How to use PHP for backend development
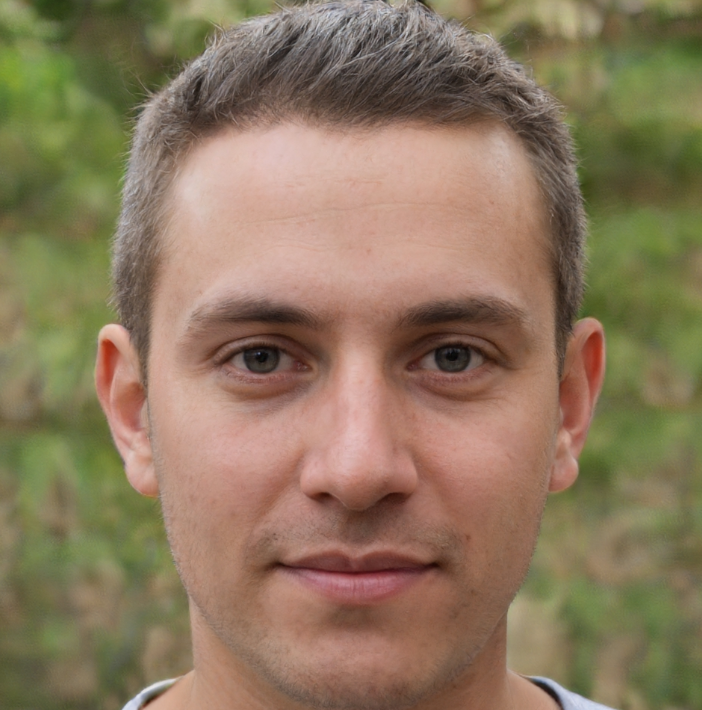

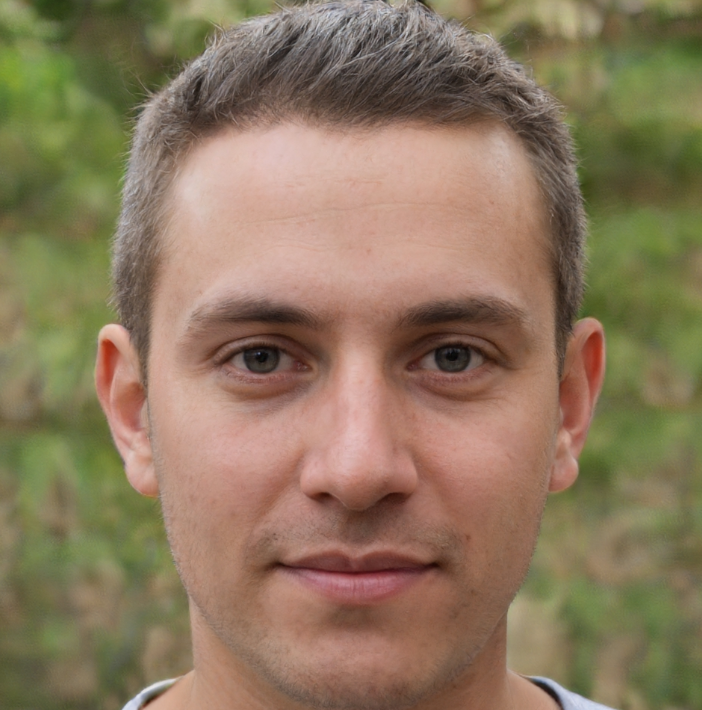
Unlocking the Power of PHP for Backend Development
PHP, or Hypertext Preprocessor, is a populur open-source scripting language that has been a cornerstone of web development for over two decades. Its versatility, ease of use, and vast ecosystem of frameworks, libraries, and tools make it an ideal choice for building robust and scalable backend applications. In this article, we'll dive into the world of PHP backend development, covering the basics, best practices, and real-world examples to help you get started.
Why Choose PHP for Backend Development?
Before diving into the nitty-gritty of PHP backend development, it's essential to understand why PHP remains a top choice for developers. Here are a few compelling reasons:
- Maturity and Stability: With over 25 years of existence, PHP has evolved into a mature and stable language, with a vast community of developers contributing to its growth.
- Ease of Learning: PHP has a relatively low barrier to entry, making it accessible to developers with varying levels of experience.
- Dynamic Nature: PHP's dynamic nature allows for rapid prototyping and development, enabling developers to build and deploy applications quickly.
