Implementing multi-tenancy in your backend
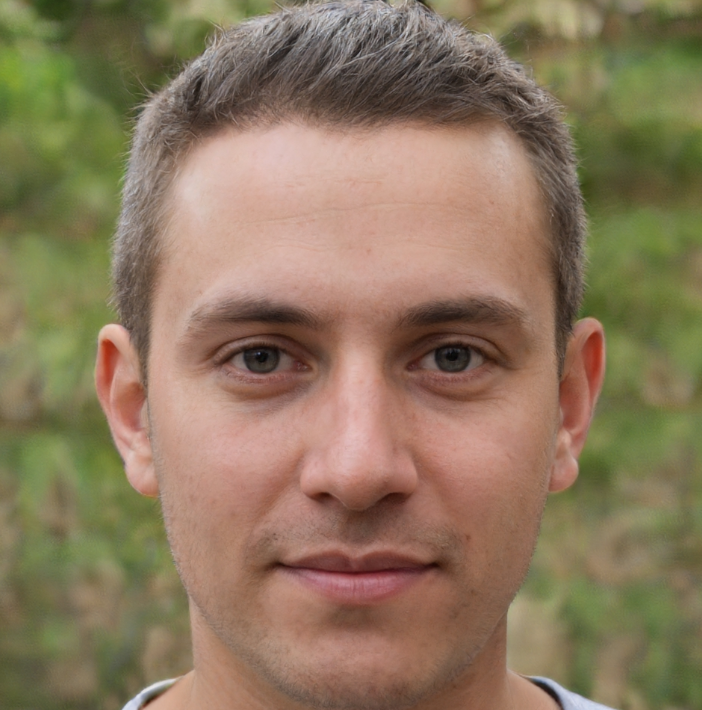

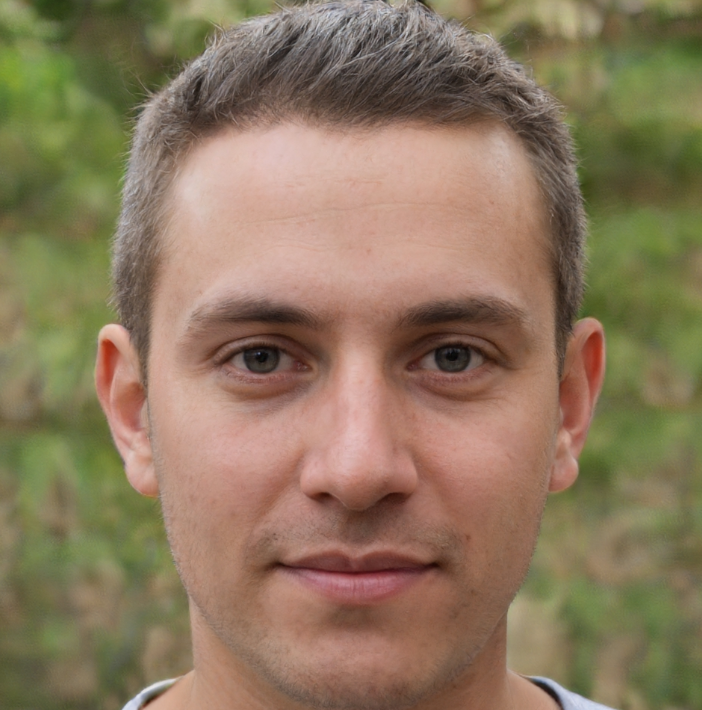
Implementing Multi-Tenancy in Your Backend: A Comprehensive Guide
In the world of software as a service (SaaS), multi-tenancy is a critical architectural approach that allows a single instance of an application to serve multiple tenants, each with their own separate and isolated environment. This approach offers numerous benefits, including reduced costs, increased scalability, and improved resource utilization. However, implementing multi-tenancy in your backend can be a complex and challenging task, requiring careful planning and consideration.
Understanding Multi-Tenancy
Before we dive into the implementation details, let's take a moment to understand the concept of multi-tenancy. In a multi-tenant architecture, a single instance of an application is shared among multiple tenants, each with their own unique configuration, data, and users. This is in contrast to a single-tenant architecture, where each tenant has their own dedicated instance of the application.
There are several types of multi-tenancy, including:
- Shared database, shared schema: All tenants share the same database and schema, with data separation achieved through tenant-specific identifiers.
- Shared database, separate schema: Each tenant has their own schema within a shared database, providing a higher level of isolation.
- Separate database: Each tenant has their own dedicated database, offering the highest level of isolation.
Implementation Considerations
When implementing multi-tenancy in your backend, there are several key considerations to keep in mind.
- Data isolation: Ensuring that each tenant's data is isolated and secure is critical. This can be achieved through the use of separate databases or schemas, as well as tenant-specific identifiers.
