How to use GraphQL for backend development
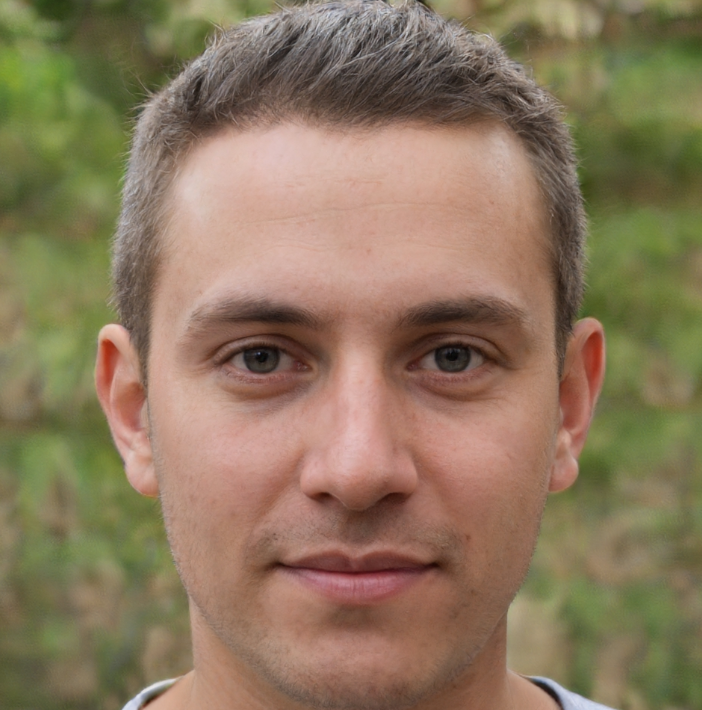

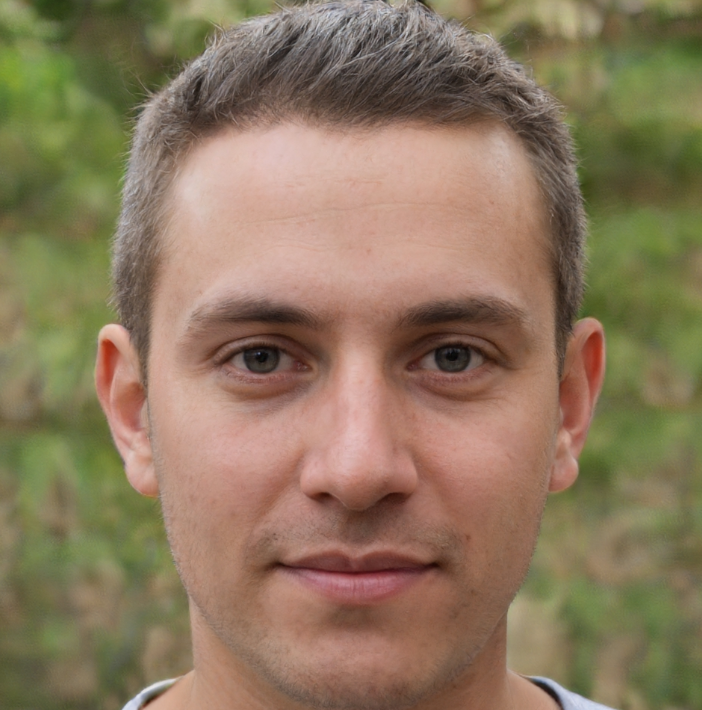
GraphQL: Unlocking Efficient Backend Development
In today's fast-paced world of backend development, REST (Representational State of Resource) has been the go-to choice for building APIs. But, with the advent of GraphQL, devlopers now have a more efficient and flexible alternative. GraphQL, introduced by Facebook back in 2015, is a query language designed to optimize network requests and provide a more intuitive way of managing data. In this article, we'll delve into the world of GraphQL, exploring how to use it for backend development, its benefits, and some best practices to get you started.
What is GraphQL?
GraphQL is a specifcation for a query language that allows clients to specify exactly what data they need from a server. Unlike REST, which typically returns a fixed set of data, GraphQL enables clients to customize their requests, reducing the amount of data transferred and improving performance. GraphQL also provides a strongly typed schema, which helps catch errors early on and ensures data consistency.
