How to use Express.js for backend development
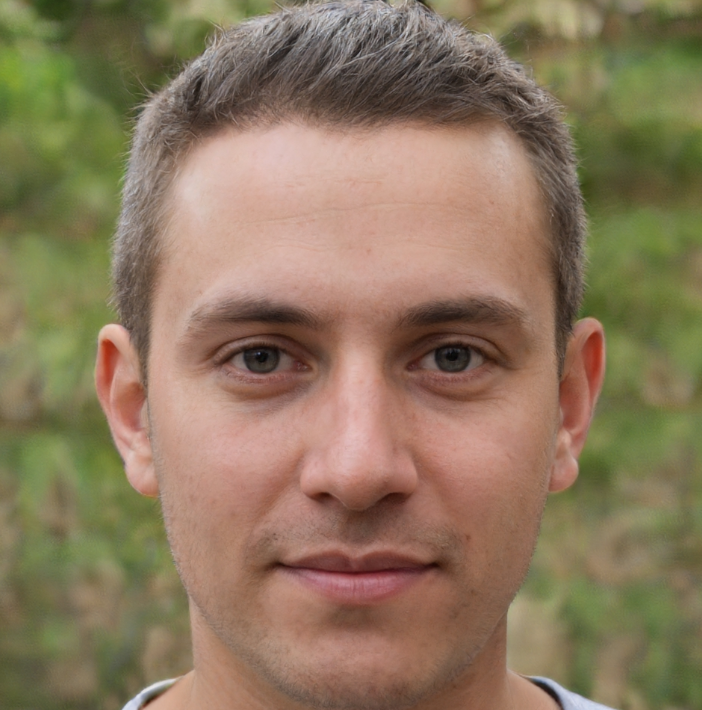

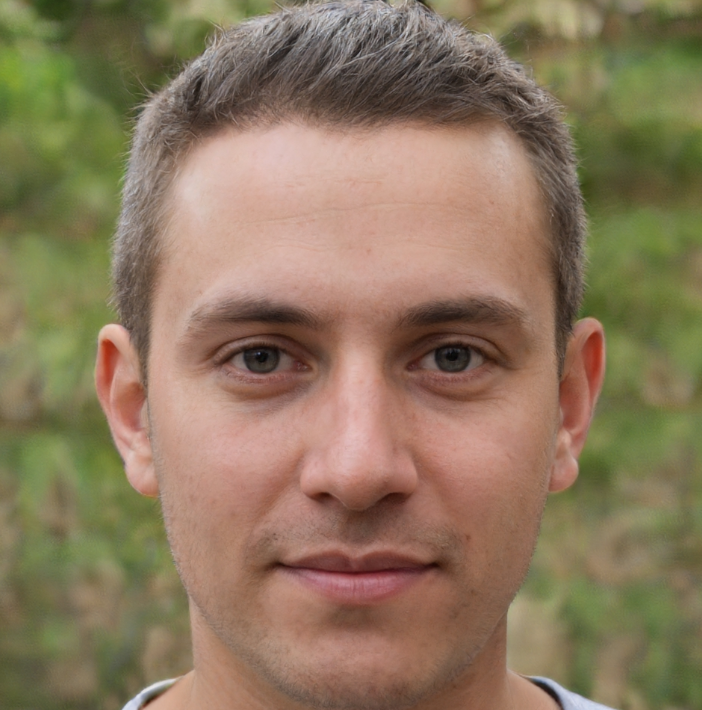
Building Scalable Backend Applications with Express.js
As a developer, chooising the right framework for building a backend application can be a daughting task. With so many options available, it's essential to select a framework that is easy to learn, flexible, and scalable. Express.js, a Node.js framework, is an exellent choice for building fast, robust, and scalable backend applications. In this article, we'll explore how to use Express.js for backend development, covering its key features, advantages, and a step-by-step guide to building a simple RESTful API.
What is Express.js?
Express.js is a lightweight, flexible, and modular Node.js web framework that allows developers to build web applications quickly and efficiently. Released in 2010, Express.js has become one of the most popular Node.js frameworks, used by companies like Uber, Accenture, and IBM. Express.js provides a thin layer of functionality, making it ideal for building fast and scalable web applications.
