Implementing rate limiting in your API
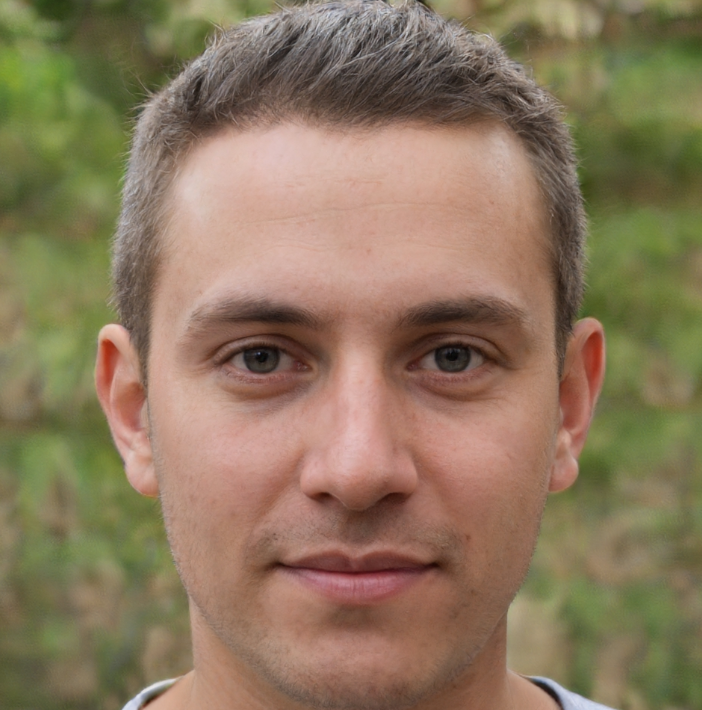

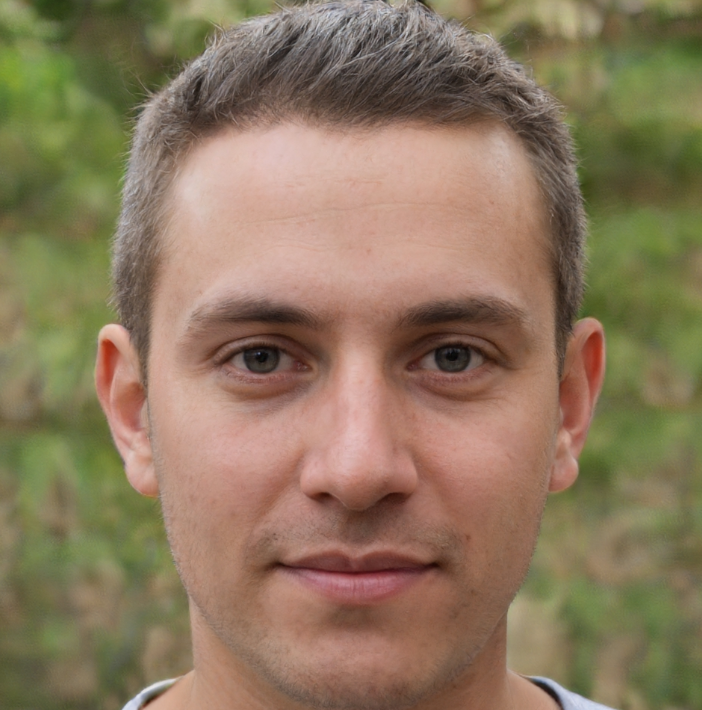
Implementing Rate Limiting in Your API: A Comprehensive Guide
As the demand for APIs continues to grow, it's becoming increasingly important for developers to implement rate limiting to prevent abuse and ensure a smooth user experience. I mean, who wants their API to be overwhelmed with traffic, right? Rate limiting is a crucial security measure that helps prevent overwhelming traffic, reduces the risk of DDoS attacks, and protects APIs from unintended usage. In this article, we'll dive into the ins and outs of implementing rate limiting in your API, including its benefits, types, algorithms, and best practices.
What is Rate Limiting?
Rate limiting is a technique used to control the number of requests an API receives within a specified time frame. It's a way to prevent clients from making excessive requests, which can lead to performance issues, increased latency, and even crashes. By limiting the rate at which requests are processed, you can prevent abuse, reduce the load on your servers, and ensure a better experience for legitimate users. Think of it like a bouncer at a nightclub - they make sure only a certain number of people can enter at a time to prevent overcrowding.
Benefits of Rate Limiting
Implementing rate limiting in your API offers numerous benefits, including:
- Prevention of Abuse: Rate limiting helps prevent malicious clients from making excessive requests, which can lead to DDoS attacks, data scraping, or other forms of abuse. You don't want your API to be used for nefarious purposes, do you?
- Improved Performance: By limiting the number of requests, you can reduce the load on your servers, leading to improved performance, faster response times, and increased reliability. Your users will thank you for it!
