The role of middleware in backend development
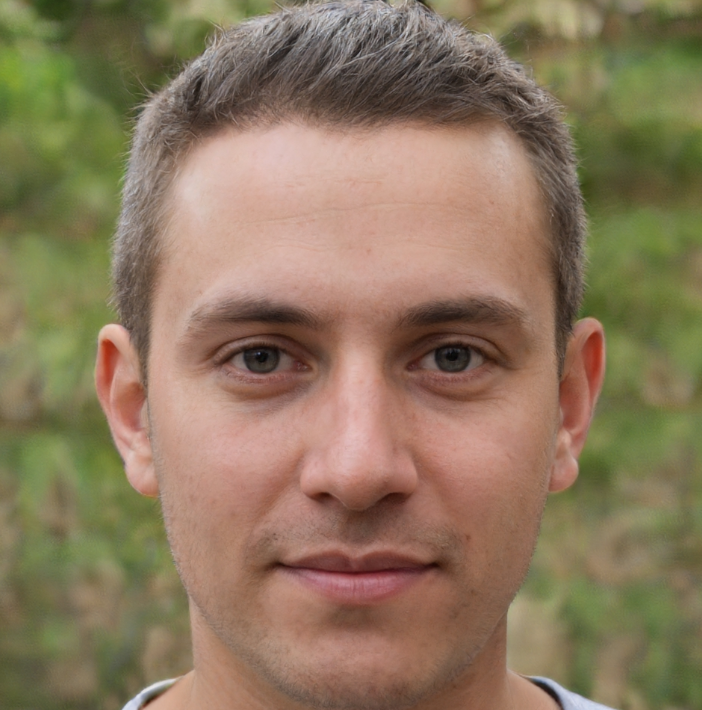

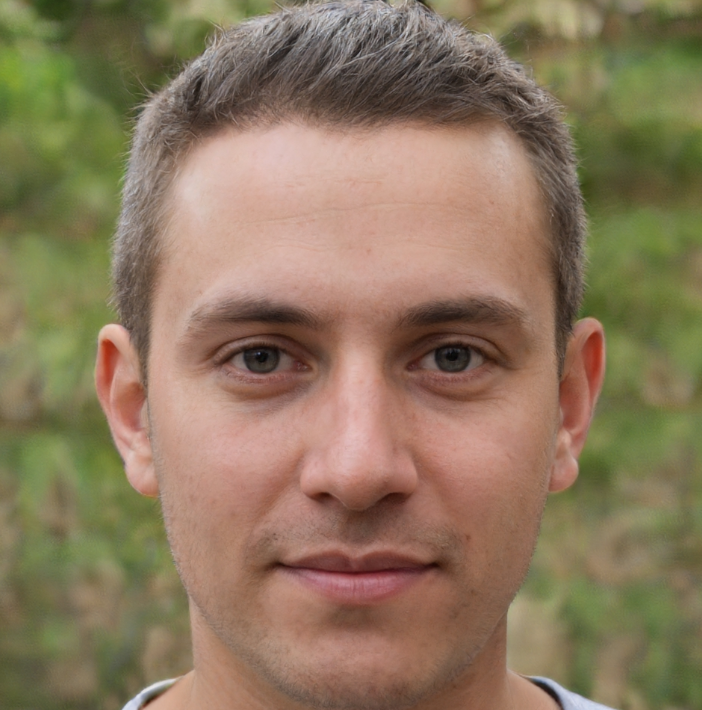
The Role of Middleware in Backend Development
When building a web application, the backend is responsable for handling requests, processing data, and storing information. However, as the complexity of the application grows, the backend can become burdened with repetitive tasks, such as authentication, caching, and error handling. This is where middleware comes into play. Middleware is a crucial component of backend development that acts as a bridge between the request and the response, allowing developers to perform tasks that are essential to the functioning of the application.
What is Middleware?
In the context of web development, middleware is a software layer that sits between the application and the network. It is responsable for processing requests and responses, and can be thought of as a series of filters that the data passes through before reaching the application. Middleware can be used to perform a wide range of tasks, including authentication, caching, rate limiting, and error handling.
Middleware can be classified into two categories: framework-specific middleware and custom middleware. Framework-specific middleware is provided by the web framework being used, such as Express.js in Node.js or Django in Python. Custom middleware, on the other hand, is written by the developer to perform specific tasks that are unique to the application.
How Middleware Works
The process of handling a request and sending a response can be broken down into several stages. The first stage is the reception of the request, followed by the execution of the middleware chain. The middleware chain is a series of middleware functions that are executed in a specific order. Each middleware function can perform a specific task, such as authentication or caching, and can either pass the request to the next middleware function in the chain or terminate the request by sending a response.
After the middleware chain has been executed, the request reaches the application, where it is processed and a response is generated. The response then passes through the middleware chain in reverse order, allowing each middleware function to perform any necessary tasks before the response is sent back to the client.
Advantages of Middleware
Middleware provides several advantages to backend development. One of the most significant benefits is the ability to modularize code and separate concerns. By breaking down the application into smaller, independent components, developers can focus on individual tasks without affecting the rest of the application.
Middleware also allows for greater flexibility and scalability. By providing a layer of abstraction between the application and the network, middleware enables developers to easily switch between different storage systems, caching mechanisms, and authentication providers.
Another significant advantage of middleware is its ability to improve security. By providing a single point of entry for requests, middleware can perform security-related tasks, such as authentication and input validation, in a centralized manner.
Middleware also provides a way to handle errors in a centralized manner. By catching and handling errors at the middleware level, developers can ensure that errors are handled consistently across the application, reducing the risk of errors being overlooked or mishandled.
