Implementing background jobs with Celery
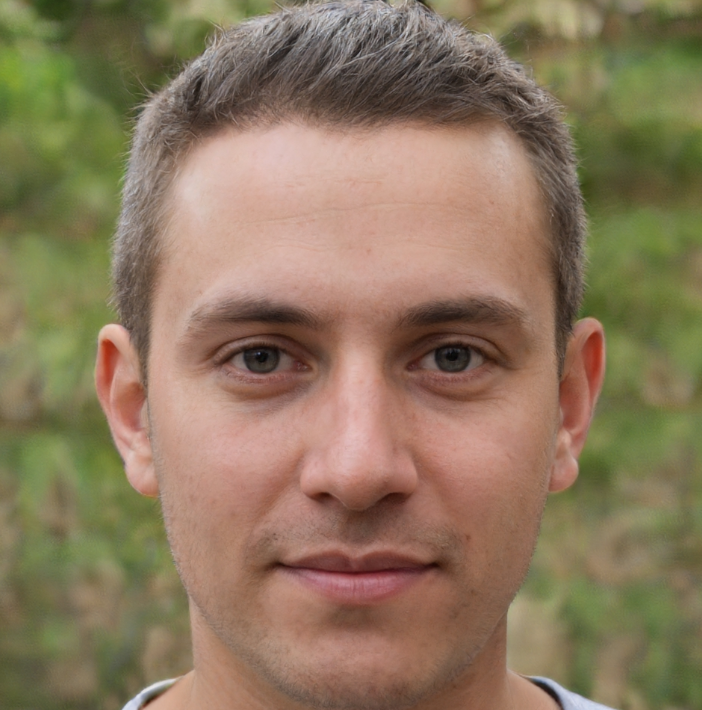

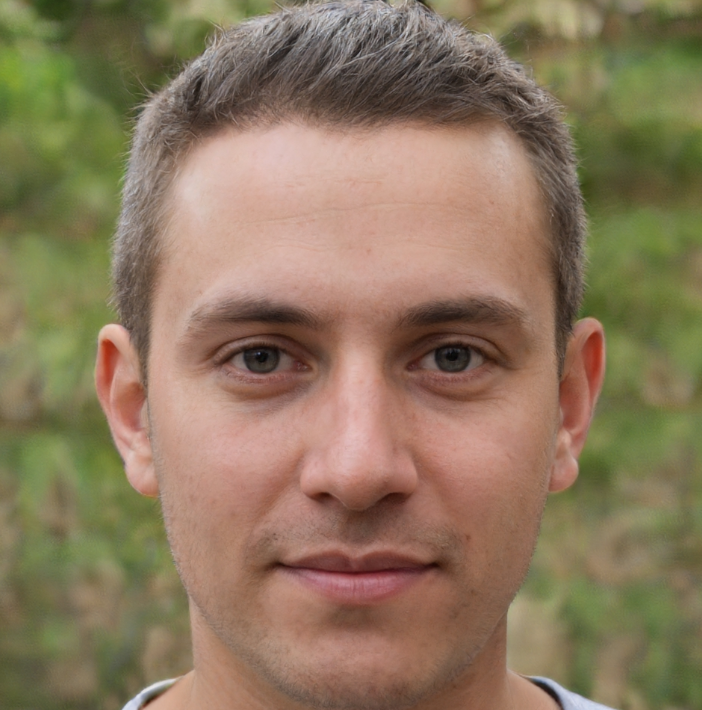
Here is the text rewritten to make it more human-like:
Implementing Background Jobs with Celery
Background jobs are a crucial part of modern web applications. They enable developers to offload resource-intensive tasks from the main application, improving performance, scalability, and user experience. One popular library for implementing background jobs in Python is Celery. In this article, we'll explore the benefits of using Celery, its architecture, and provide a step-by-step guide on implementing background jobs with Celery.