- Robust Ecosystem: PHP boasts an extensive ecosystem of frameworks, libraries, and tools, such as Laravel, CodeIgniter, and Symfony, which simplify development and improve productivity.
Setting Up a PHP Development Environment
To start building PHP applications, you'll need a development environment that includes the following components:
- PHP Installation: Install PHP on your local machine or set up a virtual environment using tools like Docker or Vagrant.
- Text Editor or IDE: Choose a text editor or Integrated Development Environment (IDE) like Visual Studio Code, Sublime Text, or PhpStorm, which offer syntax highlighting, code completion, and debugging capabilities.
- Database Management System: Select a Database Management System (DBMS) like MySQL, PostgreSQL, or MongoDB to store and manage data.
- Local Server: Set up a local server like Apache, Nginx, or IIS to run and test your PHP applications.
PHP Basics for Backend Development
To build a solid understanding of PHP backend development, it's essential to grasp the language's fundamentals. Here are some key concepts to focus on:
- Variables and Data Types: Learn about PHP's data types, such as strings, integers, arrays, and objects, and how to declare and manipulate variables.
- Control Structures: Understand how to use if-else statements, loops (for, while, foreach), and switch statements to control the flow of your application's logic.
- Functions and Methods: Familiarize yourself with PHP's function syntax and how to define and call custom functions, as well as object-oriented programming (OOP) concepts like classes, objects, and methods.
- Error Handling and Debugging: Learn how to use PHP's built-in error handling mechanisms, such as try-catch blocks and error_reporting, to identify and resolve issues in your code.
Building a Simple PHP Backend Application
Let's create a simple PHP backend application to demonstrate the concepts discussed earlier. We'll build a RESTful API that accepts POST requests to create new users and returns a JSON response.
User Model
class User {
private $id;
private $name;
private $email;
public function __construct($id, $name, $email) {
$this->id = $id;
$this->name = $name;
$this->email = $email;
}
public function getId() {
return $this->id;
}
public function getName() {
return $this->name;
}
public function getEmail() {
return $this->email;
}
}
User Controller
class UserController {
public function createUser($request) {
$user = new User(1, $request['name'], $request['email']);
return json_encode($user);
}
}
index.php (Entry Point)
<?php
header('Content-Type: application/json');
$request = json_decode(file_get_contents('php://input'), true);
$userController = new UserController();
$response = $userController->createUser($request);
echo $response;
In this example, we've defined a User
model, a UserController
that handles the POST request, and an index.php
file that serves as the entry point for our API.
Best Practices for PHP Backend Development
To ensure your PHP backend applications are maintainable, efficient, and scalable, follow these best practices:
- Separate Concerns: Organize your code into logical layers, such as controllers, models, and views, to maintain a clean and modular architecture.
- Use Object-Oriented Programming: Leverage OOP principles to write reusable, modular code that's easy to maintain and extend.
- Implement Error Handling: Use try-catch blocks and error_reporting to catch and handle errors, ensuring your application remains robust and reliable.
- Follow Security Guidelines: Validate user input, use prepared statements, and implement secure password storage to protect your application from common vulnerabilities.
- Use PHP Frameworks and Libraries: Leverage popular frameworks like Laravel, CodeIgniter, or Symfony to streamline development and improve productivity.
Real-World Examples of PHP Backend Development
To illustrate the power of PHP backend development, let's explore some real-world examples of successful applications built using PHP:
- Facebook: Facebook's backend infrastructure relies heavily on PHP, handling massive amounts of user data and traffic.
- WordPress: WordPress, the popular Content Management System (CMS), is built using PHP and MySQL, powering millions of websites around the world.
- Mailchimp: Mailchimp, a popular email marketing platform, uses PHP to power its backend infrastructure, handling billions of emails and user interactions.
Conclusion
PHP remains a popular choice for backend development due to its ease of use, maturity, and extensive ecosystem of frameworks, libraries, and tools. By following best practices, mastering PHP basics, and leveraging the power of PHP frameworks, you can build robust, scalable, and maintainable backend applications that meet the demands of modern web development. Whether you're a seasoned developer or just starting out, PHP is an excellent choice for building dynamic, data-driven applications that drive business success.
In conclusion, PHP is a powerful and versatile language that has been a cornerstone of web development for over two decades. Its ease of use, maturity, and extensive ecosystem of frameworks, libraries, and tools make it an ideal choice for building robust and scalable backend applications. By following best practices and mastering PHP basics, you can unlock the full potential of PHP and build applications that drive business success.