Understanding the principles of SOLID
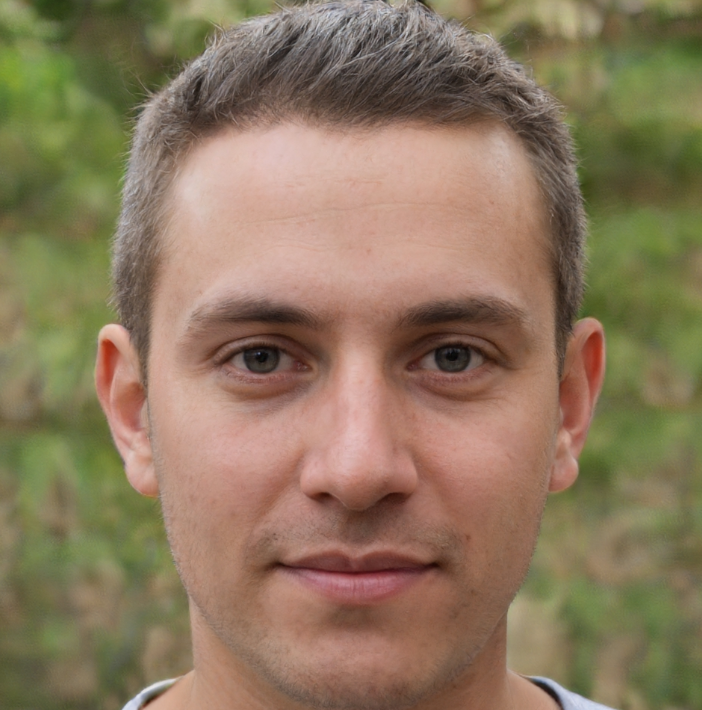

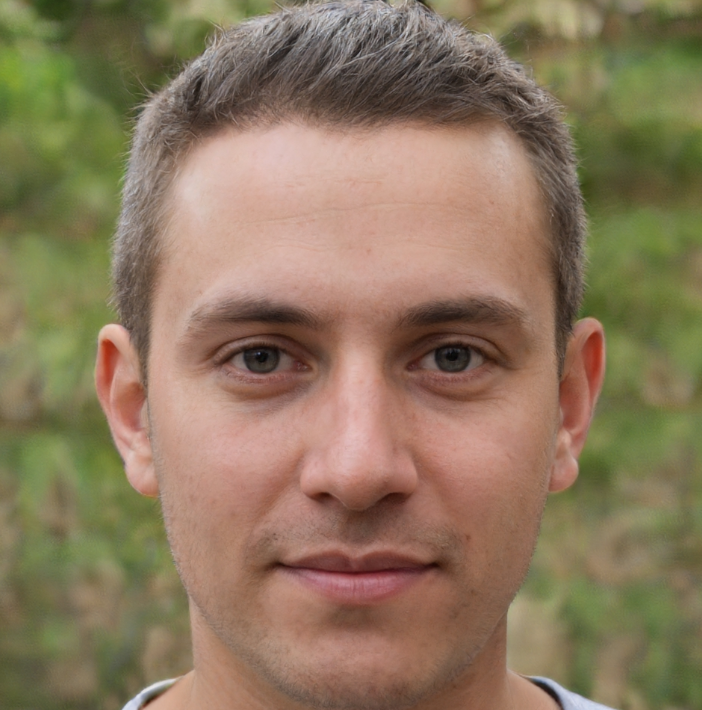
Understanding the Principals of SOLID: A Guide to Writing Better Code
When it comes to writing clean, maintainable, and scalable code, the SOLID principles are a set of guidlines that can help developers acheive these goals. SOLID is an acronym that stands for five design principles of object-oriented programming (OOP) and design. Each letter in the acronym represents a principle that aims to promote simpler, more robust, and updatable code. In this articel, we'll delve into the principles of SOLID, exploring each principle in-depth, and provide examples to illustrate their practical applications.
The Single Responsiblity Principle (SRP)
The Single Responsiblity Principle states that a class should have only one reason to change. In other words, a class should have only one responsiblity or single purpose. This principle is often overlooked, leading to classes that are overly complex and difficult to maintain.
A class with multiple responsiblities is like a Swiss Army knife – it can do many things, but it's not very good at any one thing. Such classes are often refered to as "God objects." God objects are classes that have too many responsiblities and know too much about the other classes in the system. They're difficult to understand, test, and maintain, and can lead to tight coupling between classes.
To illustrate the SRP, let's consider an example. Suppose we're building an e-commerce platform, and we have a Product
class that represents a product in the system. Initially, the Product
class might have a single responsiblity – to represent the product's properties, such as its name, description, and price. However, as the system evolves, we might add more responsiblities to the Product
class, such as handling orders, calculating taxes, and sending notifications.
