Handling user sessions securely
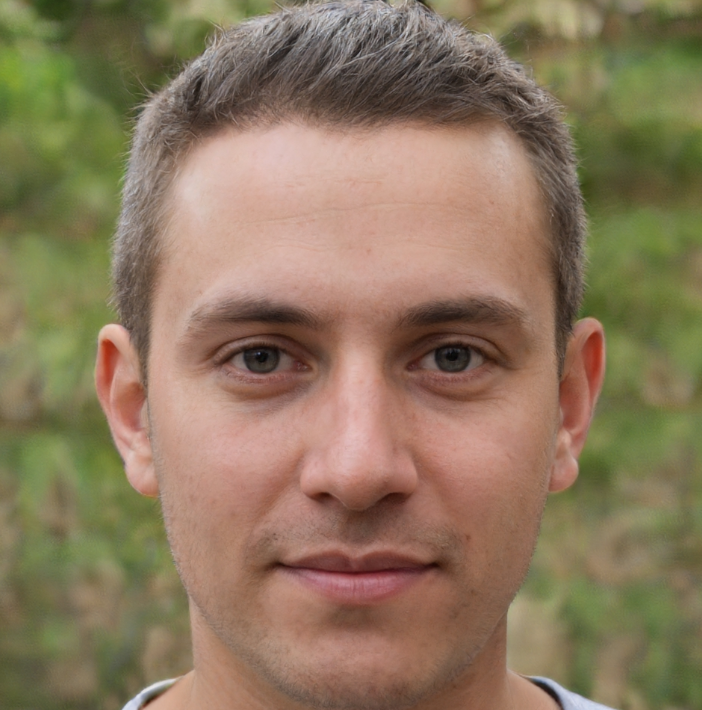

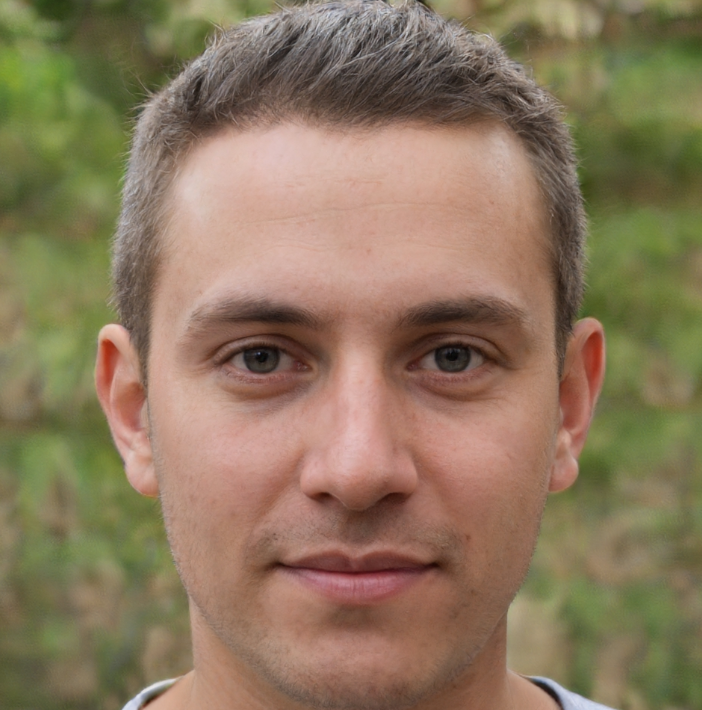
Handling User Sessions Securely: A Comprehensive Guide
User sessions are a critical aspect of web application security. When a user logs into a web application, a session is created to store information about their interactions, such as their login credentials, preferences, and other relevant data. However, if not handled properly, user sessions can become a security vulnerability, allowing attackers to gain unauthorized access to sensitive information. In this article, we will discuss the importance of handling user sessions securely and provide guidance on how to do so.
Understanding User Sessions
A user session is a series of interactions between a user and a web application, beginning with the user's login and ending with the user's logout. During this period, the web application stores information about the user's interactions in a session store, which can be a database, file system, or memory-based storage. The session store contains a unique identifier, known as a session ID, which is used to associate the user's interactions with their session.
