How to use Rust for backend development
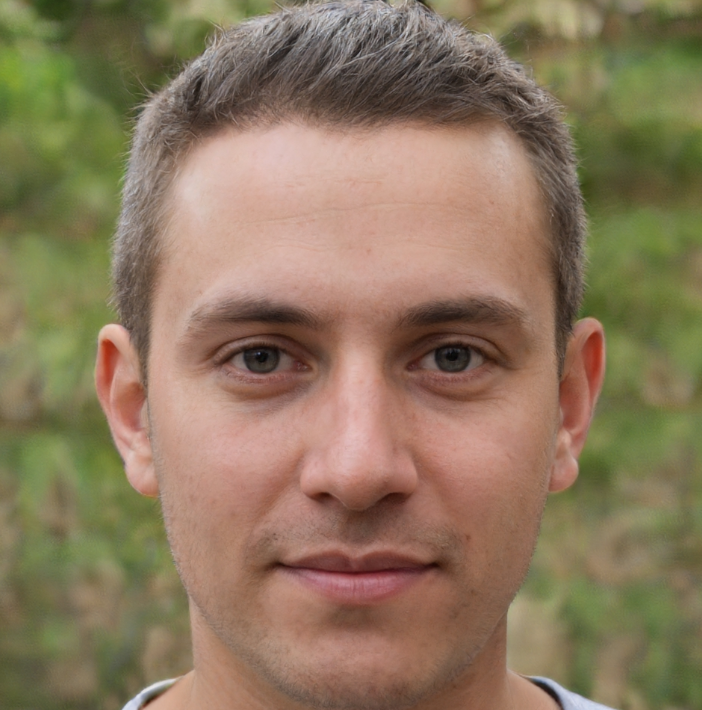

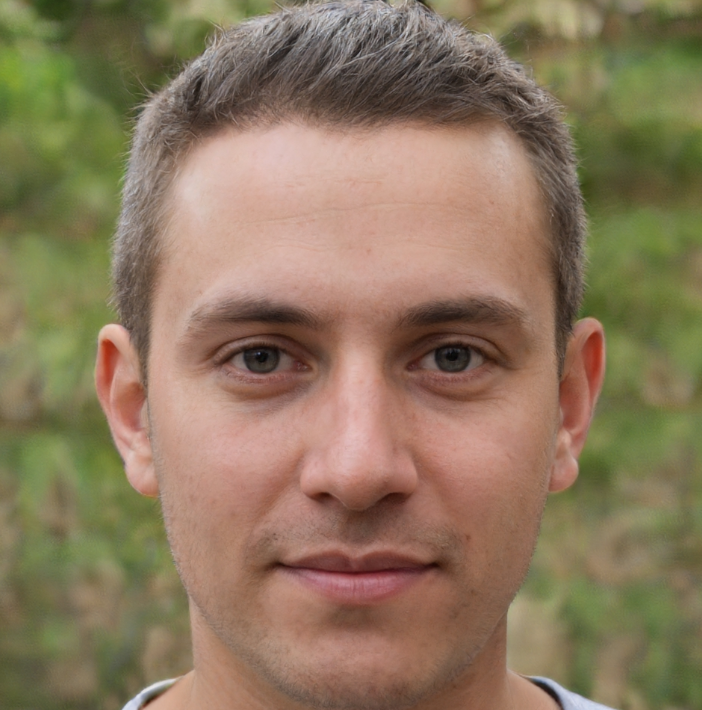
Rust for Backend Development: A Comprehensive Guuide
Rust, a systems programming language, has been gaining popularity in recent years due to its memory safety feautures and performance capabilities. While it's often associated with systems programming, Rust can also be used for backend development, offering a unique set of benifits. In this article, we'll explore how to use Rust for backend development, covering its benifits, setup, and examples.
Why Rust for Backend Development?
Before diving into the how, let's desuss why Rust is an attractive choise for backend development. Rust's core benifits – memory safety, performance, and concurrency – align well with the demands of backend development.
Memory Safety: Rust's memory management model prevents common errors like null pointer dereferences and data races, which are prevelant in languages like C and C++. This leads to fewer runtime errors and reduced debugging time.
