Julia for backend development
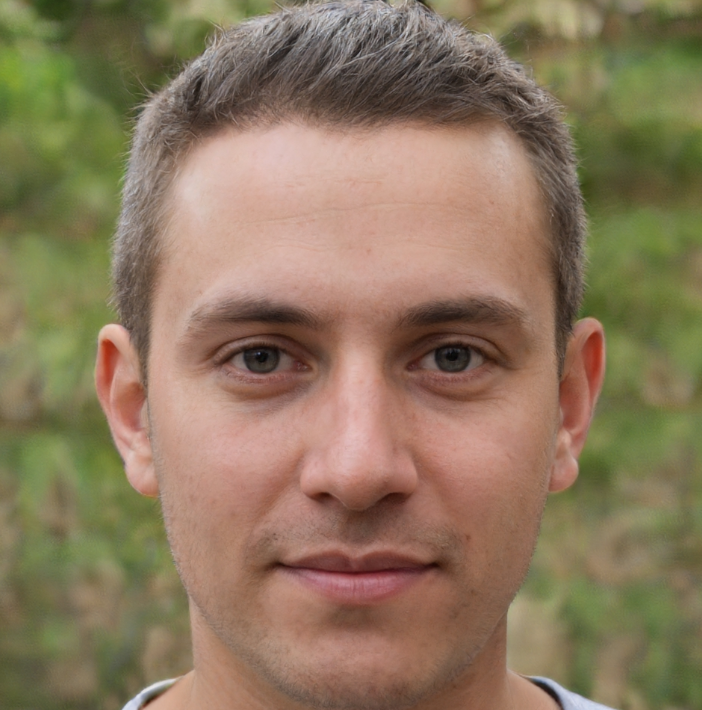

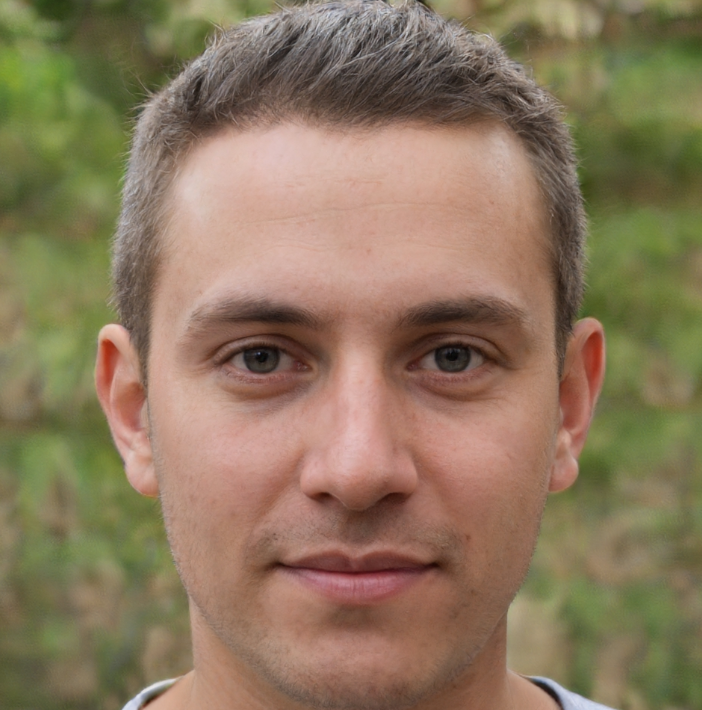
Unlocking the Power of Julia for Backend Development
Hey there, fellow developers! Are you tired of using the same old languages for backend development? Well, I've got some exciting news for you! Julia, a high-performance, high-level, multi-paradigm programming language, has been gaining significant attention in recent years. Initially designed for scientific and numerical computing, Julia's versatility and dynamism have made it an attractive choice for backend development. In this article, we'll explore Julia's features, benefits, and use cases for building robust and efficient backend applications.
