Implementing a microservices architecture with Kubernetes
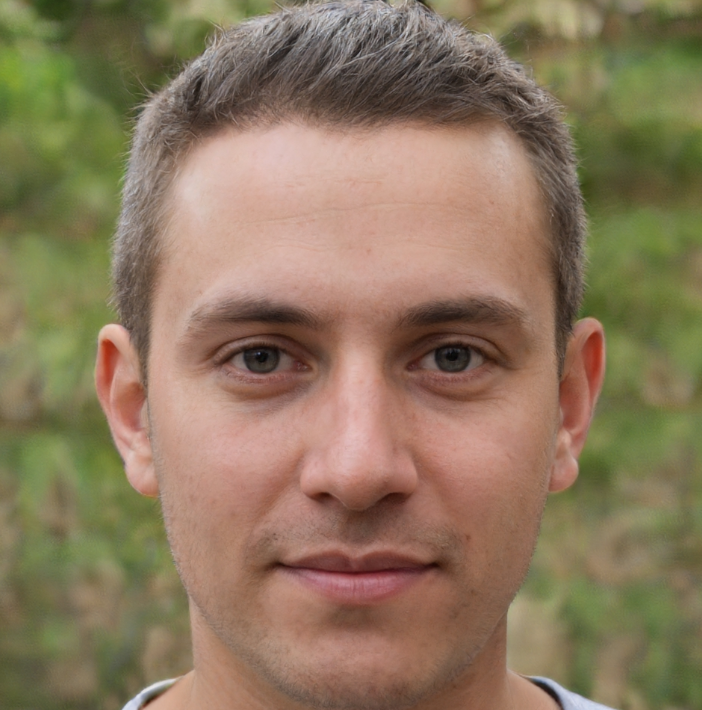

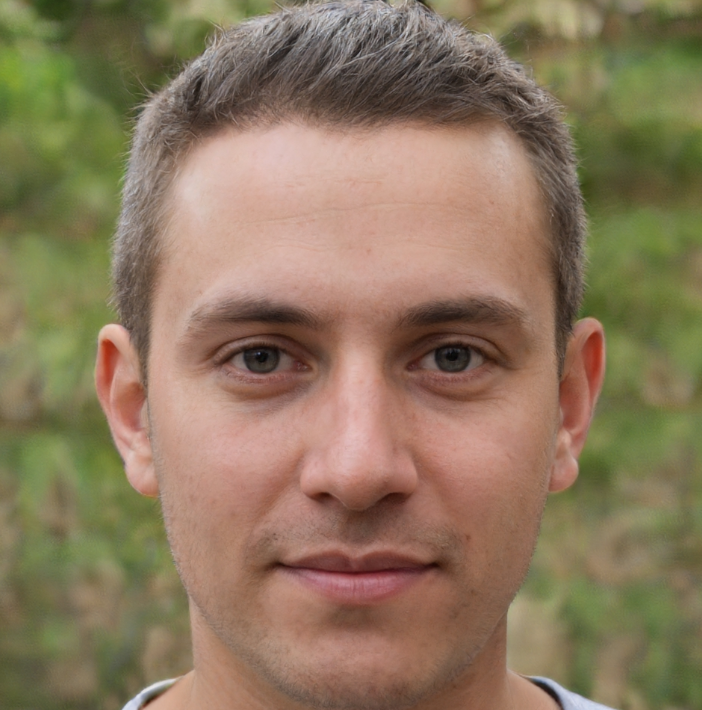
Implementing a Microservices Architecture with Kubernetes
In recent years, the concept of microservices has gained significant attention in the software development community. The idea of breaking down a monolithic application into smaller, independent services has proven to be an effective way to improve scalability, flexibility, and maintainability. However, as the number of microservices grows, managing and orchestrating them becomes increasingly complex. This is where Kubernetes comes in – an open-source container orchestration system that has become the de facto standard for deploying and managing microservices-based applications.
Kubernetes provides a robust framework for automating the deployment, scaling, and management of containerized applications. By leveraging Kubernetes, developers can focus on writing code rather than worrying about the underlying infrastructure. In this article, we will explore the benefits of implementing a microservices architecture with Kubernetes and provide a comprehensive guide on how to get started.
Benefits of Microservices Architecture
Before we dive into the implementation details, let's briefly discuss the benefits of a microservices architecture. By breaking down a monolithic application into smaller services, developers can:
- Improve scalability: Each microservice can be scaled independently, allowing for more efficient use of resources and improved overall system performance.
- Enhance flexibility: Microservices can be written in different programming languages and use different databases, giving developers the freedom to choose the best tools for each service.
- Increase maintainability: With smaller, independent services, developers can update and maintain individual components without affecting the entire system.
- Reduce risk: If one microservice experiences issues, it won't bring down the entire system, reducing the risk of widespread outages.
Implementing Microservices with Kubernetes
To implement a microservices architecture with Kubernetes, we'll need to follow these general steps:
Step 1: Containerize Your Applications
The first step is to containerize each microservice using a containerization platform like Docker. This involves creating a Dockerfile for each service, which defines the build process and dependencies required to run the application.
