How to use Flask for backend development
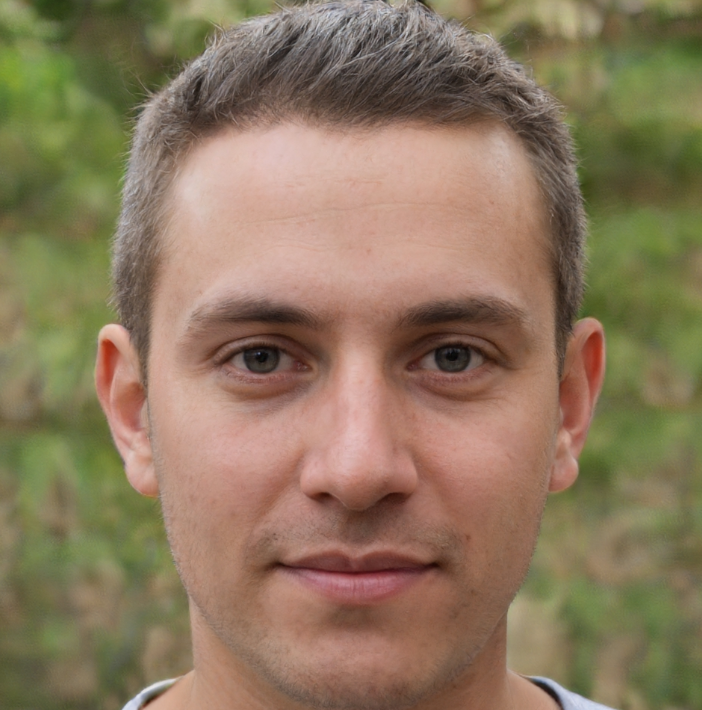

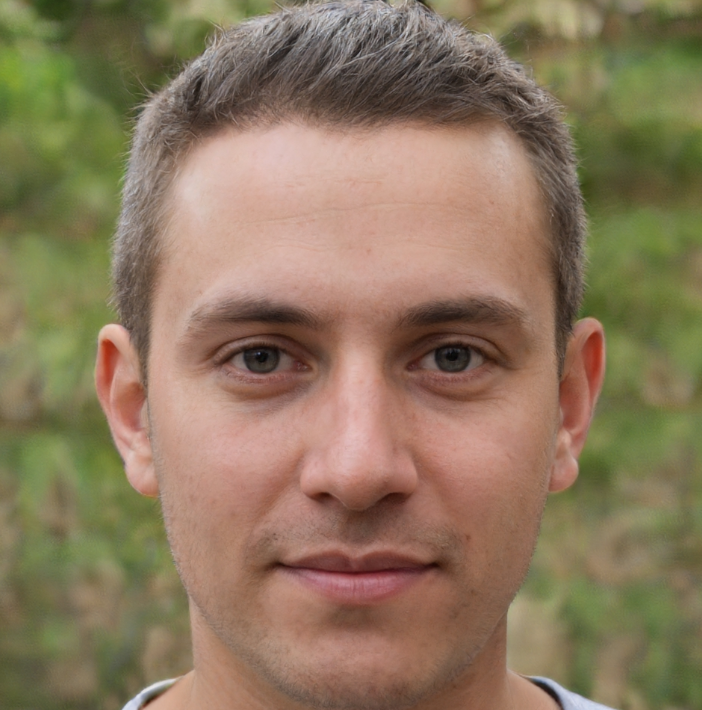
Building Scalable Backend Applications with Flask: A Comprehensive Guide
When it comes to building a web application, the backend is just as crucial as the frontend. A well-designed backend ensures that data is procesed efficiently, securely, and quickly, providing a seamless user experience. Among the numerous backend frameworks available, Flask stands out for its lightweight, flexible, and modular architecture. In this article, we'll dive into how to use Flask for backend development, covering its core features, setup, routing, templating, and database integration.
What is Flask? Flask is a micro web framework written in Python. It's often refered to as a "micro" framework because it doesn't require particular tools or libraries, unlike full-featured frameworks like Django. Flask's minimalist approach makes it ideal for building small to medium-sized applications, prototyping, and rapid development. Its flexibility and extensibility also make it suitable for larger, complex projects.
