The basics of backend development
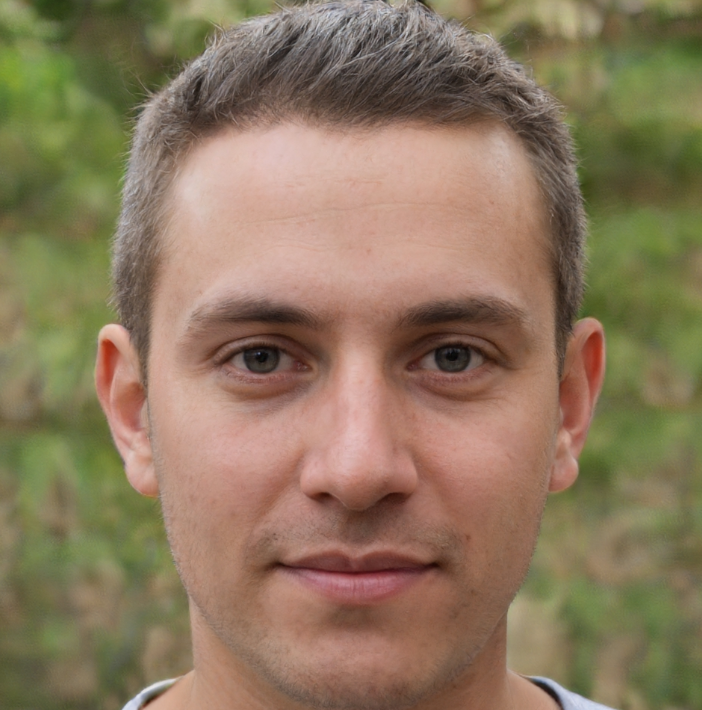

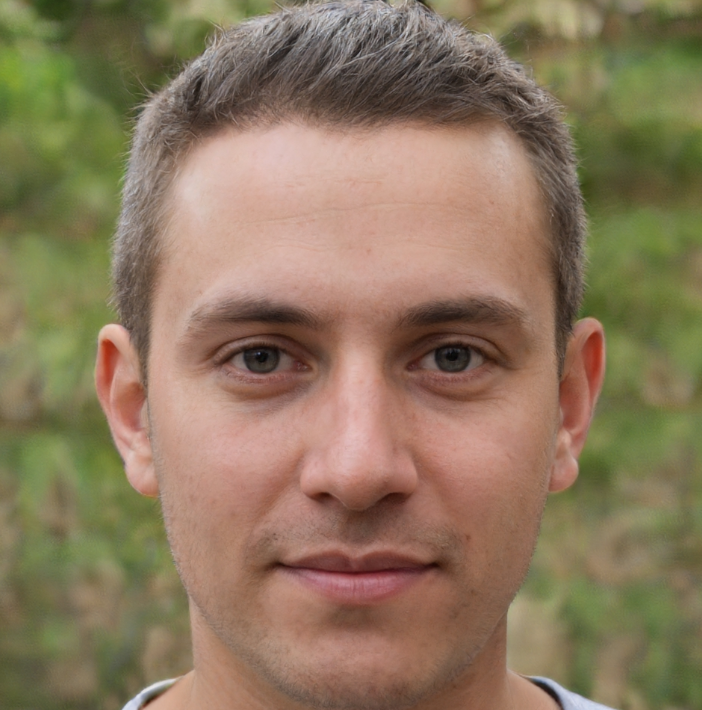
The Foundations of Backend Development: A Comprehensive Guide
The world of web development is a complex and fascinating place, where creativty meets technical prowess. While frontend development focuses on crafting the user interface and user experience, backend development is responsible for the behind-the-scenes magic that makes it all possible. In this article, we'll delve into the basics of backend development, exploring the fundamental concepts, technologies, and best practices that drive the web forward.
What is Backend Development?
At its core, backend development involves building the server-side logic, database integration, and API connectivity that powers a website or application. It's the unsung hero of web development, working tirelessly behind the scenes to provide data, storage, and functionality to the frontend. Backend developers use programming languages, frameworks, and tools to create a robust and scalable infrastructure that can handle user requests, process data, and store information.
Key Concepts: Servers, Databases, and APIs
Servers
A server is a computer or device that provides resources, services, or data to other computers or devices over a network. In the context of backend development, servers are responsible for hosting and running the backend code, receiving and responding to user requests, and managing data storage and retrieval. Common types of servers include web servers (e.g., Apache, Nginx), application servers (e.g., Node.js, Python), and database servers (e.g., MySQL, PostgreSQL).
Databases
Databases are organized collections of data that are stored in a way that allows for efficient retrieval and manipulation. Backend developers use databases to store and manage data, such as user information, product catalogs, and transactional history. Popular database management systems include relational databases (e.g., MySQL, PostgreSQL), NoSQL databases (e.g., MongoDB, Cassandra), and graph databases (e.g., Neo4j).
APIs
Application Programming Interfaces (APIs) are sets of defined rules that enable different systems to communicate with each other. In backend development, APIs act as an intermediary between the frontend and backend, allowing them to exchange data and facilitate interactions. APIs can be classified into two main categories: RESTful APIs (Representational State of Resource) and GraphQL APIs.
Programming Languages and Frameworks
Backend developers use a variety of programming languages and frameworks to build and deploy their applications. Here are some popular ones:
Programming Languages
- JavaScript: Used for both frontend and backend development, JavaScript is a popular choice for building server-side applications using Node.js.
- Python: Known for its simplicity and versitility, Python is widely used for web development, scientific computing, and data analysis.
- Ruby: A dynamic language famous for its ease of use and the Ruby on Rails framework.
- PHP: A mature language used for web development, especially for building dynamic websites and web applications.