Setting up a GraphQL Server
To get started with GraphQL, you'll need to set up a server. There are several libraries and frameworks available, including Apollo Server, GraphQL Server, and Prisma. For this example, we'll use Apollo Server, a popular and widely adopted implementation.
First, install Apollo Server using npm or yarn:
npm install apollo-server
Next, create a new file called server.js
and import the necessary dependencies:
const { ApolloServer } = require('apollo-server');
const typeDefs = require('./schema');
const resolvers = require('./resolvers');
const server = new ApolloServer({ typeDefs, resolvers });
server.listen().then(({ url }) => {
console.log(`Server ready at ${url}`);
});
In this example, we define a basic GraphQL server using the ApolloServer
class. We also import our schema definition and resolvers, which will handle the data logic for our API.
Defining a Schema
The schema is the backbone of a GraphQL API, defining the types and relationships between data. To create a schema, we'll use GraphQL's Schema Definition Language (SDL). In our schema.js
file, we'll define a simple schema with a single type, Book
:
type Book {
id: ID!
title: String!
author: String!
}
type Query {
books: [Book!]!
}
Here, we define a Book
type with three fields: id
, title
, and author
. We also define a Query
type with a single field, books
, which returns an array of Book
objects.
Resolvers: The Data Logic
Resolvers are functions that execute when a client requests data from our API. They're responsible for retrieving or creating the necessary data and returning it to the client. In our resolvers.js
file, we'll define a resolver for the books
field:
const books = [
{ id: 1, title: 'Book 1', author: 'Author 1' },
{ id: 2, title: 'Book 2', author: 'Author 2' },
];
const resolvers = {
Query: {
books: () => books,
},
};
In this example, we define a simple resolver that returns a hardcoded array of book objects.
Querying the API
Now that our server is set up, let's query the API using GraphQL's query language. We'll use the Apollo Client, a popular client-side library, to send requests to our server.
First, install the Apollo Client:
npm install @apollo/client
Next, create a new file called client.js
and import the necessary dependencies:
import { ApolloClient, InMemoryCache } from '@apollo/client';
const client = new ApolloClient({
uri: 'http://localhost:4000/graphql',
cache: new InMemoryCache(),
});
client.query({
query: gql`
query {
books {
id
title
author
}
}
`,
variables: {},
}).then(result => console.log(result.data));
In this example, we create an instance of the Apollo Client, pointing it to our server's GraphQL endpoint. We then define a query that requests the id
, title
, and author
fields for all Book
objects. Finally, we log the result to the console.
Benefits of GraphQL
GraphQL offers several benefits over traditional RESTful APIs:
- Reduced Network Requests: GraphQL enables clients to request only the necessary data, reducing the number of network requests and improving performance.
- Flexibility: GraphQL's query language allows clients to customize their requests, making it easier to adapt to changing requirements.
- Strongly Typed Schema: GraphQL's schema ensures data consistency and helps catch errors early on.
- Improved Development Experience: GraphQL's type system and schema make it easier for developers to understand the data model and build APIs.
Best Practices
When using GraphQL for backend development, keep the following best practices in mind:
- Keep Resolvers Simple: Resolvers should be simple and focused on a single task, making it easier to maintain and debug your API.
- Use a Strongly Typed Schema: GraphQL's schema is a powerful tool for ensuring data consistency and catching errors early on.
- Optimize Performance: Use caching, batching, and other optimization techniques to improve performance and reduce network requests.
- Document Your API: Use GraphQL's built-in documentation features, such as GraphQL Playground, to provide clear API documentation for clients.
Conclusion
GraphQL is a powerful tool for building efficient and scalable backend APIs. By leveraging its query language, strongly typed schema, and flexibility, developers can create APIs that are both performant and easy to maintain. With its growing ecosystem and adoption, GraphQL is an excellent choice for building modern web applications. By following the best practices outlined in this article, you'll be well on your way to creating robust and efficient GraphQL APIs that delight users and developers alike.
And, as a side note, don't forget to keep your resolver functions organized and well-documented, it's a good idear to seperate them into different files and folders, it will make your life so much easer when you need to make changes or debug your API.