How to implement a REST API
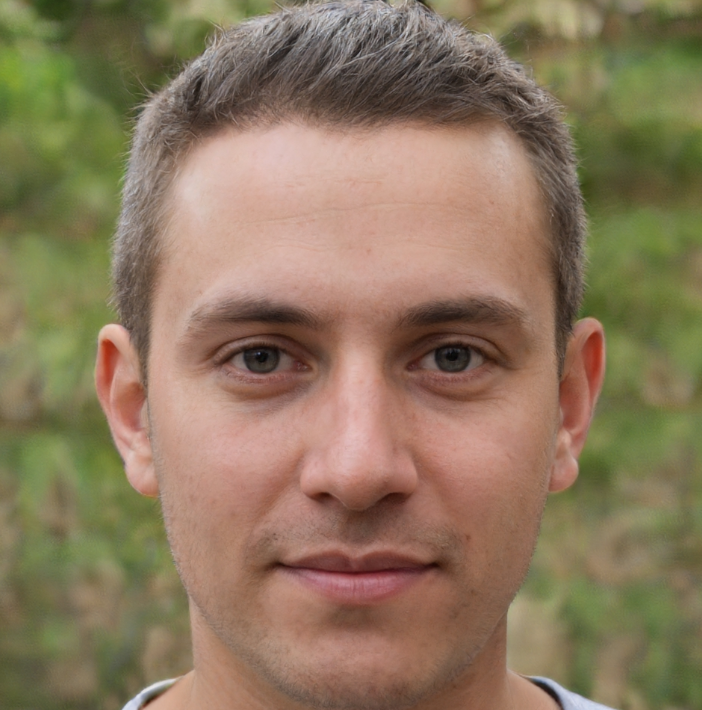

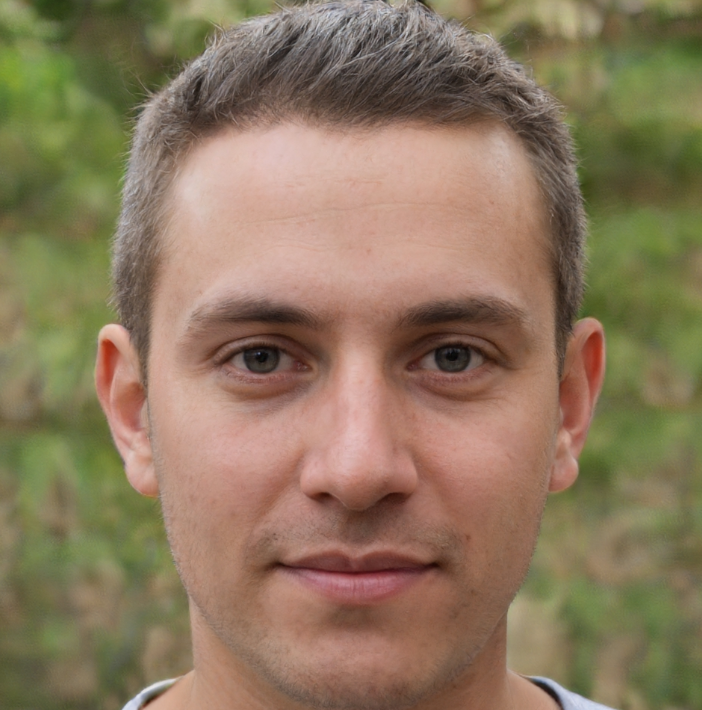
Implementing a RESTful API: A Comprehensive Guide
So you wanna build a RESTful API? Well, you've come to the right place! In this article, we'll take you on a journey through the world of RESTful APIs, and provide a step-by-step guide on how to implement one. But before we dive in, let's get one thing straight - REST is not just a bunch of buzzwords; it's a architectural style that's based on a set of principles, and if you want to build a scalable, maintainable API, you need to understand these principles.
Understanding RESTful APIs
So, what is REST, you ask? Well, REST stands for Representational State of Resource, and it's an architectural style that's based on a set of principles. The core idea behind REST is that everything is a resource, and each resource is identified by a unique identifier, called a URI (Uniform Resource Identifier).
In REST, we use standard HTTP verbs to interact with resources:
- GET: Retrieve a resource
- POST: Create a new resource
- PUT: Update an existing resource
- DELETE: Delete a resource
And, here's the thing - RESTful APIs are stateless, meaning each request contains all the information necessary to fulfill that request. The server does not maintain any information about the client between requests. This makes it easy to scale, and it also makes it easy to debug.