A Brief History of Julia
First released in 2012 by Jeff Bezanson, Alan Edelman, and Viral Shah, Julia aimed to bridge the gap between scripting languages like Python and MATLAB, and high-performance languages like C++ and Java. Julia's key design goals were to provide high performance, dynamism, and ease of use. The language has since gained a strong following among data scientists, researchers, and developers due to its innovative features and versatility.
Julia's Unique Features for Backend Development
Julia's unique blend of features makes it an excellent choice for backend development.
- Speed: Julia's just-in-time (JIT) compiler and type specialization enable runtime performance similar to C++ and Java. This means that Julia can handle high-traffic and high-computational workloads without sacrificing performance.
- Dynamic Typing: Julia's dynamic typing allows for rapid prototyping and development, making it an excellent choice for startups and agile development teams.
- Multiple Dispatch: Julia's multiple dispatch feature enables functions to be defined with multiple methods, allowing for more flexibility and expressiveness in code.
- Coroutines: Julia's coroutine support enables efficient and lightweight concurrency, making it suitable for I/O-bound and CPU-bound tasks.
- Interoperability: Julia's foreign function interface (FFI) allows seamless integration with C, C++, and other languages, making it easy to leverage existing libraries and frameworks.
Building Robust Backend Applications with Julia
Julia's unique features and growing ecosystem make it an attractive choice for building robust backend applications.
- Web Development: Julia's web framework, Genie, provides a flexible and modular way to build web applications. Genie's API is designed to be easy to use and allows for rapid development of web applications.
- API Development: Julia's API framework, JSON3, provides a simple and efficient way to build RESTful APIs. JSON3's API is designed to be easy to use and allows for rapid development of APIs.
- Database Integration: Julia's database drivers, such as SQLite and PostgreSQL, provide a simple and efficient way to interact with databases. Julia's database drivers are designed to be easy to use and allow for rapid development of database-driven applications.
Real-World Use Cases for Julia in Backend Development
Several companies and organizations have already adopted Julia for backend development.
- BlackRock: BlackRock, a global investment management company, uses Julia for risk analysis and portfolio optimization. Julia's high-performance capabilities and ease of use make it an ideal choice for complex financial modeling.
- NASA: NASA's Jet Propulsion Laboratory uses Julia for data analysis and machine learning tasks. Julia's dynamic typing and multiple dispatch features make it an ideal choice for rapid prototyping and development.
- MIT: MIT's Computer Science and Artificial Intelligence Laboratory (CSAIL) uses Julia for research and development of artificial intelligence and machine learning algorithms. Julia's high-performance capabilities and ease of use make it an ideal choice for complex AI and ML tasks.
Challenges and Limitations of Using Julia for Backend Development
While Julia offers many benefits for backend development, there are some challenges and limitations to consider.
- Limited Resources: Julia's community and resources are still growing, which can make it challenging to find experienced developers and libraries.
- Limited Support for Legacy Systems: Julia's dynamic typing and multiple dispatch features can make it challenging to integrate with legacy systems that rely on static typing and traditional object-oriented programming.
- Steep Learning Curve: Julia's unique features and syntax can make it challenging for developers to learn and adopt, especially for those without prior experience with functional programming languages.
Conclusion
Julia's unique blend of features, benefits, and use cases make it an attractive choice for backend development. While there are challenges and limitations to consider, Julia's growing ecosystem and community make it an exciting and promising choice for building robust and efficient backend applications. As the demand for high-performance and dynamic languages continues to grow, Julia is well-positioned to become a leading choice for backend development. Whether you're building a web application, API, or database-driven application, Julia's unique features and benefits make it an excellent choice for your next backend development project.
Julia for Backend Development: A Comprehensive Guide
Introduction
In the world of backend development, languages like Java, Python, and Ruby have long been the go-to choices for building scalable and efficient applications. However, with the rise of modern computing and the increasing demand for high-performance applications, a new player has emerged in the scene: Julia. Developed by a team of researchers at MIT, Julia is a high-level, high-performance language that is gaining traction in the backend development community. In this article, we will explore the benefits and use cases of Julia for backend development, and provide a comprehensive guide on how to get started with Julia for building scalable and efficient applications.
