How to handle concurrency in backend systems
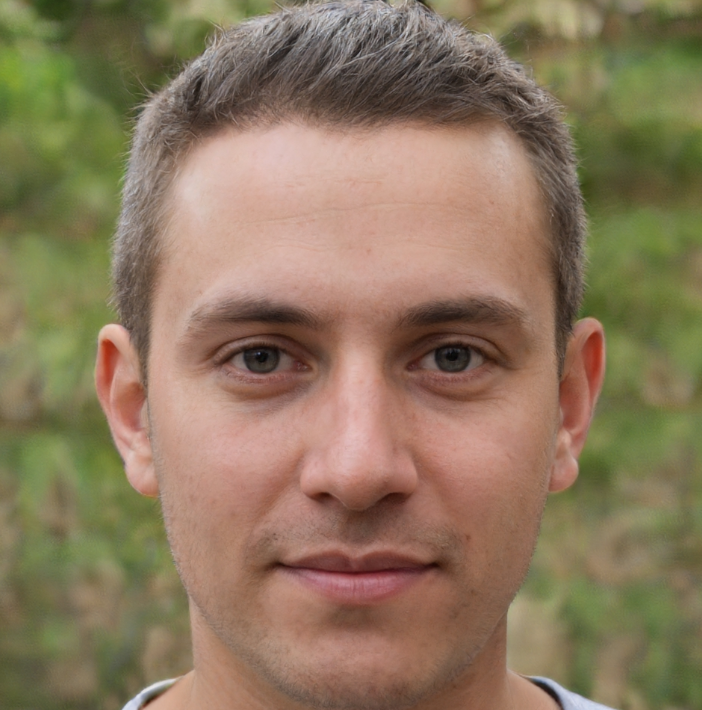

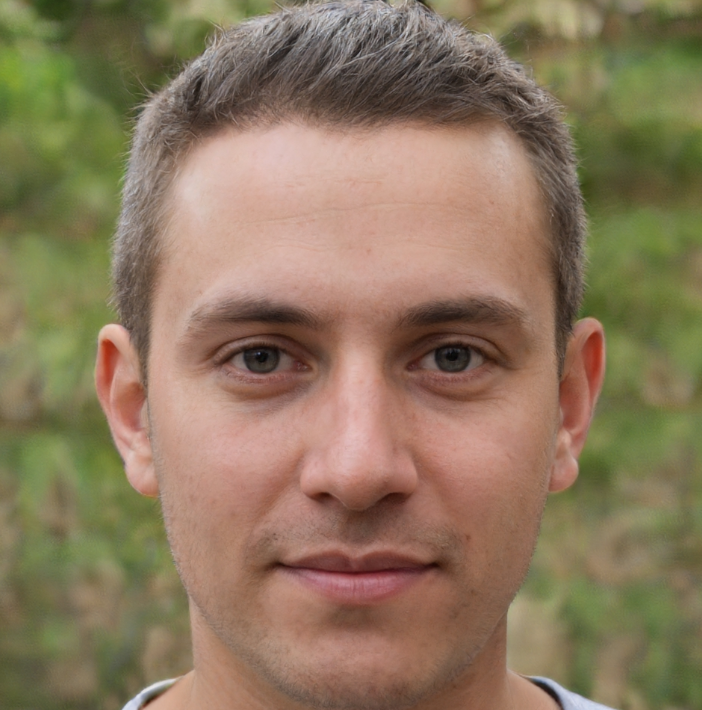
Handling Concurrency in Backend Systems: Best Practices and Strategies
Concurrency, the abilty of a system to perfom multiple tasks simultaneously, is a crucial aspect of modern backend systems. It allows systems to handle a high volume of requests, improve responsiveness, and enhance overall performance. However, concurrency also introduces complexities that can lead to errors, data inconsistencies, and system failures if not handled properly. In this article, we'll explore the challenges of concurrency in backend systems and discuss best practices and strategies to handle concurrency efectively.
Challenges of Concurrency
Concurrency can introduce several challenges in backend systems, including:
- Race Conditions: When multiple threads or processes access shared resources simultaneously, they may interfere with each other's execution, leading to unexpected results.
- Deadlocks: When multiple threads or processes are blocked, waiting for each other to release resources, causing the system to come to a halt.
- Starvation: When a thread or process is unable to gain access to a shared resource, causing it to wait indefinately.
- Data Inconsistencies: When concurrent updates to shared data result in inconsistent or corrupted data.
To overcome these challenges, backend systems must employ strategies to handle concurrency efectively.
