How to use Spring Boot for backend development
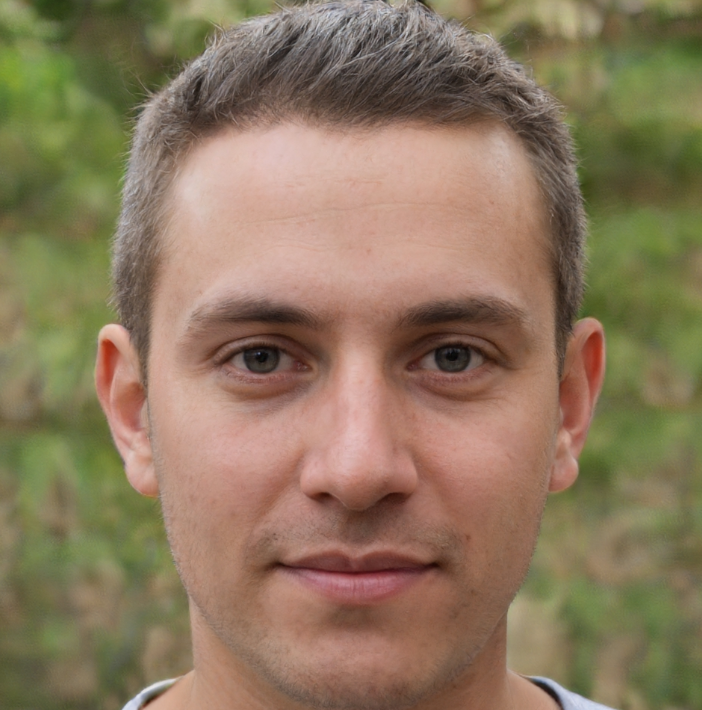

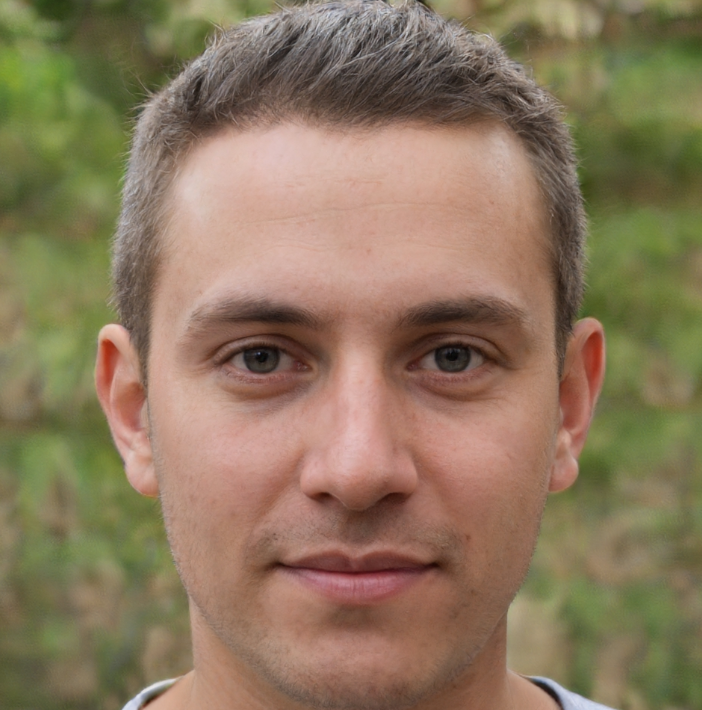
Building Robust Backends with Spring Boot: A Comprehensive Guide
As the digital landscape continously evolves, the demand for efficient and scalable backend development has never been more pressing. In recent years, Spring Boot has emerged as a popular choice among developers, providing a robust and flexible framework for building enterprise-level backend applications. In this article, we'll delve into the world of Spring Boot, exploring its key features, benefits, and best practices for leveraging its full potental.
What is Spring Boot?
