Implementing a search engine in your backend
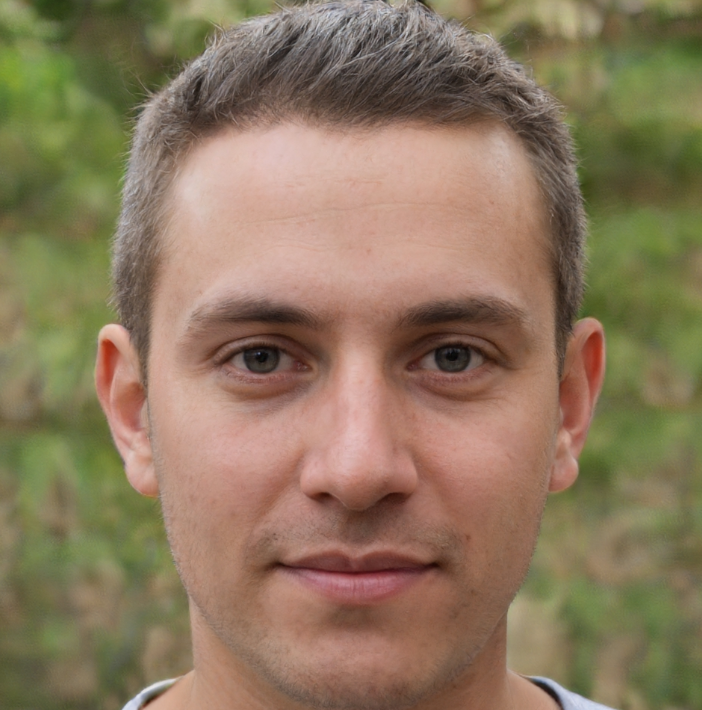

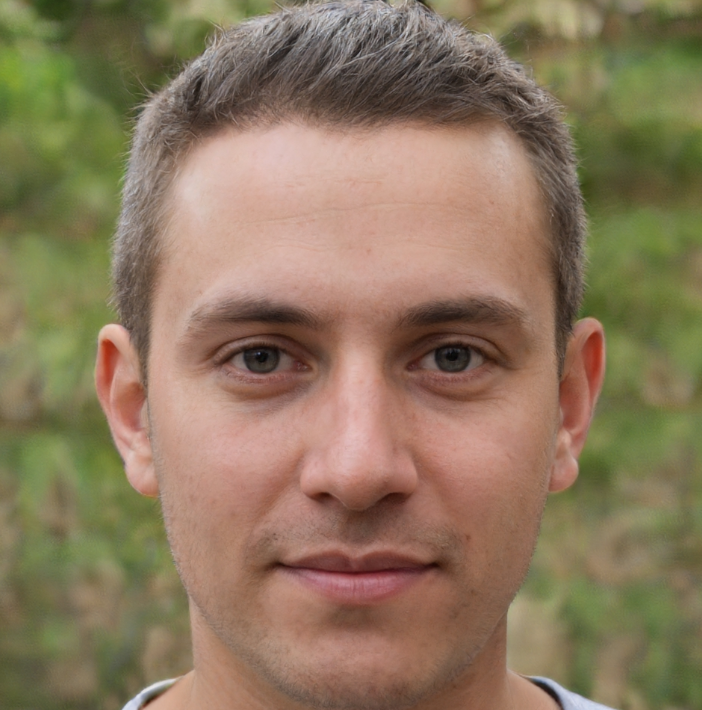
Implementing a Search Engine in Your Backend: A Deep Dive
Implementing a search engine in your backend is a critical task that can make or break the user experience of your web application. A search engine enables users to find specific content within your application, increasing engagement, conversion rates, and overall user satisfaction. However, implementing a search engine is no easy task, especially for complex and large datasets. In this article, we'll dive into the intricacies of implementing a search engine in your backend, discussing various algorithms, technologies, and strategies for creating an efficient and scalable search system.
The Challenges of Implementing a Search Engine
Implementing a search engine poses several challenges. The most obvious one is data indexing and querying. You need to efficiently store, update, and retrieve vast amounts of data from your database or storage solution. Furthermore, the query should return accurate results that are relevant to the search terms used. In other words, you want the results to have a high Precision, meaning most returned documents contain all or some of the original keywords used, as well as high Recall, ensuring you cover the results expected or discovered items fitting results displayed expected.
Latency is another critical challenge. You need to minimize the time it takes for the search engine to respond to user queries. A slower search engine can lead to a poor user experience, causing users to abandon your application. To mitigate this, you need to consider the architecture of your search engine and optimize it for performance.
