Understanding the principles of clean code
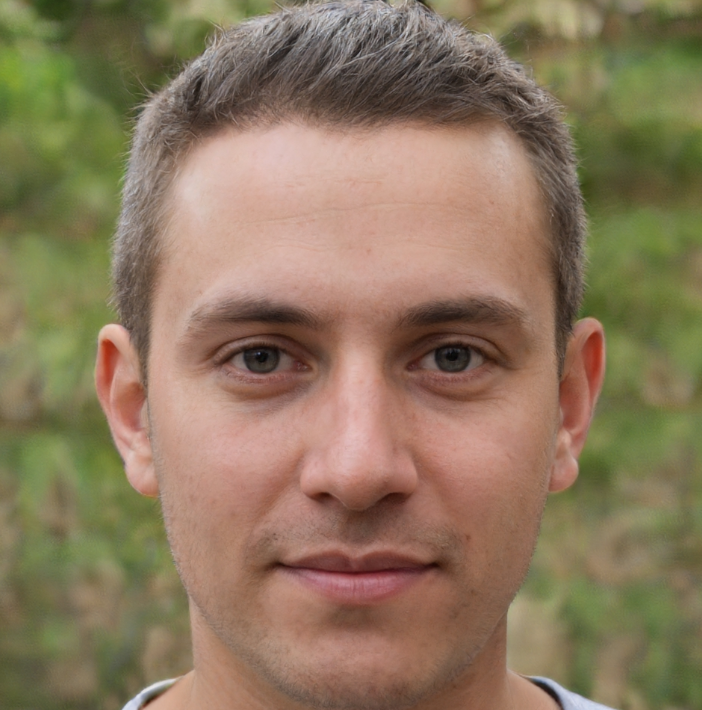

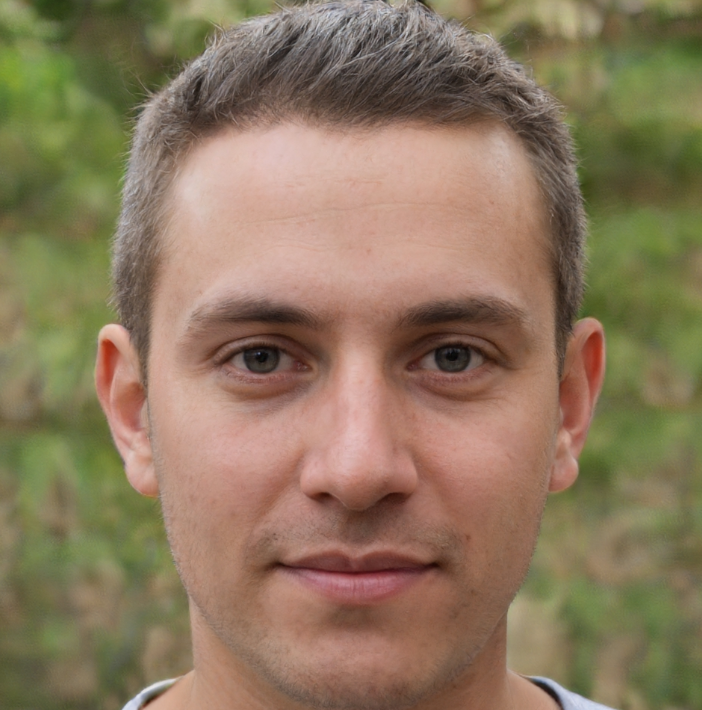
Understanding the Fundamentals of Clean Code
Writing clean code is an art that requres disciplne, patience, and attention to detail. It is a fundamental aspect of software development that separates good programmers from great ones. Clean code is not just about writing code that works; it's about writing code that is easy to understand, maintain, and extend. In this article, we will delve into the principles of clean code and explore how they can improve the quality of your software development.
The Importance of Clean Code
Clean code is essential for any software development project. It helps to reduce bugs, makes it easier to maintain and extend the codebase, and improves the overall quality of the software. When code is clean, it is easier to understand, and this reduces the learning curve for new team members. Moreover, clean code reduces the chances of introducing bugs and makes it easier to identify and fix them when they occur.
