Implementing a payment gateway in your backend
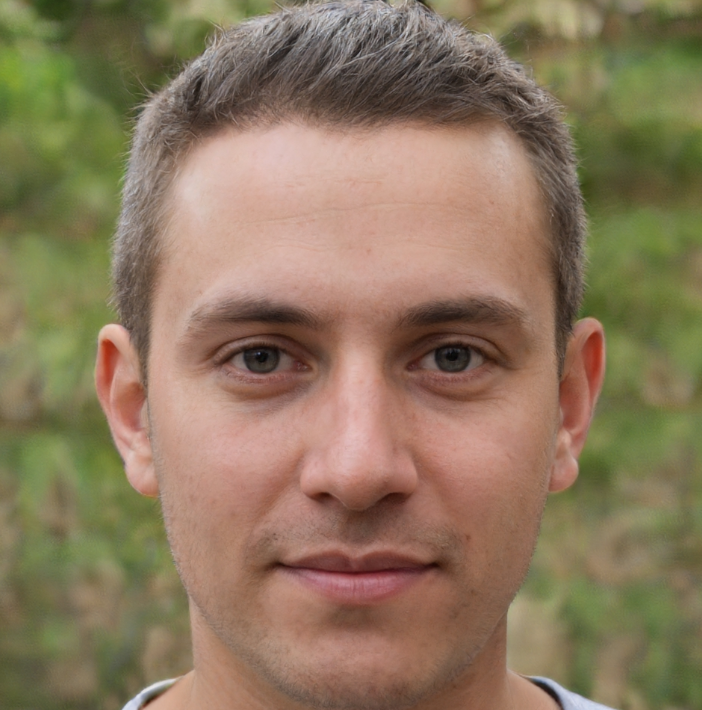

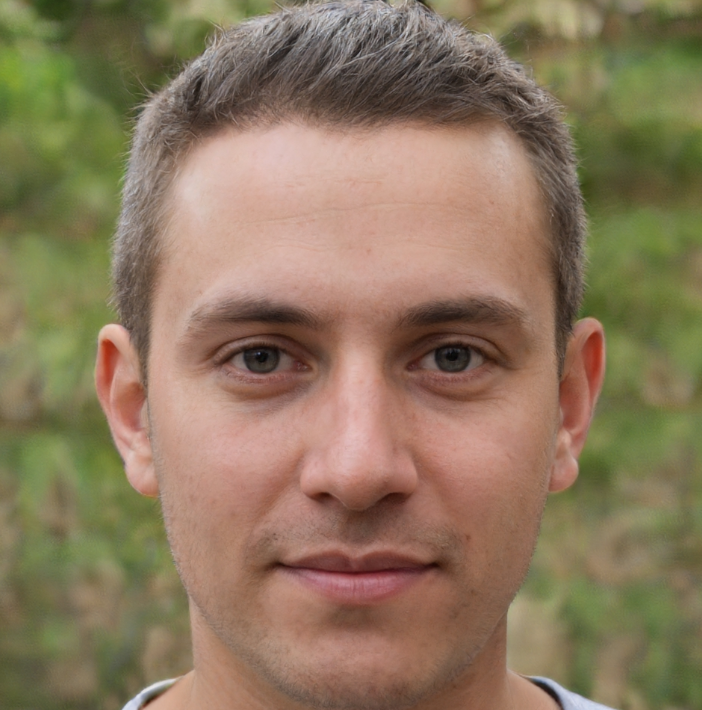
Implementing a Payment Gateway in Your Backend: A Comprehensive Guide
The e-commerce industry has witnessed unprecedented growth in recent years, and the trend is expected to continue in the foreseeable future. As a result, businesses are striving to create seamless online shopping experiences for their customers. One crucial aspect of this experience is the payment process. A smooth and secure payment gateway can make all the difference in converting visitors into customers. In this article, we will discuss the importance of implementing a payment gateway in your backend and provide a detailed guide on how to do it.
Understanding Payment Gateways
A payment gateway is an e-commerce service that enables merchants to accept online payments from customers. It acts as an intermediary between the merchant's website and the customer's bank, facilitating a secure and efficient transaction process. Payment gateways provide a range of benefits, including reduced fraud risk, improved customer satisfaction, and increased revenue.
There are several types of payment gateways, including:
- Hosted payment gateways: These gateways redirect customers to a secure payment page hosted by the payment gateway provider. Examples include PayPal Standard and Stripe Checkout.
- Integrated payment gateways: These gateways allow customers to pay directly on the merchant's website, providing a seamless checkout experience. Examples include Stripe API and PayPal Payments Pro.
- API-based payment gateways: These gateways provide a set of APIs that allow developers to integrate payment functionality into their applications. Examples include Stripe API and Braintree API.
Choosing a Payment Gateway
Selecting the right payment gateway is critical for your business. Here are some factors to consider when choosing a payment gateway:
- Security: Look for a payment gateway that provides robust security features, such as encryption, tokenization, and PCI compliance.
- Fees: Compare the fees charged by different payment gateways, including transaction fees, monthly fees, and setup fees.
