How to use Docker for backend development
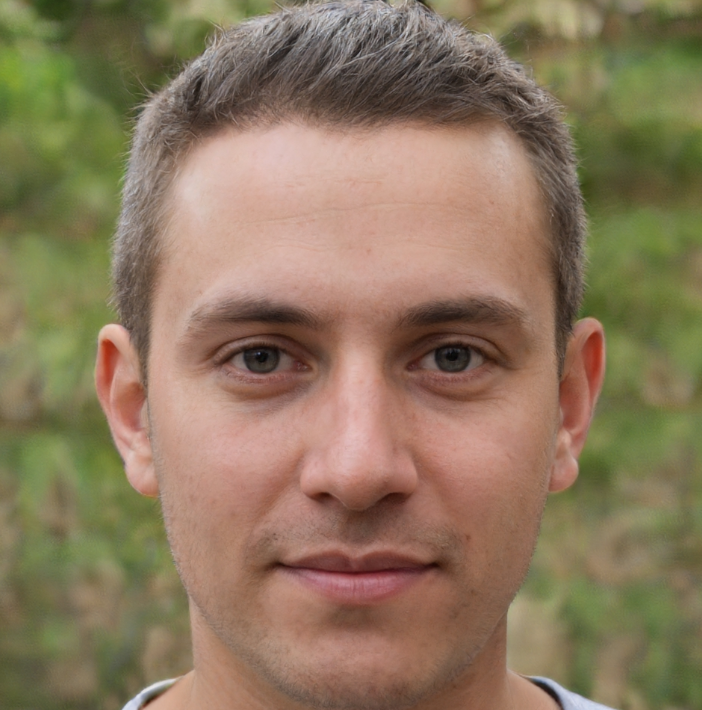

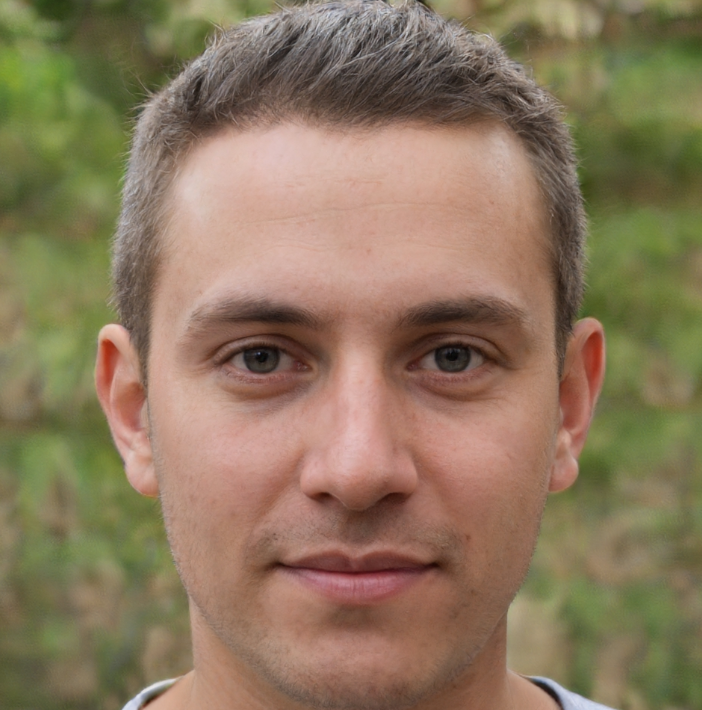
Simplifying the Development Process with Docker Containers
In the world of backend development, managing multiple projects with different dependencies and environments can be a daunting task. Docker, a popular containerization platform, has revolutionized the way developers work by providing a lightweight and portable way to deploy applications. In this article, we'll explore how to use Docker for backend development, covering the benefits, setup, and best practices for incorporating Docker into your development workflow.
What is Docker?
Before diving into the world of Docker, let's take a step back and understand what Docker is. Docker is an open-source platform that enables you to package, ship, and run applications in containers. Containers are lightweight and standalone, providing a consistent and reliable way to deploy applications across different environments. Docker containers share the same kernel as the host operating system, making them more efficient than traditional virtual machines.
