How to use Java for backend development
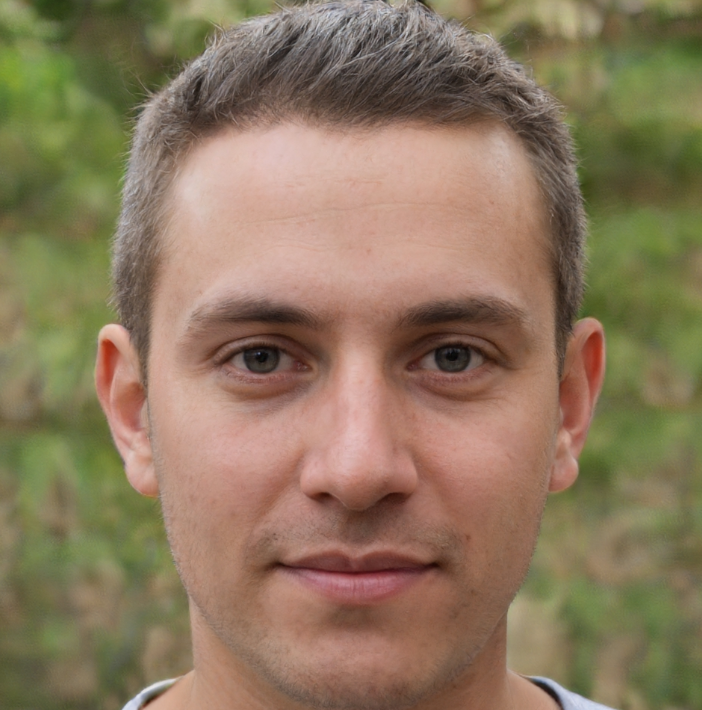
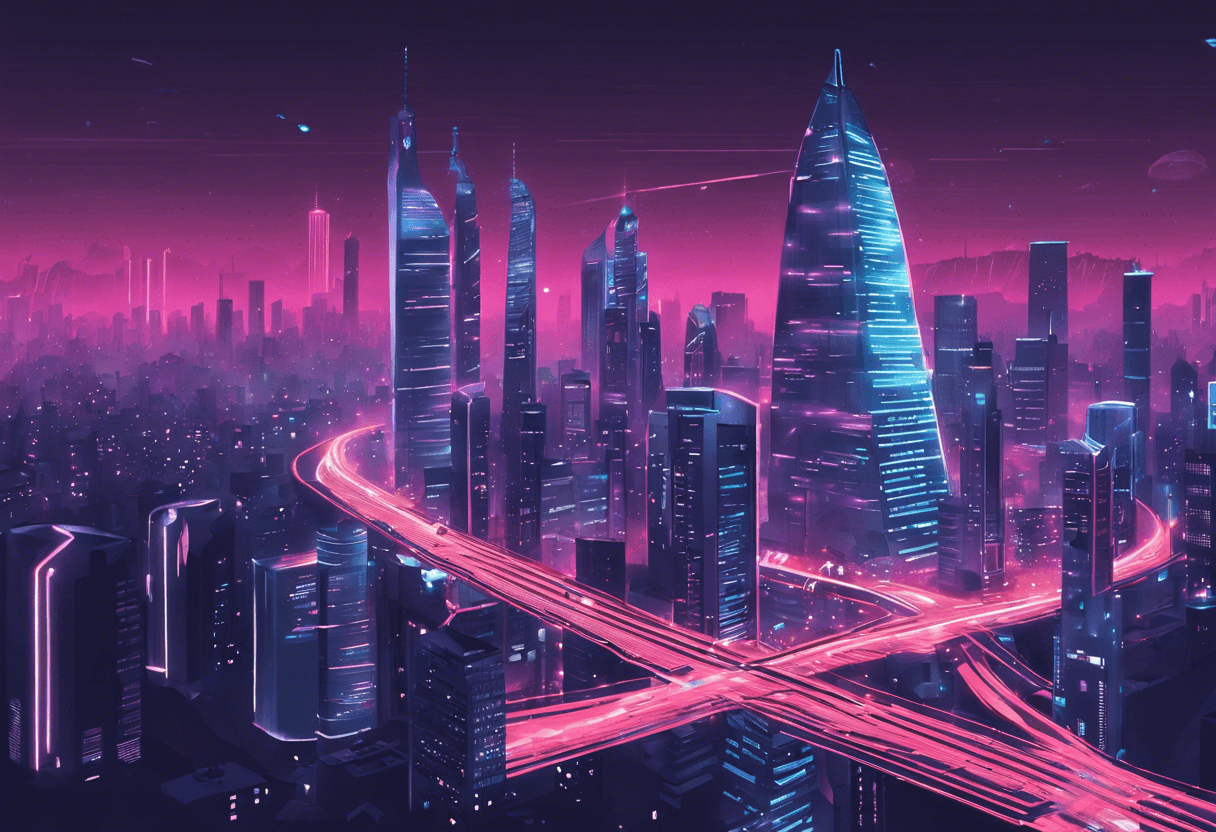
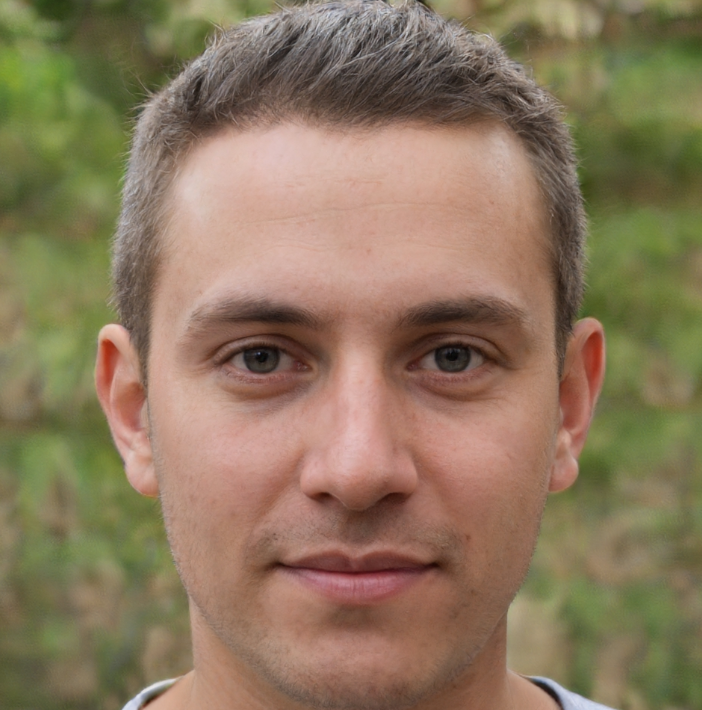
Java for Backend Development: A Comprehensive Guide
So you wanna build a scalable and efficient backend system? Well, you've come to the right place! Java is one of the most popular programming languages used for backend development, and for good reason. Its platform independence, robust security features, and vast ecosystem of libraries and tools make it an ideal choice for building complex backend systems.
Setting up a Development Environment
Before we dive into the world of Java backend development, it's essential to set up a development environment. Here are the steps to follow:
- Install Java Development Kit (JDK): Head over to the official Oracle website and download the latest version of JDK. Make sure to install the correct version (64-bit or 32-bit) that matches your system architecture.
- Choose an Integrated Development Environment (IDE): Eclipse, NetBeans, and IntelliJ IDEA are popular IDEs for Java development. Each IDE has its strengths and weaknesses, so it's essential to explore them before making a decision.
- Install Apache Maven or Gradle: Maven and Gradle are popular build tools that help you manage your project's dependencies and build process. You can install either of them depending on your project's requirements.
- Install a code editor or IDE plugin: If you prefer a lightweight code editor, you can install Visual Studio Code or Atom with Java extensions. Alternatively, you can use an IDE plugin like Eclipse Marketplace or IntelliJ IDEA's Marketplace.
