Understanding asynchronous programming
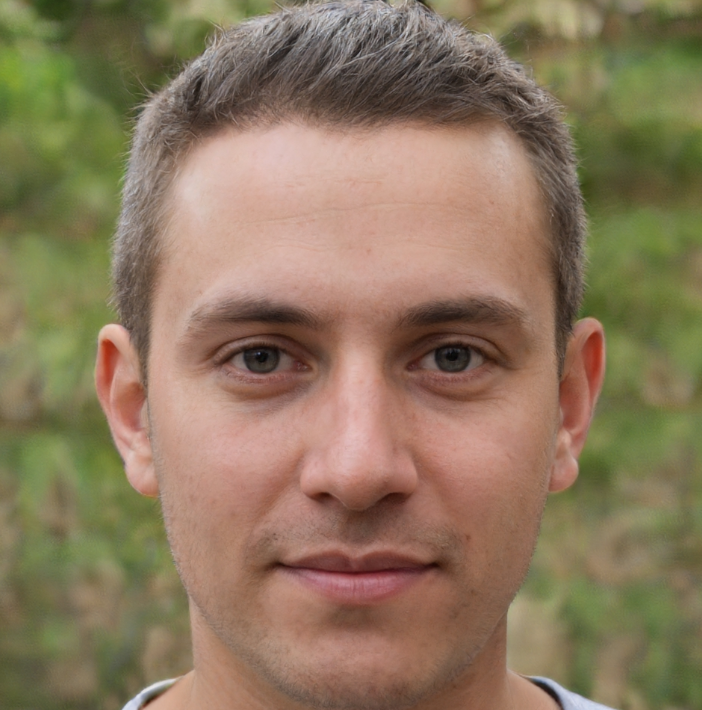

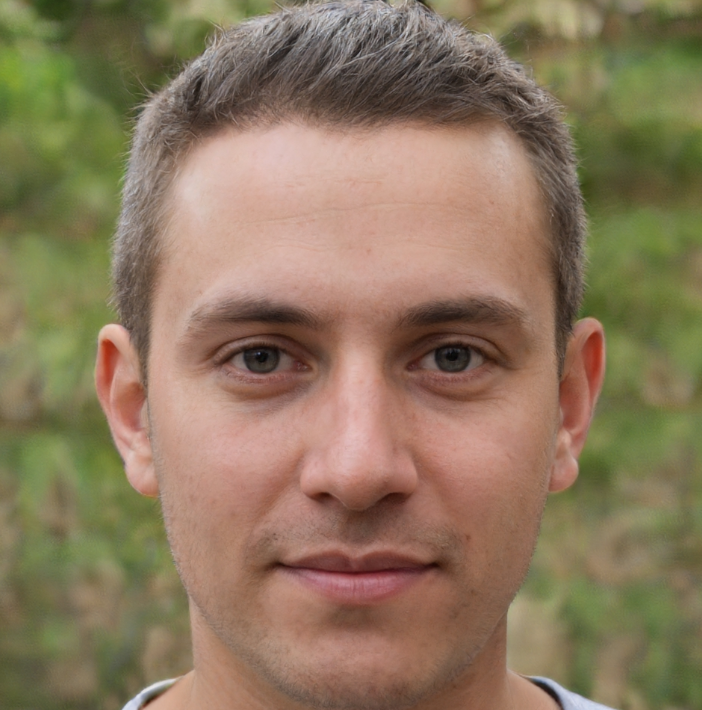
Understanding Asynchronous Programming: Unraveling the Mysteries of Concurrency
In the world of computer science, asynchronous programming has become an indispensable concept, enabling developers to create efficient, scalable, and responsive applications. Asynchronous programming allows multiple tasks to be executed simultaneously, improving the overall performance and user experience of software systems. However, grasping the fundamentals of asynchronous programming can be a challenging task, especially for novice developers. In this article, we will delve deeper into the realm of asynchronous programming, exploring its core concepts, benefits, and implementation strategies.
Synchronous vs. Asynchronous Programming
To understand asynchronous programming, it's essential to differentiate it from its counterpart, synchronous programming. In synchronous programming, tasks are executed sequentially, one after another. Each task must complete before the next one begins, resulting in a linear execution flow. This approach can lead to performance bottlenecks, as the program waits for each task to finish before proceeding.
Asynchronous programming, on the other hand, allows multiple tasks to be executed concurrently, without blocking or waiting for each other to complete. This approach enables the program to process multiple tasks simultaneously, leveraging the available system resources more efficiently. Asynchronous programming is particularly useful in scenarios where tasks involve I/O operations, network requests, or other time-consuming activities.
