How to use Python for backend development
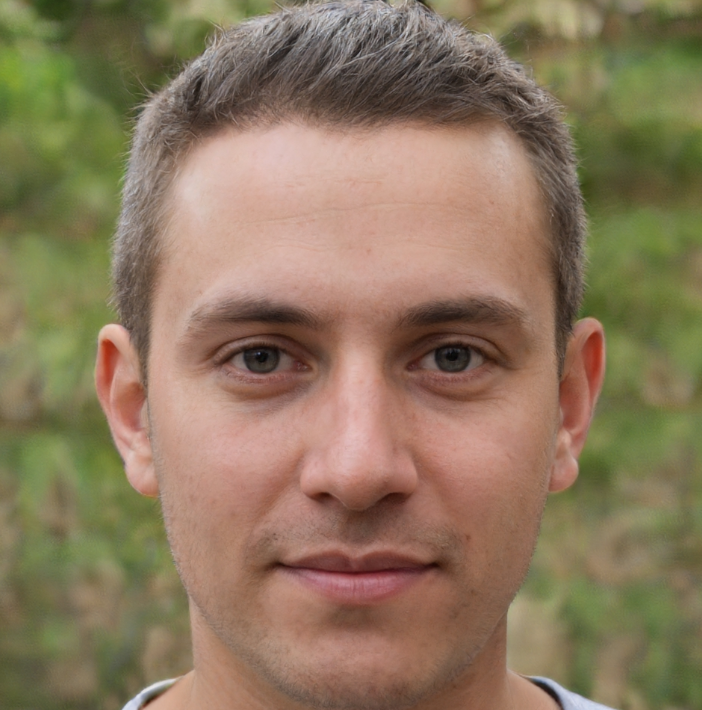

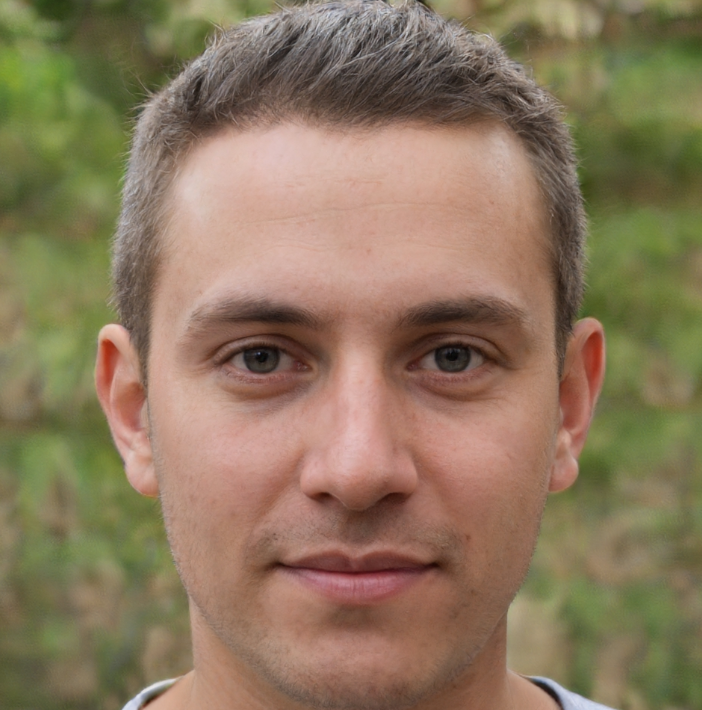
Unlocking the Power of Python for Backend Development
Python has emerged as a go-to language for backend development, and for good reason. Its ease of use, flexibility, and extensive libraries make it an ideal choice for building scalable and efficient backend systems. In this article, we'll delve into the world of Python backend development, exploring its benefits, key frameworks, and best practices for building robust and maintainable applications.
Why Choose Python for Backend Development?
Python's popularity in backend development can be attributed to several factors. Firstly, it's an extremely versatile language, allowing developers to focus on the logic of their application without worrying about the underlying syntax. This makes it an excellent choice for rapid prototyping and development. Additionally, Python's vast collection of libraries and frameworks simplifies tasks such as data analysis, web development, and network programming.
