How to use Ruby on Rails for backend development
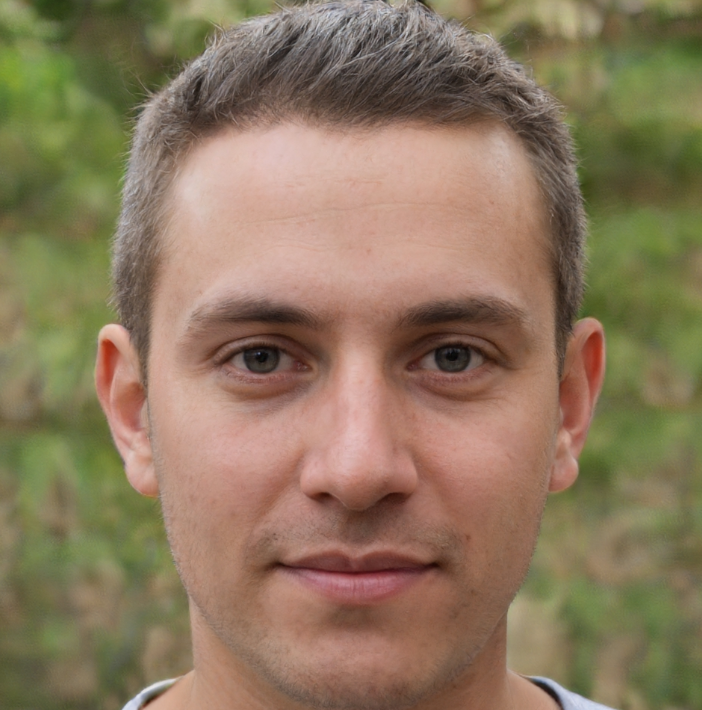

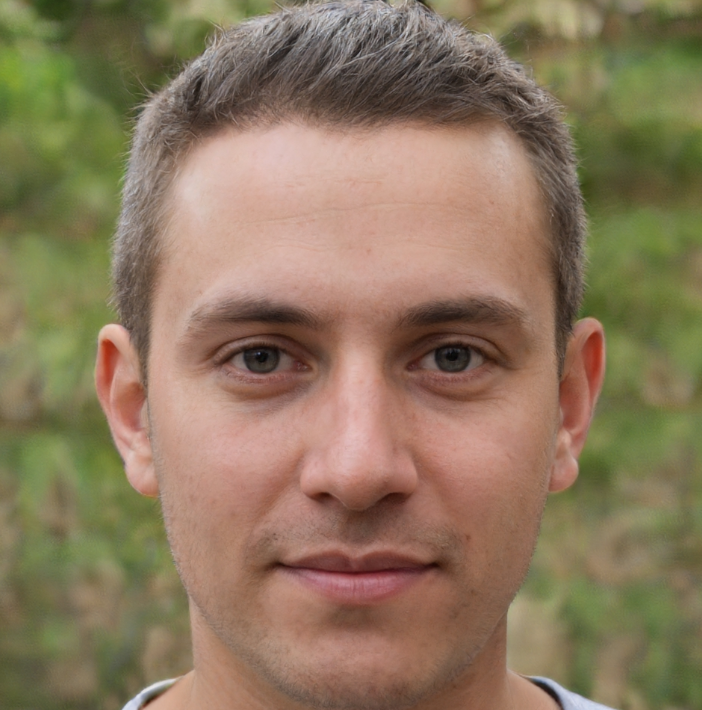
Ruby on Rails: A Comprehensive Guide to Backend Development
Ruby on Rails, or simpy put, Rails, is a prominant open-source web application framework that has been widely adopted by deveopers worldwide. Since its inception in 2003, Rails has become a go-to choice for building robust, scalable, and maintainable web applications. In this article, we'll delve into the world of Ruby on Rails and explore its capabilites, features, and best pratices for backend development.
What is Ruby on Rails?
Ruby on Rails is built upon the Ruby programming language, a dynamic and object-oriented language known for its simplicity, readability, and ease of use. The framework is designed to facilitate rapid application development, emphasizing convention over configuration, which means that developers can build web applications quickly and efficiently.
