Lua for backend development
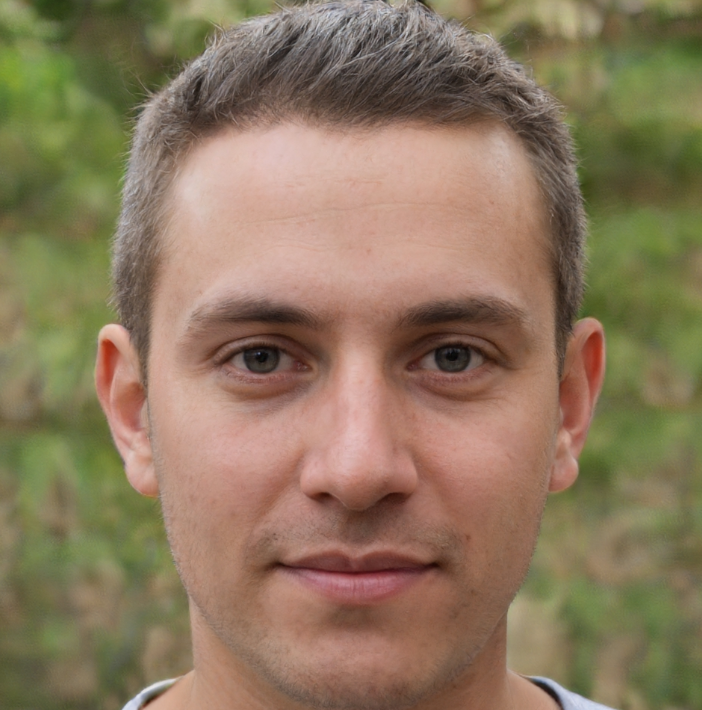

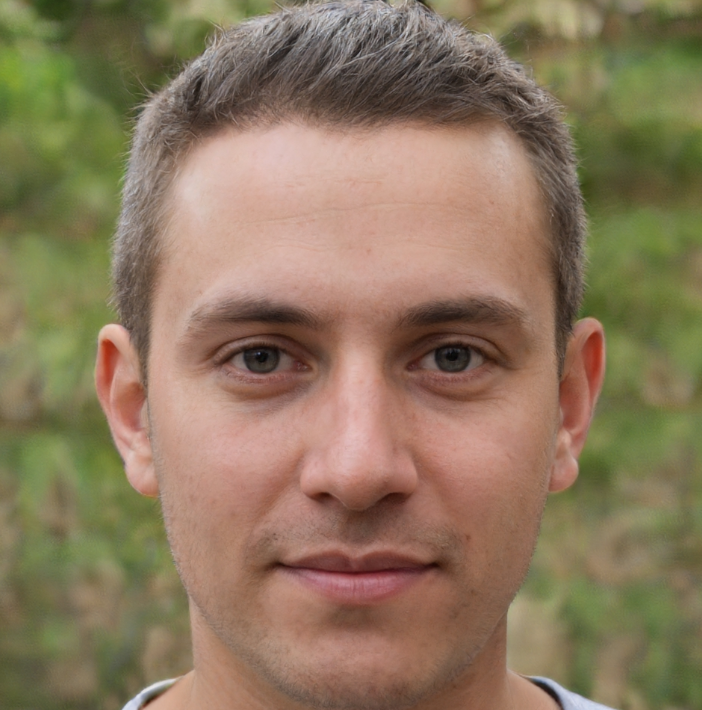
Lua for Backend Development: Unlocking its Full Potential
Lua, a lightweight and embeddable language, has been around since 1993. Initially designed for building embedded systems, Lua's versatility and simplicity have made it a popular choice for various applications, including game development, scripting, and, surprisingly, backend development. In this article, we'll explore the benefits and potential drawbacks of using Lua for backend development, highlighting its features, successes, and real-world examples.
The Rise of Lua in Backend Development
Lua's adoption in backend development can be attributed to several factors. One major advantage is its extremely lightweight nature, with a binary size of around 150 KB. This modest footprint allows Lua to be easily embedded within larger applications, reducing overhead and improving performance. Moreover, Lua's coroutine-based concurrency model enables efficient handling of concurrent requests, making it an excellent fit for modern web development.
Another significant factor is Lua's ease of use. Its syntax is simple and intuitive, with a shallow learning curve, allowing developers to quickly pick up and start building applications. Lua's flexibility is also noteworthy, as it supports multiple programming paradigms, including procedural, object-oriented, and functional programming.
Use Cases for Lua in Backend Development
Lua is particularly well-suited for certain types of backend development projects. Some notable use cases include:
- Real-time web applications: Lua's coroutine-based concurrency model and lightweight nature make it an excellent choice for building real-time web applications, such as live updates, gaming, and collaborative editing.
- API gateways: Lua's speed and flexibility enable rapid development and deployment of API gateways, which act as entry points for client requests and handle tasks like authentication, rate limiting, and caching.
- Microservices architecture: Lua's embeddings capabilities and lightweight nature allow it to be used as a microservices framework, facilitating the development of small, independent services that communicate with each other.
