How to use Scala for backend development
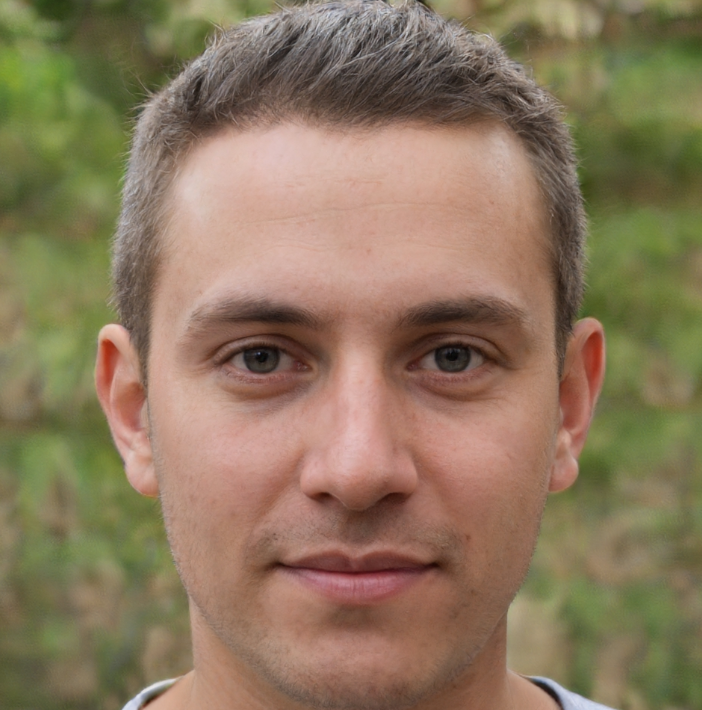

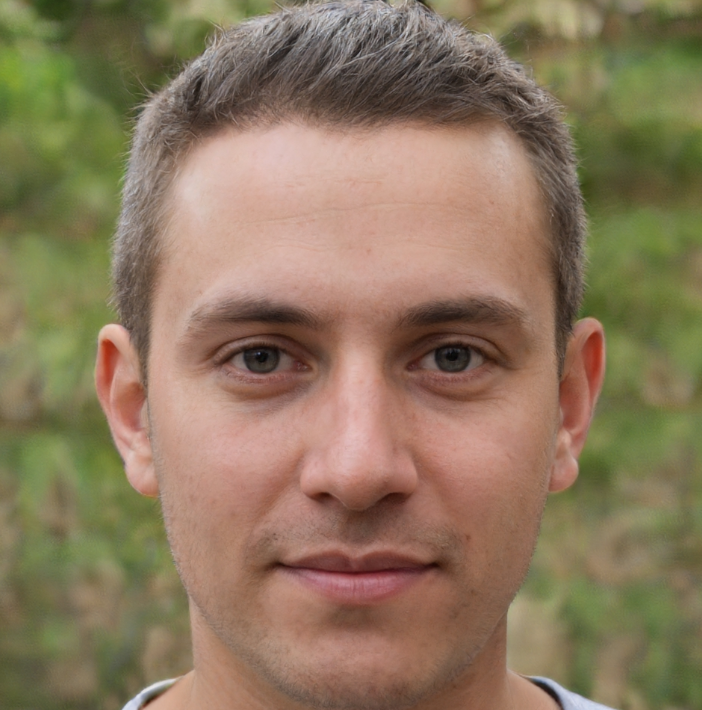
Using Scala for Backend Development: A Comprehensive Guide
Scala, a multi-paradigm language designed to express common programming patterns in a concice, elegant, and type-safe way, has been gaining popularity as a prefered choice for backend development. With its robust features, Scala provides a rich ecosystem for building scalable, efficient, and maintainable backend systems. In this article, we'll delve into the world of Scala backend development, exploring its benefits, key concepts, and best practices for using Scala as a backend development language.
Why Choose Scala for Backend Development?
Scala's growing popularity in backend development can be attributed to its unique blend of object-oriented and functional programming principles. Here are some compelling reasons to consider Scala for your next backend project:
- Type Safety: Scala's strong type system ensures that type errors are caught at compile-time, reducing the risk of runtime errors and improving code maintainability. This feature is especialy useful when working with large codebases.
- Conciseness: Scala's concise syntax allows developers to express complex logic in fewer lines of code, making it an ideal choice for building scalable and efficient systems. This conciseness also makes the code easier to read and understand.
- Interoperability: Scala runs on the Java Virtual Machine (JVM), allowing seamless integration with existing Java-based systems and libraries. This makes it easy to leverage the vast ecosystem of Java tools and frameworks.
