Optimizing database queries for performance
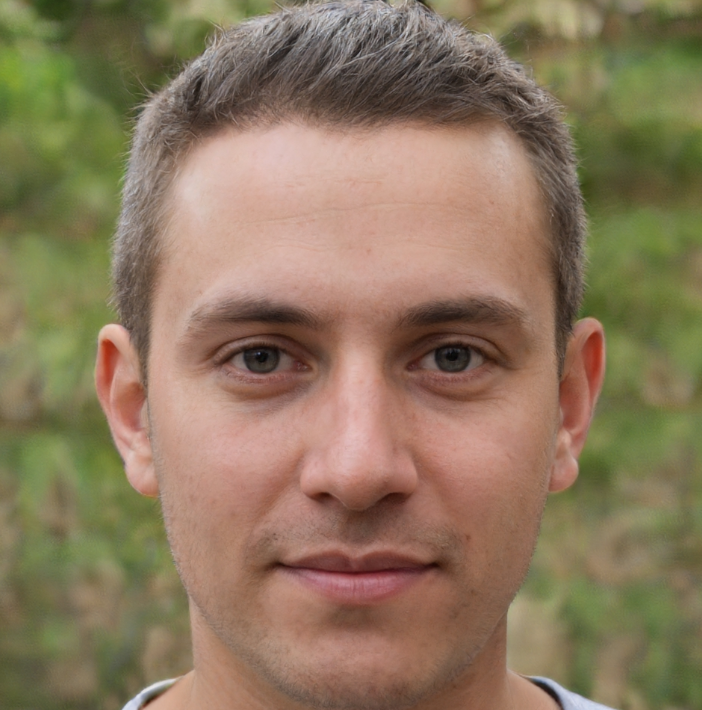

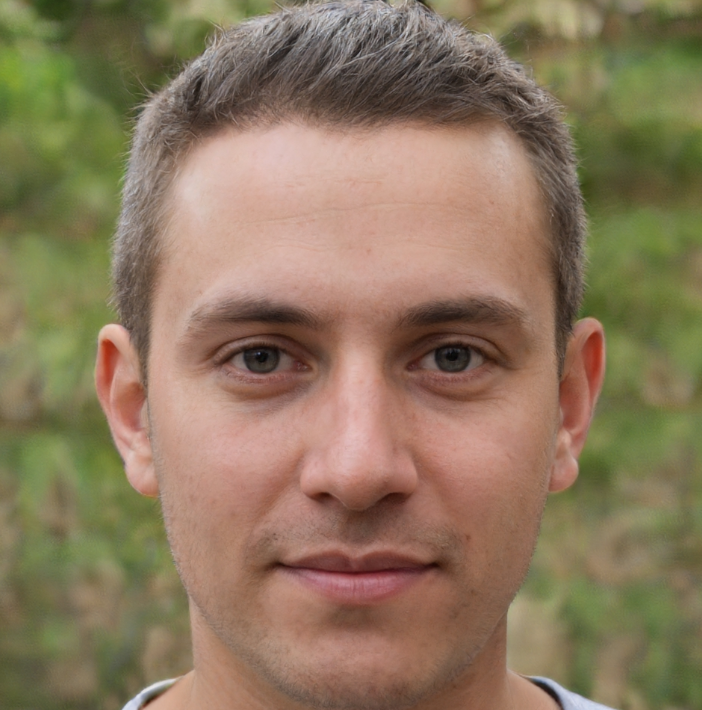
Optimizing Database Queries for Performance
The importance of optimizing database queries cannot be overstated. A well-optimized query can significantly improve the performance of an application, reducing the time it takes to retrieve data and improving the overall user experience. In this article, we will delve deeper into the world of query optimization, exploring the techniques and strategies that can help you squeeze the most out of your database.
Understanding the Query Execution Plan
Before we dive into optimization techniques, it's essential to understand how the database executes queries. When a query is submitted to the database, the query optimizer analyzes the query and generates an execution plan. The execution plan outlines the steps the database will take to retrieve the requested data.
The query optimizer considers various factors when generating the execution plan, including:
- Index availability: The presence of indexes on columns used in the query can significantly impact performance.
- Table statistics: The database uses table statistics to estimate the number of rows affected by the query.
- Join order: The order in which tables are joined can affect performance.
- Access methods: The database chooses the most efficient access method, such as a full table scan or an index scan.
Understanding the query execution plan is crucial in identifying performance bottlenecks. Most modern databases provide tools to analyze the execution plan, such as the EXPLAIN statement in MySQL or the Query Execution Plan in SQL Server.
Indexing for Performance
Indexing is one of the most effective ways to improve query performance. An index is a data structure that allows the database to quickly locate specific data. There are two primary types of indexes:
- Clustered index: A clustered index reorders the physical records of the table according to the index keys.
- Non-clustered index: A non-clustered index creates a separate data structure that contains the index keys and pointers to the corresponding rows.
