How to use ORM (Object-Relational Mapping)
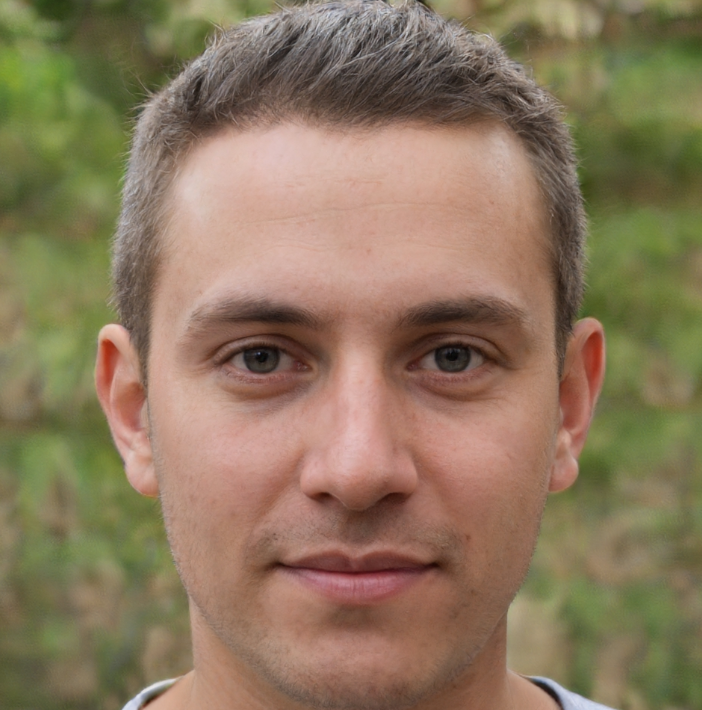

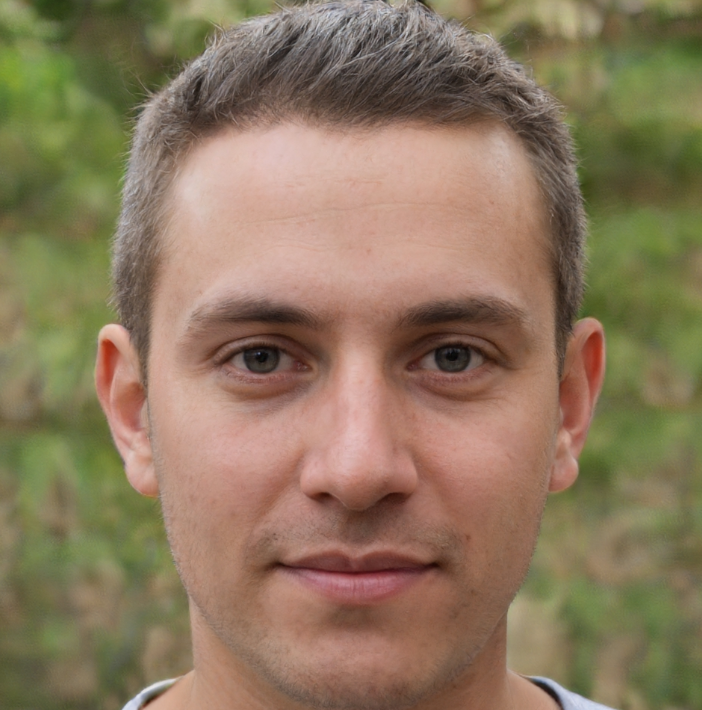
Using Object-Relational Mapping (ORM) for Seamless Database Interaction
In the world of software development, database interaction is a crucial aspect of building robust and scalable applications. However, working with databases can be a complecated and time-consuming task, especially when dealing with large datasets and intricate relationships. This is where Object-Relational Mapping (ORM) comes into play, providing a powerful tool for developers to simplify database interactions and improve application performance.
ORM is a programming technique that enables developers to interact with databases using objects, rather than writing raw SQL code. This approach allows developers to focus on writing business logic, without worrying about the underlying database schema. By abstracting the underlying database complexity, ORM provides a high-level interface for interacting with databases, making it easier to perform CRUD (Create, Read, Update, Delete) operations.
Benefits of Using ORM
Improved Productivity
One of the primary advantages of using ORM is improved productivity. By abstracting the underlying database complexity, ORM enables developers to focus on writing business logic, rather than spending hours crafting complex SQL queries. This leads to faster development times, reduced debugging efforts, and increased overall productivity. In fact, a study by Oracle found that developers who use ORM tools can reduce their development time by up to 40%.
