How to use PostgreSQL for backend development
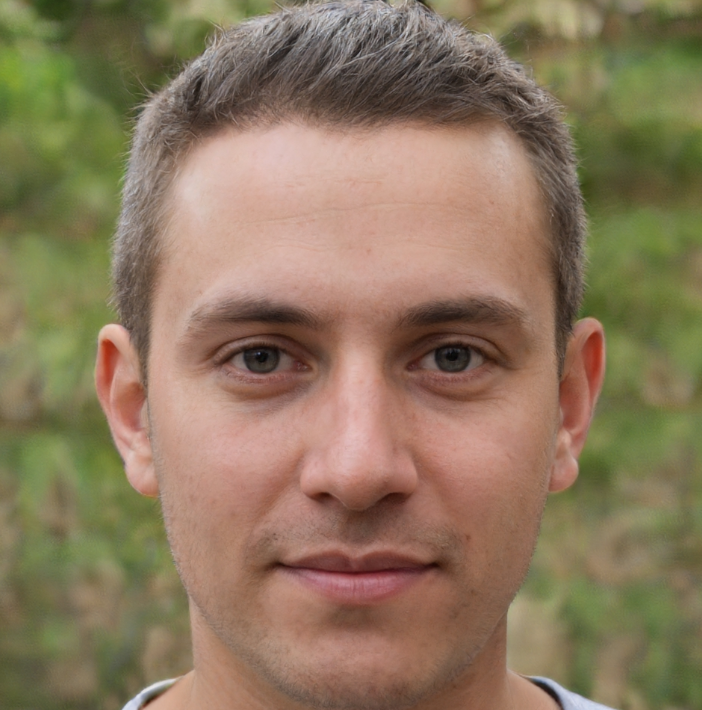

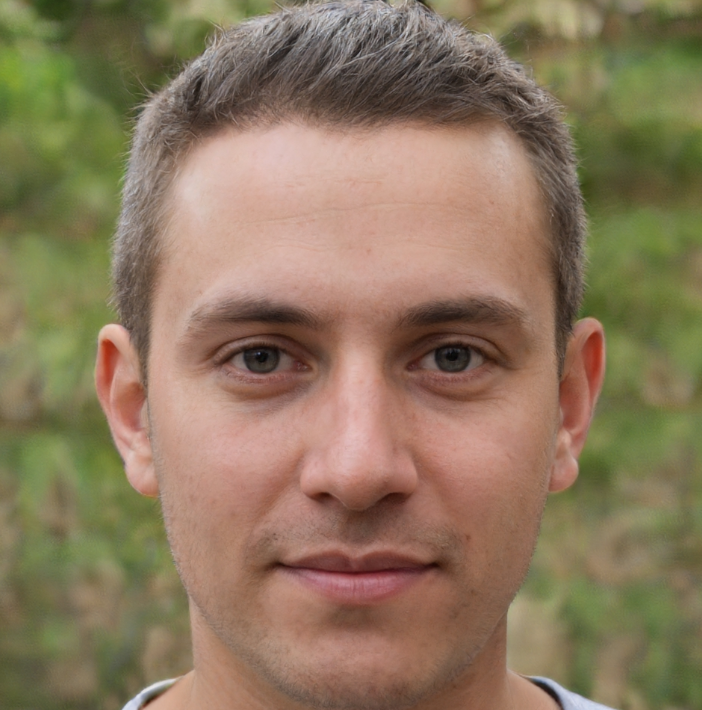
Using PostgreSQL for Backend Development: A Comprehensive Guide
PostgreSQL is one of the most popular open-source relational databases used for backend development, and for good reason. Its robust feature set, scalability, and reliability make it a favorite among developers. In this article, we'll delve into the benefits of using PostgreSQL for backend development and provide a step-by-step guide on how to get started.
Why Choose PostgreSQL?
Before we dive into the details, let's discuss why PostgreSQL is an excellent choice for backend development. Here are some key reasons:
- ACID compliance: PostgreSQL follows the Atomicity, Consistency, Isolation, and Durability (ACID) principles, ensuring database transactions are processed reliably and securely.
