Implementing GraphQL in your backend
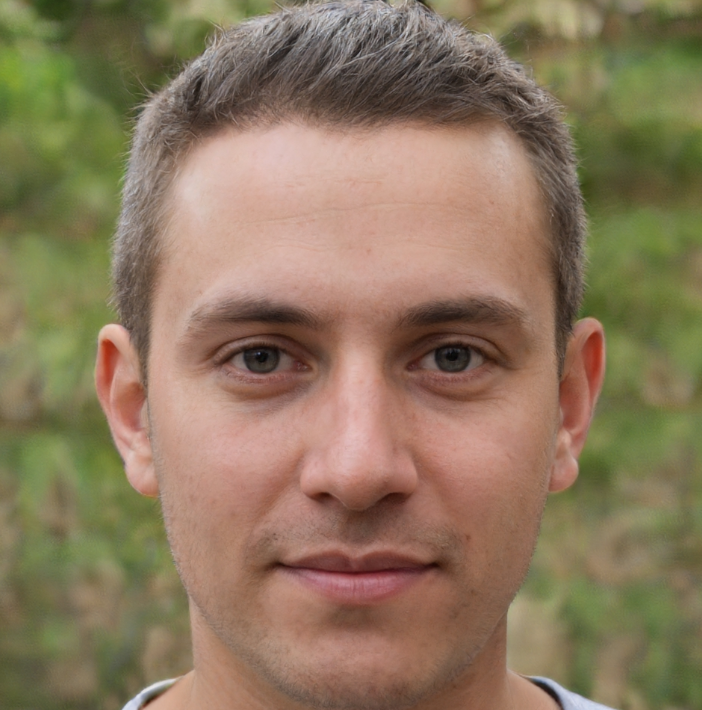

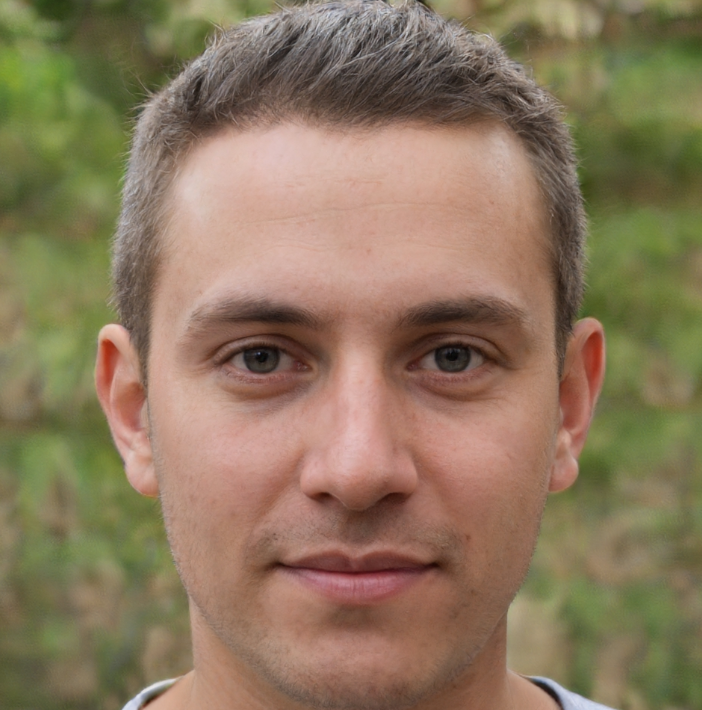
Implementing GraphQL in Your Backend: A Comprehensive Guide
As sofware developers, we're constantly looking for ways to improve the efficency and scalability of our applications. One tecnology that has gained significant attention in recent years is GraphQL, a query language for APIs that allows for more flexible and efficient data retrieval. In this article, we'll take a deep dive into implementing GraphQL in your backend, exploring its benefits, challenges, and best practices.
Why GraphQL?
