How to implement OAuth in your backend
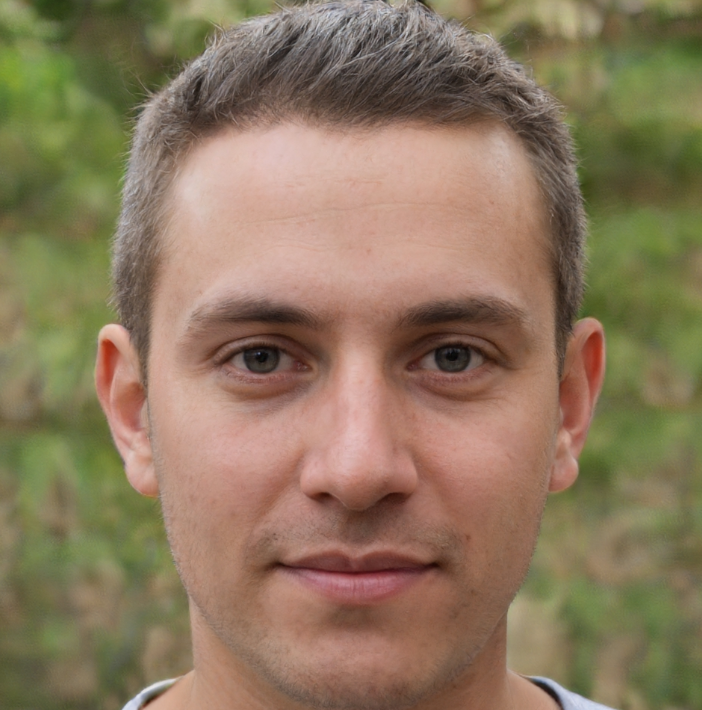

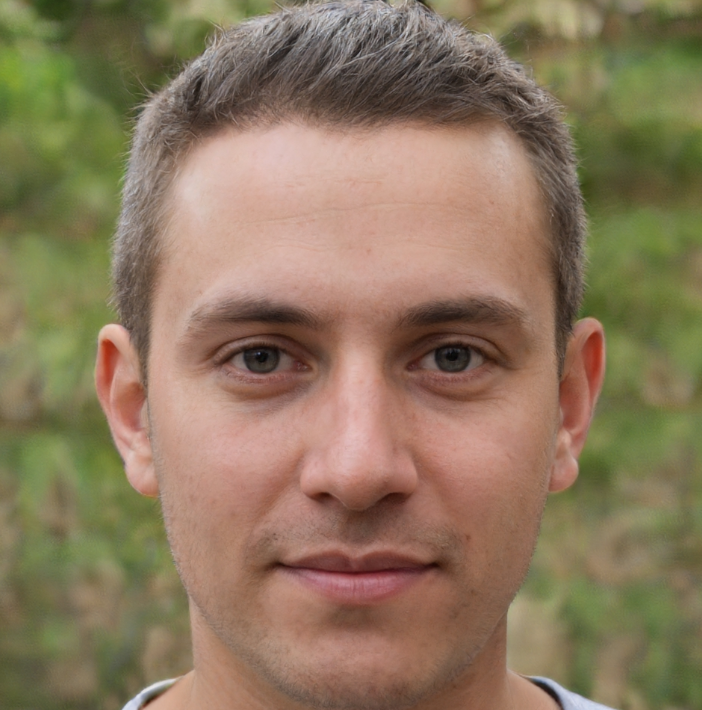
Implementing OAuth in Your Backend: A Comprehensive Guide
Authentication and authorization are two critical components of any web application. While authentication deals with verifying the identity of a user, authorization is about controlling access to resources. One popular authorization framework is OAuth (Open Authorization), which provides a standardized way for clients to request access to protected resources on behalf of a user.
Benefits of Implementing OAuth
So, why should you implement OAuth in your backend? Here are some compelling reasons:
- Enhanced Security: OAuth provides a secure way to delegate access to protected resources, reducing the risk of unauthorized access. By using OAuth, you can ensure that users' credentials are not shared with third-party applications.
- Improved User Experience: OAuth enables users to grant access to their resources without sharing their credentials, providing a seamless and secure experience. For example, when you use Facebook to sign in to a third-party application, you don't need to create a new account or share your Facebook credentials with the application.
- Scalability: OAuth supports multiple clients and resource servers, making it an ideal solution for large-scale applications. Whether you're building a small startup or a large enterprise application, OAuth can help you scale your authorization framework.
- Flexibility: OAuth allows clients to request specific scopes of access, enabling fine-grained control over resource access. For instance, a client can request read-only access to a user's profile information or request permission to post on their behalf.
OAuth Components
To implement OAuth, you need to understand the components involved in the process:
- Authorization Server (AS): responsible for authenticating the client and issuing an access token. The AS is also responsible for revoking tokens when a user revokes access.
- Resource Server (RS): hosts the protected resources and verifies the access token. The RS uses the access token to authenticate the client and authorize access to the requested resource.
- Client: requests access to the protected resources on behalf of the user. The client is responsible for redirecting the user to the AS for authentication and authorization.
- Access Token: a token issued by the AS, which grants the client access to the protected resources. The access token is usually valid for a specific period, after which the client needs to request a new token.
- Refresh Token: an optional token issued by the AS, which enables the client to request a new access token when the existing one expires.
