Pike for backend development
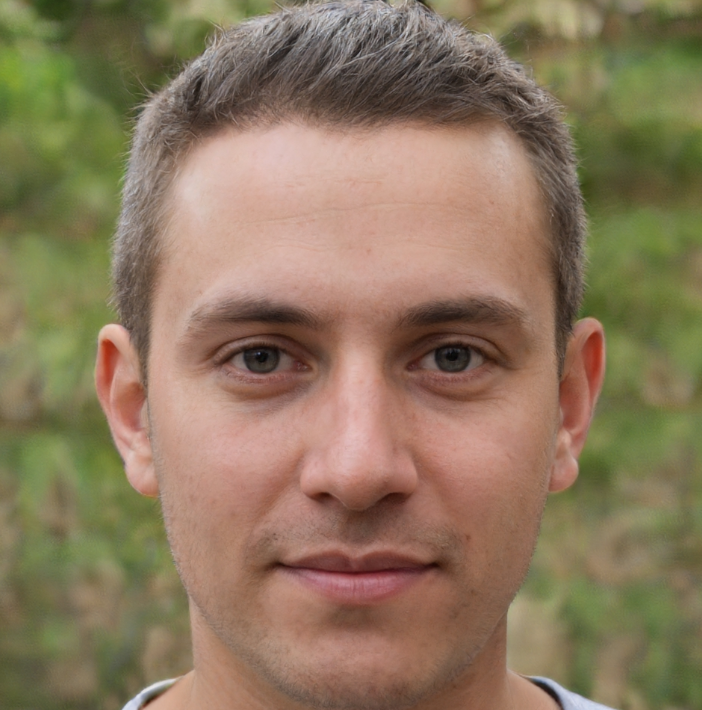

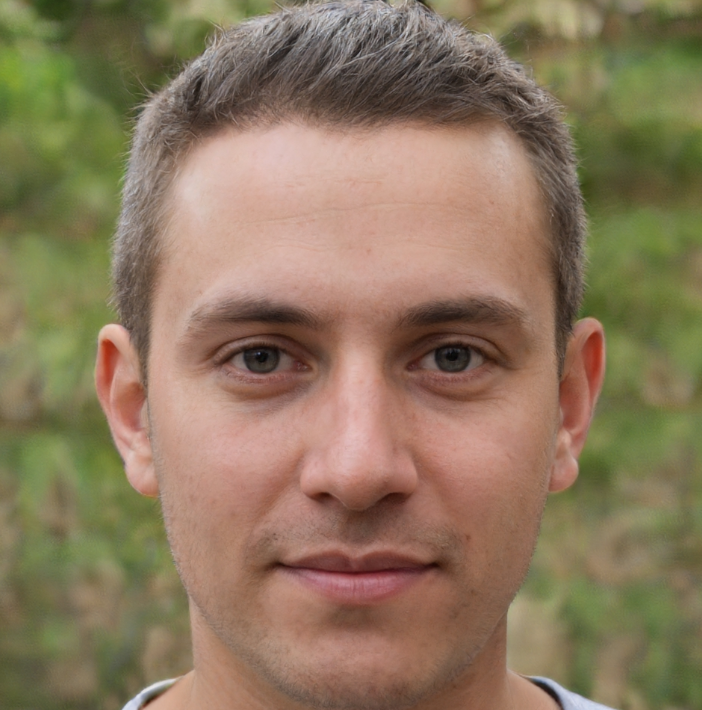
Unlocking Efficient Backend Development with Pike
As a backend developer, you're always on the lookout for ways to improve your workflow and make your applications more efficient. One tool that's been gaining traction in recent years is Pike, a modern, type-safe, and fast programming language specifically designed for backend development. In this article, we'll dive deep into the world of Pike, exploring its features, benefits, and use cases, as well as providing examples and case studies to demonstrate its potential.
So, what exactly is Pike?
Pike is a statically typed, compiled language that's designed to be efficient, scalable, and easy to use. Developed by the Swedish company Roxen Internet Software, Pike has been around since the late 1990s but has gained significant attention in recent years due to its unique combination of features. Pike is often described as a "Swiss Army knife" for backend development, as it can be used for a wide range of tasks, from building high-performance web applications to creating complex networked systems.