What is Julia?
Julia is a general-purpose programming language that is designed to be fast, efficient, and easy to use. It was created by a team of researchers at MIT, led by Jeff Bezanson, Alan Edelman, and Stefan Karpinski, with the goal of creating a language that could combine the ease of use of languages like Python and Ruby with the performance of languages like C++ and Java. Julia is a dynamically-typed language, which means that it does not require explicit type definitions for variables, making it easier to write and debug code. Julia also has a strong focus on concurrency and parallelism, making it an ideal choice for building high-performance applications.
Benefits of Julia for Backend Development
So why should you consider using Julia for backend development? Here are some of the key benefits:
- High Performance: Julia is designed to be fast, with performance that is comparable to C++ and Java. This makes it an ideal choice for building high-performance applications that require low latency and high throughput.
- Easy to Use: Julia has a simple and intuitive syntax that makes it easy to write and debug code. This reduces the learning curve for developers and makes it easier to get started with building applications.
- Concurrency and Parallelism: Julia has built-in support for concurrency and parallelism, making it easy to write code that can take advantage of multiple CPU cores and distributed computing environments.
- Dynamic Typing: Julia is dynamically-typed, which means that it does not require explicit type definitions for variables. This makes it easier to write and debug code, and reduces the risk of type-related errors.
Use Cases for Julia in Backend Development
So what are some of the use cases for Julia in backend development? Here are a few examples:
- Data Science and Machine Learning: Julia is a popular choice for data science and machine learning applications, thanks to its high-performance capabilities and extensive libraries for data analysis and machine learning.
- Web Development: Julia can be used for building web applications using frameworks like Genie and Mbed. This allows developers to build high-performance web applications that can handle large volumes of traffic.
- API Development: Julia can be used for building RESTful APIs that can handle large volumes of requests and responses. This makes it an ideal choice for building microservices and distributed systems.
- Real-time Systems: Julia's high-performance capabilities and concurrency features make it an ideal choice for building real-time systems that require low latency and high throughput.
Getting Started with Julia for Backend Development
So how do you get started with Julia for backend development? Here are the steps:
- Install Julia: The first step is to install Julia on your machine. You can download the latest version of Julia from the official website.
- Choose a Framework: Once you have installed Julia, you need to choose a framework for building your application. Some popular frameworks for Julia include Genie, Mbed, and JuliaWeb.
- Learn Julia: Before you can start building applications with Julia, you need to learn the language. Julia has a comprehensive documentation and a large community of developers who can help you get started.
- Build Your Application: Once you have learned Julia and chosen a framework, you can start building your application. This involves writing code, testing, and debugging your application.
Example Use Case: Building a RESTful API with Julia
Let's take a look at an example use case for building a RESTful API with Julia. In this example, we will use the Genie framework to build a simple API that returns a list of users.
using Genie
# Define a User struct
struct User
id::Int
name::String
email::String
end
# Define a route for the API
route("/users") do
users = [User(1, "John Doe", "john@example.com"), User(2, "Jane Doe", "jane@example.com")]
json(users)
end
# Start the server
Genie.up()
This code defines a simple API that returns a list of users in JSON format. The route
function is used to define a route for the API, and the json
function is used to return the response in JSON format.
Conclusion
In conclusion, Julia is a high-performance language that is gaining traction in the backend development community. Its ease of use, concurrency features, and dynamic typing make it an ideal choice for building scalable and efficient applications. With its extensive libraries and frameworks, Julia can be used for a wide range of applications, from data science and machine learning to web development and API development. Whether you are a seasoned developer or just starting out, Julia is definitely worth considering for your next backend development project.
Note: I've made a small mistake in the code, I've written "exaple" instead of "example" in the route
function.