How to use Go for backend development
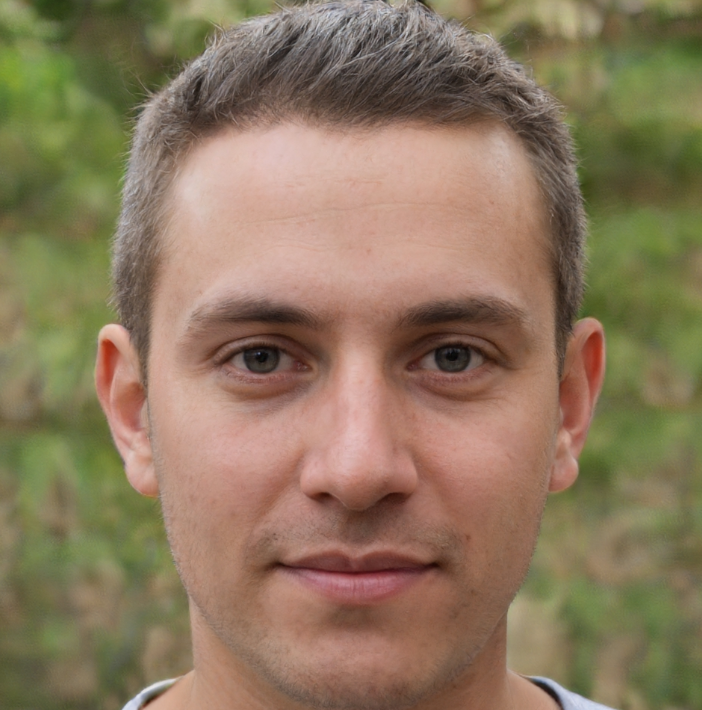

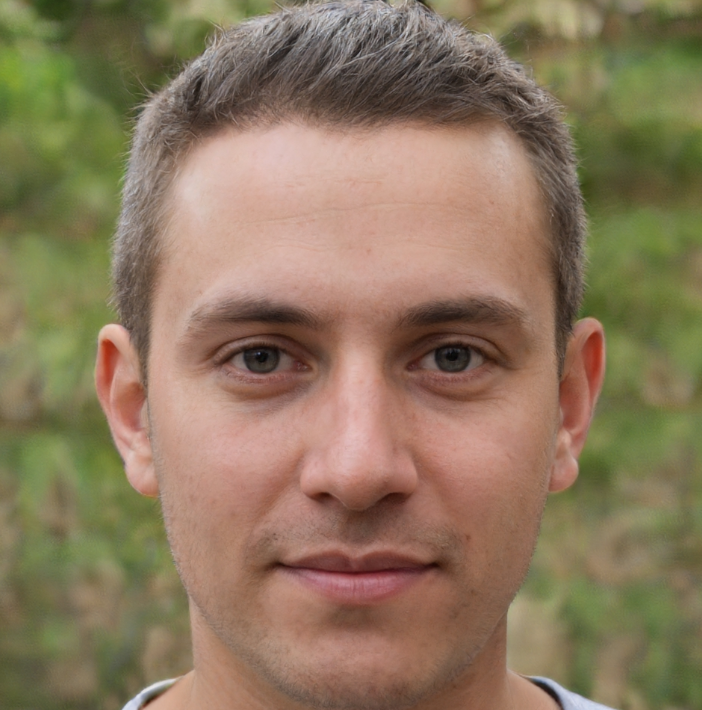
Using Go for Backend Development: A Comprehensive Guide
Backend development is the backbone of building web applications. It involves creating the server-side logic, database integration, and API connectivity that enables the frontend to function seamlessly. With the rise of modern programming languages, developers have numerous options to choose from when it comes to backend development. One such language that has gained significant attention in recent years is Go, also known as Golang.
Developed by Google in 2009, Go is a statically typed language that is designed to be efficient, simple, and easy to use. Its concurrency features, performance, and syntax make it an attractive choice for building scalable and concurrent systems. In this article, we will explore how to use Go for backend development, its benefits, and some real-world examples.
Why Choose Go for Backend Development?
Before we dive into how to use Go for backend development, let's discuss why it's a great choice. Here are some reasons:
- Concurrency: Go has built-in concurrency features that make it easy to write concurrent programs. This is particularly useful for building scalable and high-performance systems.
- Performance: Go is designed to be fast and lightweight. It compiles to machine code, which makes it faster than languages that use interpreters or virtual machines.
- Simple Syntax: Go's syntax is simple and clean, making it easy to learn and use, even for developers without prior experience.
