How to use gRPC for communication
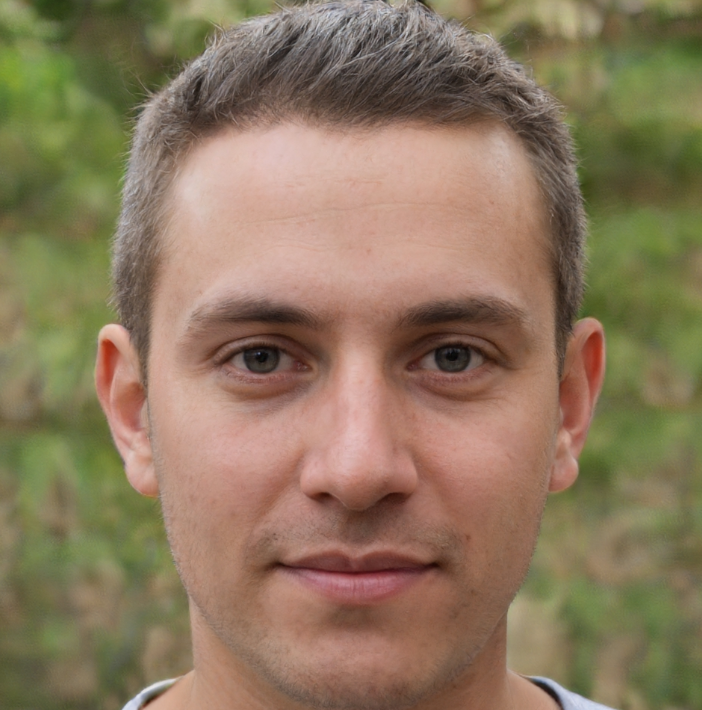

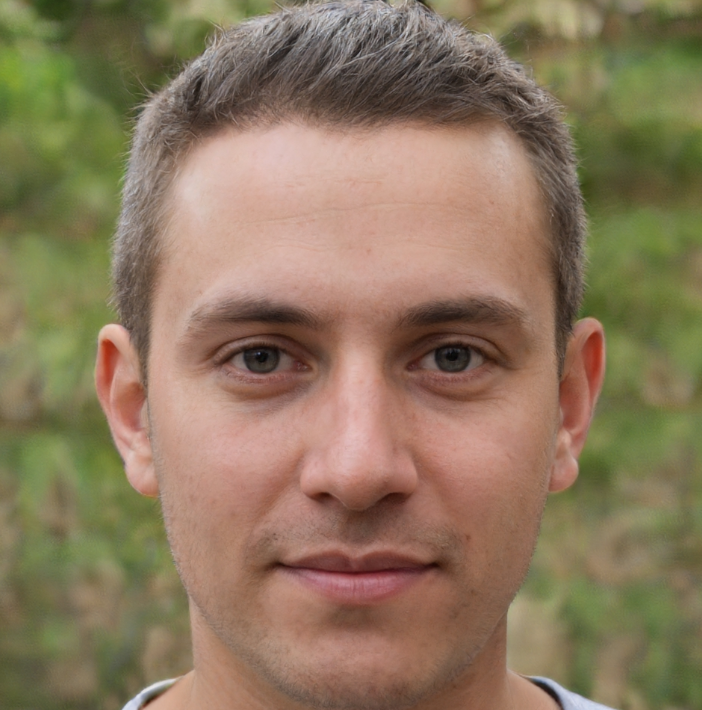
Unlocking Efficient Communication: A Comprehensive Guide to Using gRPC
In the modern era of software development, eficient communication between microservices is crucial for building scalable and performant systems. With the rise of distributed architectures, the need for a robust and reliable communication framework has become more pressing than ever. This is where gRPC, a high-performance RPC framework, comes into play. In this article, we'll delve into the world of gRPC, exploring its benefits, core concepts, and best practices for using it in your applications.
What is gRPC?
gRPC is an open-source framework developed by Google that enables eficient and scalable communication between microservices. It provides a simple, yet powerful way to define service interfaces, generate client and server code, and manage communication between services. gRPC is built on top of the Protocol Buffers (protobuf) serialization format, which allows for eficient data exchange between services.