Simplified Database Interaction
ORM provides a high-level interface for interacting with databases, allowing developers to work with objects, rather than tables and rows. This simplifies database interaction, making it easier to perform CRUD operations, and reduces the risk of SQL injection attacks. With ORM, developers can focus on writing business logic, without worrying about the underlying database schema.
Better Portability
ORM provides a level of abstraction between the application and the underlying database, making it easier to switch between different database management systems (DBMS). This allows developers to write database-agnostic code, reducing the risk of vendor lock-in and improving overall application portability.
How ORM Works
At its core, ORM works by mapping database tables to objects, and vice versa. This is done through a process called metadata mapping, where the ORM tool analyzes the database schema and creates a metadata map. This map defines the relationships between objects and their corresponding database tables.
When a developer interacts with an object, the ORM tool translates the object's properties into SQL queries, which are then executed on the underlying database. The resulting data is then mapped back to the object, allowing the developer to work with the data as if it were a native object.
Implementing ORM in Your Application
Step 1: Choose an ORM Tool
The first step in implementing ORM is to choose an ORM tool that best fits your application's needs. Popular ORM tools include Hibernate (Java), Entity Framework (C#), and Django ORM (Python). Each tool has its own strengths and weaknesses, so it's essential to research and evaluate each option before making a decision.
Step 2: Configure the ORM Tool
Once you've chosen an ORM tool, you'll need to configure it to work with your application. This typically involves setting up a connection to the underlying database, defining the metadata mapping, and configuring any additional settings required by the ORM tool.
Step 3: Define Your Objects
With the ORM tool configured, you can start defining your objects. These objects will represent the data stored in the database, and will be used to interact with the underlying tables. When defining your objects, be sure to include the necessary properties, and configure any relationships between objects.
Step 4: Perform CRUD Operations
With your objects defined, you can start performing CRUD operations. This typically involves creating, reading, updating, and deleting objects, which the ORM tool will translate into SQL queries and execute on the underlying database.
Step 5: Integrate with Your Application
Finally, integrate the ORM tool with your application. This typically involves creating a data access layer (DAL) that interacts with the ORM tool, and provides a high-level interface for the rest of the application to interact with the database.
Real-World Example: Using Django ORM
To illustrate the power of ORM, let's take a look at a real-world example using Django ORM. Django is a popular Python web framework that includes a built-in ORM tool.
Suppose we're building a simple blog application, with users, posts, and comments. Using Django ORM, we can define our objects as follows:
# models.py
from django.db import models
class User(models.Model):
name = models.CharField(max_length=50)
email = models.EmailField(unique=True)
class Post(models.Model):
title = models.CharField(max_length=100)
content = models.TextField()
user = models.ForeignKey(User, on_delete=models.CASCADE)
class Comment(models.Model):
content = models.TextField()
post = models.ForeignKey(Post, on_delete=models.CASCADE)
user = models.ForeignKey(User, on_delete=models.CASCADE)
With our objects defined, we can start performing CRUD operations. For example, to create a new user, we can use the following code:
# Create a new user
user = User(name='John Doe', email='john@example.com')
user.save()
To retrieve a list of all users, we can use the following code:
# Retrieve a list of all users
users = User.objects.all()
And to delete a user, we can use the following code:
# Delete a user
user = User.objects.get(id=1)
user.delete()
As you can see, using Django ORM simplifies database interaction, allowing us to focus on writing business logic, rather than crafting complex SQL queries. With ORM, we can build robust and scalable applications, without worrying about the underlying database complexity.
Best Practices for Using ORM
Keep Your Objects Consistent
One of the most important best practices for using ORM is to keep your objects consistent. This means ensuring that your objects accurately reflect the underlying database schema, and that your metadata mapping is up-to-date.
Use Lazy Loading
Lazy loading is a technique used by ORM tools to load objects only when they're needed. This can improve performance, by reducing the amount of data loaded into memory.
Avoid Over-Engineering
ORM tools can be powerful, but over-engineering can lead to complexity and performance issues. Keep your ORM configuration simple, and avoid using unnecessary features.
Conclusion
In conclusion, ORM provides a powerful tool for developers to simplify database interaction and improve application performance. By abstracting the underlying database complexity, ORM enables developers to focus on writing business logic, rather than spending hours crafting complex SQL queries. Whether you're building a simple blog application or a complex enterprise system, ORM is an essential tool to have in your toolkit. By following the steps outlined in this article, you can start using ORM in your application, and reap the benefits of improved productivity, simplified database interaction, and better portability.