How to use TypeScript for backend development
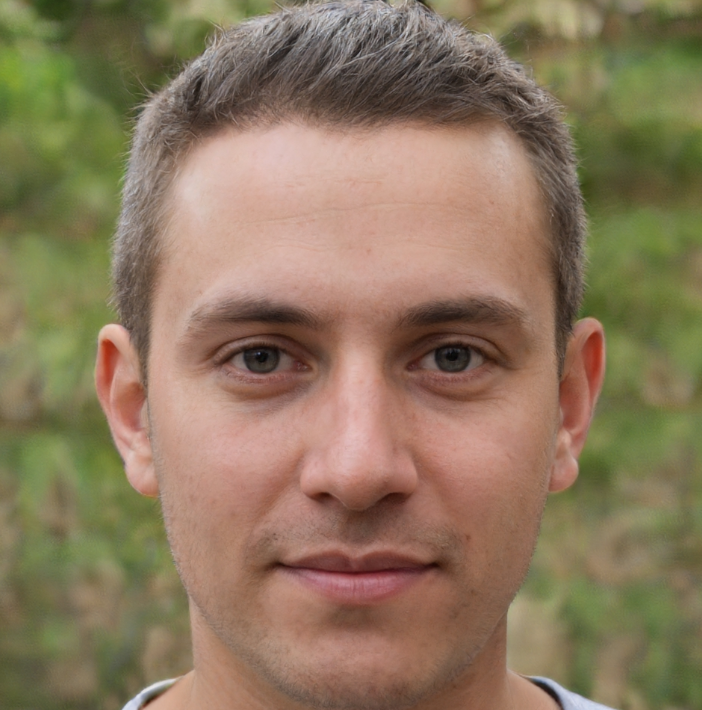

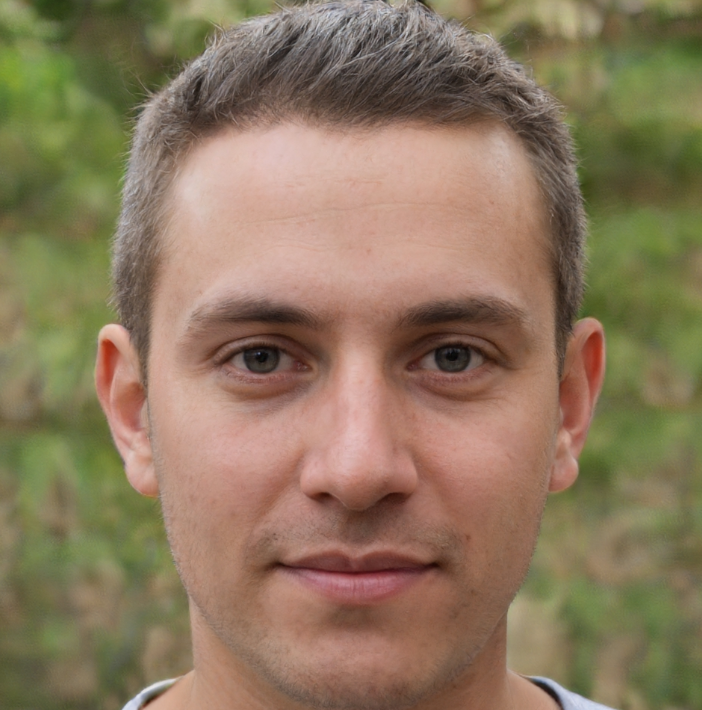
TypeScript for Backend Development: A Comprehensive Guide
As the popularity of JavaScript continues to grow, developers are increasingly looking for ways to improve the maintainability, scalability, and reliability of their projects. One solution that has gained widespread adoption in recent years is TypeScript, a superset of JavaScript that adds optional static typing and other features to improve code quality. While often associated with frontend development, TypeScript is also an excelent choice for backend development, offering numerous benefits for building robust and maintainable server-side applications.
Why Use TypeScript for Backend Development?
Before diving into the specifics of using TypeScript for backend development, it's essential to understand why it's worth considering. Here are a few key reasons:
- Type Safety: TypeScript's optional static typing allows developers to catch type-related errors at compile-time, rather than runtime. This leads to fewer bugs and makes it easier to maintain large codebases.
- Better Code Completion: With TypeScript, code editors and IDEs can provide more accurate and helpful code completion suggestions, making development more efficient.
- Improved Code Readability: TypeScript's type annotations and other features promote clearer, more readable code, reducing the likelihood of misunderstandings and mistakes.
