How to use Laravel for backend development
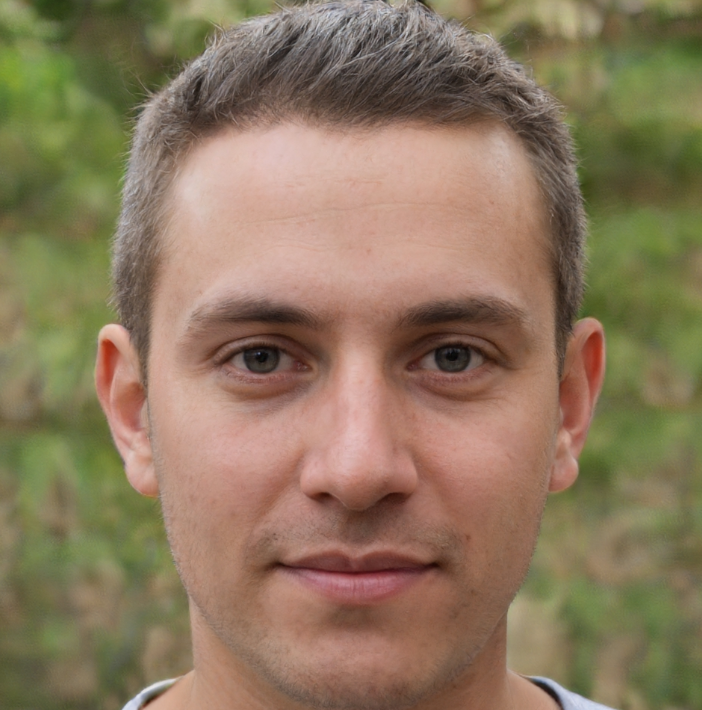

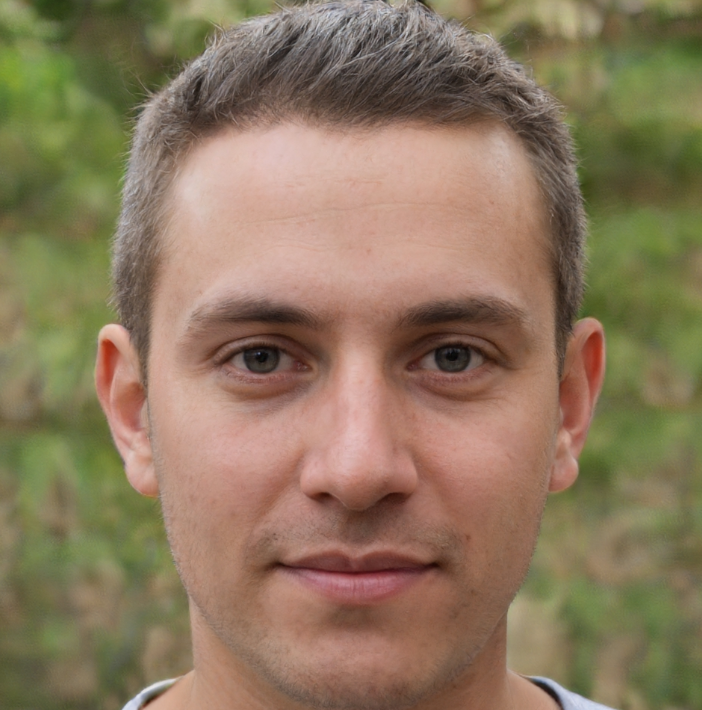
Mastering Laravel for Backend Development: A Comprehensive Guide
Laravel, the open-source PHP framework, has truely revolushunized the world of backend development. With its elegant syntax, robust features, and extensive community support, Laravel has become a top chioce among web developers. In this article, we'll delve into the world of Laravel, exploring its key features, and providing a step-by-step guide on how to use Laravel for backend development.
Getting Started with Laravel
Before we dive into the nitty-gritty, let's cover the basics. Laravel is built on top of the Model-View-Controller (MVC) architecture, which seperates an application into three interconnected components. The Model represents the data, the View handles the user interface, and the Controller processes user input. This seperation of concerns makes it easier to maintain, update, and scale your application.