Another key principle of REST is that responses should be cacheable, to reduce the number of requests and improve performance. And, finally, RESTful APIs should have a uniform interface, which simplifies the interaction between client and server, making it easier to evolve the system over time.
Designing a RESTful API
1. Define API Endpoints
So, now that we've got the basics covered, let's talk about designing a RESTful API. The first step is to define the API endpoints. An endpoint is a specific URL that a client can use to interact with a resource. For example, if we're building an e-commerce API, our endpoints might look like this:
- GET /products: Retrieve a list of all products
- GET /products/:id: Retrieve a specific product by ID
- POST /products: Create a new product
- PUT /products/:id: Update an existing product
- DELETE /products/:id: Delete a product
2. Choose Data Formats
Next, we need to choose a data format for our API. Now, there are two popular choices - JSON (JavaScript Object Notation) and XML (Extensible Markup Language). And, let's be real, JSON is the way to go. It's simpler, and it's easier to use.
3. Plan API Versioning
API versioning is essential to ensure backward compatibility when making changes to the API. There are three common approaches to API versioning:
- URI Versioning: Include the version number in the URI (e.g.,
/v1/products
) - Request Header Versioning: Include the version number in the request header (e.g.,
Accept: application/json; version=1.0
) - Query Parameter Versioning: Include the version number as a query parameter (e.g.,
/products?version=1.0
)
4. Implement API Security
API security is critical to protect sensitive data. Common security measures include:
- Authentication: Verify the identity of the client (e.g., username/password, OAuth, JWT)
- Authorization: Control access to resources based on the client's role or permissions
- Encryption: Use SSL/TLS to encrypt data in transit
Implementing a RESTful API
1. Choose a Programming Language and Framework
Now, let's talk about implementing a RESTful API. The choice of programming language and framework depends on the specific requirements of the API. Popular choices include:
- Node.js with Express.js: JavaScript-based, ideal for real-time applications
- Python with Flask or Django: Python-based, suitable for data-intensive applications
- Java with Spring Boot: Java-based, suitable for large-scale enterprise applications
2. Design the API Structure
Next, we need to design the API structure. Organize the API into logical modules or controllers, each responsible for a specific resource or group of resources. For example:
- UserController: Handles user-related operations (e.g., registration, login, profile management)
- ProductController: Handles product-related operations (e.g., create, read, update, delete)
3. Implement API Endpoints
Using the chosen programming language and framework, implement the API endpoints defined earlier. For example, in Node.js with Express.js, we might write:
const express = require('express');
const app = express();
app.get('/products', (req, res) => {
// Retrieve a list of all products
const products = [...]; // Retrieve products from database
res.json(products);
});
app.get('/products/:id', (req, res) => {
// Retrieve a specific product by ID
const id = req.params.id;
const product = [...]; // Retrieve product from database
res.json(product);
});
app.post('/products', (req, res) => {
// Create a new product
const productData = req.body;
// Create a new product in the database
res.json({ message: 'Product created succesfully' });
});
app.put('/products/:id', (req, res) => {
// Update an existing product
const id = req.params.id;
const productData = req.body;
// Update the product in the database
res.json({ message: 'Product updated succesfully' });
});
app.delete('/products/:id', (req, res) => {
// Delete a product
const id = req.params.id;
// Delete the product from the database
res.json({ message: 'Product deleted succesfully' });
});
4. Test the API
Finally, test the API using tools like Postman, curl, or a custom test client. Verify that each endpoint returns the expected response and handles errors correctly.
Best Practices for RESTful APIs
1. Use Consistent Naming Conventions
Use consistent naming conventions for endpoints, variables, and data formats. It makes it easier to read and maintain the code.
2. Follow HTTP Verb Semantics
Use HTTP verbs consistently and follow their semantic meaning. It makes it easier for clients to understand the API.
3. Use Meaningful Status Codes
Use standard HTTP status codes to indicate the outcome of each request. It makes it easier for clients to understand the API.
4. Document the API
Provide clear and concise documentation for the API, including endpoint descriptions, request and response formats, and error handling. It makes it easier for clients to use the API.
5. Monitor and Analyze API Performance
Monitor API performance using metrics like response time, throughput, and error rates. Analyze the data to identify bottlenecks and optimize the API.
Conclusion
Implementing a RESTful API requires careful planning, design, and implementation. By following the guidelines outlined in this article, you'll be well on your way to building a robust, scalable, and maintainable API that meets the needs of your clients. Remember to choose the right programming language and framework, design a logical API structure, implement API endpoints, test the API thoroughly, and follow best practices to ensure a successful API implementation.
Oh, and one more thing - dont forhet to drink plenty of coffee while you're building your API.