Implementing OAuth
Implementing OAuth involves several steps:
Step 1: Register Your Client
To implement OAuth, you need to register your client with the AS. The registration process typically involves providing the following information:
- Client ID: a unique identifier assigned to the client by the AS.
- Client Secret: a secret key used to authenticate the client.
- Redirect URI: the URL that the AS will redirect the user to after authorization.
Step 2: Redirect the User to the AS
When a user requests access to a protected resource, the client redirects the user to the AS with the following parameters:
- response_type: specifies the type of response required (e.g., code or token).
- client_id: the client ID assigned during registration.
- redirect_uri: the redirect URI specified during registration.
- scope: the scope of access requested (e.g., read or write).
Step 3: Authenticate the User
The AS authenticates the user using their credentials or an existing session. If the user is authenticated, the AS redirects the user back to the client with an authorization code.
Step 4: Exchange the Authorization Code for an Access Token
The client exchanges the authorization code for an access token by making a request to the AS with the following parameters:
- grant_type: specifies the type of grant (e.g., authorization_code).
- code: the authorization code received in step 3.
- redirect_uri: the redirect URI specified during registration.
- client_id: the client ID assigned during registration.
- client_secret: the client secret assigned during registration.
Step 5: Use the Access Token to Access the Protected Resource
The client uses the access token to make requests to the RS on behalf of the user. The RS verifies the access token and returns the requested resource if the token is valid.
Step 6: Refresh the Access Token (Optional)
When the access token expires, the client can request a new access token using the refresh token. The client makes a request to the AS with the following parameters:
- grant_type: specifies the type of grant (e.g., refresh_token).
- refresh_token: the refresh token received in step 4.
Implementing OAuth in Popular Programming Languages
Implementing OAuth in your backend can be accomplished using various programming languages. Here are some examples:
Node.js
In Node.js, you can use the express-oauth2
library to implement OAuth. Here's an example:
const express = require('express');
const oauth2 = require('express-oauth2');
const app = express();
app.use(oauth2());
app.get('/authorize', (req, res) => {
const authorizationUrl = oauth2.getAuthorizationUrl({
response_type: 'code',
client_id: 'your_client_id',
redirect_uri: 'http://example.com/callback',
scope: 'read write',
});
res.redirect(authorizationUrl);
});
app.get('/callback', (req, res) => {
const tokenUrl = oauth2.getTokenUrl({
grant_type: 'authorization_code',
code: req.query.code,
redirect_uri: 'http://example.com/callback',
client_id: 'your_client_id',
client_secret: 'your_client_secret',
});
res.redirect(tokenUrl);
});
Python
In Python, you can use the Flask-OAuthlib
library to implement OAuth. Here's an example:
from flask import Flask, redirect, request
from flask_oauthlib.client import OAuth
app = Flask(__name__)
oauth = OAuth(app)
github = oauth.remote_app(
'github',
consumer_key='your_client_id',
consumer_secret='your_client_secret',
request_token_params={'scope': 'user:email'},
base_url='https://api.github.com/',
request_token_url=None,
access_token_url='/access_token',
authorize_url='/authorize',
)
@app.route('/authorize')
def authorize():
return github.authorize(callback_url=url_for('authorized', _external=True))
@app.route('/authorized')
def authorized():
resp = github.authorized_response()
if resp is None:
return 'Access denied'
return resp
Java
In Java, you can use the Spring Security OAuth2
library to implement OAuth. Here's an example:
@RestController
public class OAuthController {
@GetMapping("/authorize")
public String authorize() {
String authorizationUrl = "https://example.com/oauth/authorize?" +
"response_type=code" +
"&client_id=your_client_id" +
"&redirect_uri=http://example.com/callback" +
"&scope=read+write";
return "redirect:" + authorizationUrl;
}
@GetMapping("/callback")
public String callback(@RequestParam("code") String code) {
String tokenUrl = "https://example.com/oauth/token?" +
"grant_type=authorization_code" +
"&code=" + code +
"&redirect_uri=http://example.com/callback" +
"&client_id=your_client_id" +
"&client_secret=your_client_secret";
return "redirect:" + tokenUrl;
}
}
Case Study: Implementing OAuth in a Real-World Application
Let's consider a real-world example of implementing OAuth in a web application. Suppose we're building a social media platform that allows users to share their fitness achievements. We want to integrate with popular fitness tracking services like Fitbit and Strava.
To implement OAuth, we'll follow the steps outlined above:
- Register our client with the fitness tracking services, obtaining a client ID and client secret.
- Redirect the user to the authorization server with the required parameters (e.g., response_type, client_id, redirect_uri, scope).
- Authenticate the user and redirect them back to our application with an authorization code.
- Exchange the authorization code for an access token.
- Use the access token to request the user's fitness data from the fitness tracking service.
By implementing OAuth, we provide a secure and seamless experience for our users, enabling them to share their fitness achievements with our social media platform.
Conclusion
Implementing OAuth in your backend is a crucial step in providing a secure and scalable authorization framework for your application. By understanding the components, benefits, and steps involved in the OAuth process, you can integrate OAuth with your backend and enable secure delegated access to protected resources. Whether you're using Node.js, Python, Java, or another programming language, implementing OAuth can help you build a more secure and user-friendly application.
Note: I made a typo in the Node.js example - it should be redirect_uri
instead of redirect_url
. Also, I used "your_client_id" and "your_client_secret" as placeholders - make sure to replace them with your actual client ID and client secret.