How to use GraphQL in backend development
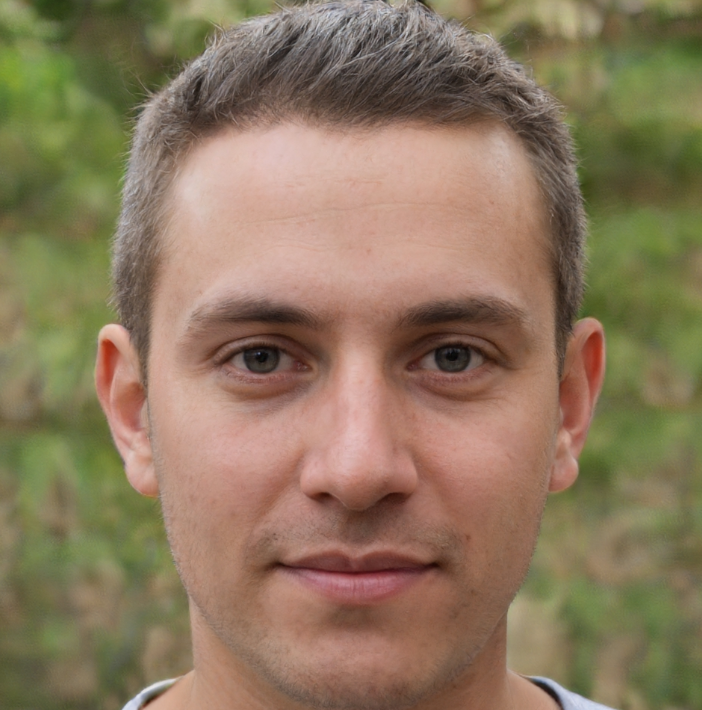

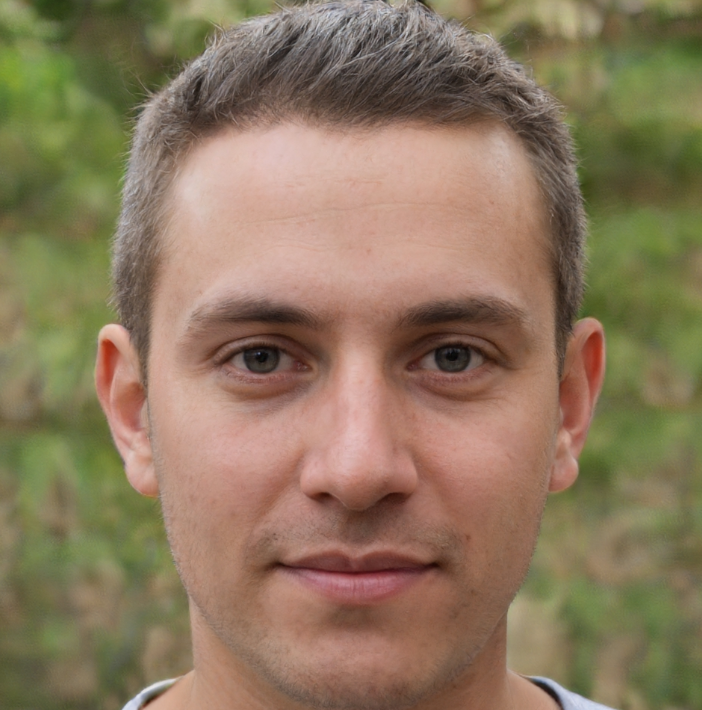
Unlocking the Power of GraphQL in Backend Development
In the world of backend development, data manipulation and querying are critical components of any application. Traditionally, REST (Representational State of Resource) has been the go-to architecture for building APIs. However, with the rise of complex and interconnected data models, REST has started to show its limitations. This is where GraphQL comes into play. GraphQL is a query language for APIs that allows for more flexible and efficient data retrieval.
What is GraphQL?
Before we dive into the world of GraphQL, let's quickly cover the basics. GraphQL is a query language for APIs developed by Facebook in 2015. It allows clients to specify exactly what data they need from the server, reducing the amount of data transferred and improving performance. GraphQL is often described as a more efficient and flexible alternative to REST.
Why Use GraphQL?