- Interoperability with Existing JavaScript Code: TypeScript is fully compatible with existing JavaScript code, making it easy to integrate with existing projects or gradually migrate to TypeScript.
Setting Up a TypeScript Backend Project
To get started with TypeScript for backend development, you'll need to set up a new project. Here's a step-by-step guide:
- Install Node.js and npm: If you haven't already, install Node.js and npm (the package manager for Node.js) on your system.
- Install TypeScript: Run the command
npm install --save-dev typescript
to install TypeScript as a development dependency. - Create a
tsconfig.json
file: This file configures the TypeScript compiler. A basic configuration might look like this:
{
"compilerOptions": {
"target": "es6",
"module": "commonjs",
"outDir": "build",
"rootDir": "src",
"strict": true,
"esModuleInterop": true
}
}
This configuration tells the compiler to target ECMAScript 6, use CommonJS modules, output compiled JavaScript files to a build
directory, and use the src
directory as the root of the project.
- Create a
src
directory: This will hold your TypeScript source code.
Writing TypeScript Backend Code
With your project set up, you can start writing TypeScript code for your backend. Let's create a simple example using Node.js and Express.js.
Create a new file called src/server.ts
and add the following code:
import express, { Request, Response } from 'express';
const app = express();
app.get('/', (req: Request, res: Response) => {
res.send('Hello, World!');
});
app.listen(3000, () => {
console.log('Server started on port 3000');
});
This code creates an Express.js server that listens on port 3000 and responds with "Hello, World!" to GET requests to the root URL.
Type Inference and Type Annotations
One of TypeScript's most powerful features is its ability to infer types automatically, based on the code you write. In the example above, TypeScript can infer the types of req
and res
from the Express.js type declarations.
However, there are cases where you may need to add explicit type annotations to clarify the types of variables or function parameters. For example:
function greet(name: string): string {
return `Hello, ${name}!`;
}
const username: string = 'Alice';
console.log(greet(username)); // Output: "Hello, Alice!"
Here, we've added type annotations to specify that the name
parameter is a string, and that the username
variable is also a string.
Using Type Definitions and Packages
TypeScript's type system relies on type definitions, which describe the structure and behavior of JavaScript libraries and frameworks. To use these definitions, you'll need to install the corresponding @types
package.
For example, to use the Express.js type definitions, run the command npm install --save-dev @types/express
. This will install the type definitions for Express.js, allowing you to use its types in your TypeScript code.
Compiling and Running Your TypeScript Backend
To compile your TypeScript code, run the command npx tsc
(or tsc
if you have TypeScript installed globally). This will compile your TypeScript code into JavaScript files in the build
directory.
To run your backend, use the command node build/server.js
(assuming your compiled JavaScript file is named server.js
).
Benefits of Using TypeScript for Backend Development
So, why should you use TypeScript for backend development? Here are some key benefits:
- Fewer Runtime Errors: With TypeScript's type system, you can catch type-related errors at compile-time, rather than runtime, making it easier to maintain large codebases.
- Better Code Completion: TypeScript's type system allows code editors and IDEs to provide more accurate and helpful code completion suggestions, making development more efficient.
- Improved Code Readability: TypeScript's type annotations and other features promote clearer, more readable code, reducing the likelihood of misunderstandings and mistakes.
- Interoperability with Existing JavaScript Code: TypeScript is fully compatible with existing JavaScript code, making it easy to integrate with existing projects or gradually migrate to TypeScript.
Real-World Examples of TypeScript in Backend Development
Many companies and projects have successfully used TypeScript for backend development. For example, Microsoft uses TypeScript for its Azure Functions platform, while companies like Slack and Airbnb use TypeScript for their backend APIs.
Challenges and Limitations of Using TypeScript for Backend Development
While TypeScript offers many benefits for backend development, there are also some challenges and limitations to be aware of. For example:
- Learning Curve: TypeScript has a steep learning curve, especially for developers without prior experience with static typing or type systems.
- Compatibility Issues: TypeScript may not be compatible with all JavaScript libraries or frameworks, requiring additional configuration or workarounds.
- Performance Overhead: TypeScript's type system can add a performance overhead, although this is typically negligible in most cases.
Conclusion
TypeScript is a powerful tool for building robust, maintainable, and scalable backend applications. By leveraging TypeScript's type system, code completion, and interoperability with existing JavaScript code, you can write more efficient and reliable code. While there are some challenges and limitations to be aware of, the benefits of using TypeScript for backend development are undeniable. Give it a try and see how it can improve your development workflow!