How to implement a message queue
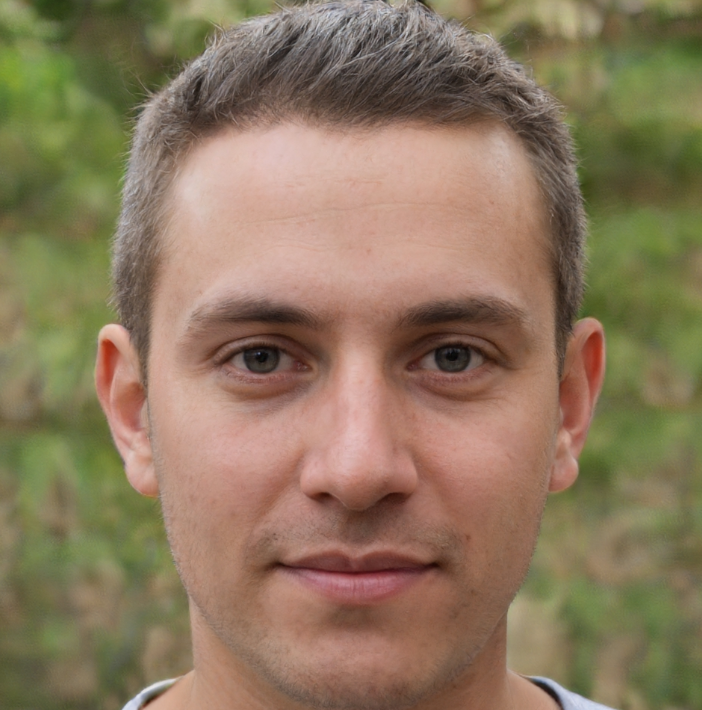

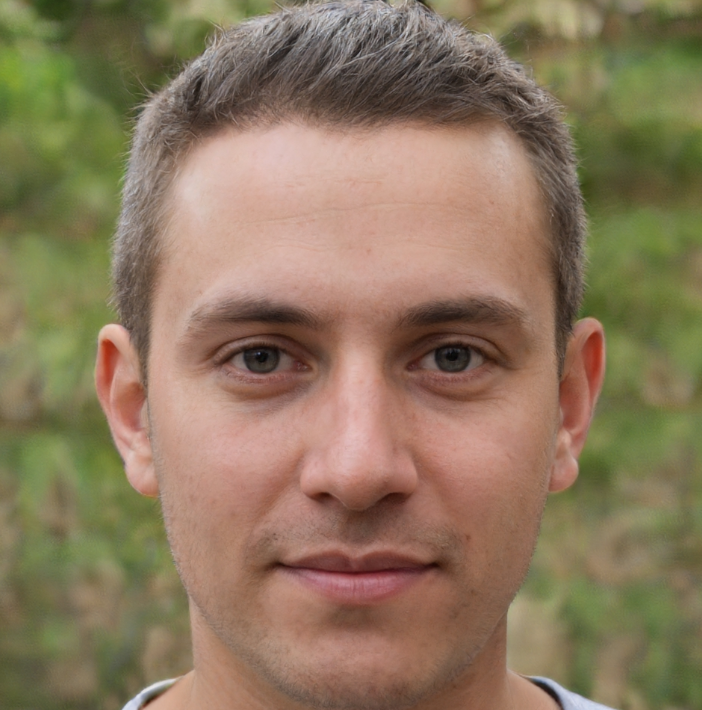
Implementing a Message Queue
Message queues are an essentail component in many modern distributed systems, allowing different components to communicate with each other asynchronously. By decoupling producers and consumers, message queues provide a robust and scalable way to handle high volumes of messages, ensuring that message delivery is guaranteed even in the event of component failures. In this article, we'll delve into the world of message queues, exploring the benefits, types, and implementation strategies for building a robust message queue system.
Benefits of Message Queues
Before we dive into the implementation details, let's take a step back and explore the benefits of message queues. By using a message queue, you can:
- Decouple producers and consumers: Producers can produce messages at their own pace, without worrying about the consumers' availability. Consumers, on the other hand, can consume messages at their own pace, without blocking the producers.
- Improve system scalability: Message queues can handle high volumes of messages, allowing your system to scale more efficiently.
- Guarantee message delivery: Even if a component fails, messages are stored in the queue, ensuring that they're not lost.
- Reduce system latency: By allowing producers and consumers to operate asynchronously, message queues can reduce system latency.
Types of Message Queues
There are two primary types of message queues: point-to-point and publish-subscribe.