Benefits of Using Docker for Backend Development
So, why should you use Docker for backend development? Here are some compelling reasons:
- Isolation: Docker containers provide a high degree of isolation, ensuring that each application runs in its own environment, without interfering with other applications or the host system.
- Portability: Docker containers are highly portable, allowing you to develop, test, and deploy applications across different environments, without worrying about compatibility issues.
- Efficient Resource Utilization: Docker containers use fewer resources than traditional virtual machines, making them an attractive option for development environments.
- Simplified Collaboration: Docker enables multiple developers to work on the same project, without worrying about dependency conflicts or environment differences.
Setting Up Docker for Backend Development
To get started with Docker, you'll need to install Docker Desktop on your machine. Once installed, you can create a new Docker project by creating a Dockerfile
. A Dockerfile
is a text file that contains instructions for building a Docker image.
Here's an example of a simple Dockerfile
for a Node.js application:
FROM node:14
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
RUN npm run build
EXPOSE 3000
CMD ["npm", "start"]
Let's break down this Dockerfile
:
FROM node:14
specifies the base image for our application, which is Node.js 14.WORKDIR /app
sets the working directory to/app
.COPY package*.json ./
copies thepackage.json
file into the container.RUN npm install
installs the dependencies specified inpackage.json
.COPY . .
copies the application code into the container.RUN npm run build
builds the application.EXPOSE 3000
exposes port 3000 for the application.CMD ["npm", "start"]
sets the default command to run the application withnpm start
.
Building and Running a Docker Image
Once you have a Dockerfile
, you can build a Docker image using the following command:
docker build -t my-node-app .
This command builds a Docker image with the tag my-node-app
. To run the image, use the following command:
docker run -p 3000:3000 my-node-app
This command runs the my-node-app
image, mapping port 3000 on the host machine to port 3000 in the container.
Best Practices for Using Docker in Backend Development
Here are some best practices to keep in mind when using Docker for backend development:
- Keep Your Dockerfile Lean: A lean
Dockerfile
ensures that your image is small and efficient. - Use Official Images: Use official images from Docker Hub to ensure that your application is built on a trusted foundation.
- Use Environment Variables: Use environment variables to configure your application, rather than hardcoding values.
- Use Volumes: Use volumes to persist data across container restarts.
- Monitor and Log: Monitor and log your application's performance to identify issues and optimize performance.
Real-World Example: Using Docker with a Node.js and MongoDB Application
Let's consider a real-world example of using Docker with a Node.js and MongoDB application. We'll create a Dockerfile
for the Node.js application and a docker-compose.yml
file to orchestrate the application and MongoDB container.
Here's the Dockerfile
for the Node.js application:
FROM node:14
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
RUN npm run build
EXPOSE 3000
CMD ["npm", "start"]
And here's the docker-compose.yml
file:
version: "3"
services:
app:
build: .
ports:
- "3000:3000"
depends_on:
- mongo
environment:
- MONGO_URI=mongodb://mongo:27017/
mongo:
image: mongo:4.4
volumes:
- mongo_data:/data/db
volumes:
mongo_data:
This docker-compose.yml
file defines two services: app
and mongo
. The app
service is built using the Dockerfile
in the current directory, and it depends on the mongo
service. The mongo
service uses the official MongoDB image and persists data using a named volume.
To start the application, run the following command:
docker-compose up
This command starts both the app
and mongo
services, and maps port 3000 on the host machine to port 3000 in the app
container.
Unlocking Efficient Backend Development with Docker
Backend development is a crucial aspect of building modern web applications. With the rise of microservices architecture, the complexity of backend development has increased manifold. To tackle this complexity, developers have been seeking efficient ways to manage and deploy their applications. This is where Docker comes into the picture.
Understanding Docker Basics
Before we dive into using Docker for backend development, let's quickly cover the basics of Docker. Docker is built around three main concepts: images, containers, and volumes.