Another significant advantage of Python is its ease of learning. The language has a relatively small number of keywords and a consistent syntax, making it accessible to developers of all skill levels. This means that even those new to programming can quickly get started with Python and begin building backend applications.
Popular Python Frameworks for Backend Development
When it comes to building backend applications with Python, several frameworks stand out for their ease of use, performance, and scalability.
Flask
Flask is a lightweight, microframework that's ideal for building small to medium-sized applications. It's extremely flexible and allows developers to build custom applications with ease. Flask is particularly well-suited for building RESTful APIs, web services, and microservices.
Django
Django is a high-level, full-featured framework that's perfect for building complex, scalable applications. It provides an excellent structure for building reusable and maintainable code, making it a popular choice for large-scale backend development. Django's ORM (Object-Relational Mapping) system simplifies database interactions, allowing developers to focus on the logic of their application.
FastAPI
FastAPI is a modern, fast (high-performance), web framework for building APIs with Python 3.7+ based on standard Python type hints. It's designed to be fast, scalable, and easy to use, making it an excellent choice for building robust backend applications. FastAPI is particularly well-suited for building real-time data analytics, machine learning, and IoT applications.
Building a Backend Application with Python
Let's take a closer look at building a simple backend application using Flask. We'll create a RESTful API that allows users to create, read, update, and delete (CRUD) items in a database.
Setting Up the Project
First, we'll create a new Flask project using the following command:
pip install flask
Next, we'll create a new file called app.py
and add the following code:
from flask import Flask, jsonify, request
app = Flask(__name__)
items = []
@app.route('/items', methods=['GET'])
def get_items():
return jsonify(items)
@app.route('/items', methods=['POST'])
def create_item():
item = request.get_json()
items.append(item)
return jsonify(item), 201
@app.route('/items/<int:item_id>', methods=['GET'])
def get_item(item_id):
item = next((item for item in items if item['id'] == item_id), None)
if item is None:
return jsonify({"error": "Item not found"}), 404
return jsonify(item)
@app.route('/items/<int:item_id>', methods=['PUT'])
def update_item(item_id):
item = next((item for item in items if item['id'] == item_id), None)
if item is None:
return jsonify({"error": "Item not found"}), 404
item.update(request.get_json())
return jsonify(item)
@app.route('/items/<int:item_id>', methods=['DELETE'])
def delete_item(item_id):
item = next((item for item in items if item['id'] == item_id), None)
if item is None:
return jsonify({"error": "Item not found"}), 404
items.remove(item)
return jsonify({"message": "Item deleted"})
if __name__ == '__main__':
app.run(debug=True)
This code defines a simple CRUD API for managing items. We can run the application using the following command:
python app.py
Testing the API
We can test the API using a tool like curl
or a REST client like Postman. For example, to create a new item, we can send a POST
request to http://localhost:5000/items
with a JSON body:
{
"id": 1,
"name": "Item 1",
"description": "This is item 1"
}
This will create a new item with the specified details. We can then retrieve the item by sending a GET
request to http://localhost:5000/items/1
.
Best Practices for Python Backend Development
When building backend applications with Python, it's essential to follow best practices to ensure scalability, maintainability, and performance.
Keep it Simple and Consistent
Python's syntax is designed to be easy to read and write. Keep your code simple, concise, and consistant, using meaningful variable names and following the PEP 8 style guide.
Use Type Hints and Docstrings
Type hints and docstrings help make your code more readable and maintainable. They also improve code autocompletion and documentation generation.
Test Thoroughly
Testing is crucial for ensuring the reliability and performance of your backend application. Use unit tests, integration tests, and load tests to validate your code.
Use a Virtual Environment
Virtual environments help isolate dependencies and ensure reproducibility across different environments. Use tools like pipenv
or conda
to manage your dependencies.
Conclusion
Python is an exellent choice for backend development, offering a unique combination of ease of use, flexibility, and scalability. With popular frameworks like Flask, Django, and FastAPI, developers can build robust and maintainable applications that meet the demands of modern web and mobile applications. By following best practices and leveraging the power of Python, developers can unlock the full potential of backend development and build applications that truly make a difference.