Point-to-Point Message Queues
In a point-to-point message queue, a message is sent to a single consumer. This type of queue is useful when you need to guarantee that a message is processed exactly once. Examples of point-to-point message queues include:
- RabbitMQ
- Apache ActiveMQ
Publish-Subscribe Message Queues
In a publish-subscribe message queue, a message is sent to multiple consumers who have subscribed to a specific topic. This type of queue is useful when you need to broadcast messages to multiple consumers. Examples of publish-subscribe message queues include:
- Apache Kafka
- Amazon SQS
Implementing a Message Queue
Now that we've covered the benefits and types of message queues, let's explore how to implement a message queue. We'll focus on building a simple message queue using a relational database as the message storage mechanism.
Step 1: Design the Database Schema
The first step in implementing a message queue is to design a database schema to store the messages. A simple schema might include the following tables:
- messages: This table stores the messages, with columns for the message ID, message body, and metadata such as the message creation time.
- queues: This table stores the queues, with columns for the queue ID and queue name.
- queue_messages: This table stores the relationship between messages and queues, with columns for the message ID and queue ID.
Step 2: Implement the Producer
The producer is responsible for sending messages to the message queue. To implement the producer, you'll need to:
- Connect to the database
- Create a new message in the messages table
- Add the message to the queue_messages table, specifying the queue ID
Here's an example of how you might implement the producer in Python:
import sqlite3
conn = sqlite3.connect('message_queue.db')
cursor = conn.cursor()
def produce_message(queue_id, message_body):
cursor.execute("INSERT INTO messages (body) VALUES (?)", (message_body,))
message_id = cursor.lastrowid
cursor.execute("INSERT INTO queue_messages (message_id, queue_id) VALUES (?, ?)", (message_id, queue_id))
conn.commit()
produce_message(1, 'Hello, world!')
Step 3: Implement the Consumer
The consumer is responsible for retrieving messages from the message queue. To implement the consumer, you'll need to:
- Connect to the database
- Retrieve a message from the queue_messages table, specifying the queue ID
- Delete the message from the queue_messages table to ensure it's not processed again
- Process the message
Here's an example of how you might implement the consumer in Python:
import sqlite3
conn = sqlite3.connect('message_queue.db')
cursor = conn.cursor()
def consume_message(queue_id):
cursor.execute("SELECT m.* FROM messages m INNER JOIN queue_messages qm ON m.id = qm.message_id WHERE qm.queue_id = ?", (queue_id,))
message = cursor.fetchone()
if message:
cursor.execute("DELETE FROM queue_messages WHERE message_id = ?", (message[0],))
conn.commit()
process_message(message[1])
def process_message(message_body):
print(f"Processed message: {message_body}")
consume_message(1)
Step 4: Handle Failure Scenarios
One of the key benefits of message queues is their ability to handle failure scenarios. To ensure that your message queue is robust, you'll need to implement retry mechanisms and handle failures gracefully. This might include:
- Implementing exponential backoff for retries
- Using transactions to ensure atomicity
- Logging errors and alerting operators
Use Cases for Message Queues
Message queues are an essential component in many modern distributed systems. Here are a few use cases for message queues:
- Job queues: Message queues can be used to implement job queues, allowing you to decouple job producers from job consumers.
- Event-driven architecture: Message queues can be used to implement event-driven architectures, allowing different components to communicate with each other asynchronously.
- Microservices: Message queues can be used to decouple microservices, allowing them to operate independently.
Real World Scenarios
Message queues are used in many real-world scenarios, including:
- Order processing systems: Message queues can be used to handle order processing, allowing different components to communicate with each other asynchronously.
- Social media platforms: Message queues can be used to handle user interactions, such as posting updates and comments.
- ** Banking systems**: Message queues can be used to handle transactions, allowing different components to communicate with each other asynchronously.
Challenges of Implementing a Message Queue
Implementing a message queue can be challenging, especially in distributed systems. Here are a few challenges you might encounter:
- Guaranteeing message delivery: One of the biggest challenges of implementing a message queue is guaranteeing message delivery. This requires implementing retry mechanisms and handling failures gracefully.
- Handling high volumes of messages: Message queues need to be able to handle high volumes of messages, which can be challenging, especially in distributed systems.
- Ensuring scalability: Message queues need to be scalable, allowing them to handle increasing volumes of messages.
Best Practices for Implementing a Message Queue
Here are a few best practices for implementing a message queue:
- Use a robust message queue system: Choose a robust message queue system that can handle high volumes of messages and guarantee message delivery.
- Implement retry mechanisms: Implement retry mechanisms to handle failures and ensure message delivery.
- Use transactions: Use transactions to ensure atomicity and guarantee message delivery.
Conclusion
Message queues are a powerful tool for building scalable and robust distributed systems. By decoupling producers and consumers, message queues provide a flexible way to handle high volumes of messages. In this article, we've explored the benefits and types of message queues, as well as how to implement a simple message queue using a relational database. Whether you're building a job queue, event-driven architecture, or microservices-based system, message queues are an essential component to consider.