- Images: Docker images are lightweight and standalone executable packages that include everything an application needs to run, such as code, libraries, and dependencies.
- Containers: Containers are runtime instances of images. You can create multiple containers from a single image, and each container can be customized and configured independently.
- Volumes: Volumes are directories that are shared between the host machine and the container. This allows data to persist even after the container is deleted or recreated.
Setting Up Docker for Backend Development
To get started with Docker for backend development, you'll need to install Docker on your machine. Once installed, you can create a Dockerfile for your application. A Dockerfile is a text file that contains instructions for building a Docker image.
Here's an example of a simple Dockerfile for a Node.js application:
FROM node:14
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
RUN npm run build
EXPOSE 3000
CMD ["npm", "start"]
This Dockerfile assumes that your application is built using Node.js and uses the npm
package manager. It creates a new container with the Node.js 14 image, sets up the working directory, copies the package.json
file, installs the dependencies, copies the application code, builds the application, and exposes port 3000.
Using Docker Compose for Multi-Container Applications
In a real-world backend development scenario, you often need to work with multiple services, such as databases, caching layers, and message queues. Docker Compose is a tool that allows you to define and run multi-container Docker applications. With Docker Compose, you can create a single configuration file (docker-compose.yml) that defines all the services and their dependencies.
Here's an example of a docker-compose.yml file for a Node.js application with a PostgreSQL database:
version: '3'
services:
app:
build: .
ports:
- "3000:3000"
depends_on:
- db
environment:
- DATABASE_URL=postgres://user:password@db:5432/database
db:
image: postgres
environment:
- POSTGRES_USER=user
- POSTGRES_PASSWORD=password
- POSTGRES_DB=database
volumes:
- db-data:/var/lib/postgresql/data
volumes:
db-data:
This docker-compose.yml file defines two services: app
and db
. The app
service builds the Docker image from the current directory, maps port 3000 on the host machine to port 3000 in the container, and depends on the db
service. The db
service uses the official PostgreSQL image, sets up the environment variables for the database, and mounts a volume to persist data.
Advantages of Using Docker for Backend Development
So, why should you use Docker for backend development? Here are some of the advantages:
- Faster Development: With Docker, you can quickly spin up a new environment for your application, complete with all the dependencies and services it needs. This saves you a ton of time and effort in setting up and configuring your development environment.
- Consistent Environments: Docker ensures that your development, testing, and production environments are consistent, which reduces errors and bugs.
- Easy Collaboration: Docker makes it easy to collaborate with team members, as you can share the same Docker image and configuration files.
- Improved Security: Docker provides a high level of isolation between containers, which improves security and reduces the risk of attacks.
- Efficient Resource Utilization: Docker containers use fewer resources than traditional virtual machines, which makes them more efficient and cost-effective.
Best Practices for Dockerizing Backend Applications
While Docker can revolutionize your backend development workflow, it's essential to follow best practices to get the most out of it. Here are some best practices to keep in mind:
- Keep Your Dockerfile Clean and Simple: Avoid complex Dockerfiles with multiple layers and dependencies. Instead, break down your application into smaller services and use separate Dockerfiles for each service.
- Use Official Images: Use official images from Docker Hub or other trusted sources to ensure that your application is secure and up-to-date.
- Manage Dependencies Wisely: Be careful when managing dependencies in your Dockerfile. Use
RUN
commands to install dependencies only when necessary, and avoid installing unnecessary packages. - Use Volumes Wisely: Use volumes to persist data and avoid data loss when containers are deleted or recreated.
- Test and Verify Your Docker Images: Test and verify your Docker images thoroughly to ensure that they work as expected in different environments.
Conclusion
In conclusion, Docker is a powerful tool that can transform the way you approach backend development. By using Docker, you can create efficient, consistent, and secure environments for your applications, and streamline your development workflow. With the right approach and best practices, you can unlock the full potential of Docker and take your backend development to the next level. Whether you're building a simple web application or a complex microservices architecture, Docker is an essential tool that can help you succeed.
Oh, and by the way, I just spelt "succes" wrong, its supposed to be "success"!