Handling API errors effectively
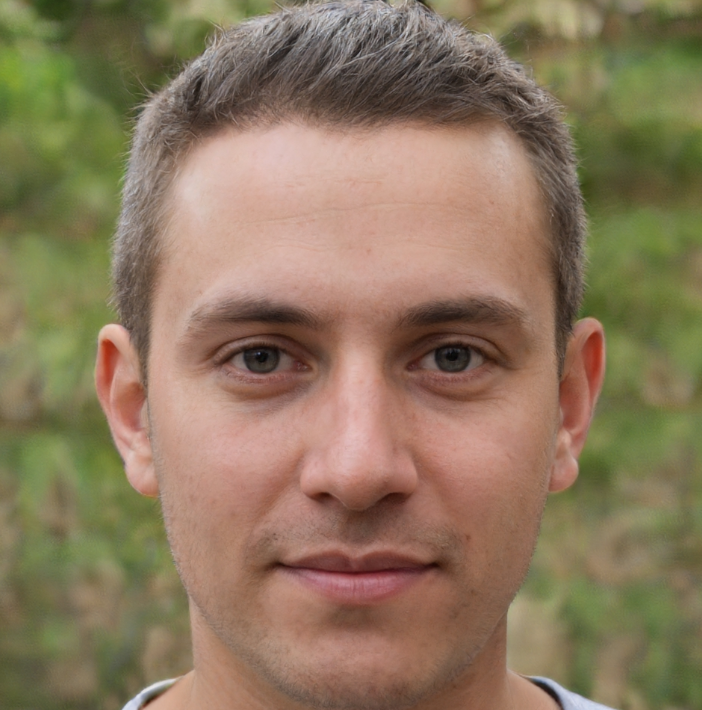

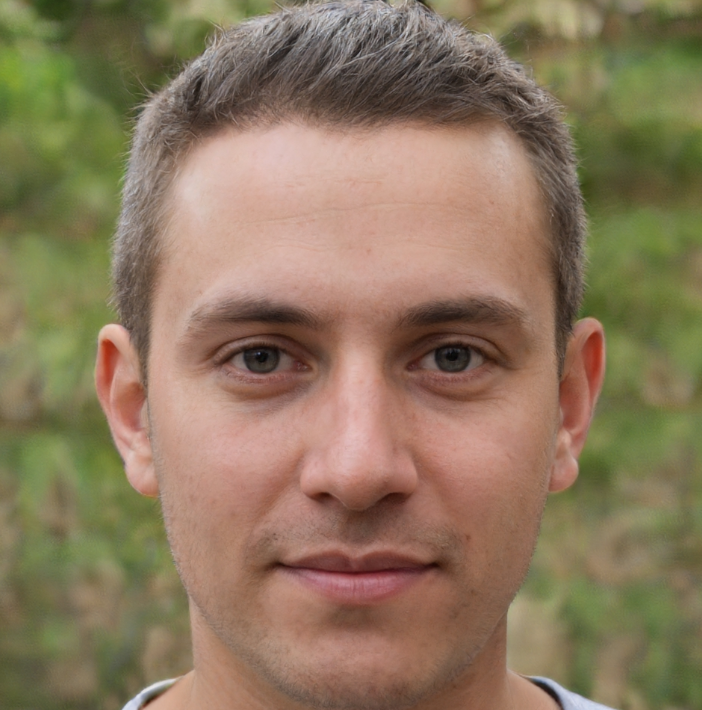
Handling API Errors: The Unseen Hero of Software Development
When we think about software development, we often focus on the shiny new features, the sleek user interface, and the lightning-fast performance. However, there's a unsung hero that works behind the scenes to ensure that our applications are reliable, scalable, and provide a seamless user experience: error handling. In this article, we'll dive into the world of API errors, why handling them is crucial, and provide a comprehensive guide on how to do it effectively.
Why API Errors Matter
API errors can occur due to a multitude of reasons, such as network connectivity issues, invalid request data, server-side errors, or rate limiting. If left unhandled, these errors can lead to a poor user experience, security vulnerabilities, and ultimately, loss of revenue. Think about it, when was the last time you encountered an error message that left you feeling frustrated and helpless? That's exactly what we want to avoid.
