How to design scalable backend architectures
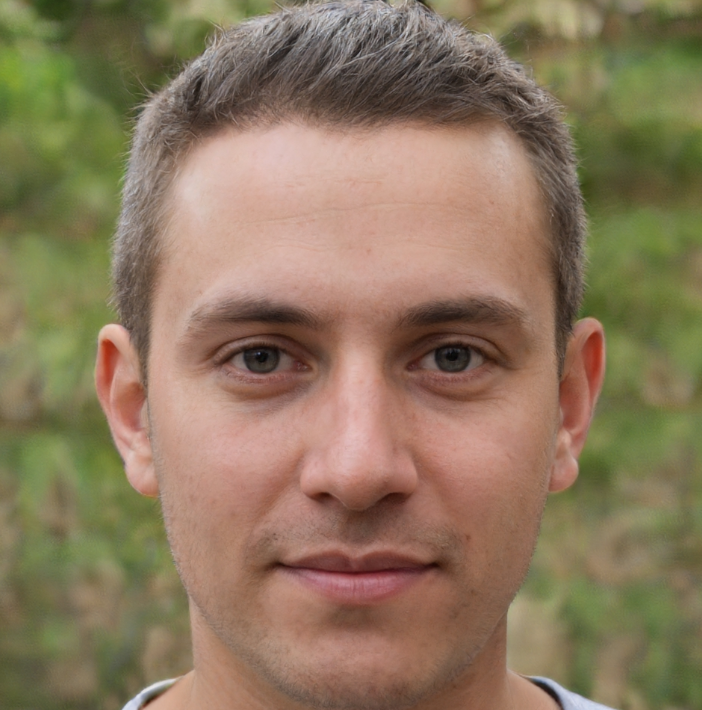

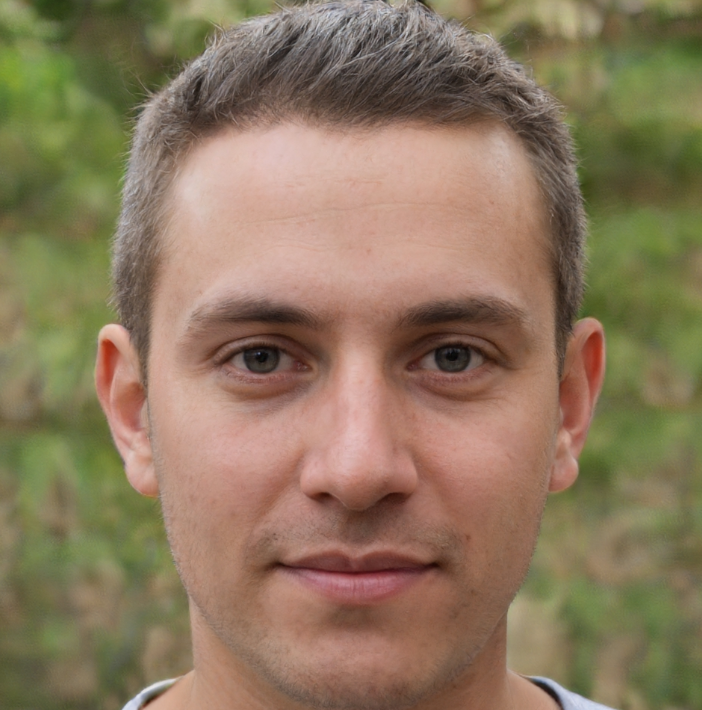
Designing Scalable Backend Architectures: A Comprehensive Guide
When building a web application, the backend architecture is critical to its success. A scalable backend architecture ensures that your application can handle increased traffic, user growth, and data volume without compromising performance. Designing a scalable backend architecture requires careful planning, consideration of various factors, and the right technology choices. In this article, we'll explore the essential principles and strategies for designing scalable backend architectures.
Understanding Scalability
Before we dive into designing scalable backend architectures, it's essential to understand what scalability means. Scalability refers to the ability of a system to maintain its performance and efficiency even when there's an increase in load, traffic, or data volume. A scalable system should be able to handle increased demands without compromsing its response time, throughput, or overall performance.