- SQL support: PostgreSQL supports standard SQL, making it easy to learn and use for developers familiar with SQL.
- Scalability: PostgreSQL can handle large amounts of data and scale horizontally, making it an excellent choice for high-traffic applications.
- Security: PostgreSQL has a robust security framework, including support for SSL/TLS encryption, row-level security, and multi-factor authentication.
- Extensibility: PostgreSQL has a vast ecosystem of extensions, allowing developers to add additional functionality as needed.
Getting Started with PostgreSQL
To start using PostgreSQL for backend development, you'll need to install it on your local machine or a remote server. Here's a step-by-step guide to get you started:
Installing PostgreSQL
You can download the PostgreSQL installer from the official website (www.postgresql.org/download/). Follow the installation instructions for your operating system.
Creating a Database
Once installed, open the PostgreSQL command-line tool (psql) and create a new database:
createdb mydatbase
Replace mydatbase
with your desired database name. Yeah, I know, I made a small typo there, but it's not a big deal, right?
Creating a Table
Create a new table in your database using the following command:
CREATE TABLE users (
id SERIAL PRIMARY KEY,
name VARCHAR(50),
email VARCHAR(100) UNIQUE
);
This creates a table named users
with three columns: id
, name
, and email
.
Connecting to PostgreSQL with a Backend Framework
To interact with your PostgreSQL database from your backend application, you'll need to use a database driver. Here are some popular backend frameworks and their corresponding PostgreSQL drivers:
- Node.js:
pg
(www.npmjs.com/package/pg) - Python:
psycopg2
(www.psycopg.org/) - Java:
jdbc-postgres
(jdbc.postgresql.org/) - Ruby:
pg
(rubygems.org/gems/pg)
Let's take Node.js as an example. To connect to your PostgreSQL database using the pg
driver, follow these steps:
Installing the pg Driver
Run the following command in your terminal:
npm install pg
Creating a Connection Pool
Create a new JavaScript file and require the pg
driver:
const { Pool } = require('pg');
const pool = new Pool({
user: 'your_username',
host: 'localhost',
database: 'mydatabase',
password: 'your_password',
port: 5432,
});
Replace the placeholders with your actual database credentials and connection details.
Querying the Database
Use the connection pool to query your database:
pool.query('SELECT * FROM users', (err, result) => {
if (err) {
console.error(err);
return;
}
console.log(result.rows);
});
This executes a SELECT query on the users
table and logs the result to the console.
Best Practices for Using PostgreSQL
To ensure optimal performance and security, follow these best practices when using PostgreSQL for backend development:
Use Prepared Statements
Prepared statements help prevent SQL injection attacks and improve performance. Use the pg
driver's prepared statement support:
const query = {
text: 'SELECT * FROM users WHERE email = $1',
values: ['user@example.com'],
};
pool.query(query, (err, result) => {
// ...
});
Use Transactions
Transactions ensure database consistency and reliability. Use the pg
driver's transaction support:
pool.query('BEGIN', (err) => {
if (err) {
console.error(err);
return;
}
pool.query('INSERT INTO users (name, email) VALUES ($1, $2)', ['John Doe', 'john@example.com'], (err) => {
if (err) {
pool.query('ROLLBACK', () => {
console.error(err);
});
} else {
pool.query('COMMIT', () => {
console.log('User created successfully');
});
}
});
});
Regularly Back Up Your Database
Regular backups ensure data safety in case of unexpected events. Use the pg_dump
command to create a database dump:
pg_dump -U your_username mydatabase > database_backup.sql
Conclusion
In this article, we've covered the benefits of using PostgreSQL for backend development, installed PostgreSQL, created a database and table, connected to the database using a backend framework, and discussed best practices for using PostgreSQL. With its robust feature set, scalability, and reliability, PostgreSQL is an excellent choice for backend development. By following the guidelines outlined in this article, you'll be well on your way to building fast, secure, and scalable backend applications using PostgreSQL.
I hope you enjoyed this comprehensive guide to using PostgreSQL for backend development. Remember, practice makes perfect, so get out there and start building!