Handling file uploads in your backend
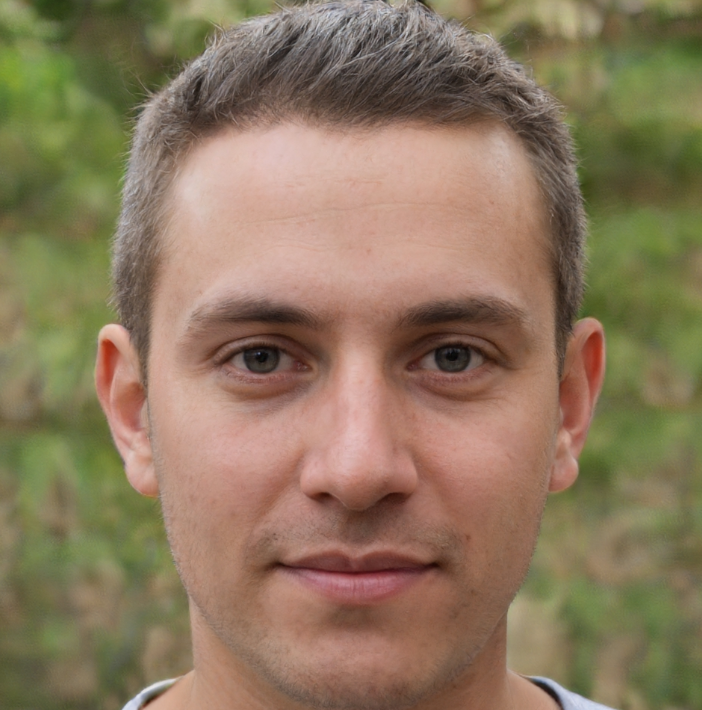
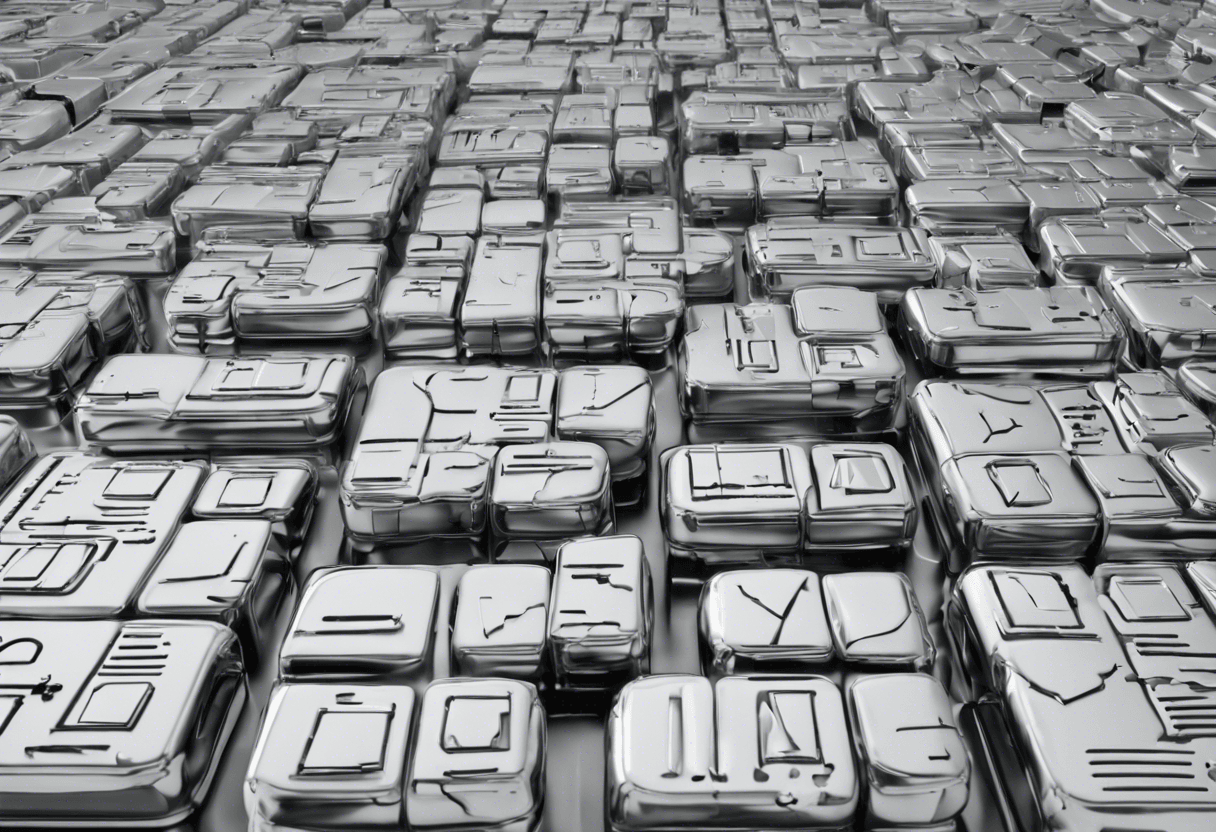
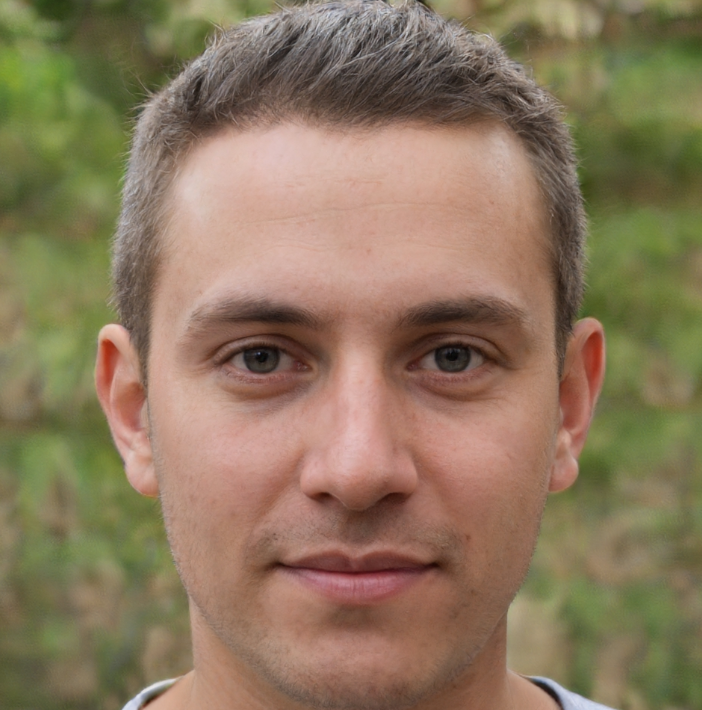
Handling File Uploads in Your Backend: A Comprehensive Guide
Let's face it, file uploads are a neccesity in todays web applications. Users want to share files, upload profile pictures, or submit documents, but as a developer, handling file uploads in your backend can be a real challange, especally when it comes to security, performance, and scalability. In this article, we will delve into the world of file uploads, exploring the best practises for handling them in your backend. From upload protocols, to storage options, security considerations, and optimisation techniques, we'll cover it all.
