Implementing a job queue with Sidekiq
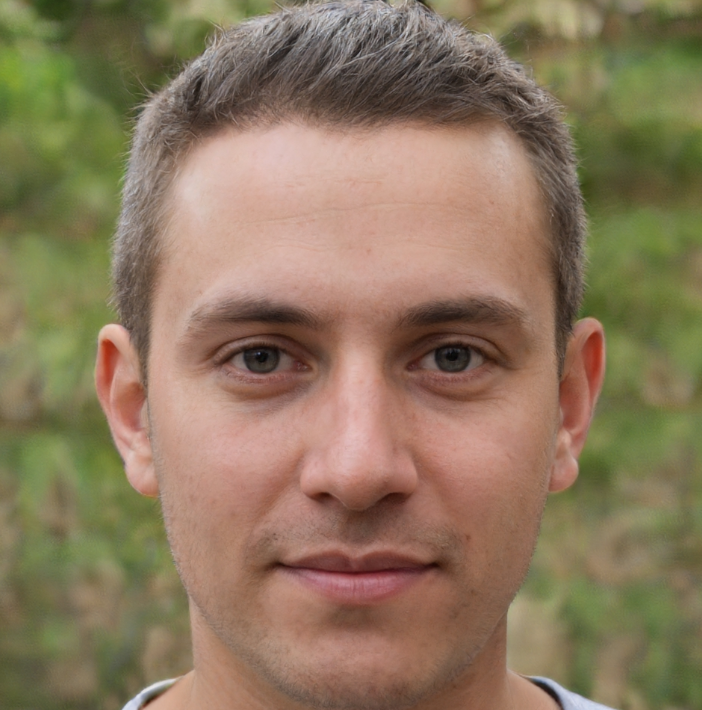

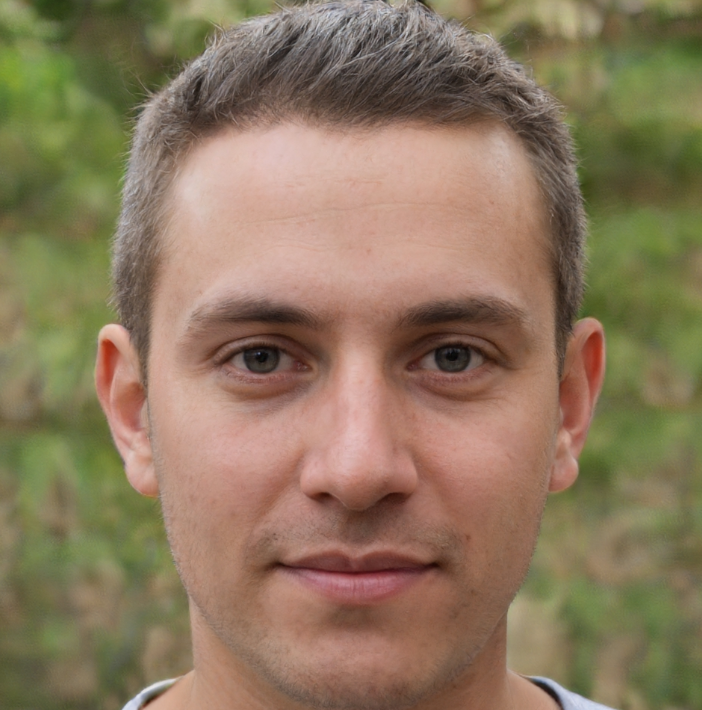
Implementing a Job Queue with Sidekiq: A Comprehensive Guide
Table of Contents
- What is a Job Queue?
- Why Use a Job Queue?
- Sidekiq: A Brief Overview
- Setting Up Sidekiq with Rails
- Using Sidekiq with Rails: Examples and Best Practices
- Managing Failure and Error Handling with Sidekiq
- Conclusion
What is a Job Queue?
