Securing your backend with security headers
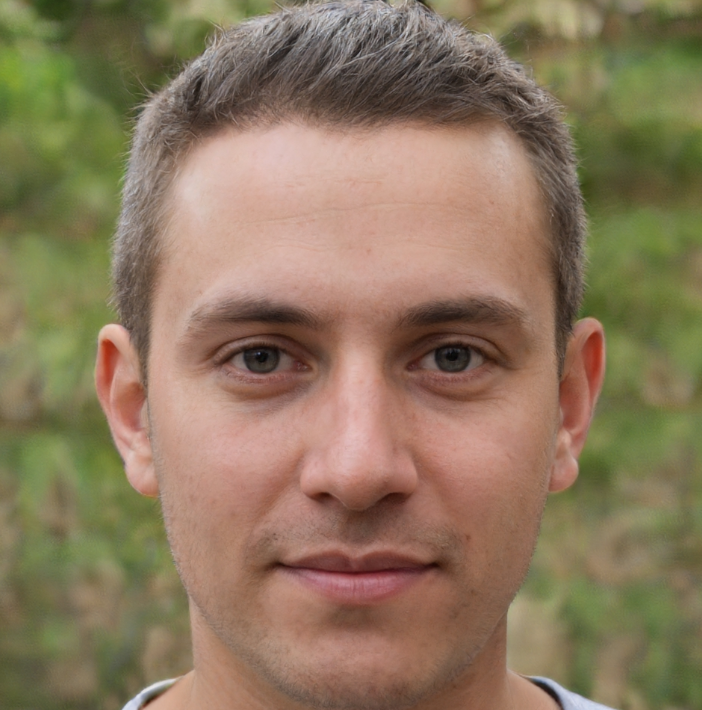
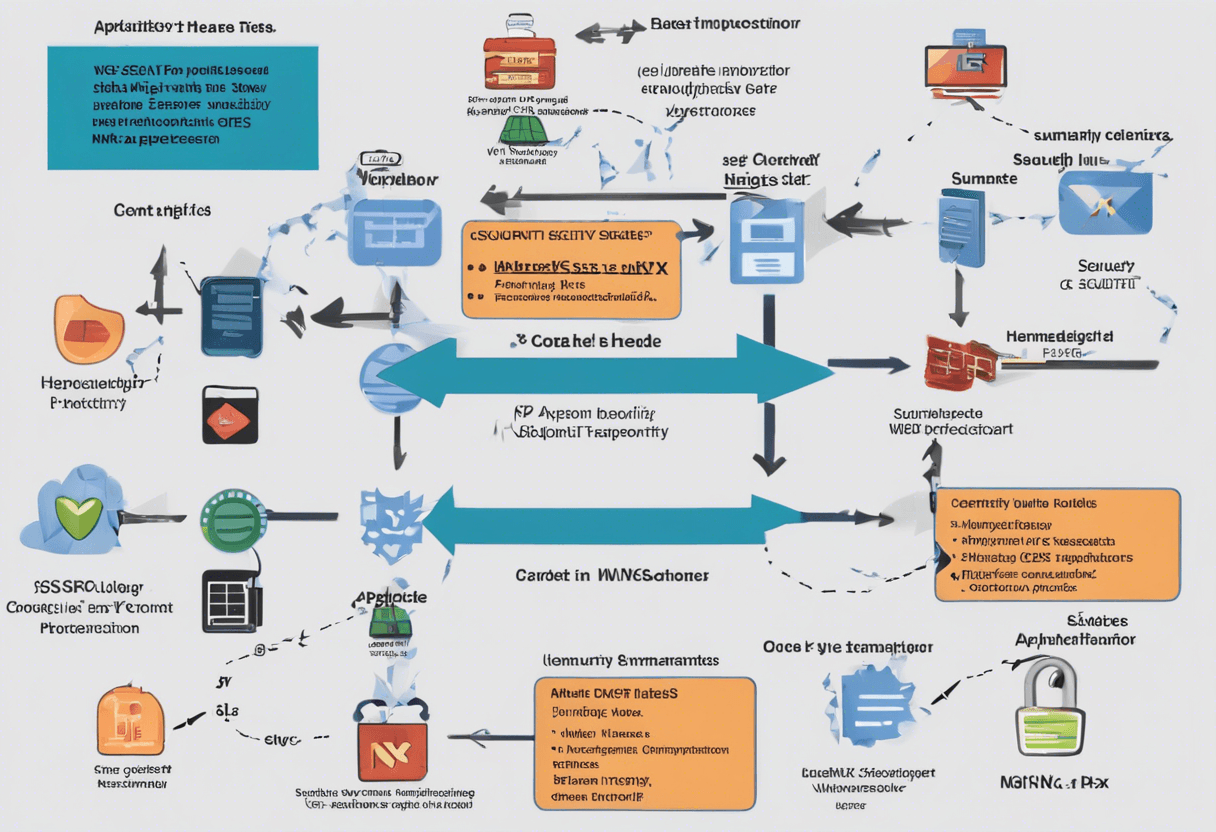
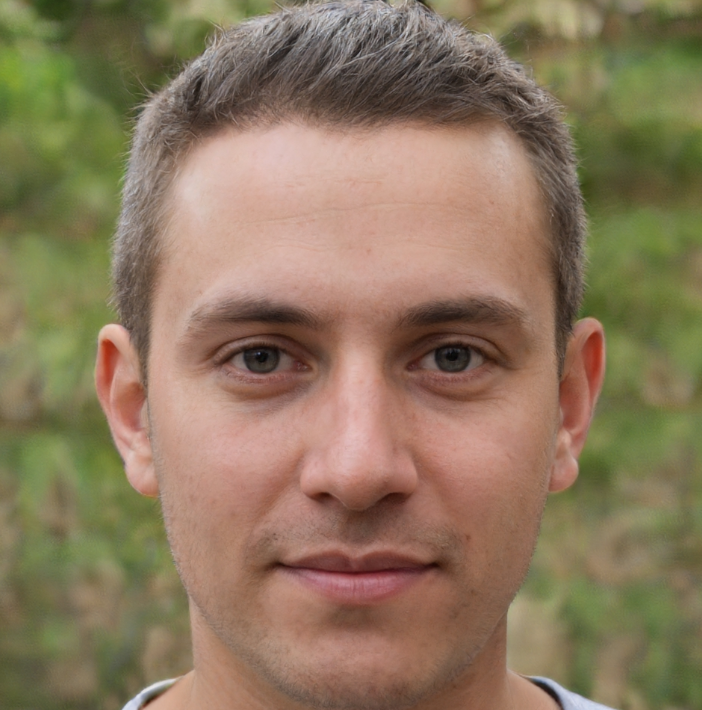
Securing Your Backend with Security Headers: A Comprehensive Guide
Security headers are an essential component of web application security, and their importance cannot be overstated. These headers provide an additional layer of protection against various types of attacks, including cross-site scripting (XSS), cross-site request forgery (CSRF), and clickjacking. In this article, we will explore the importance of security headers, the different types of headers, and how to implement them to secure your backend.
The Importance of Security Headers
Security headers are a critical component of web application security, as they provide a way to communicate security-related instructions to web browsers. These headers can help prevent a wide range of attacks, including:
- Cross-site scripting (XSS): Security headers, such as the Content-Security-Policy (CSP) header, can help prevent XSS attacks by specifying which sources of content are allowed to be executed within a web page.
- Cross-site request forgery (CSRF): Security headers, such as the X-XSS-Protection header, can help prevent CSRF attacks by specifying how web browsers should handle requests.
- Clickjacking: Security headers, such as the X-Frame-Options header, can help prevent clickjacking attacks by specifying how web browsers should handle iframes.
Types of Security Headers
There are several types of security headers that can be used to secure your backend. Some of the most common headers include:
- Content-Security-Policy (CSP) header: The CSP header is used to specify which sources of content are allowed to be executed within a web page. This header can help prevent XSS attacks by only allowing content from trusted sources.