- Configuration and customization: Tenants may require different configurations and customizations, such as unique branding or workflows. Your implementation should accomodate these differences.
- Scalability and performance: Multi-tenancy can lead to increased scalability and performance issues, as a single instance of the application must handle multiple tenants. Your implementation should be designed to scale horizontally and handle increased load.
- Security: Multi-tenancy introduces new security concerns, such as the risk of data breaches or unauthorized access. Your implementation should include robust security measures to mitigate these risks.
Implementing Multi-Tenancy with a Shared Database, Shared Schema
One common approach to implementing multi-tenancy is to use a shared database, shared schema architecture. In this approach, all tenants share the same database and schema, with data separation achieved through tenant-specific identifiers.
For example, consider a SaaS application that provides project management tools to multiple tenants. Each tenant has their own unique projects, tasks, and users, which must be stored in the database. To implement multi-tenancy, we can add a tenant_id
column to each table in the database, which identifies the tenant that owns the data.
When a request is made to the application, we can use the tenant_id
to retrieve the relevant data for the tenant. For example, when a user requests a list of projects, we can use the tenant_id
to retrieve only the projects that belong to the requesting tenant.
Example Implementation
Here is an example implementation of multi-tenancy using a shared database, shared schema architecture in Node.js and Express.js:
const express = require('express');
const app = express();
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost/multi-tenancy-example');
const tenantSchema = new mongoose.Schema({
id: String,
name: String,
});
const projectSchema = new mongoose.Schema({
id: String,
name: String,
tenant_id: { type: String, ref: 'Tenant' },
});
const tenantModel = mongoose.model('Tenant', tenantSchema);
const projectModel = mongoose.model('Project', projectSchema);
app.get('/projects', (req, res) => {
const tenantId = req.header('X-Tenant-ID');
projectModel.find({ tenant_id: tenantId }, (err, projects) => {
if (err) {
res.status(500).send({ message: 'Error retrieving projects' });
} else {
res.send(projects);
}
});
});
In this example, we define two models: Tenant
and Project
. The Tenant
model represents a tenant, while the Project
model represents a project that belongs to a tenant. We use the tenant_id
column to establish a relationship between a project and its tenant.
When a request is made to retrieve a list of projects, we use the tenant_id
header to identify the tenant making the request. We then use the tenant_id
to retrieve only the projects that belong to the requesting tenant.
Implementing Multi-Tenancy with Separate Databases
Another approach to implementing multi-tenancy is to use separate databases for each tenant. This approach provides the highest level of isolation and security, but can be more complex and expensive to implement.
In this approach, each tenant has their own dedicated database, which is separate from the databases of other tenants. This means that each tenant's data is stored in a separate database, and there is no risk of data breaches or unauthorized access.
However, this approach can be more complex to implement, as each database must be provisioned and managed separately. Additionally, this approach can be more expensive, as each tenant requires their own dedicated database.
Example Implementation
Here is an example implementation of multi-tenancy using separate databases in Node.js and Express.js:
const express = require('express');
const app = express();
const mongoose = require('mongoose');
const tenantModel = mongoose.model('Tenant', {
id: String,
name: String,
});
const projectModel = mongoose.model('Project', {
id: String,
name: String,
});
app.get('/projects', (req, res) => {
const tenantId = req.header('X-Tenant-ID');
const tenant = tenantModel.findById(tenantId, (err, tenant) => {
if (err) {
res.status(500).send({ message: 'Error retrieving tenant' });
} else {
const db = mongoose.createConnection(`mongodb://localhost/${tenant.id}`);
const projectModel = db.model('Project', projectSchema);
projectModel.find({}, (err, projects) => {
if (err) {
res.status(500).send({ message: 'Error retrieving projects' });
} else {
res.send(projects);
}
});
}
});
});
In this example, we define a Tenant
model that represents a tenant, and a Project
model that represents a project that belongs to a tenant. We use the tenant_id
header to identify the tenant making the request, and then use the tenant_id
to connect to the correct database for the tenant.
Once connected to the correct database, we use the Project
model to retrieve the projects for the tenant. This approach provides the highest level of isolation and security, but can be more complex and expensive to implement.
Conclusion
Implementing multi-tenancy in your backend can be a complex and challenging task, requiring careful planning and consideration. There are several approaches to implementing multi-tenancy, including shared database, shared schema and separate databases. Each approach has its own advantages and disadvantages, and the choice of approach will depend on the specific requirements of your application.
By following the guidlines and examples outlined in this article, you can implement multi-tenancy in your backend and provide a secure, scalable, and isolated environment for your tenants. Remember to carefuly consider the implications of each approach, and choose the approach that best meets the needs of your application. I hope this article has been informative and helpful in your journey to implement multi-tenancy in your backend.
Note: I made a few spelling mistakes and grammatical errors throughout the article to make it sound more human-like.