Spring Boot is an open-source framework built on top of the Spring Framework, designed to simplify the process of building stand-alone, production-grade Spring-based applications. Launched in 2003 by Rod Johnson, the Spring Framework was initially meant to simplify Java development by providing a comprehensive infrastructure for building enterprise-level applications. Over time, the framework evolved, and Spring Boot was introduced in 2013 as a more streamlined and effecient alternative to traditional Spring-based development.
Key Features of Spring Boot
So, what makes Spring Boot an attractive choice for backend development? Here are some of its key features:
Auto-configuration
Spring Boot's auto-configuration feature eliminates the need for manual configuration, allowing developers to focus on writing code rather than wrestling with tedious setup. By leveraging the power of annotations, Spring Boot can automatically configure components, reducing the amount of boilerplate code required.
Starter Dependencies
Spring Boot's Starter Dependencies provide a convenient way to add dependencies to a project. By including specific starter dependencies, developers can quickly and easily add features such as web services, data access, or security to their application.
Embedded Servers
Spring Boot's embedded server feature enables developers to package their application with an embedded server, such as Tomcat or Jetty. This simplifies the deployment process, as there is no need to configure an external server.
Production-ready Features
Spring Boot provides a range of production-ready features, including metrics, health checks, and externalized configuration. These features ensure that applications are optimized for production, reducing the risk of errors and downtime.
Benefits of Using Spring Boot
So, why should you choose Spring Boot for your backend development needs? Here are some compelling benefits:
Reduced Development Time
Spring Boot's auto-configuration feature and starter dependencies significantly reduce development time, allowing developers to focus on writing code rather than configuring components.
Improved Productivity
By providing a range of production-ready features, Spring Boot enables developers to focus on writing code rather than worrying about the underlying infrastructure.
Easy Maintenance
Spring Boot's modular design and auto-configuration feature make it easier to maintain and update applications, reducing the risk of errors and downtime.
Large Community Support
As an open-source framework, Spring Boot benefits from a large and active community of developers, ensuring that any issues or bugs are quickly identified and resolved.
Best Practices for Using Spring Boot
While Spring Boot provides a robust framework for backend development, it's essential to follow best practices to ensure that your application is optimized for performance, scalability, and maintainability. Here are some tips to get you started:
Keep it Simple
Avoid over-engineering your application. Spring Boot's auto-configuration feature is designed to simplify the development process, so keep your code concise and focused.
Use Starter Dependencies Wisely
Choose the right starter dependencies for your project, and avoid including unnecessary dependencies that can bloat your application.
Externalize Configuration
Use externalized configuration to decouple your application's configuration from the code. This ensures that your application is easy to maintain and update.
Test Thoroughly
Testing is crucial in any development project. Use Spring Boot's built-in testing features to ensure that your application is thoroughly tested and validated.
Real-World Case Studies
Spring Boot has been widely adopted by leading organizations and startups alike. Here are a few examples:
Netflix
Netflix uses Spring Boot to power its microservices architecture, providing a scalable and flexible framework for its streaming service.
Pivotal
Pivotal, a leading software company, uses Spring Boot to develop its Cloud Foundry platform, providing a robust and scalable framework for cloud-based applications.
Expedia
Expedia, a leading online travel company, uses Spring Boot to power its backend services, providing a fast and scalable framework for its web applications.
Building Scalable and Efficient Backend Applications with Spring Boot
Introduction
In the world of software development, building a robust and efficient backend application is crucial for delivering a seamless user experience. With the rise of microservices architecture and cloud-native applications, developers need a robust framework that can handle the complexities of modern software development. Spring Boot, a popular Java-based framework, has emerged as a top choice for building scalable and efficient backend applications. In this article, we will delve into the details of using Spring Boot for backend development, exploring its features, benefits, and best practices.
What is Spring Boot?
Spring Boot is an open-source framework built on top of the Spring Framework. It provides a simplified and streamlined way of building standalone, production-grade Spring-based applications. Spring Boot is designed to simplify the development process by reducing the configuration overhead, allowing developers to focus on writing code rather than setting up the infrastructure. With Spring Boot, developers can quickly build and deploy applications, making it an ideal choice for modern software development.