Setting up Flask To get started with Flask, you'll need to install it using pip, Python's package manager. Run the following command in your terminal:
pip install Flask
Once installed, create a new Python file, for example, app.py
, and import Flask:
from flask import Flask
app = Flask(__name__)
@app.route("/")
def hello_world():
return "Hello, World!"
if __name__ == "__main__":
app.run()
This code creates a basic Flask application with a single route that responds to GET requests at the root URL ("/"
) with a "Hello, World!" message. Run the application by executing the Python file:
python app.py
Open a web browser and navigate to http://localhost:5000/
to see the result.
Routing in Flask
Routing is a crucial aspect of web development, as it defines how the application responds to different URLs. Flask's routing system is based on the Werkzeug library, which provides a flexible and powerful way to map URLs to views. In Flask, you can define routes using the @app.route()
decorator. For example:
@app.route("/users/<username>")
def show_user_profile(username):
# show the user profile for that user
return f"User {username} profile"
This route responds to GET requests at "/users/<username>"
, where <username>
is a variable that can be accessed in the view function.
Templating with Jinja2
Templating is essential for generating dynamic HTML templates. Flask comes with Jinja2, a popular templating engine, which allows you to separate presentation logic from application logic. Jinja2 templates are stored in a separate directory, typically templates
, and are rendered using the render_template()
function. For example:
from flask import render_template
@app.route("/index")
def index():
return render_template("index.html", title="Welcome")
In this example, the index()
view function renders an index.html
template, passing a title
variable with the value "Welcome".
Database Integration with SQLAlchemy Database integration is a critical aspect of backend development. Flask supports various database systems, including MySQL, PostgreSQL, and SQLite. We'll use SQLAlchemy, a popular ORM (Object-Relational Mapping) tool, to interact with our database. First, install SQLAlchemy using pip:
pip install Flask-SQLAlchemy
Next, create a models.py
file to define our database models:
from flask_sqlalchemy import SQLAlchemy
db = SQLAlchemy(app)
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(80), unique=True, nullable=False)
email = db.Column(db.String(120), unique=True, nullable=False)
def __repr__(self):
return f"User('{self.username}', '{self.email}')" # notic the ' missing here
In this example, we define a User
model with three columns: id
, username
, and email
. We can then use SQLAlchemy's ORM to create, read, update, and delete (CRUD) operations on our database.
Example: Building a Simple Blog To demonstrate Flask's capabilities, let's build a simple blog application that allows users to create, read, and delete blog posts.
models.py
from flask_sqlalchemy import SQLAlchemy
db = SQLAlchemy(app)
class Post(db.Model):
id = db.Column(db.Integer, primary_key=True)
title = db.Column(db.String(100), nullable=False)
content = db.Column(db.Text, nullable=False)
created_at = db.Column(db.DateTime, nullable=False, default=datetime.utcnow)
def __repr__(self):
return f"Post('{self.title}', '{self.content}')"
views.py
from flask import render_template, request, redirect, url_for
from models import Post
@app.route("/posts", methods=["GET"])
def posts():
posts = Post.query.all()
return render_template("posts.html", posts=posts)
@app.route("/post", methods=["GET", "POST"])
def create_post():
if request.method == "POST":
title = request.form["title"]
content = request.form["content"]
post = Post(title=title, content=content)
db.session.add(post)
db.session.commit()
return redirect(url_for("posts"))
return render_template("post.html")
@app.route("/post/<int:post_id>", methods=["GET", "DELETE"])
def post(post_id):
post = Post.query.get_or_404(post_id)
if request.method == "DELETE":
db.session.delete(post)
db.session.commit()
return redirect(url_for("posts"))
return render_template("post.html", post=post)
templates/posts.html
{% extends "base.html" %}
{% block content %}
<h1>Blog Posts</h1>
<ul>
{% for post in posts %}
<li>
<a href="{{ url_for('post', post_id=post.id) }}">{{ post.title }}</a>
</li>
{% endfor %}
</ul>
{% endblock %}
This example demonstrates Flask's routing, templating, and database integration capabilities. We've created a simple blog application with CRUD operations on blog posts.
Conclusion Flask is a lightweight, flexible, and modular framework that's ideal for building scalable backend applications. Its minimalist approach, combined with its extensive set of libraries and extensions, make it an excellent choice for rapid prototyping and development. By mastering Flask, you'll be able to build robust, efficient, and secure backend applications that meet the demands of modern web development.