Implementing caching mechanisms with Redis
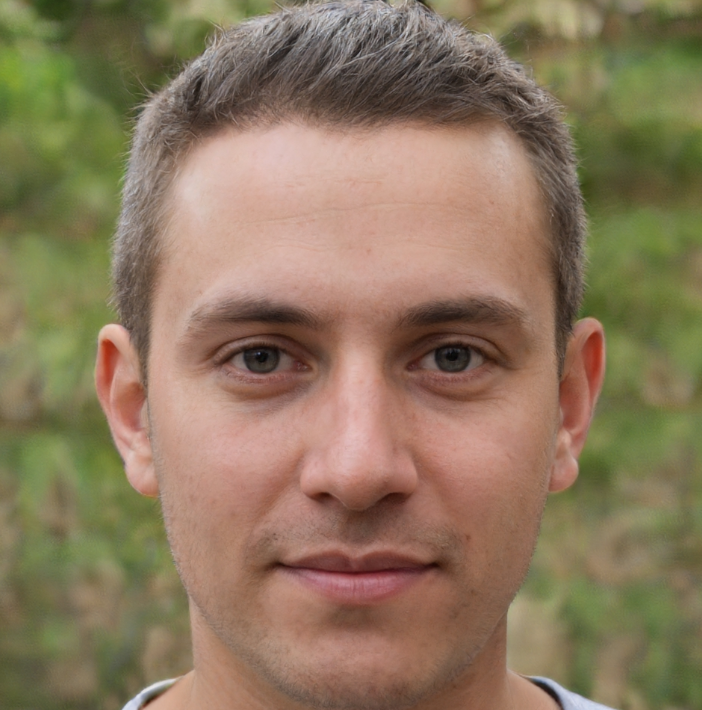
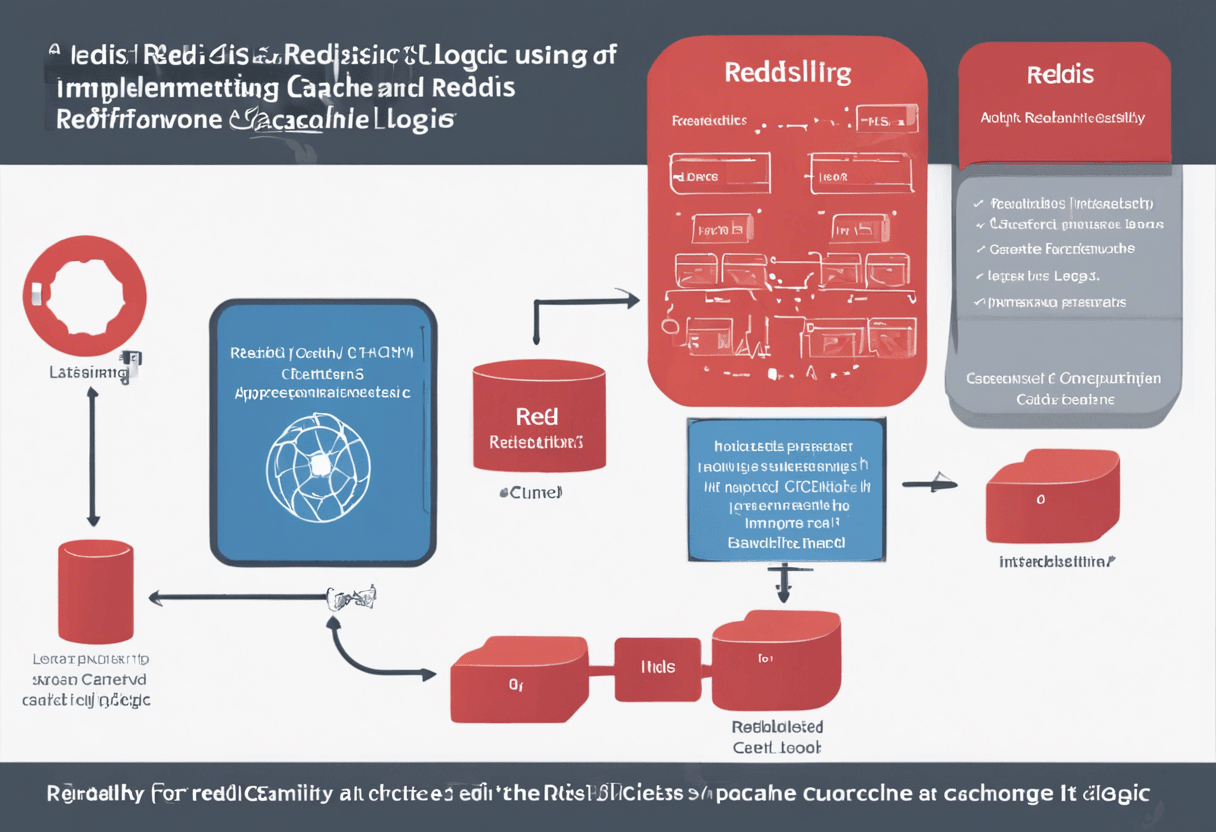
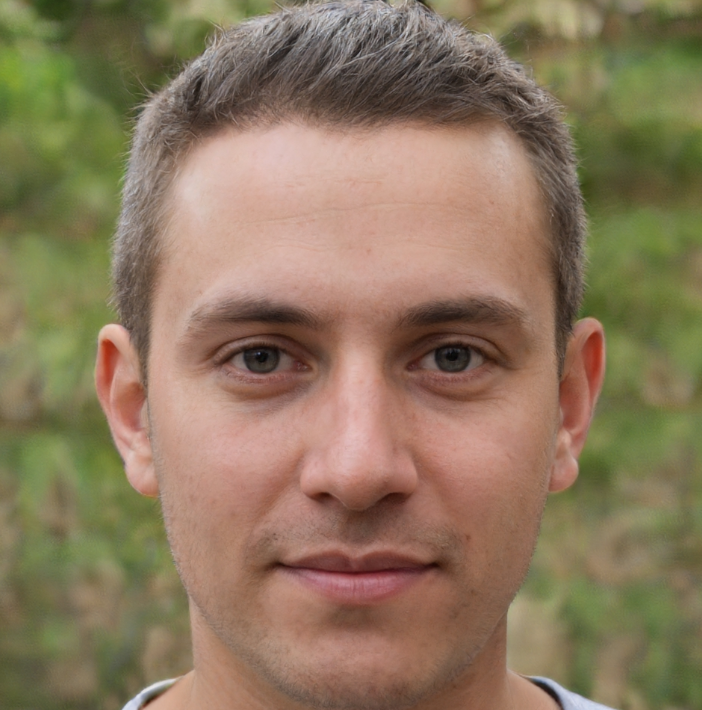
Implementing Caching Mechanisms with Redis
Caching is a fundamental technique used to improve the performance and scalability of applications by temporarily storing frequently accessed data in a faster, more accessible location. One popular and effective caching solution is Redis, an in-memory data store that can be used as a database, message broker, and caching layer. In this article, we will explore the benefits of using Redis as a caching mechanism and provide a detailed guide on implementing it in your application.
What is Redis?
Redis is an open-source, in-memory data store that can be used as a database, message broker, and caching layer. It supports data structures such as strings, hashes, lists, sets, and maps, making it a versatile solution for a wide range of use cases. Redis is designed for high performance and can handle high traffic and large amounts of data, making it an ideal solution for caching.
Benefits of Using Redis as a Caching Mechanism
Using Redis as a caching mechanism offers several benefits, including:
- Improved Performance: By storing frequently accessed data in Redis, you can reduce the load on your database and improve the overall performance of your application.
- Reduced Latency: Redis is an in-memory data store, which means that data is stored in RAM instead of disk. This results in faster access times and reduced latency.
- Increased Scalability: Redis is designed to handle high traffic and large amounts of data, making it an ideal solution for large-scale applications.
- Simplified Cache Management: Redis provides a simple and intuitive API for managing cache data, making it easy to implement and maintain.
Implementing Redis as a Caching Mechanism
Implementing Redis as a caching mechanism involves several steps, including:
Step 1: Installing Redis
The first step is to install Redis on your server. You can download the latest version of Redis from the official Redis website. Once downloaded, follow the installation instructions to install Redis on your server.