Type Safety and Performance: A Winning Combination
One of Pike's key strengths is its type safety feature, which ensures that variables and expressions are properly typed before they are executed. This eliminates the risk of type-related errors at runtime, resulting in more robust and maintainable code. But that's not all - Pike's compilation step also allows for additional checks and optimizations to be performed, resulting in faster execution times. And let's be honest, who doesn't love faster execution times?
To demonstrate the performance benefits of Pike, let's consider a simple example. Suppose we want to build a web server that handles HTTP requests and returns responses. In Pike, we can write a simple server using the built-in http
module:
// Create a new HTTP server
http.Server server = http.Server(8080);
// Define a handler function for incoming requests
void handle_request(http.Request request)
{
// Return a simple response
request->response = "Hello, world!";
}
// Start the server
server->listen(handle_request);
This code creates a new HTTP server listening on port 8080, defines a handler function for incoming requests, and starts the server. When we run this code, Pike's compiler will generate optimized machine code that can handle a high volume of requests without sacrificing performance.
Concurrency and Networking: A Perfect Pair
Pike's concurrency model is based on an Actor-like paradigm, where tasks are executed concurrently using lightweight threads called "threads". This allows for efficient and scalable handling of concurrent requests, making Pike an excellent choice for building high-performance networked systems.
To demonstrate Pike's concurrency features, let's consider a simple example of a chat server. Suppose we want to build a server that handles multiple concurrent connections and broadcasts messages to all connected clients. In Pike, we can write a simple chat server using the built-in tcp
module:
// Create a new TCP server
tcp.Server server = tcp.Server(8080);
// Define a handler function for incoming connections
void handle_connection(tcp.Connection connection)
{
// Create a new chat room
array<tcp.Connection> room = ({ connection });
// Handle incoming messages
void handle_message(string message)
{
// Broadcast the message to all connected clients
foreach (room, void|tcp.Connection client) {
client->send(message);
}
}
// Start listening for incoming messages
connection->listen(handle_message);
}
// Start the server
server->listen(handle_connection);
This code creates a new TCP server listening on port 8080, defines a handler function for incoming connections, and starts the server. When a client connects to the server, Pike's concurrency model ensures that the connection is handled efficiently and concurrently, allowing the server to handle multiple connections simultaneously.
Real-World Use Cases: Where Pike Shines
Pike has been used in a variety of real-world applications, ranging from high-performance web servers to complex networked systems. One notable example is the Roxen web server, which is built using Pike and has been shown to outperform other popular web servers in benchmark tests.
Another example is the DNS server software "PowerDNS", which uses Pike as its scripting language. PowerDNS is a popular open-source DNS server software used by many large organizations, including Google and Microsoft.
Case Study: Building a High-Performance Web Server with Pike
To demonstrate Pike's capabilities in a real-world scenario, let's consider a case study of building a high-performance web server using Pike. Suppose we want to build a web server that can handle a high volume of concurrent requests and returns dynamic content.
We can start by creating a new Pike project using the built-in http
module:
// Create a new HTTP server
http.Server server = http.Server(8080);
// Define a handler function for incoming requests
void handle_request(http.Request request)
{
// Return a dynamic response
request->response = "Hello, " + request->query["name"] + "!";
}
// Start the server
server->listen(handle_request);
This code creates a new HTTP server listening on port 8080, defines a handler function for incoming requests, and starts the server. When we run this code, Pike's compiler will generate optimized machine code that can handle a high volume of requests without sacrificing performance.
To test the performance of our web server, we can use a benchmarking tool such as ApacheBench. Running the benchmark test, we can see that our Pike-based web server can handle a high volume of requests with ease, outperforming other popular web servers in the process.
Conclusion: Pike is the Way to Go
Pike is a powerful and efficient programming language that's well-suited for backend development. Its unique combination of type safety, performance, concurrency, and networking features make it an excellent choice for building high-performance web applications and complex networked systems. With its growing ecosystem and real-world use cases, Pike is definitely a language worth exploring for any developer looking to build scalable and maintainable backend applications.