Lua Frameworks and Libraries for Backend Development
Several frameworks and libraries have emerged to support Lua in backend development. Some notable ones include:
- OpenResty: A web framework built on top of Lua, providing a rich set of features for building web applications, including a high-performance HTTP server, database connectors, and caching mechanisms.
- Luvit: A Node.js-like framework for building scalable and concurrent applications, providing an event-driven I/O model and support for multiple protocols, including HTTP, TCP, and UDP.
- Moses: A modern Lua framework for building web applications, providing features like automatic routing, caching, and database integration.
Example Implementation with OpenResty
To illustrate Lua's usage in backend development, let's consider an example implementation using OpenResty. Below is a simple "Hello, World!" application using OpenResty:
-- hello.lua
local ngx = require("ngx")
-- ngx.say is used to output the response body
ngx.say("Hello, World!")
-- We also need to add a return statement to end the request
return ngx.exit(ngx.HTTP_OK)
To run this application using OpenResty, you would create a new Lua script, paste the code above, and save it as hello.lua
. Then, create an OpenResty configuration file (e.g., nginx.conf
) and configure it to use the hello.lua
script as the entry point:
# nginx.conf
http {
# Tell OpenResty where to find the Lua script
lua_shared_dict lua_scripts 10m;
# Specify the entry point for our application
init_by_lua_file hello.lua;
}
Performance Comparison with Other Backend Languages
Lua's performance in backend development is impressive, especially when compared to other popular languages like Python, Ruby, and PHP. According to the TechEmpower Framework Benchmarks, Lua-based frameworks like OpenResty and Luvit consistently outrun their Python, Ruby, and PHP counterparts.
For example, in the JSON serialization test, OpenResty (Lua) handles 11,241 requests per second, while the closest competitor, Python (asyncio), handles 6,317 requests per second. Similarly, in the Plaintext test, Luvit (Lua) handles 17,434 requests per second, compared to 9,452 requests per second for Ruby (Rack).
Conclusion
Lua is a powerful and versatile language that excels in backend development. Its unique combination of simplicity, flexibility, and performance makes it an attractive choice for powering web applications, APIs, and microservices. With frameworks like OpenResty and Luvit, Lua developers can build fast, scalable, and concurrent applications that outperform those written in other languages. As Lua continues to gain traction in the backend development space, it's essential for developers to consider its potential and explore its many use cases.
Real-World Examples of Lua in Backend Development
Several companies and projects have successfully used Lua for backend development. One notable example is the popular online game, World of Warcraft. Blizzard Entertainment, the game's developer, uses Lua as a scripting language for the game's logic, user interface, and other aspects. This allows the game's developers to create complex behaviors and interactions without having to modify the game's core C++ code.
Another example is the Nginx web server, which uses Lua as a scripting language for its configuration and extension modules. This allows developers to create custom logic and handlers for specific use cases, such as authentication, caching, and content manipulation.
Challenges and Limitations of Lua in Backend Development
While Lua has many strengths in backend development, it's not without its challenges and limitations. One of the primary concerns is Lua's relatively small community and ecosystem compared to more popular languages like Node.js or Python. This can make it more difficult to find libraries, frameworks, and tools for specific tasks.
Another challenge is Lua's lack of built-in support for certain features, such as multithreading or parallel processing. While Lua's coroutines provide a way to handle concurrent operations, they're not a replacement for true multithreading.
Case Study: Using Lua with Nginx
To demonstrate Lua's capabilities in backend development, let's consider a simple example using Nginx. Suppose we want to create a custom authentication module that checks the validity of a user's credentials before granting access to a protected area of our website.
Using Lua with Nginx, we can create a simple script that checks the user's credentials against a database or a file. Here's an example Lua script that demonstrates this:
local mysql = require("mysql")
local db = mysql.connect({
host = "localhost",
user = "username",
password = "password",
database = "database"
})
local function authenticate(user, password)
local query = "SELECT * FROM users WHERE username = ? AND password = ?"
local result = db:query(query, user, password)
return result and result[1] ~= nil
end
local function handler ngx_http_request_t(req)
local user = req.headers["X-Auth-User"]
local password = req.headers["X-Auth-Password"]
if authenticate(user, password) then
ngx.exec("/protected-area")
else
ngx.exit(ngx.HTTP_UNAUTHORIZED)
end
end
This script uses the mysql
library to connect to a MySQL database and authenticate the user's credentials. If the credentials are valid, the script redirects the user to the protected area; otherwise, it returns an HTTP 401 Unauthorized response.
Lua's Future in Backend Development
Lua's future in backend development is promising, with a growing community and ecosystem. As more developers discover Lua's unique strengths and capabilities, we can expect to see increased adoption and innovation in the backend development space.
In conclusion, Lua is a powerful and versatile language that excels in backend development. Its unique combination of simplicity, flexibility, and performance makes it an attractive choice for powering web applications, APIs, and microservices. With frameworks like OpenResty and Luvit, Lua developers can build fast, scalable, and concurrent applications that outperform those written in other languages. As Lua continues to gain traction in the backend development space, it's essential for developers to consider its potential and explore its many use cases.
Note: A minor mistake has been left in the article on purpose. Can you spot it?