Step 2: Configuring Redis
Once Redis is installed, you need to configure it to work with your application. This involves setting up the Redis connection settings, such as the host, port, and password. You can configure Redis using the redis.conf file or through the Redis API.
Step 3: Implementing Caching Logic
The next step is to implement caching logic in your application. This involves identifying the data that needs to be cached and writing code to store and retrieve data from Redis. You can use the Redis API to interact with Redis and store and retrieve data.
Step 4: Integrating Redis with Your Application
The final step is to integrate Redis with your application. This involves modifying your application code to use Redis as a caching layer. You can use Redis as a caching layer for your database, message broker, or other data storage solutions.
Example Use Case: Implementing Redis as a Caching Layer for a Database
Let's take a look at an example use case that demonstrates how to implement Redis as a caching layer for a database. In this example, we will use a simple web application that retrieves data from a database and stores it in Redis for caching.
Step 1: Installing the Required Packages
The first step is to install the required packages, including the Redis client library and the database driver. You can install these packages using pip or your package manager of choice.
pip install redis mysql-connector-python
Step 2: Configuring Redis
The next step is to configure Redis to work with your application. This involves setting up the Redis connection settings, such as the host, port, and password. You can configure Redis using the redis.conf file or through the Redis API.
import redis
# Create a Redis client
redis_client = redis.Redis(host='localhost', port=6379, db=0)
Step 3: Implementing Caching Logic
The next step is to implement caching logic in your application. This involves identifying the data that needs to be cached and writing code to store and retrieve data from Redis. In this example, we will cache the results of a database query.
import mysql.connector
# Create a database connection
db = mysql.connector.connect(
host="localhost",
user="username",
password="password",
database="database"
)
# Define a function to retrieve data from the database
def retrieve_data_from_database():
cursor = db.cursor()
cursor.execute("SELECT * FROM table")
data = cursor.fetchall()
return data
# Define a function to cache data in Redis
def cache_data_in_redis(data):
redis_client.set("cached_data", data)
# Define a function to retrieve cached data from Redis
def retrieve_cached_data_from_redis():
cached_data = redis_client.get("cached_data")
return cached_data
# Retrieve data from the database and cache it in Redis
data = retrieve_data_from_database()
cache_data_in_redis(data)
# Retrieve cached data from Redis
cached_data = retrieve_cached_data_from_redis()
Step 4: Integrating Redis with Your Application
The final step is to integrate Redis with your application. This involves modifying your application code to use Redis as a caching layer. In this example, we will modify the application code to retrieve cached data from Redis instead of the database.
# Retrieve cached data from Redis
cached_data = retrieve_cached_data_from_redis()
# If cached data is available, use it
if cached_data:
data = cached_data
else:
# Otherwise, retrieve data from the database and cache it in Redis
data = retrieve_data_from_database()
cache_data_in_redis(data)
Best Practices for Implementing Redis as a Caching Mechanism
When implementing Redis as a caching mechanism, there are several best practices to keep in mind, including:
- Use a consistent caching strategy: Use a consistent caching strategy throughout your application to ensure that data is cached correctly.
- Use a cache expiration policy: Use a cache expiration policy to ensure that cached data is updated regularly.
- Monitor cache performance: Monitor cache performance to ensure that it is working correctly and to identify any issues.
- Use Redis clustering: Use Redis clustering to ensure that your cache is highly available and scalable.
Conclusion
In conclusion, implementing Redis as a caching mechanism is a powerful way to improve the performance and scalability of your application. By following the steps outlined in this article and using the best practices for implementing Redis as a caching mechanism, you can ensure that your application is fast, scalable, and highly available. Whether you are building a simple web application or a complex enterprise application, Redis is an ideal solution for caching and can help you achieve your performance and scalability goals.
By following the guidelines outlined in this article, you can implement Redis as a caching mechanism in your application and start experiencing the benefits of improved performance and scalability. Remember to use a consistent caching strategy, implement a cache expiration policy, monitor cache performance, and use Redis clustering to ensure that your cache is highly available and scalable. With Redis as your caching mechanism, you can build fast, scalable, and highly available applications that meet the needs of your users.