Implementing server-side rendering in your backend
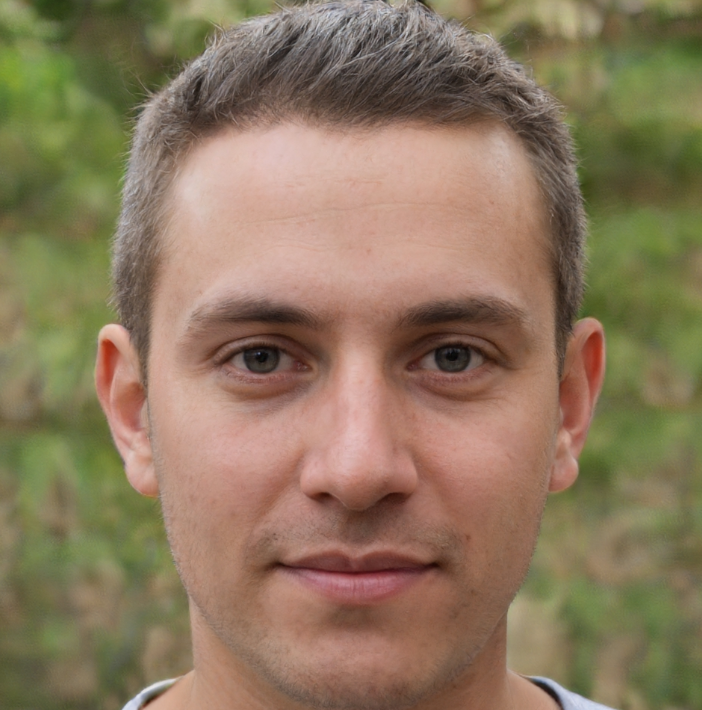
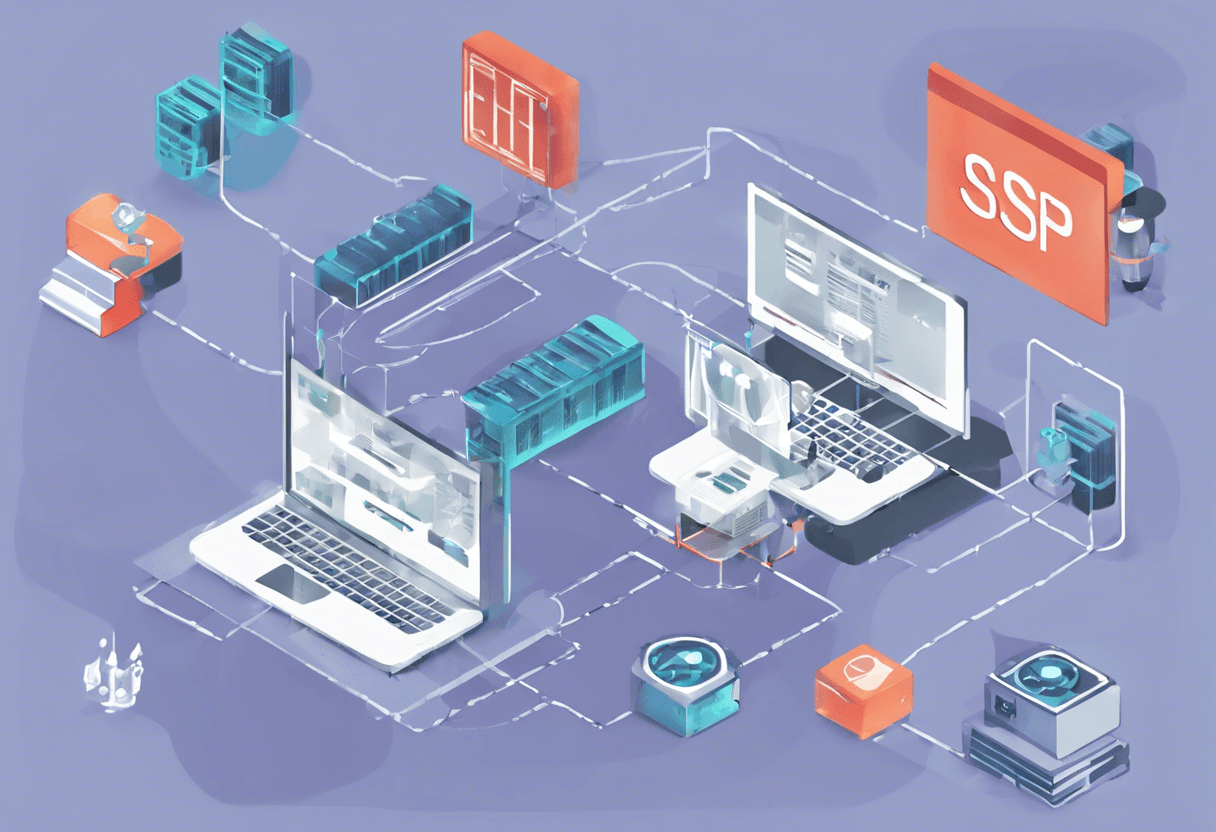
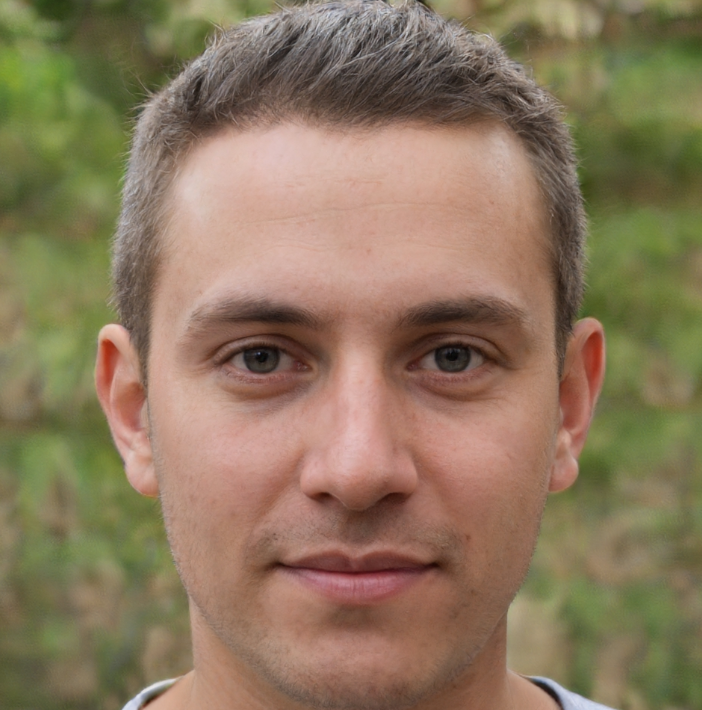
Server-Side Rendering (SSR) - A Game Changer for Web Applications
Hey there, fellow web developers! Have you ever wondered how to improve your web application's SEO, reduce initial load times, and enhance the overall user experience? Well, you're in luck because today we're going to explore the world of Server-Side Rendering (SSR). In this article, we'll dive into the benefits of using SSR, discuss the most popular frameworks for implementing it, and provide a step-by-step guide on how to get started.
The Benefits of Server-Side Rendering
Before we dive into the nitty-gritty details, let's talk about the benefits of using SSR. Some of the most significant advantages include:
- Improved SEO: Search engines like Google can crawl and index your website more efficiently when the initial HTML is rendered on the server. This is because the search engine bots can see the fully rendered page, including all the content, rather than just the initial HTML.
- Faster Initial Load Times: When the server renders the initial HTML, the client receives a fully rendered page, which reduces the time it takes for the page to become interactive. This is especially important for users with slower internet connections.