- X-XSS-Protection header: The X-XSS-Protection header is used to specify how web browsers should handle requests. This header can help prevent CSRF attacks by only allowing requests from trusted sources.
- X-Frame-Options header: The X-Frame-Options header is used to specify how web browsers should handle iframes. This header can help prevent clickjacking attacks by only allowing iframes from trusted sources.
- Strict-Transport-Security (HSTS) header: The HSTS header is used to specify how web browsers should handle HTTPS connections. This header can help prevent man-in-the-middle attacks by only allowing HTTPS connections.
Implementing Security Headers
Implementing security headers is a relatively straightforward process that involves adding specific headers to your web server configuration. Here are some examples of how to implement security headers:
Apache Configuration
To implement security headers in Apache, you can add the following configuration to your httpd.conf
file:
# Content-Security-Policy (CSP) header
Header set Content-Security-Policy "default-src 'self'; script-src 'self' https://cdn.example.com; object-src 'none'"
# X-XSS-Protection header
Header set X-XSS-Protection "1; mode=block"
# X-Frame-Options header
Header set X-Frame-Options "SAMEORIGIN"
# Strict-Transport-Security (HSTS) header
Header set Strict-Transport-Security "max-age=31536000; includeSubDomains"
Nginx Configuration
To implement security headers in Nginx, you can add the following configuration to your nginx.conf
file:
# Content-Security-Policy (CSP) header
add_header Content-Security-Policy "default-src 'self'; script-src 'self' https://cdn.example.com; object-src 'none'";
# X-XSS-Protection header
add_header X-XSS-Protection "1; mode=block";
# X-Frame-Options header
add_header X-Frame-Options "SAMEORIGIN";
# Strict-Transport-Security (HSTS) header
add_header Strict-Transport-Security "max-age=31536000; includeSubDomains";
Node.js Configuration
To implement security headers in Node.js, you can use the helmet
module:
const express = require('express');
const helmet = require('helmet');
const app = express();
app.use(helmet.contentSecurityPolicy({
directives: {
defaultSrc: ["'self'"],
scriptSrc: ["'self'", 'https://cdn.example.com'],
objectSrc: ["'none'"],
},
}));
app.use(helmet.xssFilter());
app.use(helmet.frameguard({
action: 'sameorigin',
}));
app.use(helmet.hsts({
maxAge: 31536000,
includeSubdomains: true,
}));
Best Practices
Here are some best practices to keep in mind when implementing security headers:
- Use a Content-Security-Policy (CSP) header: A CSP header can help prevent XSS attacks by specifying which sources of content are allowed to be executed within a web page.
- Use an X-XSS-Protection header: An X-XSS-Protection header can help prevent CSRF attacks by specifying how web browsers should handle requests.
- Use an X-Frame-Options header: An X-Frame-Options header can help prevent clickjacking attacks by specifying how web browsers should handle iframes.
- Use a Strict-Transport-Security (HSTS) header: An HSTS header can help prevent man-in-the-middle attacks by only allowing HTTPS connections.
Regularly Review and Update Security Headers
It's impotant to regulary review and update your security headers to ensure they are still efective. This can be done by:
- Monitoring security header reports: Many web browsers and security tools provide reports on security headers. These reports can help identify any issues or weaknesses in your security headers.
- Testing security headers: Regularly testing your security headers can help identify any issues or weaknesses. This can be done using tools such as OWASP ZAP or Burp Suite.
- Updating security headers: Based on the results of your review and testing, update your security headers to ensure they are still efective.
Conclusion
Securing your backend with security headers is a critical component of web application security. By implementing security headers, you can help prevent a wide range of attacks, including XSS, CSRF, and clickjacking. Remember to follow best practices when implementing security headers, such as using a CSP header, an X-XSS-Protection header, an X-Frame-Options header, and an HSTS header. By following these best practices, you can help ensure the security of your web application and protect your users from potential attacks.