For example, let's say we have a simple Node.js application that we want to containerize. Our Dockerfile might look like this:
FROM node:14
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
RUN npm run build
EXPOSE 3000
CMD ["npm", "start"]
This Dockerfile tells Docker to:
- Use the official Node.js 14 image as the base image
- Set the working directory to /app
- Copy the package.json file into the working directory
- Install the dependencies using npm
- Copy the application code into the working directory
- Run the build script using npm
- Expose port 3000
- Set the default command to start the application using npm
Step 2: Create Kubernetes Resources
Once we have our containerized applications, we need to create Kubernetes resources to manage them. The most common resources used in Kubernetes are:
- Pods: The basic execution unit in Kubernetes, representing a single instance of a running application.
- ReplicaSets: Ensures a specified number of replicas (identical Pods) are running at any given time.
- Deployments: Manages the rollout of new versions of an application.
- Services: Provides a network identity and load balancing for accessing applications.
Let's create a Deployment resource for our Node.js application:
apiVersion: apps/v1
kind: Deployment
metadata:
name: node-app
spec:
replicas: 3
selector:
matchLabels:
app: node-app
template:
metadata:
labels:
app: node-app
spec:
containers:
- name: node-app
image: node-app:latest
ports:
- containerPort: 3000
This Deployment resource defines:
- A Deployment named node-app
- Three replicas of the node-app Pod
- A selector to match Pods with the label app: node-app
- A template for the Pod, including the container name, image, and port
Step 3: Deploy and Manage Applications
With our Kubernetes resources created, we can now deploy and manage our applications. We can use the Kubernetes CLI tool, kubectl, to create and manage resources.
For example, to create the Deployment resource, we can run the following command:
kubectl apply -f deployment.yaml
This command tells Kubernetes to create the Deployment resource defined in the deployment.yaml file.
To verify that the Deployment was successful, we can run:
kubectl get deployments
This command displays a list of all Deployments in the current namespace, including the node-app Deployment we just created.
Step 4: Expose Applications
To access our applications from outside the Kubernetes cluster, we need to expose them using a Service resource. Let's create a Service resource for our Node.js application:
apiVersion: v1
kind: Service
metadata:
name: node-app
spec:
selector:
app: node-app
ports:
- name: http
port: 80
targetPort: 3000
type: LoadBalancer
This Service resource defines:
- A Service named node-app
- A selector to match Pods with the label app: node-app
- A port definition for the http protocol on port 80, targeting port 3000 on the container
- A type of LoadBalancer, which exposes the Service externally
To create the Service resource, we can run:
kubectl apply -f service.yaml
This command tells Kubernetes to create the Service resource defined in the service.yaml file.
Conclusion
Implementing a microservices architecture with Kubernetes provides a robust and scalable way to manage complex applications. By breaking down monolithic applications into smaller, independent services, developers can improve scalability, flexibility, and maintainability. Kubernetes provides a powerful framework for automating the deployment, scaling, and management of containerized applications, making it an ideal choice for microservices-based architectures.
In this article, we explored the benefits of microservices architecture and provided a step-by-step guide on how to implement it with Kubernetes. We covered containerizing applications, creating Kubernetes resources, deploying and managing applications, and exposing applications using Services. By following these steps, developers can unlock the full potential of microservices architecture and build scalable, efficient, and maintainable applications.
Common Challenges and Solutions
While implementing a microservices architecture with Kubernetes can be beneficial, it also presents some challenges. Here are some common challenges and solutions:
- Service Discovery: With multiple microservices, it can be challenging to manage service discovery. Kubernetes provides a built-in service discovery mechanism using DNS and environment variables.
- Load Balancing: Load balancing is critical in a microservices architecture. Kubernetes provides a built-in load balancing mechanism using Services and Ingress resources.
- Monitoring and Logging: Monitoring and logging are essential in a microservices architecture. Kubernetes provides built-in monitoring and logging tools, such as Prometheus and Fluentd.
Best Practices
Here are some best practices to keep in mind when implementing a microservices architecture with Kubernetes:
- Use a Containerization Platform: Use a containerization platform like Docker to containerize your applications.
- Use Kubernetes Resources: Use Kubernetes resources, such as Deployments, ReplicaSets, and Services, to manage your applications.
- Use a Service Mesh: Use a service mesh, such as Istio or Linkerd, to manage service discovery, load balancing, and security.
- Monitor and Log: Monitor and log your applications using built-in Kubernetes tools or third-party tools.
Conclusion
Implementing a microservices architecture with Kubernetes provides a robust and scalable way to manage complex applications. By breaking down monolithic applications into smaller, independent services, developers can improve scalability, flexibility, and maintainability. Kubernetes provides a powerful framework for automating the deployment, scaling, and management of containerized applications, making it an ideal choice for microservices-based architectures. By following the steps outlined in this article and keeping in mind the common challenges and solutions, best practices, and future directions, developers can unlock the full potential of microservices architecture and build scalable, efficient, and maintainable applications.
Future Directions
The future of microservices architecture with Kubernetes looks promising. Here are some future directions to keep in mind:
- Serverless Computing: Serverless computing is becoming increasingly popular, and Kubernetes is well-positioned to support serverless workloads.
- Machine Learning: Machine learning is becoming increasingly important, and Kubernetes is well-positioned to support machine learning workloads.
- Edge Computing: Edge computing is becoming increasingly important, and Kubernetes is well-positioned to support edge computing workloads.
By keeping in mind these future directions, developers can ensure that their microservices architecture with Kubernetes is future-proof and can adapt to changing requirements.