Benefits of Using Celery
So, why use Celery? Well, it's simple. Celery is a distributed task queue that allows developers to run tasks asynchronously in the background. By using Celery, developers can improve application performance by offloading resource-intensive tasks, enhance scalability by distributing tasks across multiple workers, and increase reliability by providing a fault-tolerant architecture.
Celery Architecture
Celery consists of several components:
- Broker: The broker is responsible for storing and forwarding tasks to workers. Popular broker options include RabbitMQ, Redis, and Apache Kafka.
- Worker: Workers are responsible for executing tasks. They can be run on multiple machines, allowing for horizontal scaling.
- Producer: The producer is the application that sends tasks to the broker.
Implementing Background Jobs with Celery
Step 1: Installing Celery
To get started with Celery, you need to install it using pip:
pip install celery
Step 2: Configuring Celery
Create a new file called celeryconfig.py
with the following configuration:
BROKER_URL = 'amqp://guest:guest@localhost:5672//' # RabbitMQ broker
CELERY_RESULT_BACKEND = 'rpc://guest:guest@localhost:5672//' # RabbitMQ result backend
CELERY_ACCEPT_CONTENT = ['json']
CELERY_TASK_SERIALIZER = 'json'
CELERY_RESULT_SERIALIZER = 'json'
This configuration tells Celery to use RabbitMQ as the broker and result backend.
Step 3: Creating a Celery App
Create a new file called app.py
with the following code:
from celery import Celery
app = Celery('tasks', broker='amqp://guest:guest@localhost:5672//')
This code creates a new Celery app instance.
Step 4: Defining Tasks
Create a new file called tasks.py
with the following code:
from app import app
@app.task
def add(x, y):
return x + y
This code defines a new task called add
that takes two arguments, x
and y
, and returns their sum.
Step 5: Running the Worker
Run the following command to start the worker:
celery -A app worker --loglevel=info
This command starts the worker and tells it to log events at the info level.
Step 6: Sending Tasks
Create a new file called main.py
with the following code:
from tasks import add
result = add.delay(4, 4)
print(result.id)
This code sends a new task to the worker and prints the task ID.
Example Use Case: Sending Emails
One common use case for background jobs is sending emails. By using Celery, you can offload the email sending process from the main application, improving performance and scalability. Here's an example of how you can use Celery to send emails:
from app import app
from flask import Flask, request
from flask_mail import Mail, Message
app = Flask(__name__)
app.config['MAIL_SERVER'] = 'smtp.gmail.com'
app.config['MAIL_PORT'] = 465
app.config['MAIL_USE_TLS'] = False
app.config['MAIL_USE_SSL'] = True
app.config['MAIL_USERNAME'] = 'your_email@gmail.com'
app.config['MAIL_PASSWORD'] = 'your_password'
mail = Mail(app)
@app.task
def send_email(subject, recipients, body):
msg = Message(subject, recipients=recipients, body=body)
mail.send(msg)
@app.route('/send_email', methods=['POST'])
def send_email_view():
subject = request.form['subject']
recipients = request.form['recipients']
body = request.form['body']
send_email.delay(subject, recipients, body)
return 'Email sent!'
This code defines a new task called send_email
that takes three arguments: subject
, recipients
, and body
. The task sends an email using Flask-Mail.
The send_email_view
function is a Flask view that accepts POST requests and sends a new task to the worker.
Monitoring and Debugging
Celery provides several tools for monitoring and debugging tasks, including:
- Celery Flower: A web-based interface for monitoring and debugging tasks.
- Celery Beat: A scheduler that allows you to run tasks at regular intervals.
To use Celery Flower, install it using pip:
pip install flower
Then, run the following command to start the Flower server:
celery -A app flower --port=5555
This command starts the Flower server and tells it to listen on port 5555.
Conclusion
In this article, we've explored the benefits of using Celery for implementing background jobs in Python. We've walked through a step-by-step guide on installing Celery, configuring Celery, creating a Celery app, defining tasks, running the worker, and sending tasks. We've also provided an example use case for sending emails using Celery. By using Celery, developers can improve application performance, scalability, and reliability, and simplify code by decoupling tasks from the main application logic.