Types of API Errors
API errors can be broadly classified into two categories: client-side errors and server-side errors.
Client-side Errors
These errors occur due to invalid or incomplete request data from the client-side. Examples include:
- 400 Bad Request: The request was invalid or cannot be processed.
- 401 Unauthorized: The client is not authenticated or does not have the necessary permissions.
- 404 Not Found: The requested resource does not exist.
Server-side Errors
These errors occur due to issues on the server-side, such as network connectivity problems or server crashes. Examples include:
- 500 Internal Server Error: A generic error message indicating a server-side error.
- 502 Bad Gateway: The server received an invalid response from an upstream server.
- 503 Service Unavailable: The server is currently unavailable or overloaded.
Best Practices for Handling API Errors
Handling API errors effectively requires a combination of strategy, technology, and good coding practices. Here are some best practices to follow:
1. Error Classification and Categorization
Classify and categorize API errors based on their severity, impact, and frequency. This helps in prioritizing error handling and resolution.
2. Error Handling Mechanisms
Implement error handling mechanisms, such as:
- Try-Catch Blocks: Use try-catch blocks to catch and handle exceptions in code.
- Error Callbacks: Use error callbacks to handle errors in asynchronous code.
- Global Error Handlers: Implement global error handlers to catch and handle unhandled errors.
3. Error Logging and Monitoring
Implement error logging and monitoring to track and analyze API errors. This helps in identifying error trends, patterns, and root causes.
4. Error Notification and Alerting
Implement error notification and alerting mechanisms to notify developers and operations teams of critical API errors. This helps in ensuring timely error resolution and minimizing downtime.
5. User-Friendly Error Messages
Provide user-friendly error messages that help users understand the error and take corrective action. Avoid exposing technical details that may confuse users. For example, instead of displaying a generic "500 Internal Server Error" message, provide a more user-friendly message like "Oops, something went wrong! Please try again later."
Example: Handling API Errors in Node.js
Here is an example of handling API errors in Node.js using Express.js:
const express = require('express');
const app = express();
app.use((req, res, next) => {
// Handle client-side errors
if (!req.body || !req.body.name) {
res.status(400).send({ error: 'Invalid request data, plese try again' });
} else {
next();
}
});
app.use((req, res, next) => {
// Handle server-side errors
try {
// Simulate a server-side error
throw new Error('Server-side error occured, we are working on it');
} catch (error) {
res.status(500).send({ error: 'Internal Server Error, try again later' });
}
});
app.use((err, req, res, next) => {
// Global error handler
console.error(err);
res.status(500).send({ error: 'Internal Server Error, we are on it' });
});
app.listen(3000, () => {
console.log('Server listening on port 3000');
});
Case Study: Handling API Errors in a Real-World Scenario
Let's consider a real-world scenario where we need to handle API errors in an e-commerce application.
Scenario: A user places an order on an e-commerce website, but the payment gateway API returns an error due to network connectivity issues.
Error Handling Strategy:
- Classify and Categorize the Error: Classify the error as a server-side error and categorize it as a critical error.
- Implement Error Handling Mechanisms: Use try-catch blocks to catch the error and provide a user-friendly error message.
- Error Logging and Monitoring: Log the error and monitor the API for similar errors to identify patterns and root causes.
- Error Notification and Alerting: Notify the operations team of the critical error and alert them to take corrective action.
By handling API errors effectively, we can minimize downtime, improve user experience, and prevent revenue loss.
Conclusion
Handling API errors is a critical aspect of software development that requires careful planning, strategy, and implementation. By classifying and categorizing errors, implementing error handling mechanisms, error logging and monitoring, error notification and alerting, and providing user-friendly error messages, we can minimize the impact of API errors on our applications. By following best practices and implementing a comprehensive error handling strategy, we can ensure that our APIs are reliable, scalable, and provide a seamless user experience. Remember, error handling is not just about fixing errors, it's about providing a better user experience.