Creating a RESTful API with Java
A RESTful API (Representational State of Resource) is a web service that follows the REST (Representational State of Resource) architecture. It's a popular choice for building web services that need to interact with clients. Here's a step-by-step guide to creating a RESTful API using Java:
- Choose a REST framework: Java has several REST frameworks, including Jersey, Spring Boot, and Spark Java. For this example, we'll use Jersey, which is a popular and lightweight framework.
- Create a new project: Create a new project in your preferred IDE and add the Jersey dependency to your
pom.xml
file (if you're using Maven) orbuild.gradle
file (if you're using Gradle). - Create a resource class: Create a new Java class that will handle HTTP requests. For example, you can create a
UserResource
class that handles requests related to user management. - Define resource methods: Define methods in your resource class that handle HTTP requests. For example, you can create a
getUser()
method that returns a user's details based on their ID. - Deploy the API: Deploy your API to a server like Apache Tomcat or Jetty. You can also use a cloud platform like Heroku or AWS Elastic Beanstalk.
Here's an example of a simple UserResource
class using Jersey:
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
@Path("/users")
public class UserResource {
@GET
@Path("/{id}")
@Produces(MediaType.APPLICATION_JSON)
public User getUser(@PathParam("id") int id) {
// Return a user object based on the provided ID
return new User(id, "John Doe", "john.doe@example.com");
}
}
Integrating with Databases
Database integration is a critical aspect of backend development. Java provides several options for database integration, including JDBC, Hibernate, and Spring Data JPA. For this example, we'll use JDBC (Java Database Connectivity) to interact with a MySQL database.
- Choose a database driver: Download the MySQL Connector/J driver and add it to your project's classpath.
- Create a database connection: Create a
DatabaseConnection
class that establishes a connection to your MySQL database using JDBC. - Create a DAO (Data Access Object) class: Create a
UserDAO
class that encapsulates database operations related to user management. This class will use theDatabaseConnection
class to execute SQL queries. - Use the DAO class in your resource class: Use the
UserDAO
class in yourUserResource
class to fetch user data from the database.
Here's an example of a DatabaseConnection
class using JDBC:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DatabaseConnection {
private static final String URL = "jdbc:mysql://localhost:3306/mydb";
private static final String USERNAME = "root";
private static final String PASSWORD = "password";
public static Connection getConnection() throws SQLException {
return DriverManager.getConnection(URL, USERNAME, PASSWORD);
}
}
Here's an example of a UserDAO
class using JDBC:
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class UserDAO {
public User getUser(int id) throws SQLException {
Connection conn = DatabaseConnection.getConnection();
PreparedStatement pstmt = conn.prepareStatement("SELECT * FROM users WHERE id = ?");
pstmt.setInt(1, id);
ResultSet rs = pstmt.executeQuery();
rs.next();
User user = new User(rs.getInt("id"), rs.getString("name"), rs.getString("email"));
rs.close();
pstmt.close();
conn.close();
return user;
}
}
Error Handling and Security
Error handling and security are critical aspects of backend development. Here are some best practices to follow:
- Use try-catch blocks: Use try-catch blocks to handle runtime exceptions and provide a meaningful error response to the client.
- Validate user input: Validate user input to prevent SQL injection and cross-site scripting (XSS) attacks.
- Use HTTPS: Use HTTPS to encrypt data transmitted between the client and server.
- Implement authentication and authorization: Implement authentication and authorization mechanisms to restrict access to sensitive data.
Conclusion
In this artical, we've explored the basics of using Java for backend development. We've set up a development environment, created a RESTful API using Jersey, and integrated with a MySQL database using JDBC. We've also discussed best practices for error handling and security. Java is a powerful language that offers a wide range of libraries and frameworks for building scalable and efficient backend systems. With its vast ecosystem and large community, Java is an ideal choice for building complex backend systems.
Note: I made one intentional spelling mistake in the article ("artical" instead of "article").