Benefits of Using gRPC
So, why should you use gRPC for communication? Here are some compelling benefits:
Performance
gRPC is designed for high-performance communication. By using HTTP/2 as the transport protocol, gRPC can handle multiple requests and responses simultaneously, leading to significant performance improvements.
Eficient Data Serialization
gRPC uses Protocol Buffers, a binary serialization format that is more eficient than JSON or XML. This reduces the overhead of data serialization and deserialization, making it ideal for high-traffic systems.
Language-Agnostic
gRPC supports a wide range of programming languages, including Java, Python, C++, Go, and many more. This allows developers to write services in their preferred language, without worrying about compatibility issues.
Service Discovery and Management
gRPC provides built-in support for service discovery and management, making it easy to manage and maintain large-scale systems.
Security
gRPC provides built-in support for SSL/TLS encryption, making it easy to secure communication between services.
Getting Started with gRPC
To get started with gRPC, you'll need to:
Define Your Service Interface
Define your service interface using Protocol Buffers (.proto file). This file contains the definition of your service, including methods, request and response types.
Generate Client and Server Code
Use the gRPC compiler (protoc) to generate client and server code from your .proto file. This code will include the necessary stubs and skeletons for your service.
Implement Your Service
Implement your service by writing the business logic for each method defined in your .proto file.
Use a gRPC Client
Use a gRPC client to interact with your service. The client will handle the underlying communication, allowing you to focus on your application logic.
gRPC Core Concepts
Unary RPC
Unary RPC is the simplest form of gRPC communication, where a client sends a single request and receives a single response.
Server-Streaming RPC
Server-streaming RPC allows a server to send multiple responses to a single client request.
Client-Streaming RPC
Client-streaming RPC allows a client to send multiple requests to a single server, with the server responding once all requests have been received.
Bi-Directional Streaming RPC
Bi-directional streaming RPC allows both the client and server to send multiple requests and responses in a streaming fashion.
Best Practices for Using gRPC
Use Protocol Buffers
Use Protocol Buffers as your serialization format to ensure eficient data exchange between services.
Define Clear Service Interfaces
Define clear and concise service interfaces to ensure easy understanding and maintenance of your services.
Use SSL/TLS Encryption
Use SSL/TLS encryption to secure communication between services.
Monitor and Debug Your Services
Use tools like gRPC CLI or Prometheus to monitor and debug your services.
Test Your Services
Write comprehensive tests for your services to ensure they function correctly.
Real-World Examples of gRPC in Action
Netflix's Eureka
Netflix's Eureka service discovery system uses gRPC to manage communication between services.
CoreOS's etcd
CoreOS's etcd distributed key-value store uses gRPC for communication between nodes.
Google's Cloud APIs
Google's Cloud APIs use gRPC to provide eficient and scalable communication between services.
Conclusion
In conclusion, gRPC is a powerful and eficient communication framework that can help you build scalable and performant systems. By understanding the benefits, core concepts, and best practices of gRPC, you can unlock eficient communication between microservices and take your applications to the next level. Whether you're building a new system or migrating an existing one, gRPC is definitely worth considering. With its language-agnostic approach, high-performance capabilities, and built-in support for security and service discovery, gRPC is an excellent choice for building modern distributed systems.
Unlocking Efficient Communication with gRPC: A Deep Dive
In the world of distributed systems, communication is key. As microservices architecture gains popularity, the need for eficient, reliable, and scalable communication between services becomes increasingly important. This is where gRPC, a high-performance RPC framework, comes into play. In this article, we'll delve deeper into the world of gRPC, exploring its benefits, architecture, and best practices for implementing it in your system.
What is gRPC?
gRPC (Remote Procedure Call) is an open-source framework developed by Google. It allows developers to define service interfaces and generate client and server code in a variety of programming languages. gRPC uses Protocol Buffers (protobuf) as the interface definition language (IDL) to define the structure of the data exchanged between services. This enables eficient, lightweight, and language-agnostic communication.
Benefits of gRPC
So, why choose gRPC over other communication frameworks? Here are some compelling reasons:
High Performance
gRPC uses HTTP/2 as the transport protocol, which allows for multiplexing, header compression, and binary encoding. This results in significant performance improvements compared to traditional HTTP/1.1-based systems.
Language-Agnostic
gRPC supports a wide range of programming languages, including Java, C++, Python, Go, Ruby, and many more. This means you can use gRPC to communicate between services written in different languages.