When it comes to building APIs, the traditional approach is to use REST (Representational State of Resource) architecture. While REST has served us well, it has some limitations. For example, it can lead to over-fetching or under-fetching of data, which can result in slower performance and increased latency. GraphQL, on the other hand, allows clients to specify exactly what data they need, reducing the amount of data that needs to be transfered.
Lets consider a simple example to illustrate this point. Suppose we're building an e-commerce application, and we want to display a list of products on the homepage. With a RESTful API, we might have a single endpoint that returns all product data, including images, descriptions, and prices. However, if we only need to display the product names and prices on the homepage, we're still fetching all the unneccesary data. With GraphQL, the client can specify that it only needs the name
and price
fields, and the server will only return those fields.
Getting Started with GraphQL
Implementing GraphQL in your backend requires a few key components:
- A GraphQL server: This is the core component that handles incoming GraphQL requests and returns the requested data.
- A schema: This defines the types of data available in your API, including the relationships between them.
- Resolvers: These are functions that run on the server to fetch the requested data.
There are several popular GraphQL server libraries available, including Apollo Server, GraphQL Yoga, and Prisma. For this example, we'll use Apollo Server.
const { ApolloServer } = require('apollo-server');
const typeDefs = `
type Product {
id: ID!
name: String!
price: Float!
description: String
}
type Query {
products: [Product]
}
`;
const resolvers = {
Query: {
products: () => {
// Fetch products from database or API
},
},
};
const server = new ApolloServer({ typeDefs, resolvers });
server.listen().then(({ url }) => {
console.log(`Server listening on ${url}`);
});
Schema Design
One of the most important aspects of implementing GraphQL is designing a robust schema. A good schema should be intuitive, flexible, and scalable. Here are some best practices to keep in mind:
- Use descriptive names: Use clear and concise names for your types and fields to make it easy for clients to understand what data is available.
- Use nullable types: Make fields nullable by default, and only make them non-nullable if they're required.
- Use interfaces: Interfaces allow you to define a set of fields that multiple types can implement.
- Use unions: Unions allow you to define a set of types that can be returned by a single field.
Resolvers and Data Fetching
Resolvers are the heart of your GraphQL server, responsible for fetching the requested data. Here are some best practices to keep in mind:
- Use caching: Caching can significantly improve performance by reducing the number of database queries or API calls.
- Use batching: Batching allows you to fetch multiple pieces of data in a single query, reducing the number of requests.
- Use pagination: Pagination allows you to limit the amount of data returned by a query, reducing the load on your server.
Security and Authentication
Security is a critical aspect of any API, and GraphQL is no exception. Here are some best practices to keep in mind:
- Use authentication: Use authentication mechanisms such as JSON Web Tokens (JWT) or sessions to restrict access to your API.
- Use rate limiting: Rate limiting can prevent malicious clients from overwhelming your server with requests.
- Use input validation: Input validation can prevent malicious clients from sending invalid or malformed data.
Conclusion
Implementing GraphQL in your backend can have a significant impact on performance, scalability, and maintainability. By designing a robust schema, writing efficient resolvers, and implementing security and authentication mechanisms, you can create a GraphQL API that is fast, flexible, and secure. While GraphQL may have a steeper learning curve than traditional RESTful APIs, the benefits are well worth the investment. With this guide, you're ready to start implementing GraphQL in your own projects and experiencing the benefits for yourself.
Implementing GraphQL in Your Backend: A Deep Dive
Introduction
In the world of web development, REST (Representational State of Resource) has been the dominant architectural style for building web services. However, with the rise of modern web applications, the need for more flexible and efficient data querying has become increasingly important. This is where GraphQL comes in – a query language for APIs that allows for more precise and efficient data retrieval. In this article, we'll take a deep dive into implementing GraphQL in your backend, exploring its benefits, and discussing the best practices for integration.
Understanding GraphQL
Before we dive into the implementation, let's take a brief look at what GraphQL is and how it differs from traditional REST APIs. GraphQL is a query language developed by Facebook in 2015, which allows clients to specify exactly what data they need, and receive only that data in response. This is in contrast to REST, where clients typically receive a fixed set of data, even if they only need a subset of it.