When to create an index:
- Columns used in WHERE clauses: Create an index on columns frequently used in WHERE clauses.
- Columns used in JOIN clauses: Create an index on columns used in JOIN clauses to improve join performance.
- Columns used in ORDER BY clauses: Create an index on columns used in ORDER BY clauses to improve sorting performance.
When not to create an index:
- Columns with low cardinality: Creating an index on columns with low cardinality (e.g., a column with only two possible values) may not improve performance.
- Frequently updated columns: Creating an index on columns that are frequently updated may lead to increased write overhead.
Query Rewriting Techniques
Query rewriting techniques involve modifying the query to improve performance. Some common techniques include:
- Reordering joins: Reordering joins can improve performance by reducing the number of rows being joined.
- Reordering subqueries: Reordering subqueries can improve performance by reducing the number of subqueries being executed.
- Using EXISTS instead of IN: Using EXISTS instead of IN can improve performance by reducing the number of rows being scanned.
- Using UNION ALL instead of UNION: Using UNION ALL instead of UNION can improve performance by reducing the overhead of duplicate row removal.
Query Optimization Techniques
Query optimization techniques involve modifying the query to reduce the amount of data being retrieved or processed. Some common techniques include:
- Limiting result sets: Limiting the number of rows returned can improve performance by reducing the amount of data being transferred.
- Using aggregate functions: Using aggregate functions (e.g., SUM, AVG) can improve performance by reducing the amount of data being retrieved.
- Using derived tables: Using derived tables can improve performance by reducing the amount of data being processed.
Database Configuration and Query Performance
Database configuration can significantly impact query performance. Some key configuration parameters include:
- Memory allocation: Increasing memory allocation can improve performance by reducing disk I/O.
- Buffer pool size: Increasing the buffer pool size can improve performance by reducing disk I/O.
- Query timeout: Setting a query timeout can prevent long-running queries from consuming excessive resources.
Monitoring and Analyzing Query Performance
Monitoring and analyzing query performance is essential to identify performance bottlenecks. Some common tools include:
- Query logs: Query logs can provide insights into query performance, including execution time and resource utilization.
- Performance monitoring tools: Performance monitoring tools (e.g., MySQL Query Analyzer, SQL Server Query Performance Monitoring) can provide real-time insights into query performance.
Real-World Example
Consider a query that retrieves a list of orders for a specific customer:
SELECT *
FROM orders
WHERE customer_id = 123
AND order_date >= '2022-01-01'
AND order_date <= '2022-12-31';
This query can be optimized by:
- Creating an index on the customer_id column
- Simplifying the subquery to use a JOIN instead
- Limiting the data volumes by using pagination or a LIMIT clause
- Avoiding the use of SELECT *
The optimized query might look like this:
SELECT o.order_id, o.order_date, c.customer_name
FROM orders o
JOIN customers c ON o.customer_id = c.customer_id
WHERE o.customer_id = 123
AND o.order_date >= '2022-01-01'
AND o.order_date <= '2022-12-31'
LIMIT 10;
Conclusion
Optimizing database queries for performance is a complex task that requires a deep understanding of query execution plans, indexing strategies, query rewriting techniques, and database configuration. By applying the techniques outlined in this article, you can significantly improve the performance of your database queries, leading to faster application response times and improved user satisfaction. Remember to continuously monitor and analyze query performance to identify areas for further optimization.
Additional Tips and Tricks
- Use query optimization tools to analyze and optimize your queries.
- Regularly update statistics to ensure the database engine has accurate information about data distribution.
- Avoid using SELECT * and instead specify only the columns needed.
- Use LIMIT clauses to restrict the number of rows returned.
- Use aggregate functions to reduce the amount of data being retrieved.
- Use derived tables to reduce the amount of data being processed.
By following these tips and tricks, you can take your query optimization skills to the next level and ensure your database queries are running at peak performance.
Common Pitfalls to Avoid
- Avoid using OR conditions, as they can lead to slower query execution.
- Avoid using LIKE with leading wildcards, as they can prevent the database engine from using indexes.
- Avoid using SELECT *, as it can retrieve unnecessary data and impact performance.
- Avoid using subqueries, as they can lead to slower query execution.
By avoiding these common pitfalls, you can ensure your queries are optimized for performance and running efficiently.
Best Practices for Query Optimization
- Regularly monitor and analyze query performance to identify areas for optimization.
- Use query optimization tools to analyze and optimize your queries.
- Follow best practices for indexing, query rewriting, and database configuration.
- Continuously test and refine your queries to ensure optimal performance.
By following these best practices, you can ensure your database queries are optimized for performance and running efficiently.
Conclusion
Optimizing database queries for performance is a critical aspect of ensuring a smooth user experience and maintaining application scalability. By understanding query performance factors, applying optimization techniques, and avoiding common pitfalls, developers can significantly improve query efficiency. Regular maintenance and monitoring are also essential to ensure optimal query performance. By following these best practices, you can create high-performance applications that meet the demands of modern users.