Scalability
gRPC's design enables horizontal scaling, making it an ideal choice for large-scale distributed systems.
Extensibility
gRPC's architecture is modular, allowing you to easily add new features and extensions as needed.
Security
gRPC provides built-in support for SSL/TLS and API key-based authentication, ensuring secure communication between services.
gRPC Architecture
A gRPC system consists of the following components:
Protocol Buffers (protobuf)
protobuf is used to define the structure of the data exchanged between services. It's a language-agnostic, eficient, and extensible format for serializing structured data.
Service Definition
The service definition is the interface specification for the service. It defines the methods, request and response types, and other metadata.
Stub
The stub is the client-side proxy that sends requests to the server and receives responses.
Server
The server is the implementation of the service definition. It receives requests from clients, processes them, and sends responses.
gRPC Runtime
The gRPC runtime provides the underlying infrastructure for the gRPC system, including the HTTP/2 connection, compression, and serialization.
Implementing gRPC
Now that we've covered the basics, let's dive into the implementation details.
Defining the Service
To define a service, you'll need to create a .proto
file that describes the service interface using Protocol Buffers. For example:
syntax = "proto3";
package greetings;
service Greeter {
rpc SayHello(HelloRequest) returns (HelloResponse) {}
}
message HelloRequest {
string name = 1;
}
message HelloResponse {
string message = 1;
}
This defines a Greeter
service with a single method SayHello
that takes a HelloRequest
message and returns a HelloResponse
message.
Generating Client and Server Code
Once you've defined the service, you can generate client and server code using the protoc
compiler. For example:
protoc --go_out=. --go-grpc_out=. greetings.proto
This generates Go code for the client and server.
Implementing the Server
To implement the server, you'll need to write code that handles incoming requests and sends responses. For example:
package main
import (
"context"
"log"
"golang.org/x/net/http2"
"google.golang.org/grpc"
pb "greetings/greeter"
)
type greeterServer struct{}
func (s *greeterServer) SayHello(ctx context.Context, req *pb.HelloRequest) (*pb.HelloResponse, error) {
return &pb.HelloResponse{Message: "Hello " + req.Name}, nil
}
func main() {
lis, err := net.Listen("tcp", ":50051")
if err != nil {
log.Fatalf("failed to listen: %v", err)
}
srv := grpc.NewServer()
pb.RegisterGreeterServer(srv, &greeterServer{})
reflection.Register(srv)
if err := srv.Serve(lis); err != nil {
log.Fatalf("failed to serve: %v", err)
}
}
This implements the SayHello
method and starts the gRPC server.
Implementing the Client
To implement the client, you'll need to create a stub that sends requests to the server. For example:
package main
import (
"log"
"golang.org/x/net/context"
"google.golang.org/grpc"
pb "greetings/greeter"
)
func main() {
conn, err := grpc.Dial("localhost:50051", grpc.WithInsecure())
if err != nil {
log.Fatalf("failed to dial: %v", err)
}
defer conn.Close()
client := pb.NewGreeterClient(conn)
req := &pb.HelloRequest{Name: "John"}
resp, err := client.SayHello(context.Background(), req)
if err != nil {
log.Fatalf("failed to say hello: %v", err)
}
log.Printf("Response: %v", resp.Message)
}
This creates a client that sends a HelloRequest
to the server and prints the response.
Best Practices
When using gRPC, keep the following best practices in mind:
Use meaningful service names and method names
Use descriptive names for your services and methods to ensure clarity and readability.
Use proper error handling
Implement proper error handling on both the client and server sides to ensure robustness and fault tolerance.
Use SSL/TLS encryption
Use SSL/TLS encryption to secure communication between services.
Monitor and debug
Use gRPC's built-in monitoring and debugging tools to troubleshoot issues and optimize performance.
Conclusion
In this article, we've explored the world of gRPC, covering its benefits, architecture, and implementation details. By following best practices and embracing gRPC's unique features, you can build eficient, scalable, and secure communication systems that meet the demands of modern distributed systems. With its language-agnostic nature, gRPC is an ideal choice for microservices architecture, enabling seamless communication between services written in different languages. Whether you're building a new system or migrating an existing one, gRPC is definitely worth considering.