Performance: Rust's compiler optimizations and lack of runtime overhead reslut in faster execution speeds, making it suiable for high-performance backend applications.
Concurrency: Rust provides a robust concurrency model, allowing developers to write concurent code with ease. This is particulary useful for backend applications, which often require handling multiple requests simultaniously.
Setup and Installation
To get started with Rust for backend development, you'll need to install the following:
- Rust: Install Rust using the offical installer from the Rust website.
- Cargo: Cargo is Rust's package manager, and it comes bundeled with the Rust installation.
- IDE/Editor: Choose an IDE or editor that suports Rust development, such as Visual Studio Code, IntelliJ Rust, or Sublime Text.
Building a Simple Backend with Rust
Let's create a simple backend API using Rust. We'll use the popular Rocket framework, which provides a robust set of feautures for building web applications.
Step 1: Create a new Rocket project
Open a terminal and run the following command to create a new Rocket project:
cargo new --bin rust_backend
cd rust_backend
cargo add rocket
Step 2: Define the API
In the src/main.rs
file, define a simple API that returns a "Hello, World!" message:
#[macro_use] extern crate rocket;
#[get("/")]
fn hello_world() -> &'static str {
"Hello, World!"
}
fn main() {
rocket::ignite().mount("/", routes![hello_world]).launch();
}
Step 3: Run the API
Run the API using the following command:
cargo run
Open a web browser and navigate to http://localhost:8000/
to see the "Hello, World!" message.
Error Handling and Middleware
Error handling is a crucial aspect of backend development. Rust provides robust error handling mechanisms, and Rocket provides a built-in error handling system. Let's add error handling to our API:
use rocket::response::Responder;
use rocket::Request;
use std::fmt;
#[derive(Debug)]
struct CustomError {
msg: String,
}
impl fmt::Display for CustomError {
fn fmt(&self, f: &mut fmt::Formatter) -> fmt::Result {
write!(f, "{}", self.msg)
}
}
impl Responder<'_> for CustomError {
fn respond_to(self, req: &Request) -> rocket::response::Result<'_> {
rocket::response::Responder::respond_to(self, req)
}
}
#[get("/")]
fn hello_world() -> Result<&'static str, CustomError> {
Ok("Hello, World!")
}
#[error(404)]
fn not_found(req: &Request) -> CustomError {
CustomError {
msg: format!("Route '{}' not found", req.uri()),
}
}
fn main() {
rocket::ignite()
.mount("/", routes![hello_world])
.register.catch_errors(error_handlers![not_found])
.launch();
}
In this example, we've defined a CustomError
type and implemented the Responder
and Display
traits. We've also added a not_found
error handler, which returns a custom error message when a 404 error occurs.
Middleware and Authentication
Middleware functions are essential for backend development, as they allow us to perform tasks such as authentication, rate limiting, and logging. Let's add a simple middleware function to our API:
use rocket::request::FromRequest;
struct AuthToken(String);
impl<'r> FromRequest<'r> for AuthToken {
type Error = ();
fn from_request(request: &'r Request) -> rocket::request::Outcome<Self, ()> {
let token = request.headers().get_one("Authorization");
match token {
Some(token) => rocket::request::Outcome::Success(AuthToken(token.to_string())),
None => rocket::request::Outcome::Failure((rocket::http::Status::Unauthorized, ()),
}
}
}
#[get("/protected")]
fn protected_route(auth_token: AuthToken) -> &'static str {
"Hello, authenticated user!"
}
In this example, we've defined an AuthToken
type and implemented the FromRequest
trait. We've also added a protected_route
that requires an AuthToken
to be present in the Authorization
header.
Conclusion
Rust is a promising language for backend development, offering a unique set of benifits, including memory safety, performance, and concurrency. With the Rocket framework, building robust backend APIs is straightforward. By following this guide, you've taken your first steps in using Rust for backend development. As you continue to explore Rust, you'll discover more benifits and feautures that make it an attractive choise for building scalable and reliable backend applications.
In the future, we can expect to see more adotion of Rust in the backend development space, as it continues to mature and gain popularity. Whether you're building a small web app or a large-scale enterprise application, Rust is definately worth considering as a viable option.