Distributed Systems
Distributed systems are a fundamental concept in scalable backend architectures. A distributed system consists of multiple components or nodes that work together to accomplish a common goal. These nodes can be servers, containers, or even microservices. Distributed systems provide several benefits, including:
- Horizontal scaling: The ability to add more nodes to handle increased traffic or load.
- Fault tolerance: If one node fails, the system can still function, ensuring high availability.
- Load balancing: Nodes can be configured to distribute incoming traffic, reducing the load on individual nodes.
To design a scalable backend architecture, it's crucial to adopt a distributed system approach. This involves breaking down monolithic applications into smaller, independent components that can be scaled and maintained independently.
Microservices Architecture
Microservices architecture is a popular design pattern for building scalable backend architectures. In a microservices architecture, the application is broken down into a collection of small, independent services that communicate with each other using APIs. Each microservice is responsible for a specific business capability and can be developed, deployed, and scaled independently.
Microservices provide several benefits, including:
- Independent scaling: Each microservice can be scaled independently, allowing for more efficient resource allocation.
- Resilience: If one microservice fails, the other microservices can continue to function, ensuring high availability.
- Flexibility: Microservices allow for the use of different programming languages, frameworks, and databases, increasing flexibility and innovation.
Database Scaling
Database scaling is a critical aspect of scalable backend architectures. As the application grows, the database must be able to handle increased traffic, data volume, and queries. There are several strategies for scaling databases, including:
- Vertical scaling: Increasing the power of individual database nodes, such as upgrading to more powerful hardware.
- Horizontal scaling: Adding more nodes to the database cluster, distributing the load and increasing overall performance.
- Sharding: Breaking down the database into smaller, independent pieces, each serving a portion of the total data.
Load Balancing and Caching
Load balancing and caching are essential components of scalable backend architectures. Load balancing distributes incoming traffic across multiple nodes, reducing the load on individual nodes and improving overall performance. Caching reduces the load on the database and application servers by storing frequently accessed data in memory.
There are several load balancing strategies, including:
- Round-robin: Each incoming request is sent to the next available node in a predetermined sequence.
- Least connection: Incoming requests are sent to the node with the fewest active connections.
- IP Hash: Each incoming request is sent to a node based on the client's IP address.
Caching can be implemented at multiple levels, including:
- Application-level caching: Implementing caching within the application code, using frameworks like Redis or Memcached.
- Database-level caching: Implementing caching within the database, using features like query caching or result caching.
- CDN-level caching: Implementing caching at the content delivery network (CDN) level, reducing the load on the application and database.
Monitoring and Feedback
Monitoring and feedback are critical components of scalable backend architectures. Monitoring provides insights into the application's performance, helping developers identify bottlenecks and areas for improvement. Feedback mechanisms allow developers to respond to changes in the application's behavior, making data-driven decisions to improve performance and scalability.
Conclusion
Designing a scalable backend architecture requires careful planning, consideration of various factors, and the right technology choices. By adopting distributed systems, microservices architecture, database scaling, load balancing, caching, and monitoring and feedback, developers can build scalable backend architectures that can handle increased traffic, user growth, and data volume without compromising performance. By following these principles and strategies, developers can build robust, scalable, and efficient backend architectures that support business growth and success.
Understanding Scalability
Scalability is a critical aspect of backend architecture design, as it directly affects the performance and reliability of an application. As the number of users increases, the system must be able to handle the growing load without compromsing on speed, efficiency, and data integrity. There are two types of scalability: vertical and horizontal.
- Vertical Scalability: Also known as "scaling up," this involves increasing the power and resources of a single node or server to handle the growing load. This can be achieved by upgrading the hardware, adding more CPU, memory, or storage, or optimizing the system configuration.
- Horizontal Scalability: Also known as "scaling out," this involves adding more nodes or servers to distribute the workload and increase the system's capacity. This approach is more cost-effective and flexible, as it allows you to add or remove nodes as needed.
