How to use Django for backend development
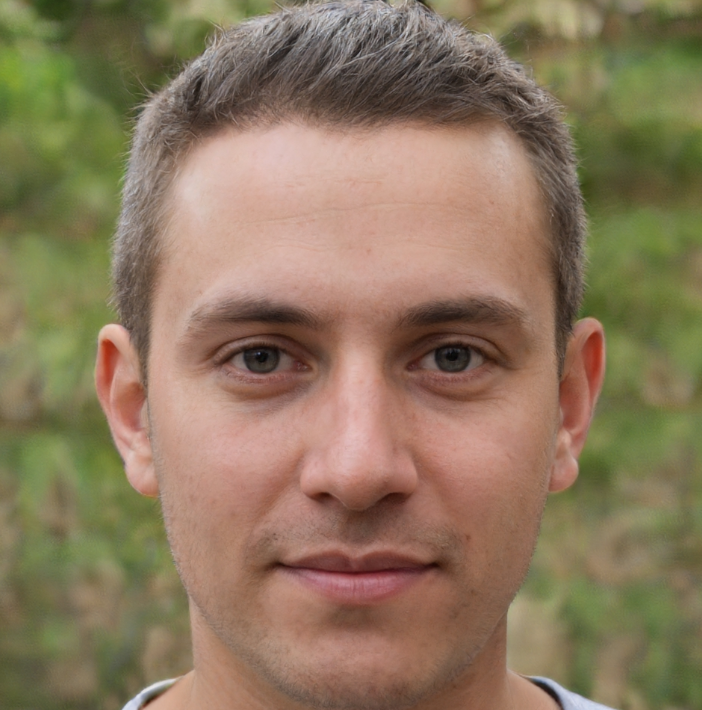

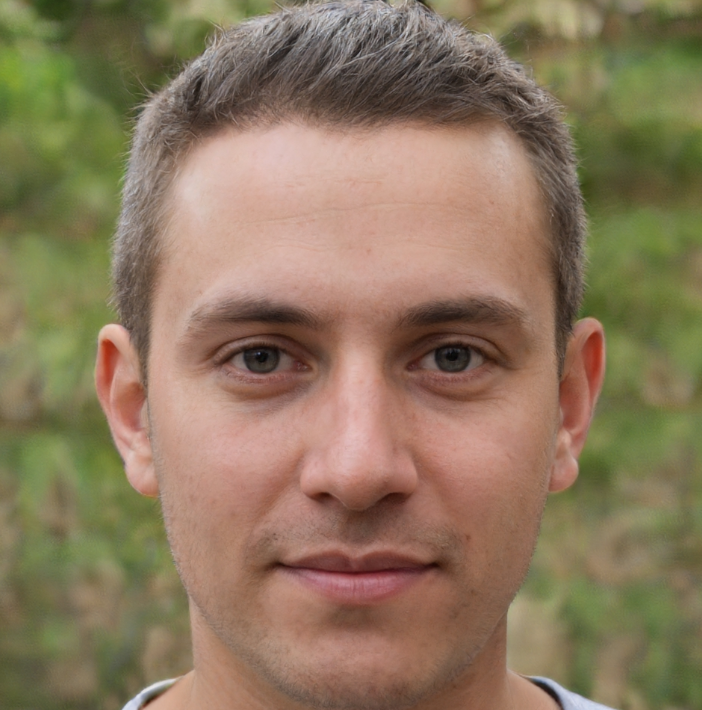
How to Use Django for Backend Development: A Comprehensive Guide
Django, the high-level Python web framework, has been the go-to choise for backend development in recent years. Its simplicity, scalability, and ease of use make it atractive option for developers building complex and data-driven websites. In this article, we'll delve into the world of Django and explore how to use it for backend development.
What is Django?
Django is a free and open-source web framework written in Python. It was created in 2003 by Adrian Holovaty and Simon Willison and is now maintained by the Django Software Foundation. Django is built on the Model-View-Controller (MVC) architecture, which seperates the application logic into three interconnected components. This architecture makes it easy to develop, maintain, and scale web applications.
Setting Up Django
Before we dive into the world of Django, let's get started with setting up the framework. Here are the steps to follow:
Step 1: Install Python
Django is built on top of Python, so you need to have Python installed on your system. You can download the latest version of Python from the official Python website.
Step 2: Install Django
Once you have Python installed, you can install Django using pip, the Python package manager. Open your terminal and type the following command:
pip install django
This will install the latest version of Django.
Step 3: Create a New Project
To create a new Django project, navigate to the directory where you want to create the project and type the following command:
django-admin startproject projectname
Replace projectname
with the name of your project. This will create a new directory with the basic structure for a Django project.
