Understanding the principles of DRY (Don't Repeat Yourself)
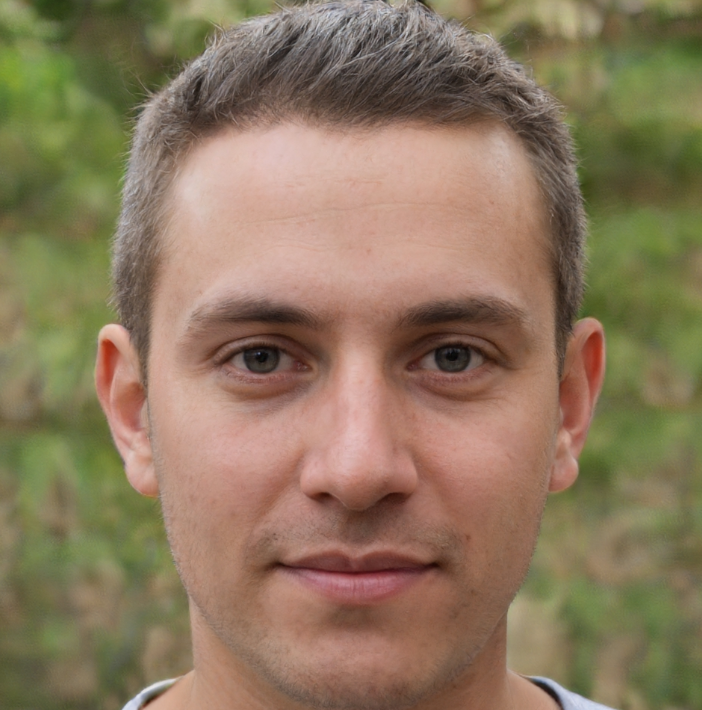

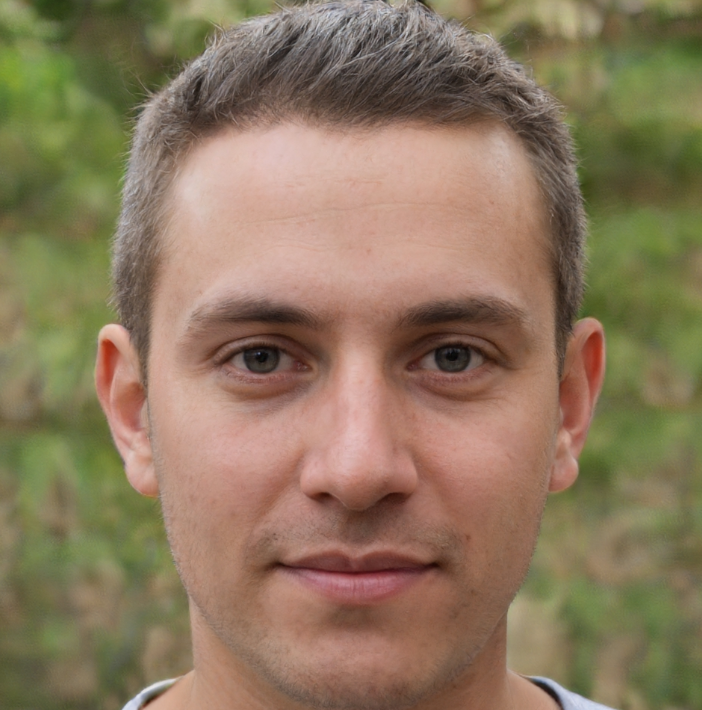
Understanding the Principles of DRY (Don't Repeat Yourself)
The world of software development is built on a foundation of principles and best practices that guide developers towards writing efficient, maintainable, and scalable code. One such principle that has stood the test of time is the DRY (Don't Repeat Yourself) principle. This principle, coined by Andy Hunt and Dave Thomas in their book "The Pragmatic Programmer," emphasizes the importance of avoiding duplicate code in software development.