Real-World Examples of Middleware
One of the most common examples of middleware is authentication middleware. This type of middleware checks the credentials of incoming requests and verifies the identity of the user. If the credentials are valid, the request is allowed to pass through to the application. If the credentials are invalid, the middleware sends a response back to the client indicating that the request was unauthorized.
Another common example of middleware is caching middleware. This type of middleware caches frequently accessed data, reducing the load on the application and improving response times.
Rate limiting middleware is another example of middleware that is commonly used. This type of middleware limits the number of requests that can be made to the application within a certain time frame, preventing abuse and denial-of-service attacks.
Challenges of Middleware
While middleware provides several advantages, it also presents some challenges. One of the most significant challenges is the complexity of middleware chains. As the number of middleware functions increases, the risk of bugs and performance issues also increases.
Another challenge of middleware is the difficulty of debugging. Because middleware functions are executed in a specific order, it can be difficult to identify which middleware function is causing an issue.
Best Practices for Middleware
To overcome the challenges of middleware, developers should follow best practices when writing and using middleware. One of the most important best practices is to keep middleware functions simple and focused on a single task. This reduces the risk of bugs and makes it easier to debug issues.
Another best practice is to use established middleware libraries and frameworks whenever possible. This not only reduces the amount of code that needs to be written, but also ensures that the middleware is thoroughly tested and reliable.
Conclusion
Middleware plays a crucial role in backend development, providing a way to modularize code, improve security, and reduce the complexity of the application. By understanding how middleware works and following best practices, developers can build faster, more scalable, and more secure applications. Whether it's authentication, caching, or rate limiting, middleware is an essential component of any web application, and its importance cannot be overstated. By leveraging the power of middleware, developers can build applications that are capable of meeting the demands of modern users, and providing a seamless and intuitive experience.
A Deep Dive into Middleware
In the world of backend development, building a robust and scalable application requires a multitude of moving parts working in harmony. One such crucial component is middleware, which plays a vital role in facilitating communication between different layers of an application. In this article, we'll delve deeper into the world of middleware, exploring its definition, types, benefits, and real-world examples.
What is Middleware?
Middleware is a software layer that mediates between the client and the server, enabling seamless communication and data exchange between the two. It acts as a bridge, allowing the client to request data or perform actions on the server, while also providing a layer of abstraction, making it easier to manage complexity and scalability. Middleware can be thought of as a series of filters that incoming requests and outgoing responses pass through, enabling features such as authentication, caching, and input validation.
Types of Middleware
Middleware can be broadly classified into two categories: infrastructure middleware and application middleware.
Infrastructure Middleware
Infrastructure middleware focuses on providing low-level, platform-specific functionality, such as:
- Load Balancers: Distributing incoming traffic across multiple servers to improve performance and availability.
- Proxies: Acting as an intermediary between clients and servers, often used for caching, security, or content filtering.
- Gateways: Providing a single entry point for clients, masking the underlying server architecture.
Application Middleware
Application middleware, on the other hand, concentrates on providing high-level, application-specific functionality, such as:
- Authentication: Verifying user credentials and controlling access to resources.
- Rate Limiting: Regulating the number of requests from a client to prevent abuse or overloading.
- Caching: Storing frequently accessed data to reduce latency and improve performance.
Benefits of Middleware
The judicious use of middleware can bring numerous benefits to a backend application, including:
Improved Security
Middleware can implement security features like authentication, authorization, and encryption, shielding the application from potential threats.
Enhanced Performance
By caching frequently accessed data and optimizing database queries, middleware can significantly improve response times and reduce latency.
Scalability and Flexibility
Middleware enables developers to introduce new features and services without modifying the underlying application code, making it easier to adapt to changing requirements.
Simplified Development
Middleware can abstract away complex functionality, providing a simpler, more intuitive development experience for backend developers.
Real-World Examples of Middleware
1. Express.js Middleware
In the popular Node.js framework Express.js, middleware is used extensively to handle tasks such as:
- Logger Middleware: Logging requests and responses for debugging and analytics purposes.
- Body Parser Middleware: Parsing JSON payloads and making them accessible to the application.
- Authentication Middleware: Verifying user credentials and controlling access to protected routes.
2. Nginx as a Reverse Proxy
Nginx, a popular web server, can be used as a reverse proxy, acting as a middleware layer between clients and backend applications. This setup enables:
- Load Balancing: Distributing incoming traffic across multiple backend servers.
- Content Caching: Storing frequently accessed resources to reduce latency.
- SSL Termination: Offloading encryption and decryption tasks, reducing the load on backend servers.
3. message queues
Message queues, such as RabbitMQ or Apache Kafka, serve as middleware between producers and consumers, enabling asynchronous communication and decoupling.
Best Practices for Implementing Middleware
When incorporating middleware into your backend application, keep the following best practices in mind:
1. Keep it Simple and Focused
Each middleware function should have a single, well-defined responsibility, making it easier to maintain and debug.
2. Use Established Frameworks and Libraries
Leverage existing middleware frameworks and libraries, such as Express.js or Django, to reduce development time and improve code quality.
3. Monitor and Analyze Performance
Regularly monitor middleware performance, identifying bottlenecks and optimizing accordingly to ensure optimal application performance.
4. Test Thoroughly
Comprehensively test middleware functionality, ensuring it meets the required security, performance, and scalability standards.
Conclusion
Middleware plays a vital role in backend development, providing a layer of abstraction, security, and scalability to applications. By understanding the different types of middleware, their benefits, and real-world examples, developers can harness the power of middleware to build robust, efficient, and scalable applications. By following best practices and choosing the right middleware for the task at hand, developers can create a seamless and efficient communication channel between clients and servers, ultimately leading to better user experiences and business outcomes.