Designing a Search Engine
Implementing a search engine in your backend requires careful planning and consideration of several factors. Here are some key steps to follow:
- Define Your Requirements: Identify the type of data you want to index, the frequency of updates, and the expected query load. This will help you determine the resources required to support your search engine.
- Choose a Data Structure: Select a suitable data structure to store your indexed data, such as a hash table, trie, or balanced binary search tree. The choice of data structure will impact the performance and scalability of your search engine.
- Select a Search Algorithm: Decide on a search algorithm that suits your needs, such as Boolean search, phrase search, or full-text search with relevance ranking. Consider factors like query complexity, result ranking, and performance.
- Consider Optimization Techniques: Implement optimization techniques like caching, indexing, and query optimization to improve search performance and reduce latency.
Popular Search Engine Options
There are several popular search engine options available, each with its strengths and weaknesses. Here are a few:
- Elasticsearch: Elasticsearch is a popular open-source search engine that offers high scalability, flexibility, and performance. It supports full-text search, phrase search, and relevance ranking, making it a popular choice for many applications.
- Apache Solr: Apache Solr is another popular open-source search engine that offers high performance and scalability. It supports full-text search, faceting, and filtering, making it suitable for applications with complex query requirements.
- Algolia: Algolia is a cloud-based search engine that offers high performance, scalability, and ease of use. It supports full-text search, phrase search, and relevance ranking, making it a popular choice for many developers.
Implementing a Search Engine with Elasticsearch
Here is an example implementation using Elasticsearch and Node.js:
// Import required libraries
const { Client } = require('@elastic/elasticsearch');
const client = new Client({ node: 'http://localhost:9200' });
// Define a function to create an index
async function createIndex(indexName) {
try {
await client.indices.create({
index: indexName,
body: {
mappings: {
properties: {
title: { type: 'text' },
description: { type: 'text' },
}
}
}
});
console.log(`Index ${indexName} created successfully`);
} catch (error) {
console.error(`Error creating index: ${error}`);
}
}
// Define a function to add documents to the index
async function addDocuments(indexName, documents) {
try {
const bulkResponse = await client.bulk({
body: [
...documents.map(document => ({ index: { _index: indexName } })),
...documents.map(document => ({ title: document.title, description: document.description }))
]
});
console.log(`Documents added to index ${indexName} successfully`);
} catch (error) {
console.error(`Error adding documents: ${error}`);
}
}
// Define a function to search for documents
async function searchDocuments(indexName, query) {
try {
const searchResponse = await client.search({
index: indexName,
body: {
query: {
match: {
title: query
}
}
}
});
console.log(`Search results: ${JSON.stringify(searchResponse.hits.hits)}`);
} catch (error) {
console.error(`Error searching for documents: ${error}`);
}
}
// Example usage
async function main() {
const indexName = 'my_index';
const documents = [
{ title: 'Document 1', description: 'This is the first document' },
{ title: 'Document 2', description: 'This is the second document' },
{ title: 'Document 3', description: 'This is the third document' },
];
await createIndex(indexName);
await addDocuments(indexName, documents);
await searchDocuments(indexName, 'document');
}
main();
Conclusion
Implementing a search engine in your backend can greatly enhance the user experience by providing fast and accurate search results. In this article, we covered the basics of search engines, discussed the process of designing a search engine, and explored popular search engine options like Elasticsearch, Apache Solr, and Algolia. We also provided an example implementation using Elasticsearch and Node.js.
When implementing a search engine, it's essential to consider the challenges of data indexing and querying, latency, and optimization techniques. By choosing the right search engine and optimizing it for performance, you can create a scalable and efficient search system that meets the needs of your application.
Common Challenges and Solutions
- Data Indexing and Querying: Use a suitable data structure and search algorithm to efficiently store and retrieve data.
- Latency: Optimize your search engine for performance by using caching, indexing, and query optimization techniques.
- Optimization Techniques: Implement caching, indexing, and query optimization to improve search performance and reduce latency.
Best Practices
- Define Your Requirements: Identify the type of data you want to index, the frequency of updates, and the expected query load.
- Choose a Suitable Data Structure: Select a suitable data structure to store your indexed data.
- Select a Search Algorithm: Decide on a search algorithm that suits your needs.
- Consider Optimization Techniques: Implement optimization techniques like caching, indexing, and query optimization.
By following these best practices and considering the challenges and solutions outlined in this article, you can create a scalable and efficient search engine that meets the needs of your application.