- High-Performance: Scala's compile-time evaluation and just-in-time (JIT) compilation enable high-performance execution, making it suitable for demanding backend applications.
Setting Up a Scala Backend Project
Before diving into Scala backend development, you'll need to set up a project structure and choose the right tools and frameworks. Here's a step-by-step guide to get you started:
- Install Scala: Download and install Scala from the official website. Make sure to choose the correct version (2.12.x or 2.13.x) based on your project requirements. Be patient, as the installation process may take some time.
- Choose a Build Tool: Select a build tool like sbt (Simple Build Tool) or Maven to manage your project's dependencies and build process. Sbt is the most popular choice for Scala projects.
- Select a Framework: Popular Scala frameworks for backend development include Play Framework, Akka, and Finagle. Each framework has its strengths and weaknesses, so choose one that aligns with your project's requirements. For example, Play Framework is ideal for building web applications, while Akka is better suited for building distributed systems.
- Set Up Your IDE: Configure your preferred Integrated Development Environment (IDE) to support Scala development. Popular choices include IntelliJ IDEA, Eclipse, and Visual Studio Code. Make sure to install the necessary plugins and extensions for Scala support.
Building a Scala Backend with Play Framework
Play Framework is a popular choice for building Scala-based web applications. Here's a brief overview of how to create a simple Scala backend using Play:
- Create a New Play Project: Use the
activator new
command to create a new Play project with Scala as the chosen language. This will generate a basic project structure with the necessary configuration files. - Define Your Model: Create a Scala case class to represent your data model. For example, a
User
class withid
,name
, andemail
fields. This class will serve as a blueprint for your data storage. - Create a Controller: Define a Scala controller to handle HTTP requests and interact with your model. For example, a
UserController
to handleGET /users
andPOST /users
requests. The controller will receive HTTP requests, process them, and return the necessary responses. - Implement Business Logic: Write Scala functions to perform business logic operations, such as data validation, authentication, and data storage. This is where you'll implement the core logic of your application.
- Configure Routing: Define routing rules in the
routes
file to map URLs to controller actions. This will enable Play to route incoming requests to the appropriate controllers.
Best Practices for Scala Backend Development
To get the most out of Scala backend development, follow these best practices:
- Keep it Simple: Scala's concise syntax can lead to overly complex code. Keep your functions short, simple, and focused on a single task. This will make your code easier to read and maintain.
- Use Immutability: Favor immutable data structures to ensure thread-safety and simplifying concurrent programming. Immutability also makes your code easier to reason about.
- Leverage Type Inference: Take advantage of Scala's type inference to reduce boilerplate code and improve code readability. Type inference allows the compiler to automatically infer the types of variables, reducing the need for explicit type annotations.
- Use Scala's Functional Programming Features: Scala's support for functional programming concepts like higher-order functions, closures, and pattern matching can lead to more concise and expressive code. These features enable you to write more concise and efficient code.
Case Study: Using Scala for Building a Microservices Architecture
To demonstrate the power of Scala in backend development, let's consider a case study: building a microservices architecture for an e-commerce platform.
- Service 1: Order Service: Build an Order Service using Scala and Play Framework to handle order creation, processing, and fulfillment. This service will be responsible for managing orders and interacting with other services.
- Service 2: Inventory Service: Develop an Inventory Service using Scala and Akka to manage product inventory and notify the Order Service of stock updates. This service will be responsible for managing inventory levels and alerting other services of changes.
- Service 3: Payment Service: Create a Payment Service using Scala and Finagle to handle payment processing and notify the Order Service of payment success or failure. This service will be responsible for processing payments and interacting with payment gateways.
By using Scala for building these microservices, you can take advantage of its concurrency features, type safety, and concise syntax to create scalable, efficient, and maintainable services.
Conclusion
Scala's unique blend of object-oriented and functional programming principles makes it an ideal choice for backend development. By following best practices, choosing the right frameworks and tools, and leveraging Scala's features, you can build scalable, efficient, and maintainable backend systems. Whether you're building a microservices architecture or a monolithic application, Scala provides a robust ecosystem for backend development. So, get started with Scala today and unlock its full potential for your next backend project!
Additional Resources
For further learning and exploration, here are some additional resources:
- Scala Documentation: The official Scala documentation is an exhaustive resource for learning Scala.
- Scala School: Scala School is an online tutorial series that covers the basics of Scala programming.
- Scala Books: There are several excellent books on Scala programming, including "Programming in Scala" and " Scala in Action".
- Scala Communities: Join online Scala communities, such as the Scala subreddit and Scala Gitter channel, to connect with other developers and get help with any questions you may have.
By utilizing these resources and following the guidelines outlined in this article, you'll be well-equipped to tackle your next backend project with Scala. Happy coding!