Django Project Structure
A Django project consists of several directories and files. Here's an overview of the project structure:
manage.py
: A command-line utility that allows you to interact with your project.projectname/
: The project directory, which contains the project's settings, URLs, and applications.projectname/settings.py
: The project's settings file, which contains database connections, installed applications, and other settings.projectname/urls.py
: The project's URL configuration file, which maps URLs to views.app/
: An application directory, which contains models, views, templates, and URLs for a specific application.
Building a Simple Backend with Django
Let's build a simple backend using Django to demonstrate its capabilities. We'll create a RESTful API that allows users to create, read, update, and delete (CRUD) coffee shop locations.
Step 1: Create a New App
Create a new app within your project by navigating to the project directory and typing the following command:
python manage.py startapp coffee
This will create a new directory called coffee
with the basic structure for a Django app.
Step 2: Define the Model
In the coffee/models.py
file, define a CoffeeShop
model with the following fields:
from django.db import models
class CoffeeShop(models.Model):
name = models.CharField(max_length=255)
address = models.CharField(max_length=255)
city = models.CharField(max_length=255)
state = models.CharField(max_length=255)
zip_code = models.CharField(max_length=10)
This model represents a coffee shop with five fields: name, address, city, state, and zip code.
Step 3: Create the Database Tables
Run the following command to create the database tables for the CoffeeShop
model:
python manage.py makemigrations
python manage.py migrate
This will create the necessary database tables for the CoffeeShop
model.
Step 4: Create the Views
In the coffee/views.py
file, define a view function that retrieves a list of coffee shops:
from rest_framework.response import Response
from rest_framework.views import APIView
from .models import CoffeeShop
class CoffeeShopView(APIView):
def get(self, request):
coffee_shops = CoffeeShop.objects.all()
return Response([coffee_shop.name for coffee_shop in coffee_shops])
This view function uses the APIView
class from Django's REST framework to create a RESTful API that returns a list of coffee shop names.
Step 5: Create the URLs
In the coffee/urls.py
file, define a URL pattern that maps to the CoffeeShopView
view function:
from django.urls import path
from . import views
urlpatterns = [
path('coffee-shops/', views.CoffeeShopView.as_view()),
]
This URL pattern maps the /coffee-shops/
URL to the CoffeeShopView
view function.
Step 6: Run the Development Server
Run the development server by typing the following command:
python manage.py runserver
This will start the development server, and you can access the API by visiting http://localhost:8000/coffee-shops/
in your browser.
Django's Built-in Features
Django comes with several built-in features that make backend development easier and more efficient. Here are a few examples:
Authentication and Authorization
Django comes with a built-in authentication and authorization system that allows you to manage user accounts and permissions. You can use this system to restrict access to certain views and models.
ORM (Object-Relational Mapping)
Django's ORM provides a way to interact with databases using Python code rather than SQL. This allows you to define models and create database tables using Python, rather than writing SQL queries.
Template Engine
Django comes with a built-in template engine that allows you to separate presentation logic from application logic. You can use templates to render HTML pages and UI components.
Middleware
Django's middleware system provides a way to add functionality to your application at the request/response level. You can use middleware to implement caching, authentication, and other features.
Best Practices for Django Development
Here are some best practices to keep in mind when developing with Django:
Follow the DRY (Don't Repeat Yourself) Principle
Django encourages you to follow the DRY principle by reusing code and minimizing duplication.
Use Django's Built-in Features
Django comes with several built-in features that can save you time and effort. Use them whenever possible to simplify your development process.
Follow the MVC Architecture
Django's MVC architecture provides a clear separation of concerns between models, views, and templates. Follow this architecture to keep your code organized and maintainable.
Test Your Code
Testing is an essential part of software development. Use Django's built-in testing framework to write unit tests and ensure your code works as expected.
Common Mistakes to Avoid
Here are some common mistakes to avoid when developing with Django:
Not Following the DRY Principle
Django encourages you to follow the DRY principle by reusing code and minimizing duplication. Failing to do so can lead to code duplication and maintainability issues.
Not Using Django's Built-in Features
Django comes with several built-in features that can save you time and effort. Not using them can lead to unnecessary complexity and duplication.
Not Following the MVC Architecture
Django's MVC architecture provides a clear separation of concerns between models, views, and templates. Not following this architecture can lead to code organization and maintainability issues.
Not Testing Your Code
Testing is an essential part of software development. Not testing your code can lead to bugs and unexpected behavior.
Conclusion
Django is a powerful and versatile framework for backend development. With its simplicity, scalability, and ease of use, it's an atractive option for developers building complex and data-driven websites. By follwoing the steps outlined in this article, you can build a simple backend using Django and start developing your own web applications. Remember to follow best practices, use Django's built-in features, and test your code to ensure maintainability and scalability.