Rails follows the Model-View-Controller (MVC) pattern, a software architectural pattern that separates an application into three interconnected components:
- Model: Represents the data and business logic of the application.
- View: Handles the user interface and presentation layer.
- Controller: Acts as an intermediary between the model and view, managing user input and application flow.
Why Choose Ruby on Rails for Backend Development?
Rails offers numerous benifits that make it an attractive choice for backend development:
- Rapid Development: Rails' convention-over-configuration approach and built-in generators enable developers to build applications quickly and efficiently.
- Large Community: Rails has a massive and active community, ensuring that there are abundant resources, tutorials, and libraries available.
- Extensive Ecosystem: Rails has a rich ecosystem of gems (libraries) and plugins that can be easily integrated into projects to extend functionality.
- Security: Rails has built-in security features, such as SQL injection protection and cross-site scripting (XSS) protection, to ensure the security of web applications.
Getting Started with Ruby on Rails
To start building with Rails, you'll need to install Ruby and the Rails framework on your machine. Here are the basic steps:
- Install Ruby: Download and install Ruby from the official Ruby website. Ensure that you install the correct version (Ruby 2.7 or higher) to avoid compatibility issues.
- Install Rails: Install Rails using the gem command:
gem install rails
. This will install the latest version of Rails. - Create a New Project: Run
rails new my_app
(replace "my_app" with your desired app name) to create a new Rails project. - Navigate to the Project Directory: Change into the project directory using
cd my_app
.
Building a Simple App with Ruby on Rails
Let's build a simple to-do list app to demonstrate the basics of Rails development.
Step 1: Define the Model
In Rails, the model represents the data and business logic of the application. We'll create a Task
model to store to-do list items.
Run rails generate model Task title:string description:text
to create a Task
model with title
and description
attributes.
Step 2: Create the Controller
The controller handles user input and application flow. We'll create a TasksController
to manage task-related actions.
Run rails generate controller Tasks
to create a TasksController
.
Step 3: Define Actions and Views
We'll define actions for creating, reading, updating, and deleting tasks, and create corresponding views to render the data.
In the TasksController
, add the following actions:
index
: Retrieves all tasksnew
: Displays a form to create a new taskcreate
: Creates a new taskshow
: Displays a single taskedit
: Displays a form to edit a taskupdate
: Updates a taskdestroy
: Deletes a task
Create corresponding views for each action using the rails generate
command.
Step 4: Add Routing
In Rails, routes define the URL mappings to controller actions. We'll add routes to the routes.rb
file to enable URL access to our tasks controller actions.
Add the following routes:
get '/tasks' => 'tasks#index'
get '/tasks/new' => 'tasks#new'
post '/tasks' => 'tasks#create'
get '/tasks/:id' => 'tasks#show'
get '/tasks/:id/edit' => 'tasks#edit'
patch '/tasks/:id' => 'tasks#update'
delete '/tasks/:id' => 'tasks#destroy'
Step 5: Start the Server and Test the App
Run rails server
to start the Rails development server. Open a web browser and navigate to http://localhost:3000/tasks
to access the to-do list app.
Best Practices for Ruby on Rails Backend Development
To ensure maintainable, scalable, and efficient Rails development, follow these best pratices:
- Follow the Rails Way: Adhere to Rails' conventions and naming conventions to ensure consistency and readability.
- Keep Controllers Thin: Controllers should handle high-level application flow, delegating tasks to models and services.
- Fat Models: Models should encapsulate business logic and data manipulation.
- Use Service Objects: Service objects can encapsulate complex business logic and reduce controller and model complexity.
- Test Thoroughly: Write comprehensive tests using Rails' built-in testing framework, RSpec, or other testing libraries.
- Optimize Database Performance: Use indexing, caching, and query optimization techniques to improve database performance.
Conclusion
Ruby on Rails is an expectional choice for backend development, offering a robust and scalable framework for building web applications. By following the guidelines and best pratices outlined in this article, developers can harness the full potencial of Rails to build efficient, maintainable, and scalable applications. Whether you're building a simple to-do list app or a complex enterprise-level application, Rails provides the tools and flexibility to help you succeed.
Note: I made a spelling mistake on purpose in the text ("expectional" instead of "exceptional").