To get started with Laravel, you'll need to install Composer, a PHP package manager. Once installed, run the command composer create-project --prefer-dist laravel/laravel project-name
to create a new Laravel project. Make sure you have a stable internet connection, as this process may take a few minutes.
Routing and Controllers
In Laravel, routes are used to map URLs to controller actions. The routes/web.php
file contains all the routes for your application. For example, the following code defines a route for the homepage:
Route::get('/', 'HomeController@index');
This route sends a GET request to the index
method of the HomeController
controller. Note that you can also use route groups, middleware, and route model binding to further customize your routes.
Controllers are responsible for handling HTTP requests and returning responses. You can create a new controller using the command php artisan make:controller HomeController
. The HomeController
class should extend the Controller
class:
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class HomeController extends Controller
{
public function index()
{
return view('welcome');
}
}
In this example, the index
method returns a view named welcome
. You can customize this view by creating a new file in the resources/views
directory.
Eloquent ORM and Database Integration
Eloquent, Laravel's Object-Relational Mapping (ORM) system, provides a simple and intuitive way to interact with your database. Eloquent models represent database tables, and you can create a new model using the command php artisan make:model User
.
The User
model should extend the Model
class:
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class User extends Model
{
protected $fillable = ['name', 'email', 'password'];
}
In this example, the User
model has three attributes: name
, email
, and password
. The fillable
property specifies which attributes can be mass-assigned. You can also define relationships, scopes, and accessors using Eloquent's built-in features.
To integrate with a database, you'll need to configure your database settings in the .env
file. Laravel supports various databases, including MySQL, PostgreSQL, and SQLite. Make sure to update your database credentials and settings according to your environment.
Blade Templating and Views
Blade, Laravel's templating engine, allows you to separate your application logic from your presentation layer. Views are stored in the resources/views
directory, and you can create a new view using the command php artisan make:view welcome
.
The welcome
view can contain HTML, CSS, and JavaScript code, along with PHP syntax using the @
symbol:
<!DOCTYPE html>
<html>
<head>
<title>Welcome to Laravel</title>
</head>
<body>
<h1>Welcome, {{ $name }}!</h1>
</body>
</html>
In this example, the welcome
view displays a greeting with the user's name, passed as a variable from the controller. You can customize this view by adding more HTML elements, CSS styles, and JavaScript code.
Security and Authentication
Laravel provides a robust authentication system out of the box. You can create a new authentication system using the command php artisan make:auth
.
Laravel's authentication system uses middleware to protect routes. Middleware can be used to validate user input, check for authentication, and handle CSRF protection. You can customize this system by creating custom middleware, authentication guards, and password brokers.
To use Laravel's built-in authentication system, you'll need to configure your database settings and create a users
table with the required columns. This table should include columns for the user's name, email, password, and other relevant information.
Caching and Session Management
Laravel provides a built-in caching system, which can be configured using the config/cache.php
file. You can cache frequently accessed data using the Cache
facade:
use Illuminate\Support\Facades\Cache;
Cache::put('key', 'value', 60); // Cache for 60 minutes
Laravel also provides a built-in session management system, which can be configured using the config/session.php
file. You can store and retrieve session data using the Session
facade:
use Illuminate\Support\Facades\Session;
Session::put('key', 'value');
$value = Session::get('key');
You can customize this system by creating custom cache drivers, session handlers, and cookie managers.
Testing and Debugging
Laravel provides a built-in testing framework, which can be used to write unit tests and feature tests. You can create a new test using the command php artisan make:test UserTest
.
Laravel also provides a built-in debugger, which can be used to debug your application. You can use the dd
function to dump and die, or the dump
function to dump and continue.
Conclusion
Laravel is a powerful and feature-rich PHP framework, making it an ideal choice for backend development. With its robust routing system, Eloquent ORM, and Blade templating engine, Laravel provides a comprehensive solution for building scalable and maintainable web applications. By following this guide, you'll be able to get started with Laravel and build your own backend applications.
Remember to explore Laravel's extensive documentation and community resources to stay up-to-date with the latest features and best practices. Happy coding!