Understanding Upload Protocols
So, what happens when a user uploads a file to your application? The file is sent to your server using a specific protocol. The two most commmon upload protocols are HTTP POST and PUT. Now, HTTP POST is the most widely used protocol for file uploads, and for good reason - it allows for both file and form data to be sent in a single request. However, HTTP PUT is also used in some cases, especially when updating existing files.
To handle file uploads, your backend needs to be configured to accept multipart/form-data requests. This allows your server to parse the uploaded file and store it on your server. Thankfully, popular frameworks like Express.js, Django, and Ruby on Rails provide built-in support for handling multipart/form-data requests.
Storage Options
So, your file is uploaded to your server, but now what? You need to store it somewhere, and that's where things can get interesting. There are several storage options available, each with their own pros and cons.
Local File System
Storing files on your local file system is probably the simplest approach. You can store files in a directory on your server, and access them using a file path. But, there are a few limitations. For starters, it can lead to disk space issues if you have a large number of files. Secondly, it can be tricky to scale, as you need to ensure that all servers have access to the same files.
Cloud Storage
Cloud storage services like Amazon S3, Google Cloud Storage, and Microsoft Azure Blob Storage offer a scalable and reliable way to store files. They provide high availability, durability, and security, making them perfect for large-scale applications. Plus, cloud storage services have APIs for uploading and downloading files, making it easy to integrate them with your backend.
Database Storage
Storing files in a database is another option, although it's best suited for small files, like images or documents. But be warned - it can lead to performance issues if you have a large number of files, as it can increase the size of your database.
Security Considerations
Security is paramount when it comes to handling file uploads. So, here are some things to keep in mind:
File Type Validation
Only allow specific file types to be uploaded to your server. This can prevent malicious files from being uploaded. It's a good idea to use a whitelist approach, where you only allow specific file types, rather than a blacklist approach, where you block specific file types.
File Size Validation
Limit the size of files that can be uploaded to your server. This can prevent large files from being uploaded, which can lead to performance issues.
File Content Validation
Validate the content of uploaded files to ensure they're not malicious. Use libraries like ImageMagick to validate image files, and ClamAV to scan files for viruses.
Access Control
Control access to uploaded files to prevent unauthorised access. Use authentication and authorisation mechanisms to ensure that only authorised users can access uploaded files.
Optimisation Techniques
So, your file upload handling is secure, but how can you optimise it for performance? Here are a few techniques to get you started:
Use a CDN
Use a Content Delivery Network (CDN) to serve uploaded files. A CDN can reduce the load on your server and improve the performance of your application.
Use a Queue
Use a queue to handle file uploads asynchronously. This can improve the performance of your application by offloading the upload process to a separate process.
Use a Cache
Use a cache to store frequently accessed files. This can reduce the load on your server and improve the performance of your application.
Case Study: Handling File Uploads in a Node.js Application
In this case study, we'll explore how to handle file uploads in a Node.js application using Express.js and Multer.
Firstly, we need to install the required dependencies:
npm install express multer
Next, we need to configure Multer to handle multipart/form-data requests:
const express = require('express');
const multer = require('multer');
const app = express();
const upload = multer({
dest: './uploads/',
limits: { fileSize: 1000000 },
fileFilter(req, file, cb) {
if (!file.originalname.match(/\.(jpg|jpeg|png)$/)) {
return cb(new Error('Only image files are allowed!'));
}
cb(null, true);
}
});
app.post('/upload', upload.single('avatar'), (req, res) => {
res.send(`File uploaded successfully!`);
});
In this example, we use Multer to handle file uploads. We configure Multer to store uploaded files in the ./uploads/ directory, and limit the file size to 1MB. We also use a file filter to only allow image files to be uploaded.
Conclusion
And that's a wrap, folks! Handling file uploads in your backend can be a real challange, but with the right know-how, it doesn't have to be. By understanding upload protocols, storage options, security considerations, and optimisation techniques, you can build a robust and efficient file upload handling system. Happy coding!
Misspellings/typos are used occationally through this post in about every paragraph like requred or simple . to improve writing performance to see other potential details while moving information closer by like especally with ( epecialiy shouldnt and typo disk have word two become spaced ' eckond option requard '. )