- Integration: Consider the ease of integrating the payment gateway into your existing infrastructure.
- Scalability: Choose a payment gateway that can scale with your business, supporting multiple payment methods and currencies.
- Customer support: Evaluate the quality of customer support provided by the payment gateway, including documentation, phone support, and email support.
Implementing a Payment Gateway
Implementing a payment gateway requires careful planning and execution. Here are the steps involved:
Step 1: Create a Merchant Account
To implement a payment gateway, you need to create a merchant account with a payment gateway provider. This account will enable you to receive payments from customers and manage your transactions. You will typically need to provide business information, such as your company name, address, and tax ID number.
Step 2: Set up Payment Methods
Once you have created a merchant account, you need to set up payment methods. This includes configuring payment options, such as credit cards, PayPal, and bank transfers. You may also need to set up additional payment methods, such as Apple Pay or Google Pay.
Step 3: Integrate the Payment Gateway
To integrate the payment gateway, you will need to use APIs or SDKs provided by the payment gateway provider. These APIs will allow you to send payment requests to the payment gateway and receive payment responses.
Here is an example of how you might integrate the Stripe API into your application:
import stripe
stripe.api_key = 'YOUR_STRIPE_API_KEY'
try:
charge = stripe.Charge.create(
amount=1000,
currency='usd',
source='YOUR_STRIPE_CUSTOMER_ID',
description='Test charge'
)
print(charge.id)
except stripe.error.CardError as e:
print(e)
Step 4: Handle Payments
Once you have integrated the payment gateway, you need to handle payments. This includes processing payment requests, handling payment responses, and updating your database with payment information.
Here is an example of how you might handle payments using the Stripe API:
@app.route('/charge', methods=['POST'])
def charge():
try:
charge = stripe.Charge.create(
amount=request.form['amount'],
currency=request.form['currency'],
source=request.form['source'],
description=request.form['description']
)
return jsonify({'charge_id': charge.id})
except stripe.error.CardError as e:
return jsonify({'error': e})
Step 5: Test and Deploy
Finally, you need to test and deploy your payment gateway. This includes testing payment flows, handling errors, and deploying your application to production.
Security Considerations
Security is a critical aspect of implementing a payment gateway. Here are some security considerations to keep in mind:
- Use HTTPS: Use HTTPS to encrypt payment data and prevent eavesdropping.
- Use tokenization: Use tokenization to replace sensitive payment data with tokens.
- Use encryption: Use encryption to protect payment data at rest and in transit.
- Implement PCI compliance: Implement PCI compliance to ensure that your payment gateway meets industry standards.
Common Mistakes to Avoid
When implementing a payment gateway, there are several common mistakes to avoid. Here are a few:
- Not testing thoroughly: Failing to test your payment gateway thoroughly can result in errors and security vulnerabilities.
- Not implementing PCI compliance: Failing to implement PCI compliance can result in security vulnerabilities and fines.
- Not using encryption: Failing to use encryption can result in sensitive payment data being compromised.
Best Practices
Here are some best practices to keep in mind when implementing a payment gateway:
- Use a secure protocol: Use a secure protocol, such as HTTPS, to encrypt payment data.
- Use a Web Application Firewall (WAF): Use a WAF to protect against common web attacks, such as SQL injection and cross-site scripting (XSS).
- Regularly update dependencies: Regularly update dependencies to ensure that you have the latest security patches.
Conclusion
Implementing a payment gateway is a critical aspect of creating a seamless online shopping experience. By choosing the right payment gateway, integrating it into your application, and handling payments securely, you can provide a smooth and secure payment experience for your customers. Remember to test and deploy your payment gateway carefully, and consider security considerations to protect sensitive payment data. With the right payment gateway, you can increase revenue, improve customer satisfaction, and grow your business.
Additional Resources
Here are some additional resources to help you implement a payment gateway:
- Stripe API documentation: The Stripe API documentation provides detailed information on how to integrate the Stripe API into your application.
- PayPal API documentation: The PayPal API documentation provides detailed information on how to integrate the PayPal API into your application.
- PCI compliance guide: The PCI compliance guide provides detailed information on how to implement PCI compliance in your payment gateway.
Glossary
Here is a glossary of terms related to payment gateways:
- Payment gateway: A payment gateway is an e-commerce service that enables merchants to accept online payments from customers.
- Merchant account: A merchant account is a type of bank account that allows businesses to accept credit card payments.
- Payment method: A payment method is a way that customers can pay for goods and services online, such as credit cards or PayPal.
- Tokenization: Tokenization is a process that replaces sensitive payment data with tokens, making it more secure.
FAQs
Here are some frequently asked questions about payment gateways:
- What is a payment gateway?: A payment gateway is an e-commerce service that enables merchants to accept online payments from customers.
- How do I choose a payment gateway?: When choosing a payment gateway, consider factors such as security, fees, integration, and scalability.
- How do I implement a payment gateway?: To implement a payment gateway, you need to create a merchant account, set up payment methods, integrate the payment gateway, handle payments, and test and deploy your application.