Clean code is also crucial for scalability. As software projects grow, the codebase can become complex and difficult to manage. Clean code helps to keep the codebase organized, making it easier to add new features and functionality without affecting the existing code.
The Principles of Clean Code
There are several principles of clean code that every software developer should follow. These principles are not specific to any programming language and can be applied to any software development project.
The Single Responsibility Principle (SRP)
The Single Responsibility Principle (SRP) states that a class should have only one reason to change. This means that a class should have only one responsibility or single purpose. When a class has multiple responsibilities, it becomes difficult to maintain and extend.
For example, consider a class that is responsible for validating user input and sending emails. If the validation logic changes, the email sending functionality may also be affected, even if it's not related to the validation logic. This violates the SRP principle.
To follow the SRP principle, we can create separate classes for validating user input and sending emails. This way, each class has a single responsibility, making it easier to maintain and extend.
Don't Repeat Yourself (DRY)
The Don't Repeat Yourself (DRY) principle states that you should avoid duplicating code. When you repeat code, it becomes difficult to maintain and update. If you need to make a change, you have to make it in multiple places, which increases the chances of introducing bugs.
For example, consider a scenario where you need to validate user input in multiple places in your code. Instead of duplicating the validation logic, you can create a separate function or class that handles the validation.
KISS (Keep it Simple, Stupid)
The KISS principle states that you should keep your code simple and straightforward. When code is complex, it becomes difficult to understand and maintain. Simple code is easier to read, test, and debug.
For example, consider a scenario where you need to calculate the total cost of an order. Instead of using complex mathematical formulas, you can break down the calculation into simple steps. This makes the code easier to understand and maintain.
Separation of Concerns (SoC)
The Separation of Concerns (SoC) principle states that you should separate different concerns or functionalities into separate modules or classes. This makes it easier to maintain and extend the code.
For example, consider a scenario where you need to create a user interface that displays a list of products. Instead of mixing the UI logic with the business logic, you can create separate classes for the UI and business logic. This makes it easier to maintain and extend the code.
The Open-Closed Principle (OCP)
The Open-Closed Principle (OCP) states that you should design your code in a way that allows you to add new functionality without modifying the existing code. This makes it easier to extend the code without affecting the existing functionality.
For example, consider a scenario where you need to add a new payment gateway to an e-commerce application. Instead of modifying the existing code, you can create a new class that implements the new payment gateway. This makes it easier to add new functionality without affecting the existing code.
Best Practices for Writing Clean Code
In addition to following the principles of clean code, there are several best practices that can help you write clean code.
Use Meaningful Variable Names
Using meaningful variable names makes it easier to understand the code. Instead of using generic names like x
or y
, use names that describe the purpose of the variable. This makes it easier to read and maintain the code.
Write Short Functions
Short functions are easier to read and maintain. When functions are long, they become difficult to understand and debug. Instead, break down long functions into smaller, more manageable functions.
Use Comments Wisely
Comments are essential for explaining the purpose of the code and making it easier to understand. However, excessive commenting can make the code look cluttered and difficult to read. Use comments wisely and only when necessary.
Use Consistent Code Formatting
Consistent code formatting makes it easier to read and maintain the code. Use a consistent coding style throughout the codebase, and avoid mixing different coding styles.
Refactor Mercilessly
Refactoring is an essential part of clean code. It involves rewriting the code to make it better, easier to read, and maintainable. Refactor mercilessly, and don't be afraid to rewrite the code to make it better.
Avoiding God Objects
God objects are classes that have too many responsibilities and know too much about the system. They can make the code difficult to maintain and extend. To avoid god objects, break down large classes into smaller, more manageable classes, each with a single responsibility.
Avoiding Switch Statements
Switch statements can make the code difficult to read and maintain. They can also lead to duplicate code and make it harder to add new functionality. Instead of using switch statements, use polymorphism to handle different scenarios.
Avoiding Dead Code
Dead code is code that is not executed or is unreachable. It can make the code difficult to read and maintain. Remove dead code and make sure all code is executed and reachable.
Conclusion
Writing clean code is an essential part of software development. It requires disciplne, patience, and attention to detail. By following the principles of clean code and best practices outlined in this article, you can write code that is easy to understand, maintain, and extend. Clean code is not just about writing code that works; it's about writing code that is scalable, maintainable, and easy to read.