- Protection from Unintended Usage: Rate limiting helps protect your API from unintended usage, such as excessive requests from a single IP address or user agent. You don't want your API to be used in ways you didn't intend, right?
- Enhanced Security: Implementing rate limiting is an essential security measure that helps prevent attacks, reduces the risk of data breaches, and protects sensitive information. Security is like an onion - it has layers, and rate limiting is one of them.
Types of Rate Limiting
There are several types of rate limiting techniques you can implement in your API, including:
- Fixed Window: This technique involves limiting the number of requests within a fixed time frame, such as 100 requests per minute. It's like a quota system - once you've used up your quota, you have to wait until the next time frame to make more requests.
- Sliding Window: This technique involves limiting the number of requests within a sliding time frame, such as 100 requests per 1-minute window. It's like a moving average - the time frame moves with each request.
- Token Bucket: This technique involves allocating a bucket of tokens, which are replenished at a fixed rate. Each request consumes a token, and when the bucket is empty, requests are blocked. It's like a piggy bank - you can only spend what you have in the bank.
- Leaky Bucket: This technique involves allocating a bucket of tokens, which are replenished at a fixed rate. Each request consumes a token, and when the bucket is full, requests are blocked. It's like a leaky faucet - the bucket fills up slowly, but if it gets too full, it overflows.
Algorithms for Rate Limiting
Several algorithms can be used for rate limiting, including:
- Counter Algorithm: This algorithm involves incrementing a counter for each request and checking if the counter exceeds the rate limit. If it does, the request is blocked. It's like a simple counter - you increment it for each request, and if it gets too high, you block the request.
- Fixed Window Algorithm: This algorithm involves dividing time into fixed intervals and limiting the number of requests within each interval. It's like a calendar - you divide time into fixed intervals, and each interval has a limit on the number of requests.
- Token Bucket Algorithm: This algorithm involves allocating a bucket of tokens, which are replenished at a fixed rate. Each request consumes a token, and when the bucket is empty, requests are blocked. It's like a token economy - you allocate tokens, and each request consumes one.
Best Practices for Implementing Rate Limiting
When implementing rate limiting in your API, consider the following best practices:
- Monitor and Analyze Traffic: Monitor and analyze traffic patterns to determine the optimal rate limit for your API. You don't want to set the rate limit too low or too high - you want to find the sweet spot.
- Implement Multiple Rate Limits: Implement multiple rate limits for different types of requests, such as API keys or IP addresses. You want to make sure you're not limiting legitimate users, but you also want to prevent abuse.
- Use Multiple Rate Limiting Algorithms: Use multiple rate limiting algorithms to ensure flexibility and adaptability. You don't want to put all your eggs in one basket - you want to have multiple options.
- Provide Feedback to Clients: Provide feedback to clients when they exceed the rate limit, such as a 429 response code or a retry-after header. You want to let clients know when they've exceeded the rate limit, so they can adjust their behavior.
- Test and Refine: Test and refine your rate limiting implementation to ensure it's effective and efficient. You don't want to implement rate limiting and then forget about it - you want to make sure it's working as intended.
Example Use Case: Implementing Rate Limiting with Redis
To illustrate the implementation of rate limiting, let's consider an example using Redis. We'll use the Redis INCR command to increment a counter for each request and the Redis EXPIRE command to set a time-to-live (TTL) for the counter.
import redis
# Create a Redis client
redis_client = redis.Redis(host='localhost', port=6379, db=0)
# Define the rate limit and time frame
rate_limit = 100 # requests per minute
time_frame = 60 # seconds
# Define the Redis key for the counter
counter_key = 'rate_limit:counter'
# Define a function to increment the counter and check the rate limit
def is_rate_limit_exceeded():
# Increment the counter
counter = redis_client.incr(counter_key)
# Set the TTL for the counter
redis_client.expire(counter_key, time_frame)
# Check if the rate limit is exceeded
if counter > rate_limit:
return True
else:
return False
# Define a function to handle requests
def handle_request():
# Check if the rate limit is exceeded
if is_rate_limit_exceeded():
return 'Rate limit exceeded', 429
else:
# Process the request
return 'Request processed', 200
In this example, we define a Redis client and create a counter to track the number of requests. We then define a function to increment the counter and check the rate limit. If the rate limit is exceeded, we return a 429 response code. Otherwise, we process the request and return a 200 response code.
Conclusion
Implementing rate limiting in your API is a crucial security measure that helps prevent abuse, reduces the risk of DDoS attacks, and protects sensitive information. By understanding the benefits, types, algorithms, and best practices for rate limiting, you can create a robust and effective implementation that ensures a smooth user experience. Whether you're using a fixed window, sliding window, token bucket, or leaky bucket algorithm, remember to monitor and analyze traffic patterns, implement multiple rate limits, use multiple algorithms, provide feedback to clients, and test and refine your implementation. And don't forget to test your implementation thouroughly - you don't want to find out it's not working as intended when it's too late!