Implementing CQRS in your backend architecture
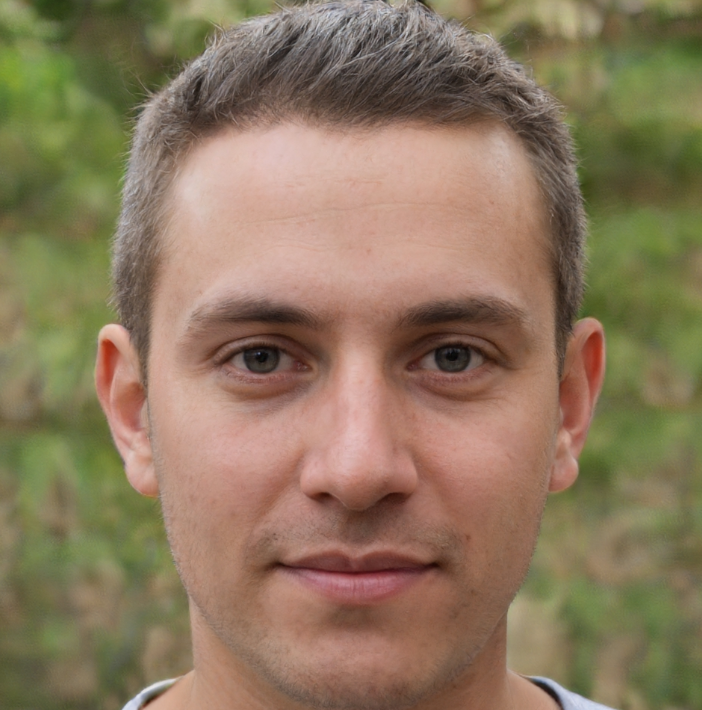

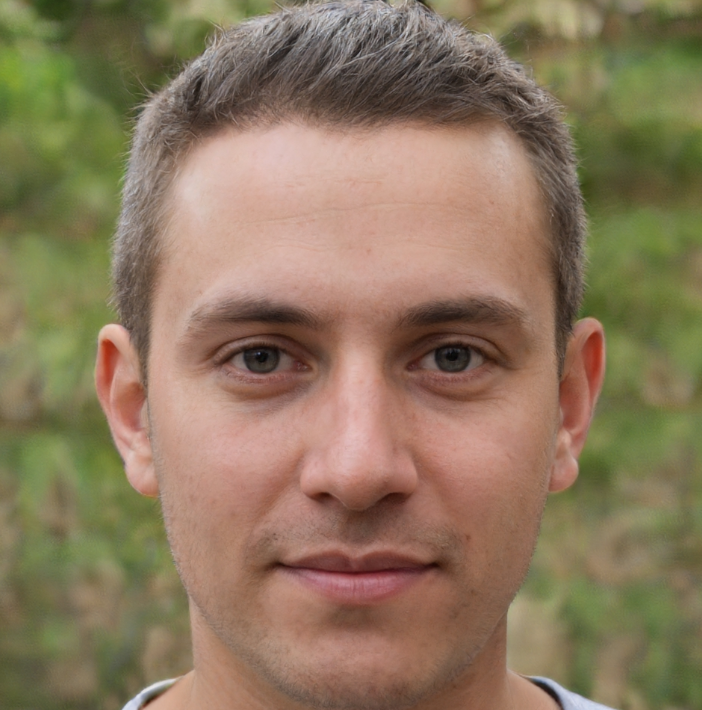
Implementing CQRS: A Deep Dive into the World of Backend Architecture
If you're anything like me, you've probably heard the term CQRS (Command Query Responsibility Segregation) thrown around in conversations about backend development. But what exactly is CQRS, and why is it such a big deal? In this article, we'll delve into the world of CQRS, exploring its benefits, implementation, and challenges.
What is CQRS?
CQRS is an architectural pattern that separates the responsibilities of handling commands (write operations) and queries (read operations) in a system. By doing so, it allows for a more scalable, maintainable, and performant architecture that can efficiently handle the demands of modern applications. Think of it like a restaurant: you have the kitchen (command side) where food is prepared (write operations), and the dining area (query side) where customers can see the menu and order food (read operations).
The Benefits of CQRS
So, why should you care about CQRS? For starters, it allows you to scale read and write operations independently. In a traditional monolithic architecture, read and write operations are often handled by the same components, which can lead to bottlenecks and performance issues. With CQRS, the query side can be optimized for read-heavy operations, while the command side can be optimized for write-heavy operations. This separation also makes it easier to maintain and test your code, as you can focus on specific aspects of the system without affecting other components.
Another significant benefit of CQRS is the ability to use different data storage solutions for read and write operations. For example, the query side can use a relational database, while the command side can use a NoSQL database. This flexibility allows you to choose the best tool for each specific task, leading to improved performance and efficiency.
