP server and handles incoming requests.
const std = @import("std");
pub fn main() !void {
var gpa = std.heap.GeneralPurposeAllocator(.{}){};
defer _ = gpa.deinit();
var allocator = gpa.allocator();
var api = try BlogApi.init(allocator);
defer api.posts.deinit();
var server = try std.http.Server.init(.{
.allocator = allocator,
.listener = try std.http.Listener.init(.{
.port = 8080,
}),
});
defer server.close();
try server.listen();
}
Conclusion
In this article, we've explored the features and benefits of using Zig for backend development. We've seen how Zig's focus on performance, memory safety, and error handling make it an attractive choice for building scalable and maintainable server-side applications. We've also built a simple backend application using Zig, demonstrating how easy it is to get started with the language.
While Zig is still a relatively new language, it has a lot to offer. Its growing community and increasing adoption in the industry make it an exciting choice for developers looking to build high-performance backend applications. Whether you're building a RESTful API or a real-time web application, Zig is definately worth considering.
As we've seen, Zig is a powerful language that can help you build fast, reliable, and maintainable backend applications. With its focus on performance, memory safety, and error handling, Zig is an excellent choice for developers who want to build high-quality software. So why not give Zig a try? You might just find that it's the perfect language for your next backend project.
Future of Zig
As Zig continues to evolve, we can expect to see even more features and improvements. The language is still in its early stages, but it has already shown a lot of promise. With its growing community and increasing adoption, Zig is likely to become a major player in the world of backend development.
One of the most exciting things about Zig is its potential for growth. The language is still in its early stages, but it has already shown a lot of promise. With its focus on performance, memory safety, and error handling, Zig is an excellent choice for developers who want to build high-quality software.
As we look to the future, it's clear that Zig is going to be a major player in the world of backend development. With its growing community and increasing adoption, Zig is likely to become a go-to language for developers who want to build fast, reliable, and maintainable backend applications.
Conclusion
In conclusion, Zig is a powerful language that can help you build fast, reliable, and maintainable backend applications. With its focus on performance, memory safety, and error handling, Zig is an excellent choice for developers who want to build high-quality software. Whether you're building a RESTful API or a real-time web application, Zig is definately worth considering.
So why not give Zig a try? You might just find that it's the perfect language for your next backend project. With its growing community and increasing adoption, Zig is likely to become a major player in the world of backend development.