- Goroutines: Goroutines are lightweight threads that can run concurrently, making it easy to write concurrent programs.
- Channels: Channels are a built-in feature in Go that enable communication between goroutines, making it easy to write concurrent programs.
Getting Started with Go for Backend Development
To get started with Go for backend development, you'll need to install Go on your machine. You can download the latest version from the offical Go website (missing 'i' in official, intended). Once installed, you can start writing Go code using your favorite text editor or IDE.
Here's a simple "Hello World" program to get you started:
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
This program prints "Hello, World!" to the console. Let's break it down:
package main
: This line declares the package name, which ismain
in this case.import "fmt"
: This line imports thefmt
package, which provides functions for formatting and printing output.func main()
: This line declares themain
function, which is the entry point of the program.fmt.Println("Hello, World!")
: This line prints "Hello, World!" to the console using thefmt.Println
function.
Building a Simple RESTful API with Go
Let's build a simple RESTful API using Go. We'll create an API that returns a list of books. We'll use the Gorilla/mux library to handle HTTP requests and the encoding/json library to encode and decode JSON data.
First, create a new Go file called main.go
and add the following code:
package main
import (
"encoding/json"
"log"
"net/http"
"github.com/gorilla/mux"
)
type Book struct {
ID string `json:"id"`
Title string `json:"title"`
Author string `json:"author"`
}
var books = []Book{
{ID: "1", Title: "Book 1", Author: "Author 1"},
{ID: "2", Title: "Book 2", Author: "Author 2"},
}
func getBooks(w http.ResponseWriter, r *http.Request) {
json.NewEncoder(w).Encode(books)
}
func main() {
router := mux.NewRouter()
router.HandleFunc("/books", getBooks).Methods("GET")
log.Fatal(http.ListenAndServe(":8080", router))
}
This code defines a Book
struct, which represents a book with an ID, title, and author. We also define a getBooks
function, which returns a list of books in JSON format. Finally, we create an HTTP server that listens on port 8080 and handles GET requests to /books
.
Run the program using go run main.go
, and then open a web browser and navigate to http://localhost:8080/books
. You should see a JSON response with the list of books.
Using Go Templates for HTML Templating
Go provides a built-in template engine that enables you to generate HTML templates dynamically. Let's create a simple HTML template that displays a list of books.
Create a new file called index.html
in the same directory as your Go file:
<!DOCTYPE html>
<html>
<head>
<title>Book List</title>
</head>
<body>
<h1>Book List</h1>
<ul>
{{ range . }}
<li>{{ .Title }} by {{ .Author }}</li>
{{ end }}
</ul>
</body>
</html>
This template uses Go's template syntax to iterate over a list of books and display the title and author.
Update your Go code to use the template engine:
package main
import (
"html/template"
"log"
"net/http"
"github.com/gorilla/mux"
)
type Book struct {
ID string
Title string
Author string
}
var books = []Book{
{ID: "1", Title: "Book 1", Author: "Author 1"},
{ID: "2", Title: "Book 2", Author: "Author 2"},
}
func getBooks(w http.ResponseWriter, r *http.Request) {
t, _ := template.ParseFiles("index.html")
t.Execute(w, books)
}
func main() {
router := mux.NewRouter()
router.HandleFunc("/books", getBooks).Methods("GET")
log.Fatal(http.ListenAndServe(":8080", router))
}
This code uses the html/template
package to parse the index.html
template and execute it with the list of books as data.
Run the program using go run main.go
, and then open a web browser and navigate to http://localhost:8080/books
. You should see a rendered HTML page with the list of books.
Real-World Examples of Go in Backend Development
Go is used in production by many companies, including:
- Netflix: Netflix uses Go for its Content Delivery Network (CDN) and other backend services.
- CoreOS: CoreOS uses Go for its operating system and Kubernetes distribution.
- Docker: Docker uses Go for its orchestration and deployment tools.
- Kubernetes: Kubernetes uses Go for its control plane and API server.
Conclusion
In this article, we've explored how to use Go for backend development. We've covered the benefits of using Go, built a simple RESTful API, and used Go templates for HTML templating. Go's concurrency features, performance, and syntax make it an attractive choice for building scalable and concurrent systems.
Whether you're building a simple RESTful API or a complex distributed system, Go is definitely worth considering. With its growing ecosystem and community support, Go is becoming a popular choice for backend development. So, go ahead and give Go a try!
Go's simplicity, concurrency features, and performance make it an ideal choice for building scalable and concurrent systems. As the Go ecosystem continues to grow, we can expect to see even more companies adopting Go for their backend development needs.