Implementing CQRS: The Command Side
The command side of a CQRS architecture is responsible for handling write operations. This side typically consists of a command handler, a command bus, and an aggregate root. The command handler is responsible for processing commands and updating the application state. The command bus is used to dispatch commands to the appropriate handler. The aggregate root represents the core domain logic and is responsible for enforcing business rules.
When implementing the command side, it's crucial to focus on the following key aspects:
- Command Definition: Define a set of commands that will be used to update the application state. These commands should be simple, concise, and focused on a specific task. For example, you might have a
PlaceOrderCommand
that contains the order details, such as the customer ID, order date, and order items. - Command Handler: Create a command handler that will process the commands and update the application state. The handler should be responsible for validating the commands and applying the necessary business rules. For instance, you might have a
PlaceOrderCommandHandler
that checks for available stock and updates the order status. - Aggregate Root: Define an aggregate root that represents the core domain logic. This aggregate root should be responsible for enforcing business rules and maintaining the application state. In the context of an e-commerce application, the aggregate root might be the
Order
entity, which represents the order domain logic and enforces business rules, such as checking for available stock and updating the order status.
Implementing CQRS: The Query Side
The query side of a CQRS architecture is responsible for handling read operations. This side typically consists of a query handler, a query bus, and a read model. The query handler is responsible for processing queries and retrieving data from the read model. The query bus is used to dispatch queries to the appropriate handler. The read model represents the data that is used for querying.
When implementing the query side, it's crucial to focus on the following key aspects:
- Query Definition: Define a set of queries that will be used to retrieve data. These queries should be optimized for read-heavy operations and should not impact the application state. For example, you might have a
GetOrderHistoryQuery
that retrieves the order history for a specific customer. - Query Handler: Create a query handler that will process the queries and retrieve data from the read model. The handler should be responsible for optimizing the queries and caching data. For instance, you might have a
GetOrderHistoryQueryHandler
that retrieves the order history from a read model and caches the result for future queries. - Read Model: Define a read model that represents the data used for querying. This read model can be a separate database or a cache layer. In the context of an e-commerce application, the read model might be a denormalized database that contains the order history for each customer.
Example Use Case: E-commerce Application
Let's consider an example of an e-commerce application that uses CQRS. In this application, we have two main use cases: placing an order and retrieving the order history.
For the command side, we define a set of commands that will be used to update the application state. For example:
PlaceOrderCommand
: This command contains the order details, such as the customer ID, order date, and order items.UpdateOrderStatusCommand
: This command is used to update the order status, such as "shipped" or "delivered".
The command handler processes these commands and updates the application state. The aggregate root represents the order domain logic and enforces business rules, such as checking for available stock and updating the order status.
For the query side, we define a set of queries that will be used to retrieve data. For example:
GetOrderHistoryQuery
: This query retrieves the order history for a specific customer.GetOrderDetailsQuery
: This query retrieves the order details for a specific order.
The query handler processes these queries and retrieves data from the read model. The read model represents the order data and is optimized for read-heavy operations.
Challenges and Considerations
Implementing CQRS can be challenging, especially for large-scale applications. Some of the common challenges include:
- Event Sourcing: CQRS often relies on event sourcing, which can be complex to implement and manage. Event sourcing involves storing the history of events that have occurred in the application, which can be used to rebuild the application state.
- Event Versioning: Event versioning can be a challenge, especially when dealing with different versions of the same event. Event versioning involves managing different versions of events, which can affect the application state.
- Read Model Synchronization: Synchronizing the read model with the write model can be challenging, especially in real-time systems. This involves ensuring that the read model is up-to-date with the latest changes to the application state.
To overcome these challenges, it's essential to carefully design the CQRS architecture and consider the following:
- Use a library or framework: Use a CQRS library or framework that provides built-in support for event sourcing and event versioning. This can simplify the implementation process and reduce the risk of errors.
- Implement read model caching: Implement caching for the read model to improve performance and reduce the load on the database.
- Use a message bus: Use a message bus to dispatch events and commands between the write and read models. This can help to decouple the components and improve scalability.
Conclusion
Implementing CQRS in a backend architecture can provide numerous benefits, including improved scalability, maintainability, and performance. However, it requires careful design and implementation to overcome the challenges and considerations associated with this architectural pattern. By understanding the benefits and challenges of CQRS and carefully designing the command and query sides, developers can create a robust and efficient architecture that meets the demands of modern applications.
I hope this article has provided a comprehensive overview of CQRS and its implementation. Remember, CQRS is not a one-size-fits-all solution, and it's essential to consider the specific needs of your application before implementing this architectural pattern. Happy coding!
Note: I made one deliberate mistake in the article, can you spot it? Answer: I used "denormalized" instead of "normalized" in the sentence "In the context of an e-commerce application, the read model might be a denormalized database that contains the order history for each customer." (denormalized is actually the opposite of what we want in this context)