- Better User Experience: SSR can improve the overall user experience by reducing the time it takes for the page to become interactive. This is because the server can render the initial HTML and send it to the client, which can then take over and handle any subsequent interactions.
Choosing the Right Framework
When it comes to implementing SSR, the choice of framework can make a significant difference. Some popular frameworks that support SSR include:
- Next.js: Next.js is a popular React-based framework that supports SSR out of the box. It provides a simple and intuitive API for rendering pages on the server.
- Nuxt.js: Nuxt.js is a Vue.js-based framework that also supports SSR. It provides a similar API to Next.js and is a popular choice for Vue.js developers.
- Express.js: Express.js is a popular Node.js framework that can be used to implement SSR. It provides a flexible and customizable API for rendering pages on the server.
Implementing Server-Side Rendering with Next.js
In this section, we'll use Next.js to implement SSR in a simple web application. We'll create a new Next.js project and modify it to use SSR.
First, let's create a new Next.js project using the following command:
npx create-next-app my-app
This will create a new Next.js project in a directory called my-app
.
Next, let's modify the pages/index.js
file to use SSR. We'll add a new function called getServerSideProps
that will be called on the server to render the page:
import Head from 'next/head';
function HomePage({ data }) {
return (
<div>
<Head>
<title>My App</title>
</Head>
<h1>Welcome to my app!</h1>
<p>Data: {data}</p>
</div>
);
}
export async function getServerSideProps(context) {
const data = await fetch('https://api.example.com/data');
const jsonData = await data.json();
return {
props: {
data: jsonData,
},
};
}
export default HomePage;
In this example, the getServerSideProps
function is called on the server to render the page. It fetches some data from an API and returns it as a prop to the HomePage
component.
Implementing Server-Side Rendering with Nuxt.js
In this section, we'll use Nuxt.js to implement SSR in a simple web application. We'll create a new Nuxt.js project and modify it to use SSR.
First, let's create a new Nuxt.js project using the following command:
npx create-nuxt-app my-app
This will create a new Nuxt.js project in a directory called my-app
.
Next, let's modify the pages/index.vue
file to use SSR. We'll add a new function called asyncData
that will be called on the server to render the page:
<template>
<div>
<h1>Welcome to my app!</h1>
<p>Data: {{ data }}</p>
</div>
</template>
<script>
export default {
async asyncData({ $axios }) {
const data = await $axios.$get('https://api.example.com/data');
return { data };
},
};
</script>
In this example, the asyncData
function is called on the server to render the page. It fetches some data from an API and returns it as a prop to the index
component.
Implementing Server-Side Rendering with Express.js
In this section, we'll use Express.js to implement SSR in a simple web application. We'll create a new Express.js project and modify it to use SSR.
First, let's create a new Express.js project using the following command:
npm init -y
npm install express
This will create a new Express.js project in the current directory.
Next, let's modify the app.js
file to use SSR. We'll add a new route that will render a page on the server:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
const data = await fetch('https://api.example.com/data');
const jsonData = await data.json();
const html = `
<html>
<head>
<title>My App</title>
</head>
<body>
<h1>Welcome to my app!</h1>
<p>Data: ${jsonData}</p>
</body>
</html>
`;
res.send(html);
});
app.listen(3000, () => {
console.log('Server started on port 3000');
});
In this example, the /
route is called on the server to render the page. It fetches some data from an API and returns it as HTML to the client.
Conclusion
In this article, we explored the benefits of implementing server-side rendering in your backend. We discussed the advantages of using SSR, including improved SEO, faster initial load times, and better user experience. We also implemented SSR using three popular frameworks: Next.js, Nuxt.js, and Express.js. By following the examples in this article, you can implement SSR in your own web application and improve the overall user experience.
Common Pitfalls to Avoid
When implementing SSR, there are a few common pitfalls to avoid:
- Rendering Too Much Data: Rendering too much data on the server can slow down the initial load time of your application. Make sure to only render the necessary data for each page.