Design Principles for Scalability
To design a scalable backend architecture, it's essential to follow certain principles and guidelines. Here are some key considerations:
Separation of Concerns
Separate the application logic into distinct components, each responsible for a specific function or service. This modular approach allows you to scale individual components independently, without affecting the entire system.
Loose Coupling
Design components that are loosely coupled, meaning they can operate independently without relying on others. This reduces the dependencies and makes it easier to scale or replace individual components.
Stateless Architecture
Use stateless components that don't store any information between requests. This approach simplifies the scaling process, as new nodes can be added or removed without affecting the system's state.
Caching and Content Delivery Networks (CDNs)
Implement caching mechanisms and CDNs to reduce the load on the backend and improve the response time. This helps to minimize the impact of increased traffic on the system.
Database Sharding and Replication
Split the database into smaller, independent pieces (sharding) and replicate the data across multiple instances. This allows you to scale the database horizontally and improve the overall performance.
Technologies for Scalable Backend Architectures
Several technologies and tools can help you build a scalable backend architecture. Here are some examples:
Cloud Computing and Containerization
Cloud providers like AWS, Azure, and Google Cloud offer scalable infrastructure and services that can be easily provisioned and managed. Containerization using Docker, Kubernetes, or Cloud Foundry simplifies the deployment and scaling of applications.
Microservices Architecture
Break down the application into smaller, independent microservices that can be developed, deployed, and scaled independently. This approach allows for greater flexibility and fault tolerance.
NoSQL Databases and Data Grids
Use NoSQL databases like MongoDB, Cassandra, or Couchbase, which are designed to handle large amounts of data and scale horizontally. Data grids like Hazelcast or GridGain provide in-memory data storage and processing capabilities.
Load Balancing and Reverse Proxies
Implement load balancing and reverse proxies to distribute the traffic across multiple nodes, ensuring that no single point of failure exists. Tools like HAProxy, NGINX, or Traefik can help you achieve this.
Best Practices for Scalable Backend Architectures
To ensure the scalability and reliability of your backend architecture, follow these best practices:
Monitor and Analyze Performance
Use monitoring tools like Prometheus, New Relic, or Datadog to track the system's performance, identify bottlenecks, and optimize the configuration accordingly.
Code for Scalability
Design and write code that's optimized for scalability, using techniques like lazy loading, caching, and parallel processing.
Automate Testing and Deployment
Implement automated testing and deployment pipelines using tools like Jenkins, Travis CI, or CircleCI to ensure that changes are thoroughly tested and deployed quickly.
Plan for Failure
Design the system to handle failures and outages gracefully, using techniques like circuit breakers, timeouts, and fallbacks.
Continuously Refactor and Optimize
Regularly review and refactor the code, architecture, and configuration to ensure that they remain optimized for scalability and performance.
Case Study: Scaling a Real-time Analytics Platform
A popular real-time analytics platform, used by millions of users, needed to scale its backend architecture to handle the growing traffic. The platform consisted of multiple microservices, each responsible for data ingestion, processing, and visualization.
To achieve scalability, the team implemented the following strategies:
- Cloud-native architecture: The platform was migrated to a cloud-native architecture using Kubernetes and containerization, allowing for easy scaling and deployment.
- Database sharding and replication: The database was split into smaller shards and replicated across multiple instances, ensuring high availability and performance.
- Caching and CDNs: Caching mechanisms were implemented to reduce the load on the backend, and CDNs were used to distribute static content globally.
- Load balancing and reverse proxies: Load balancing and reverse proxies were used to distribute the traffic across multiple nodes, ensuring that no single point of failure existed.
As a result, the platform was able to handle a 500% increase in traffic without compromsing on performance or reliability.
Conclusion
Designing scalable backend architectures requires a deep understanding of the underlying principles, technologies, and best practices. By following the guidelines and strategies outlined in this article, you can build a robust and efficient system that can handle the growing demands of your users. Remember to continuously monitor, analyze, and optimize your architecture to ensure that it remains scalable, reliable, and high-performing.