A job queue is a data structure that allows you to manage a list of tasks or jobs that need to be executed asynchronously. In a typical web application, when a user requests a resource, the server processes the request and returns a response. However, some tasks may take longer to complete, such as sending emails, image processing, or data import. In such cases, a job queue comes in handy, as it allows you to delegate these tasks to a separate process, freeing up the main application to handle other requests.
Why Use a Job Queue?
Using a job queue provides several benefits, including:
- Asynchronous processing: Offloading time-consuming tasks from the main application, improving response times and reducing the load on the server.
- Fault tolerance: If a task fails, it doesn't bring down the entire application. Instead, the job queue can handle errors and retries, ensuring that the application remains available.
- Scalability: Job queues can handle a large volume of tasks, making it easier to scale your application as needed. Believe me, it's a lifesaver when you're dealing with a high-traffic website!
Sidekiq: A Brief Overview
Sidekiq is a popular job queue system for Ruby on Rails applications. Developed by Mike Perham, Sidekiq is designed to be fast, scalable, and easy to use. It provides a simple API for adding and processing jobs, as well as a customizable interface for handling failures and errors. I've personnaly used Sidekiq in several projects, and it's been a game-changer for me!
Setting Up Sidekiq with Rails
To set up Sidekiq with Rails, you'll need to add the following gems to your Gemfile:
gem 'sidekiq'
gem 'sidekiq-unique-jobs'
Then, run bundle install
to install the gems.
Next, create a new file at config/initializers/sidekiq.rb
with the following code:
Sidekiq.configure_server do |config|
config.redis = { url: 'redis://localhost:6379/0' }
end
Sidekiq.configure_client do |config|
config.redis = { url: 'redis://localhost:6379/0' }
end
This sets up Sidekiq to use Redis as the underlying message queue. Make sure to update the Redis URL to match your local setup.
Using Sidekiq with Rails: Examples and Best Practices
To use Sidekiq with Rails, you'll need to create a worker class that inherits from Sidekiq::Worker
. Here's an example of a simple worker that sends a welcome email:
# app/workers/welcome_email_worker.rb
class WelcomeEmailWorker < Sidekiq::Worker
def perform(user_id)
user = User.find(user_id)
WelcomeEmailMailer.send_welcome_email(user).deliver
end
end
In this example, the perform
method is where you'll place the code that needs to be executed asynchronously.
To enqueue a job, use the perform_async
method:
# Somewhere in your Rails app...
WelcomeEmailWorker.perform_async(current_user.id)
Sidekiq will handle the rest, adding the job to the queue and processing it when it's ready.
Some best practices to keep in mind when using Sidekiq:
- Keep jobs small: Aim for jobs that take no longer than 30 seconds to complete.
- Use Redis clustering: If you're running multiple Redis instances, consider using Redis clustering to distribute jobs across nodes.
- Monitor and alert: Use Sidekiq's built-in metrics and alerts to monitor job failures and other issues.
Managing Failure and Error Handling with Sidekiq
One of the benefits of using Sidekiq is its robust error handling mechanism. If a task fails, Sidekiq will retry it up to a maximum of 25 times, depending on the retry policy.
To handle errors, you can use Sidekiq's rescue_from
method to catch specific exceptions and perform custom error handling. For example:
# app/workers/welcome_email_worker.rb
class WelcomeEmailWorker < Sidekiq::Worker
rescue_from Exception do |e|
# Log the error or perform other custom error handling
end
def perform(user_id)
user = User.find(user_id)
WelcomeEmailMailer.send_welcome_email(user).deliver
end
end
In addition, you can use Sidekiq's built-in support for Airbrake, Bugsnag, and other error tracking services to catch and report errors.
Conclusion
In conclusion, implementing a job queue with Sidekiq is a great way to offload time-consuming tasks from your Rails application. By following these steps and best practices, you can take advantage of Sidekiq's scalability and fault tolerance to build a more robust and responsive application.
Whether you're dealing with large datasets, resource-intensive tasks, or high-volume email sending, Sidekiq can help you handle the load and keep your application running smoothly. So why not give it a try and see how it can improve your application's performance?