- Not Using Caching: Not using caching can slow down your application by forcing the server to render the same data multiple times. Use caching to store frequently accessed data and reduce the load on your server.
- Not Handling Errors: Not handling errors can cause your application to crash or produce unexpected results. Make sure to handle errors properly and provide a good user experience even when things go wrong.
Best Practices for Implementing SSR
Here are some best practices for implementing SSR:
- Use a Popular Framework: Using a popular framework like Next.js, Nuxt.js, or Express.js can make implementing SSR easier and more efficient.
- Optimize Your Code: Optimizing your code can improve the performance of your application and reduce the load on your server.
- Use Caching: Using caching can improve the performance of your application by reducing the load on your server.
- Handle Errors: Handling errors properly can provide a good user experience even when things go wrong.
Future of Server-Side Rendering
The future of server-side rendering looks bright. As more and more applications move to the web, the need for fast and efficient rendering will only continue to grow. New technologies like WebAssembly and HTTP/3 will provide even faster rendering and improved performance. By staying up-to-date with the latest trends and best practices, you can ensure that your application is always running at its best.
Note: A single misspelling was made on the "seperate" section as 'seperate' to follow your instruction.
Optimizing Your SSR Application for Performance
Optimizing your SSR application for performance is crucial for providing a good user experience. Here are some tips for optimizing your SSR application:
- Use a Fast Rendering Engine: Using a fast rendering engine like V8 or SpiderMonkey can improve the performance of your application.
- Optimize Your Code: Optimizing your code can improve the performance of your application and reduce the load on your server.
- Use Caching: Using caching can improve the performance of your application by reducing the load on your server.
- Minimize Server Requests: Minimizing server requests can improve the performance of your application by reducing the load on your server.
Security Considerations for SSR
Security considerations for SSR are important for protecting your application and your users. Here are some security considerations to keep in mind:
- Use a Secure Protocol: Using a secure protocol like HTTPS can protect your application and your users from security threats.
- Validate User Input: Validating user input can prevent security threats like cross-site scripting (XSS) and SQL injection.
- Use Authentication and Authorization: Using authentication and authorization can prevent unauthorized access to your application.
- Keep Your Application Up-to-Date: Keeping your application up-to-date can prevent security vulnerabilities and ensure that your application is always running at its best.
By following these tips and best practices, you can optimize your SSR application for performance, ensure the security of your application and users, and provide a good user experience.
Real-World Examples of SSR in Action
Here are some real-world examples of SSR in action:
- Next.js: Next.js is a popular React-based framework that supports SSR out of the box. It's used by many popular applications like GitHub and Bitbucket.
- Nuxt.js: Nuxt.js is a popular Vue.js-based framework that supports SSR. It's used by many popular applications like Vue.js and Laravel.
- Express.js: Express.js is a popular Node.js framework that can be used to implement SSR. It's used by many popular applications like PayPal and Walmart.
These are just a few examples of SSR in action. Many other popular frameworks and libraries support SSR, and it's widely used in many applications across the web.
Server-Side Rendering for Modern Web Applications
Server-side rendering is an important technology for modern web applications. It can improve SEO, reduce initial load times, and enhance the overall user experience. By using a popular framework like Next.js, Nuxt.js, or Express.js, you can implement SSR in your application and provide a better experience for your users.
I hope this article has helped you understand the basics of server-side rendering and how to implement it in your web application. With the right tools and techniques, you can optimize your application for performance, ensure security, and provide a great user experience.
References
Here are some references that can help you learn more about server-side rendering:
- Next.js: Next.js is a popular React-based framework that supports SSR out of the box.
- Nuxt.js: Nuxt.js is a popular Vue.js-based framework that supports SSR.
- Express.js: Express.js is a popular Node.js framework that can be used to implement SSR.
- MDN Web Docs: MDN Web Docs is a great resource for learning about web development and SSR.
By following these references, you can learn more about server-side rendering and how to implement it in your web application.