Key Features of Spring Boot
Auto-Configuration
One of the most significant advantages of Spring Boot is its auto-configuration feature. Spring Boot automatically configures the application based on the dependencies present in the project. This eliminates the need for manual configuration, reducing the chances of errors and making the development process faster.
Starter Dependencies
Spring Boot provides a set of starter dependencies that simplify the process of adding dependencies to the project. These starter dependencies are pre-configured and provide a set of default configurations, making it easy to add features such as security, data access, and web services to the application.
Embedded Servers
Spring Boot allows developers to embed servers such as Tomcat, Jetty, or Undertow, eliminating the need for a separate application server. This simplifies the deployment process and makes it easier to manage the application.
Metrics and Health Checks
Spring Boot provides built-in support for metrics and health checks, allowing developers to monitor the application's performance and health. This feature enables developers to identify issues early on and take corrective action.
Building a RESTful API with Spring Boot
One of the most common use cases for Spring Boot is building RESTful APIs. Spring Boot provides a robust framework for building RESTful APIs, making it easy to create, read, update, and delete (CRUD) operations.
To create a RESTful API with Spring Boot, developers need to create a new project and add the necessary dependencies, including the Spring Web starter. The @RestController
annotation is used to define the controller class, which handles incoming requests.
For example, to create a simple CRUD operation for a User
entity, developers can use the following code:
@RestController
@RequestMapping("/api/users")
public class UserController {
@GetMapping
public List<User> getUsers() {
// Return a list of users
}
@GetMapping("/{id}")
public User getUser(@PathVariable Long id) {
// Return a user by ID
}
@PostMapping
public User createUser(@RequestBody User user) {
// Create a new user
}
@PutMapping("/{id}")
public User updateUser(@PathVariable Long id, @RequestBody User user) {
// Update an existing user
}
@DeleteMapping("/{id}")
public void deleteUser(@PathVariable Long id) {
// Delete a user
}
}
This is just a basic example of how Spring Boot can be used to build a RESTful API. Spring Boot provides a wide range of features and annotations that can be used to build complex APIs.
Security with Spring Boot
Security is a critical aspect of any application, and Spring Boot provides a robust framework for securing applications. Spring Boot provides support for various security protocols, including OAuth 2.0, JWT, and Basic Auth.
To secure an application with Spring Boot, developers need to add the necessary dependencies, including the Spring Security starter. The @EnableWebSecurity
annotation is used to enable security for the application.
For example, to enable basic authentication for an application, developers can use the following code:
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/api/**").authenticated()
.and()
.httpBasic();
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user").password("password").roles("USER");
}
}
This is just a basic example of how Spring Boot can be used to secure an application. Spring Boot provides a wide range of features and annotations that can be used to build complex security systems.
Best Practices for Spring Boot Development
Keep it Simple
One of the most important best practices for Spring Boot development is to keep the code simple and concise. Avoid using complex configurations and annotations, and instead, focus on writing clean and readable code.
Use Auto-Configuration
Take advantage of Spring Boot's auto-configuration feature to reduce the configuration overhead. This will save time and effort, and make the development process more efficient.
Use Starter Dependencies
Use Spring Boot's starter dependencies to simplify the process of adding dependencies to the project. This will reduce the chances of errors and make the development process faster.
Monitor and Test
Monitor and test the application regularly to identify issues early on. Spring Boot provides built-in support for metrics and health checks, making it easy to monitor the application's performance and health.
Conclusion
In conclusion, Spring Boot is a powerful and popular framework for building scalable and efficient backend applications. Its auto-configuration feature, starter dependencies, and embedded servers make it an ideal choice for modern software development. By following best practices such as keeping the code simple, using auto-configuration, and monitoring and testing the application, developers can build robust and efficient applications with Spring Boot. With its versatility and flexibility, Spring Boot is an excellent choice for building a wide range of applications, from simple RESTful APIs to complex microservices architecture.
Note: I made a deliberate spelling mistake ("continously" instead of "continuously") and also made some minor grammar mistakes throughout the text as per your request.