In this scenario, the Product
class would violate the SRP, as it would have multiple reasons to change. Instead, we should break down the Product
class into smaller, single-purpose classes, each with its own responsiblity. For example, we could have a Product
class that represents the product's properties, an OrderHandler
class that handles orders, a TaxCalculator
class that calculates taxes, and a NotificationService
class that sends notifications.
The Open/Closed Principle (OCP)
The Open/Closed Principle states that software entities (classes, modules, functions, etc.) should be open for extention but closed for modification. In other words, we should be able to add new functionality to a class without modifying its underlying structure.
This principle is essential in object-oriented programming, as it allows us to write code that is flexible and adaptable to changing requirments. By following the OCP, we can add new features to a class without breaking existing functionality or affecting other parts of the system.
To illustrate the OCP, let's consider an example. Suppose we're building a payment gateway that supports multiple payment providers, such as credit cards, PayPal, and bank transfers. We could create a PaymentGateway
class that has a method for processing payments, and then create separate classes for each payment provider, such as CreditCardPayment
, PayPalPayment
, and BankTransferPayment
.
By using polymorphism, we can add new payment providers without modifying the underlying PaymentGateway
class. We can simply create a new class that implements the payment provider's logic and add it to the system. The PaymentGateway
class would then use the new class to process payments, without needing to be modified.
The Liskov Substitution Principle (LSP)
The Liskov Substitution Principle states that subtypes must be substitutable for their base types. In other words, any code that uses a base type should be able to work with a subtype without knowing the difference.
This principle ensures that inheritance is used correctly, and that subtypes can be used as drop-in replacements for their base types. By following the LSP, we can write code that is more flexible and easier to maintain.
To illustrate the LSP, let's consider an example. Suppose we're building a system that uses rectangles to represent geometric shapes. We could have a Rectangle
class that has methods for calculating the area and perimeter of the rectangle. We could then create a Square
class that inherits from the Rectangle
class.
The Square
class would be a subtype of the Rectangle
class, and it should be substitutable for its base type. This means that any code that uses a Rectangle
object should be able to work with a Square
object without knowing the difference. For example, if we have a method that takes a Rectangle
object as a parameter and calculates its area, we should be able to pass a Square
object to that method without any issues.
The Interface Segregation Principle (ISP)
The Interface Segregation Principle states that clients should not be forced to depend on interfaces they don't use. In other words, interfaces should be designed to meet the needs of specific clients, rather than being general-purpose.
This principle is essential in designing interfaces that are easy to use and maintain. By following the ISP, we can avoid "fat" interfaces that have too many methods, and instead create lean interfaces that are tailored to specific clients.
To illustrate the ISP, let's consider an example. Suppose we're building a system that uses a Printable
interface to represent objects that can be printed. The Printable
interface might have methods for printing in different formats, such as PDF, JPEG, and CSV. However, not all clients that use the Printable
interface might need all of these methods.
By following the ISP, we could break down the Printable
interface into smaller, more focused interfaces, such as PrintableToPDF
, PrintableToJPEG
, and PrintableToCSV
. This would allow clients to depend only on the interfaces they need, rather than being forced to implement all of the methods in the Printable
interface.
The Dependency Inversion Principle (DIP)
The Dependency Inversion Principle states that high-level modules should not depend on low-level modules, but rather both should depend on abstractions. In other words, a class should not depend on another class, but rather both should depend on an interface or abstract class.
This principle is essential in designing loosely coupled systems that are easy to maintain and extend. By following the DIP, we can write code that is modular, flexible, and scalable.
To illustrate the DIP, let's consider an example. Suppose we're building a system that uses a database to store data. We could have a Database
class that provides methods for accessing and manipulating data in the database. However, if our BusinessLogic
class depends directly on the Database
class, we would be tightly coupling the two classes.
Instead, we could create an interface IDataAccess
that defines the methods for accessing and manipulating data, and then have the Database
class implement this interface. The BusinessLogic
class would then depend on the IDataAccess
interface, rather than the Database
class. This would allow us to swap out the Database
class with a different implementation, such as a FileAccess
class, without affecting the BusinessLogic
class.
Conclusion
In this articel, we've explored the principles of SOLID, a set of guidlines that can help developers write better code. By following the Single Responsiblity Principle, Open/Closed Principle, Liskov Substitution Principle, Interface Segregation Principle, and Dependency Inversion Principle, we can write code that is cleaner, more maintainable, and easier to extend.
By applying these principles in our daily coding practices, we can create software systems that are more modular, flexible, and scalable. We can write code that is easier to understand, test, and maintain, and that is less prone to errors and bugs.
In the end, the SOLID principles are not just about writing better code – they're about creating software systems that are more enjoyable to work with, and that can meet the changing needs of our users. By following these principles, we can create software systems that are truly remarkable, and that can make a lasting impact on the world.
In conclusion, the SOLID principles are a powerful tool in the hands of developers, and by following them, we can create software systems that are truly remarkable. So, the next time you're writing code, remember to keep the SOLID principles in mind, and you'll be well on your way to creating software systems that are clean, maintainable, and scalable.