Key Features of Express.js
1. Route-Based Architecture
Express.js uses a route-based architecture, which means that you can define routes to handle specific HTTP requests. This approach makes it easy to manage and organize your code, making it more maintainable and scalable.
2. Middleware Functions
Middleware functions are a key feature of Express.js. These functions are executed in a specific order, allowing you to perform tasks such as authentication, error handling, and caching. Middleware functions can be used to extend the functionality of your application, making it more robust and secure.
3. Templating Engines
Express.js supports a variety of templating engines, including EJS, Jade, and Pug. Templating engines allow you to render dynamic web pages, making it easy to separate presentation logic from application logic.
4. Error Handling
Express.js provides a built-in error handling mechanism, allowing you to catch and handle errors in a centralized way. This feature makes it easy to debug and troubleshoot your application.
Advantages of Using Express.js
1. Fast Development
Express.js is built on top of Node.js, making it ideal for building fast and scalable web applications. With Express.js, you can quickly develop and deploy your application, reducing the time-to-market.
2. Flexible and Modular
Express.js is a modular framework, allowing you to add or remove features as needed. This flexibility makes it easy to customize your application, making it suitable for a wide range of use cases.
3. Large Community
Express.js has a large and active community, with numerous third-party libraries and plugins available. This community support makes it easy to find solutions to common problems, reducing the development time.
A Step-by-Step Guide to Building a Simple RESTful API with Express.js
In this section, we'll build a simple RESTful API using Express.js. We'll create a book management system that allows users to create, read, update, and delete books.
Step 1: Install Express.js
To install Express.js, run the following command in your terminal:
npm install express
Step 2: Create a New Express.js Project
Create a new folder for your project and navigate to it in your terminal. Run the following command to create a new Express.js project:
express book-management-system
This command will create a new Express.js project with the basic directory structure.
Step 3: Define Routes
In Express.js, routes are defined using the app.get()
, app.post()
, app.put()
, and app.delete()
methods. We'll define four routes for our book management system:
- GET /books: Retrieves a list of all books
- POST /books: Creates a new book
- GET /books/:id: Retrieves a specific book
- PUT /books/:id: Updates a specific book
- DELETE /books/:id: Deletes a specific book
Here's an example of how you can define these routes:
const express = require('express');
const app = express();
app.get('/books', (req, res) => {
// Retrieve a list of all books
const books = [
{ id: 1, title: 'Book 1', author: 'Author 1' },
{ id: 2, title: 'Book 2', author: 'Author 2' },
];
res.json(books);
});
app.post('/books', (req, res) => {
// Create a new book
const book = { id: 3, title: req.body.title, author: req.body.author };
res.json(book);
});
app.get('/books/:id', (req, res) => {
// Retrieve a specific book
const id = req.params.id;
const book = { id: 1, title: 'Book 1', author: 'Author 1' };
res.json(book);
});
app.put('/books/:id', (req, res) => {
// Update a specific book
const id = req.params.id;
const book = { id: 1, title: req.body.title, author: req.body.author };
res.json(book);
});
app.delete('/books/:id', (req, res) => {
// Delete a specific book
const id = req.params.id;
res.json({ message: 'Book deleted succesfully' });
});
app.listen(3000, () => {
console.log('Server started on port 3000');
});
Step 4: Test the API
To test the API, you can use a tool like Postman or cURL. Send a GET request to http://localhost:3000/books
to retrieve a list of all books.
Conclusion
In this article, we've explored the world of Express.js, covering its key features, advantages, and a step-by-step guide to building a simple RESTful API. With its route-based architecture, middleware functions, and templating engines, Express.js is an ideal choice for building fast, scalable, and robust backend applications. Whether you're building a simple web application or a complex enterprise-level system, Express.js provides the flexibility and customizability you need to succeed.
Additional Resources
For further learning, I recomend checking out the official Express.js documentation and the Node.js documentation. You can also explore other Node.js frameworks like Koa.js and Hapi, which offer similar functionality to Express.js.
I hope this article has provided you with a comprehensive understanding of Express.js and how to use it for building scalable backend applications. Happy coding!