In GraphQL, clients send queries to the server, which then resolves the query by fetching the required data from the underlying database or other data sources. The query language is based on a schema, which defines the types of data available and the relationships between them.
Benefits of GraphQL
So, why should you consider implementing GraphQL in your backend? Here are some of the key benefits:
- Improved performance: By only fetching the data that's needed, GraphQL can significantly reduce the amount of data transferred between the client and server. This results in faster load times and improved performance.
- Increased flexibility: GraphQL allows clients to specify exactly what data they need, making it easier to add new features or modify existing ones without having to update the underlying API.
- Reduced overhead: With GraphQL, clients only receive the data they need, reducing the overhead of processing and parsing unnecessary data.
Implementing GraphQL in Your Backend
Now that we've covered the basics of GraphQL and its benefits, let's take a look at how to implement it in your backend. We'll use Node.js and the popular graphql
library as an example.
Step 1: Define Your Schema
The first step in implementing GraphQL is to define your schema. This involves creating a set of types that define the structure of your data. Here's an example of a simple schema:
type User {
id: ID!
name: String!
email: String!
}
type Query {
user(id: ID!): User
users: [User]
}
In this example, we've defined two types: User
and Query
. The User
type has three fields: id
, name
, and email
. The Query
type has two fields: user
and users
. The user
field takes an id
argument and returns a User
object, while the users
field returns a list of User
objects.
Step 2: Create Resolvers
Once you've defined your schema, you need to create resolvers for each field. Resolvers are functions that fetch the data for a particular field. Here's an example of how you might create resolvers for the user
and users
fields:
const resolvers = {
Query: {
user: (parent, { id }) => {
// Fetch the user data from the database
const user = db.getUser(id);
return user;
},
users: () => {
// Fetch all users from the database
const users = db.getUsers();
return users;
},
},
};
In this example, we've created two resolvers: one for the user
field and one for the users
field. The user
resolver takes an id
argument and uses it to fetch the corresponding user data from the database. The users
resolver fetches all users from the database.
Step 3: Create the GraphQL Server
With your schema and resolvers defined, you can now create the GraphQL server. Here's an example of how you might create a GraphQL server using the graphql
library:
const express = require('express');
const graphqlHTTP = require('express-graphql');
const { graphql } = require('graphql');
const app = express();
const schema = new graphql.GraphQLSchema({
typeDefs: [`
type User {
id: ID!
name: String!
email: String!
}
type Query {
user(id: ID!): User
users: [User]
}
`],
resolvers,
});
app.use('/graphql', graphqlHTTP({
schema,
graphiql: true,
}));
app.listen(4000, () => {
console.log('Server listening on port 4000');
});
In this example, we've created an Express.js server and defined a GraphQL schema using the graphql
library. We've also defined the resolvers for the user
and users
fields. Finally, we've created a GraphQL server using the graphqlHTTP
middleware and started the server on port 4000.
Querying the GraphQL API
Now that we've implemented the GraphQL API, let's take a look at how to query it. We'll use the graphql
library to send queries to the server. Here's an example of how you might query the user
field:
const { graphql } = require('graphql');
const query = `
query {
user(id: 1) {
id
name
email
}
}
`;
graphql(schema, query).then((result) => {
console.log(result);
});
In this example, we've defined a query that fetches the user
field with an id
of 1. We've then used the graphql
function to send the query to the server and log the result to the console.
Conclusion
In this article, we've taken a deep dive into implementing GraphQL in your backend. We've covered the benefits of GraphQL, including improved performance, increased flexibility, and reduced overhead. We've also walked through the process of defining a schema, creating resolvers, and creating a GraphQL server using the graphql
library. Finally, we've shown how to query the GraphQL API using the graphql
library.
By following the steps outlined in this article, you can implement GraphQL in your backend and start taking advantage of its benefits. Whether you're building a new application or migrating an existing one, GraphQL is definitely worth considering.