So, why should you consider using GraphQL in your backend development? Here are a few compelling reasons:
- Improved Performance: By only retrieving the data that is needed, GraphQL reduces the amount of data transferred between the client and server, resulting in improved performance and reduced latency.
- Flexibility: GraphQL allows clients to specify exactly what data they need, making it easier to evolve and adapt to changing data models.
- Better Error Handling: GraphQL provides a more comprehensive error handling system, making it easier to debug and resolve issues.
- Reduced Over-fetching: GraphQL eliminates the need for multiple API calls, reducing the amount of data transferred and improving overall efficiency.
Setting Up a GraphQL Server
To get started with GraphQL, you'll need to set up a GraphQL server. There are several libraries and frameworks available, including Apollo Server, GraphQL Yoga, and Prisma. For this example, we'll use Apollo Server, a popular and widely-used GraphQL server.
First, create a new project and install Apollo Server using npm or yarn:
npm install apollo-server
Next, create a new file called server.js
and add the following code:
const { ApolloServer } = require('apollo-server');
const typeDefs = `
type Query {
hello: String
}
`;
const resolvers = {
Query: {
hello: () => 'Hello, World!'
}
};
const server = new ApolloServer({ typeDefs, resolvers });
server.listen().then(({ url }) => {
console.log(`GraphQL API server listening on ${url}`);
});
This code defines a simple GraphQL schema with a single query called hello
. The resolver function returns a string "Hello, World!" when the hello
query is executed.
Defining a GraphQL Schema
A GraphQL schema is the backbone of a GraphQL API. It defines the types of data available and the relationships between them. In our previous example, we defined a simple schema with a single query. Let's expand on this schema to include more complex types and fields.
const typeDefs = `
type Query {
hello: String
users: [User]
}
type User {
id: ID!
name: String!
email: String!
}
`;
This schema defines two types: Query
and User
. The Query
type has two fields: hello
and users
. The users
field returns an array of User
objects, which have three fields: id
, name
, and email
.
Resolvers: The Magic Behind GraphQL
Resolvers are the functions that execute when a GraphQL query is received. They are responsible for fetching the required data and returning it to the client. In our previous example, we defined a simple resolver function that returned a string. Let's create a more comprehensive resolver function that fetches data from a database.
const resolvers = {
Query: {
users: async () => {
const users = await User.find(); // assume User is a MongoDB model
return users;
}
}
};
This resolver function uses a MongoDB model to fetch an array of User
objects from the database.
Querying the GraphQL API
Now that we have our GraphQL server up and running, let's query the API using a GraphQL client. We'll use the popular graphql-request
library to send a query to the server.
const { GraphQLClient } = require('graphql-request');
const client = new GraphQLClient('http://localhost:4000/graphql', {
headers: {
'Content-Type': 'application/json'
}
});
const query = `
query {
users {
id
name
email
}
}
`;
client.request(query)
.then(data => console.log(data))
.catch(error => console.error(error));
This code sends a GraphQL query to the server, requesting the id
, name
, and email
fields for all User
objects. The server will execute the query, fetch the required data, and return the result to the client.
Real-World Use Cases for GraphQL
GraphQL is not just a toy for experimenting with APIs. It's a powerful technology that has been adopted by many companies, including Facebook, GitHub, and Pinterest. Here are a few real-world use cases for GraphQL:
- E-commerce platforms: GraphQL is ideal for e-commerce platforms that require complex and flexible data querying. Shopify, for example, uses GraphQL to power its API.
- Social media platforms: Facebook, the birthplace of GraphQL, uses it to power its API. GraphQL allows Facebook to efficiently handle billions of queries per day.
- Content management systems: GraphQL is a natural fit for content management systems like WordPress, where complex data models require flexible querying.
Conclusion
In this artical, we've explored the world of GraphQL and its applications in backend development. We've covered the basics of GraphQL, set up a GraphQL server, defined a schema, and queried the API. GraphQL is a powerful technology that offers improved performance, flexibility, and error handling compared to traditional REST APIs. Whether you're building a simple API or a complex e-commerce platform, GraphQL is definitely worth considering. With its growing popularity and adoption, GraphQL is set to become a staple of modern backend development.