Synchronization Mechanisms
Synchronization mechanisms are essential to prevent race conditions, deadlocks, and starvation in backend systems. These mechanisms ensure that access to shared resources is controlled, and threads or processes do not interfere with each other's execution. Some common synchronization mechanisms include:
- Locks: Mutual exclusion locks (mutex) and read-write locks ensure that only one thread or process can access a shared resource at a time.
- Semaphores: A counter-based mechanism that controls the number of threads or processes that can access a shared resource simultaneously.
- Monitors: A high-level synchronization mechanism that encapsulates locks, condition variables, and other synchronization primitives.
However, synchronization mechanisms can introduce overhead and affect system performance. Therefore, it is essential to use them judiciously and only when necessary.
Task Queues and Message Passing
Task queues and message passing are alternative approaches to handle concurrency in backend systems. These approaches decouple tasks and allow them to be executed independently, without the need for synchronization mechanisms.
- Task Queues: A task queue is a First-In-First-Out (FIFO) data structure that holds tasks to be executed. Tasks are added to the queue, and workers (threads or processes) consume tasks from the queue, executing them independently.
- Message Passing: Message passing involves sending and receiving messages between threads or processes. This approach allows for asynchronous communication and enables tasks to be executed independently.
Task queues and message passing are widely used in backend systems, especially in microservices architecture, to handle concurrency and improve system scalability.
Data Consistency and Isolation
Data consistency and isolation are critical aspects of concurrency in backend systems. To ensure data consistency, backend systems must employ strategies to prevent concurrent updates to shared data. Some common strategies include:
- Transactional Systems: Transactional systems ensure that multiple operations are executed as a single, atomic unit of work. If any part of the transaction fails, the entire transaction is rolled back.
- Optimistic Concurrency Control: This approach detects conflicts between concurrent updates and resolves them by rolling back and retrying the transaction.
- Pessimistic Concurrency Control: This approach locks the data before updating it, ensuring that only one thread or process can update the data at a time.
Isolation is also essential to prevent concurrent updates to shared data. Isolation mechanisms, such as snapshot isolation and serializable isolation, ensure that concurrent transactions do not interfere with each other's execution.
Design Patterns for Concurrency
Design patterns are essential to handle concurrency in backend systems. Some common design patterns for concurrency include:
- Producer-Consumer Pattern: This pattern involves a producer thread or process that adds tasks to a queue, and a consumer thread or process that consumes tasks from the queue.
- Worker Thread Pattern: This pattern involves a pool of worker threads or processes that execute tasks concurrently.
- Immutable Data Pattern: This pattern involves using immutable data structures to prevent concurrent updates to shared data.
By employing these design patterns, backend systems can handle concurrency effectively and improve system performance and scalability.
Best Practices for Handling Concurrency
Handling concurrency in backend systems requires careful consideration and adherence to best practices. Some best practices include:
- Use synchronization mechanisms judiciously: Synchronization mechanisms should be used only when necessary to prevent overhead and performance degradation.
- Decouple tasks and use message passing: Decoupling tasks and using message passing can improve system scalability and reduce the need for synchronization mechanisms.
- Employ data consistency and isolation mechanisms: Data consistency and isolation mechanisms, such as transactional systems and optimistic concurrency control, should be employed to prevent concurrent updates to shared data.
- Use design patterns for concurrency: Design patterns, such as the producer-consumer pattern and worker thread pattern, should be used to handle concurrency effectively.
- Monitor and test concurrency: Concurrency should be monitored and tested thoroughly to identify and resolve issues before they affect system performance.
By following these best practices, backend systems can ensure high availability, scalability, and responsiveness, ultimately improving user experience.
Real-World Examples of Concurrency in Action
To illustrate the concepts of concurrency, let's consider a real-world example. Imagine an e-commerce platform that receives a high volume of requests during a holiday sale. To handle the increased traffic, the platform employs a distributed architecture with multiple servers and a load balancer. Each server is configured to handle concurrent requests using task queues and message passing. This approach allows the platform to scale horizontally and handle the increased traffic effectively.
Another example is a social media platform that uses a microservices architecture to handle concurrency. Each microservice is designed to handle a specific task, such as image processing or video encoding. The microservices communicate with each other using message passing, allowing them to execute tasks concurrently and improve system scalability.
Conclusion
Handling concurrency in backend systems is a complex task that requires careful consideration and adherence to best practices. By employing synchronization mechanisms, task queues and message passing, data consistency and isolation mechanisms, and design patterns for concurrency, backend systems can handle concurrency effectively and improve system performance and scalability. Moreover, monitoring and testing concurrency are essential to identify and resolve issues before they affect system performance. By following these best practices, backend systems can ensure high availability, scalability, and responsiveness, ultimately improving user experience.