Frameworks
- Express.js: A lightweight Node.js framework for building web applications and APIs.
- Django: A high-level Python framework that provides an architecture, templates, and APIs for building web applications.
- Ruby on Rails: A server-side framework that provides a structure for building web applications using Ruby.
- Laravel: A PHP framework that provides a robust set of tools and features for building web applications.
Best Practices and Tools
Code Organization and Structure
- Modularization: Break down code into smaller, reusable modules to improve maintainability and scalability.
- Separation of Concerns: Keep different components of the application separate, such as business logic, data access, and presentation.
Security and Authentication
- Input Validation: Validate user input to prevent SQL injection and cross-site scripting (XSS) attacks.
- Authentication and Authorization: Implement secure authentication and authorization mechanisms to control access to resources.
- Encryption: Use encryption to protect sensitive data in transit and at rest.
Deployment and Scaling
- Containerization: Use containerization technologies like Docker to simplify deployment and management.
- Cloud Computing: Leverage cloud providers like AWS, Azure, or Google Cloud to scale and deploy applications.
- Load Balancing: Distribute incoming traffic across multiple servers to improve performance and availability.
Conclusion
Backend development is a complex and multifaceted field that requires a deep understanding of programming languages, frameworks, and technologies. By mastering the basics of backend development, you'll be well on your way to building scalable, secure, and efficient applications that provide a seamless user experience. Remember to stay up-to-date with industry trends, best practices, and emerging technologies to stay ahead of the curve. With persistence, dedication, and a passion for learning, you can unlock the full potential of backend development and create innovative solutions that transform the web.
Understanding the Request-Response Cycle
At the heart of backend development is the request-response cycle, a fundamental concept that describes how a client (such as a web browser) interacts with a server. This cycle involves four stages:
- Request: A client sends a request to the server, typically in the form of an HTTP request (e.g., GET, POST, PUT, DELETE).
- Server-side processing: The server processes the request, which may involve executing server-side logic, querying a database, or interacting with third-party services.
- Response: The server sends a response back to the client, which may include data, HTML, CSS, or JavaScript files.
- Client-side rendering: The client receives the response and renders the content to the user.
Understanding the request-response cycle is crucial for building efficient and scalable backend systems. By optimizing each stage of the cycle, developers can improve the performance, security, and reliability of their applications.
Core Backend Technologies
Backend development involves a range of technologies, each serving a specific purpose in the request-response cycle. Some of the most popular backend technologies include:
Programming Languages
- JavaScript: Node.js, Express.js, and Koa.js are popular JavaScript frameworks for building server-side applications.
- Python: Django, Flask, and Pyramid are popular Python frameworks for building web applications.
- Ruby: Ruby on Rails is a popular Ruby framework for building web applications.
Databases
- Relational databases: MySQL, PostgreSQL, and SQLite are popular relational databases for storing structured data.
- NoSQL databases: MongoDB, Cassandra, and Redis are popular NoSQL databases for storing unstructured or semi-structured data.
API Frameworks
- RESTful APIs: REST (Representational State of Resource) is an architectural style for designing networked applications. Popular RESTful API frameworks include Express.js, Django, and Flask.
- GraphQL: GraphQL is a query language for APIs that provides a more flexible and efficient alternative to RESTful APIs.
Server-Side Frameworks
- Containerization: Docker, Kubernetes, and container orchestration platforms enable developers to package and deploy backend applications efficiently.
- Microservices architecture: This architectural style involves breaking down a monolithic application into smaller, independent services that communicate with each other using APIs.
Best Practices for Backend Development
While there are many ways to approach backend development, adhering to best practices can help ensure the scalability, maintainability, and security of an application. Some key best practices include:
Code Organization and Structure
- Separation of Concerns: Organize code into modular, independent components that each serve a specific purpose.
- Modular Architecture: Break down a monolithic application into smaller, independent modules that can be developed and maintained separately.
Error Handling and Debugging
- Error Logging: Implement logging mechanisms to track and diagnose errors, ensuring that issues can be quickly identified and resolved.
- Error Handling: Implement try-catch blocks and error handling mechanisms to gracefully handle exceptions and errors.
Security and Authentication
- Authentication and Authorization: Implement secure authentication and authorization mechanisms to protect against unauthorized access.
- Data Encryption: Encrypt sensitive data, both in transit and at rest, to protect against data breaches.
Performance Optimization
- Caching: Implement caching mechanisms to reduce the load on backend systems and improve response times.
- Optimization: Optimize database queries, server-side logic, and API responses to minimize latency and improve performance.
Real-World Examples and Case Studies
To illustrate the concepts and best practices discussed above, let's consider a few real-world examples and case studies:
Example 1: Instagram's Backend Architecture
Instagram's backend architecture is built using a microservices approach, with multiple services communicating with each other using APIs. Each service is designed to handle a specific task, such as image processing, user authentication, or feed generation. This approach enables Instagram to scale its backend infrastructure efficiently, handling millions of users and requests per day.
Case Study: Airbnb's API Gateway
Airbnb's API gateway is built using a combination of Node.js, Express.js, and GraphQL. The gateway handles millions of API requests per day, providing a scalable and efficient interface for Airbnb's mobile and web applications. By using GraphQL, Airbnb's developers can query and fetch only the data required for each request, reducing latency and improving performance.
Conclusion
Backend development is a complex and multifaceted field, requiring a deep understanding of core technologies, best practices, and real-world examples. By mastering the request-response cycle, core backend technologies, and best practices, developers can build scalable, efficient, and secure backend systems that power the digital products and services we use every day. Whether you're building a simple web application or a complex enterprise system, understanding the basics of backend development is essential for success in the digital economy.