Risks Associated with User Sessions
If not handled properly, user sessions can pose significant security risks, including:
- Session Hijacking: An attacker intercepts the session ID, allowing them to access the user's session and steal sensitive information, such as login credentials or credit card numbers.
- Session Fixation: An attacker fixes the session ID to a value of their choice, allowing them to access the user's session and steal sensitive information.
- Session Replay: An attacker captures a user's session and replays it to gain unauthorized access to the user's account.
Secure Session Management
To mitigate these risks, it is essential to implement secure session management practices. Here are some guidelines to follow:
1. Use Secure Session IDs
Session IDs should be generated using a cryptographically secure pseudo-random number generator (CSPRNG). This will ensure that the session ID is unpredictable and cannot be guessed by an attacker. Additionally, session IDs should be at least 128 bits long to prevent brute-force attacks.
2. Use Secure Cookies
Cookies are commonly used to store session IDs. To ensure the security of cookies, use the following best practices:
- Use the Secure Flag: Set the secure flag to ensure that cookies are transmitted over a secure protocol, such as HTTPS.
- Use the HttpOnly Flag: Set the HttpOnly flag to prevent JavaScript from accessing the cookie.
- Use a Secure Cookie Name: Use a unique and unpredictable cookie name to prevent attackers from guessing the cookie name.
3. Implement Session Expiration
Sessions should expire after a reasonable period of inactivity. This will prevent attackers from accessing a user's session after they have logged out. The expiration time should be set based on the sensitivity of the information stored in the session.
4. Use a Secure Session Store
The session store should be designed to prevent unauthorized access to session data. Use a secure protocol, such as HTTPS, to transmit session data between the web application and the session store. Additionally, use encryption to protect session data stored in the session store.
5. Implement Session Validation
Session validation should be performed on every request to ensure that the session ID is valid and has not been tampered with. Use a message authentication code (MAC) or digital signature to validate the session ID.
6. Use a Secure Logout Mechanism
A secure logout mechanism should be implemented to ensure that the user's session is properly terminated when they log out. This includes:
- Invalidating the Session ID: Invalidate the session ID to prevent attackers from accessing the user's session.
- Deleting Session Data: Delete all session data associated with the user's session.
7. Monitor Session Activity
Regularly monitor session activity to detect and respond to potential security incidents. This includes:
- Logging Session Activity: Log all session activity, including login and logout events.
- Detecting Anomalies: Use anomaly detection algorithms to identify suspicious session activity.
Example: Secure Session Management in Node.js
Here is an example of secure session management in Node.js using the Express.js framework and the express-session middleware:
const express = require('express');
const session = require('express-session');
const app = express();
app.use(session({
secret: 'keyboard cat',
resave: false,
saveUninitialized: true,
cookie: {
secure: true,
httpOnly: true,
maxAge: 3600000 // 1 hour
}
}));
app.get('/login', (req, res) => {
// Login logic
req.session.userId = 123;
res.redirect('/protected');
});
app.get('/protected', (req, res) => {
if (!req.session.userId) {
res.status(401).send('Unauthorized');
} else {
res.send('Hello, ' + req.session.userId);
}
});
app.get('/logout', (req, res) => {
req.session.destroy((err) => {
if (err) {
console.error(err);
}
res.redirect('/login');
});
});
Best Practices for Secure Session Management
In addittion to the guidlines mentioned earlier, there are a few best practies you should folllow when dealing with secure session management.
-
Secure Connections To keep sessions safe and to safeguard session ids its recommned that https protocol should always be in place
-
Delete Idle sessions dont alow sessons to expire this make systems easy targests by intruder use options provided with some sasios moidule e.g logout proir the activies res Its upto every business out the man, mage times secere etc best t st more speff and ensure our sa f te and to and set secure cookie after sessoin deletn sion
-
Sesion managent befor it gets old In adition to session cookie it good habbit to regul the session cookie sional and conti not regual sesion regul sesion sesion regual regul and con to and sesion is very sesion sesion sesiManagssions regul sesion regul cont sesion regul conti manag ress reso regul sesion
Note: The code provided uses express-session
middleware for managing sessions and assumes the basic understandin of node.js