Core Concepts of Asynchronous Programming
Asynchronous programming relies on several fundamental concepts that ensure efficient and concurrent task execution. These concepts include:
1. Threads and Processes
In asynchronous programming, tasks are executed in separate threads or processes. Threads are lightweight, sharing the same memory space as the parent process. Processes, on the other hand, are heavier, with their own memory space and execution context. Both threads and processes enable concurrent task execution, but threads are generally more efficient due to their lighter weight.
2. Synchronization and Locking
To ensure data consistency and integrity, asynchronous programming employs synchronization mechanisms, such as locks, semaphores, and monitors. These mechanisms regulate access to shared resources, preventing data corruption and race conditions.
3. Callbacks and Event-Driven Programming
Callbacks and event-driven programming are essential components of asynchronous programming. Callbacks are functions that are executed upon the completion of a task, while event-driven programming involves responding to events or notifications generated by tasks.
4. Promises and Futures
Promises and futures are abstractions that represent the outcome of an asynchronous operation. They provide a way to handle the result of an asynchronous task, allowing developers to write more concise and expressive code.
5. Async/Await and Coroutines
Async/await and coroutines are higher-level abstractions that simplify asynchronous programming. Async/await enables developers to write asynchronous code that resembles synchronous code, while coroutines provide a way to write cooperative multitasking code.
Benefits of Asynchronous Programming
Asynchronous programming offers numerous benefits, including:
1. Improved Responsiveness
Asynchronous programming enables applications to respond quickly to user input, even when performing time-consuming tasks in the background.
2. Increased Throughput
By executing tasks concurrently, asynchronous programming can significantly improve the overall throughput and performance of applications.
3. Better Resource Utilization
Asynchronous programming allows developers to make efficient use of system resources, such as CPU, memory, and I/O devices.
4. Enhanced Scalability
Asynchronous programming enables applications to scale more effectively, handling a larger number of concurrent tasks and users.
Implementing Asynchronous Programming
Implementing asynchronous programming involves choosing the right programming paradigm, framework, and tools. Some popular frameworks and libraries for asynchronous programming include:
1. Node.js and Async.js
Node.js is a popular JavaScript runtime that provides built-in support for asynchronous programming. Async.js is a library that simplifies asynchronous programming in Node.js.
2. Python's asyncio
Python's asyncio is a built-in library that provides support for asynchronous programming in Python.
3. Java's CompletableFuture
Java's CompletableFuture is a class that represents a computation that may not have completed yet. It provides a way to handle the result of an asynchronous operation.
Real-World Examples of Asynchronous Programming
Asynchronous programming is used in a wide range of applications, including:
1. Web Applications
Web applications, such as social media platforms and online shopping websites, use asynchronous programming to handle multiple requests concurrently, improving the responsiveness and usability of the application.
2. Mobile Applications
Mobile applications, such as games and social media apps, use asynchronous programming to handle tasks such as networking, storage, and UI updates, improving the overall user experience.
3. IoT Devices
IoT devices, such as smart home devices and sensors, use asynchronous programming to handle tasks such as data processing, network communication, and sensor readings, improving the efficiency and effectiveness of the device.
Common Mistakes in Asynchronous Programming
Asynchronous programming can be challenging, and developers often make mistakes that can lead to bugs, performance issues, and security vulnerabilities. Some common mistakes include:
1. Callback Hell
Callback hell occurs when developers use callbacks excessively, leading to complex and unreadable code. This can be avoided by using promises or async/await syntax.
2. Unhandled Errors
Unhandled errors can occur when developers fail to handle errors properly in asynchronous code. This can lead to unexpected behavior, crashes, and security vulnerabilities.
3. Race Conditions
Race conditions occur when multiple tasks access shared resources simultaneously, leading to unexpected behavior and errors. This can be avoided by using synchronization mechanisms such as locks, semaphores, and atomic operations.
Best Practices for Asynchronous Programming
To write efficient and effective asynchronous code, developers should follow best practices such as:
1. Use Promises or Async/Await Syntax
Use promises or async/await syntax to write asynchronous code that is readable and maintainable.
2. Handle Errors Properly
Handle errors properly by using try-catch blocks and error handling mechanisms such as error callbacks and promise rejection handlers.
3. Use Synchronization Mechanisms
Use synchronization mechanisms such as locks, semaphores, and atomic operations to avoid race conditions and ensure thread safety.
4. Test Asynchronous Code Thoroughly
Test asynchronous code thoroughly by using testing frameworks and tools that support asynchronous testing.
Conclusion
Asynchronous programming is a powerful technique that allows developers to write efficient, scalable, and responsive applications. By understanding the core concepts, benefits, and implementation strategies of asynchronous programming, developers can unlock the full potential of concurrency and parallelism. By following best practices and choosing the right programming paradigm, framework, and tools, developers can write high-quality asynchronous code that meets the demands of modern software systems.