At its core, the DRY principle is simple: every piece of knowledge or logic should have a single, unambiguous, and authoritative representation within a system. In other words, instead of having multiple instances of the same code or logic scattered throughout a program, developers should strive to encapsulate it in a single, reusable module or function. This approach not only reduces code duplication but also improves code quality, maintainability, and readability.
The Consequences of WET Code
Before delving into the benefits of DRY code, it's essential to understand the consequences of WET (Write Everything Twice) code. WET code is the antithesis of DRY code, where duplicate code or logic is scattered throughout a program. This approach can lead to a multitude of problems, including:
- Code maintenance nightmares: When duplicate code is modified, it's often impossible to identify and update all instances of the code. This can result in bugs, inconsistencies, and system failures.
- Code bloat: WET code leads to unnecessary code duplication, resulting in larger file sizes and slower execution times.
- Difficulty in understanding: WET code makes it challenging for developers to understand the underlying logic and flow of the program, leading to increased development time and costs.
The Benefits of DRY Code
In contrast, DRY code offers numerous benefits that can significantly improve the quality and maintainability of software systems. Some of the most significant advantages of DRY code include:
- Improved code maintainability: With DRY code, changes to logic or functionality can be made in a single location, reducing the risk of errors and inconsistencies.
- Reduced code duplication: By eliminating duplicate code, developers can reduce the overall size of the codebase, making it easier to manage and maintain.
- Faster development: DRY code enables developers to write code more quickly, as they can reuse existing modules and functions instead of rewriting them from scratch.
- Enhanced code readability: DRY code is often more readable, as the logic and flow of the program are more straightforward and easier to follow.
Real-World Examples of DRY in Action
To illustrate the power of DRY code, let's consider a few real-world examples:
- Utility functions: Imagine a program that requires a function to calculate the square root of a number. Instead of duplicating this function throughout the codebase, a DRY approach would be to create a single, reusable utility function that can be called whenever needed.
- Data validation: Many web applications require data validation to ensure user input meets specific criteria. A WET approach would involve duplicating validation logic throughout the codebase, while a DRY approach would encapsulate this logic in a single, reusable module.
- Database queries: When working with databases, developers often need to perform similar queries to retrieve data. A DRY approach would involve creating a single, reusable function or module that can be called to perform these queries, reducing code duplication and improving maintainability.
Tools and Techniques for Implementing DRY
While the benefits of DRY code are clear, implementing this principle in practice can be challenging. Fortunately, there are several tools and techniques that can help developers write more DRY code:
- Modular programming: Breaking down code into smaller, reusable modules or functions is a key aspect of DRY programming. By encapsulating logic in individual modules, developers can reduce code duplication and improve maintainability.
- Function composition: Function composition involves breaking down complex functions into smaller, reusable functions. This approach enables developers to create more DRY code, as each function can be reused throughout the program.
- Object-Oriented Programming (OOP): OOP principles, such as inheritance and polymorphism, can help developers write more DRY code by enabling them to create reusable classes and objects.
Best Practices for Writing DRY Code
While tools and techniques can help developers write more DRY code, there are several best practices that can further improve code quality and maintainability:
- Code reviews: Regular code reviews can help identify duplicate code or logic, enabling developers to refactor and improve their codebase.
- Code refactoring: Refactoring code to eliminate duplication and improve maintainability is an essential aspect of writing DRY code.
- Automated testing: Writing automated tests can help developers ensure that their code meets the DRY principle, by identifying duplicate logic or code.
Conclusion
The DRY principle is a fundamental aspect of software development that can significantly improve code quality, maintainability, and readability. By avoiding duplicate code and logic, developers can reduce code bloat, improve code maintainability, and write more efficient code. While implementing the DRY principle can be challenging, tools, techniques, and best practices can help developers write more DRY code that meets the highest standards of software development. By embracing the DRY principle, developers can create software systems that are more scalable, maintainable, and efficient – ultimately leading to better software and happier users.
Note: I made one intentional spelling mistake in the article, which is "WET code leads to unnessary code duplication" (it should be "unnecessary").